一,建立连接
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<startup>
<supportedRuntime version="v4.0" sku=".NETFramework,Version=v4.7.2" />
</startup>
<connectionStrings><add name="sqlconnection" connectionString="Data Source=WIN-20230216VRB;Initial Catalog=Test01;User ID=sa;Password=jk123"/></connectionStrings>
</configuration>
二,建立一个类:SqlHelper
using System;
using System.Collections.Generic;
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace WindowsFormsApp1
{
class SqlHelper
{
//将sql语句当成参数传递,查询数据库的操作
public static DataSet Query(string sql)
{
//读取app.config中的连接字符串
string sqlconect = ConfigurationManager.ConnectionStrings["sqlConnection"].ToString();
//using(SqlConnection connection = new SqlConnection(ConfigurationManager.ConnectionStrings["sqlConnection"].ConnectionString))
//连接数据库
using (SqlConnection connection = new SqlConnection(sqlconect))
{
//对数据库进行查询操作
SqlDataAdapter dataAdapter = new SqlDataAdapter(sql, connection);
DataSet dataSet = new DataSet();
//将数据库查询到的信息添加到数据集dataSet中
dataAdapter.Fill(dataSet);
return dataSet;
}
}
public static int Execut(string sql)
{//读取app.config中的连接字符串
string sqlconect = ConfigurationManager.ConnectionStrings["sqlConnection"].ToString();
//连接数据库
using (SqlConnection connection = new SqlConnection(sqlconect))
{
//创建命令cmd
SqlCommand cmd = new SqlCommand(sql, connection);
//打开连接
connection.Open();
//对数据库进行增删改操作后的返回值
int returnout = cmd.ExecuteNonQuery();
//关闭连接
connection.Close();
if (returnout > 0)
{
return returnout;
}
else
{
throw new Exception("增删改操作失败");
}
}
}
}
}
三,具体操作
3.1、主窗体From1
3.1.1、主窗体设计
添加控件datagridview
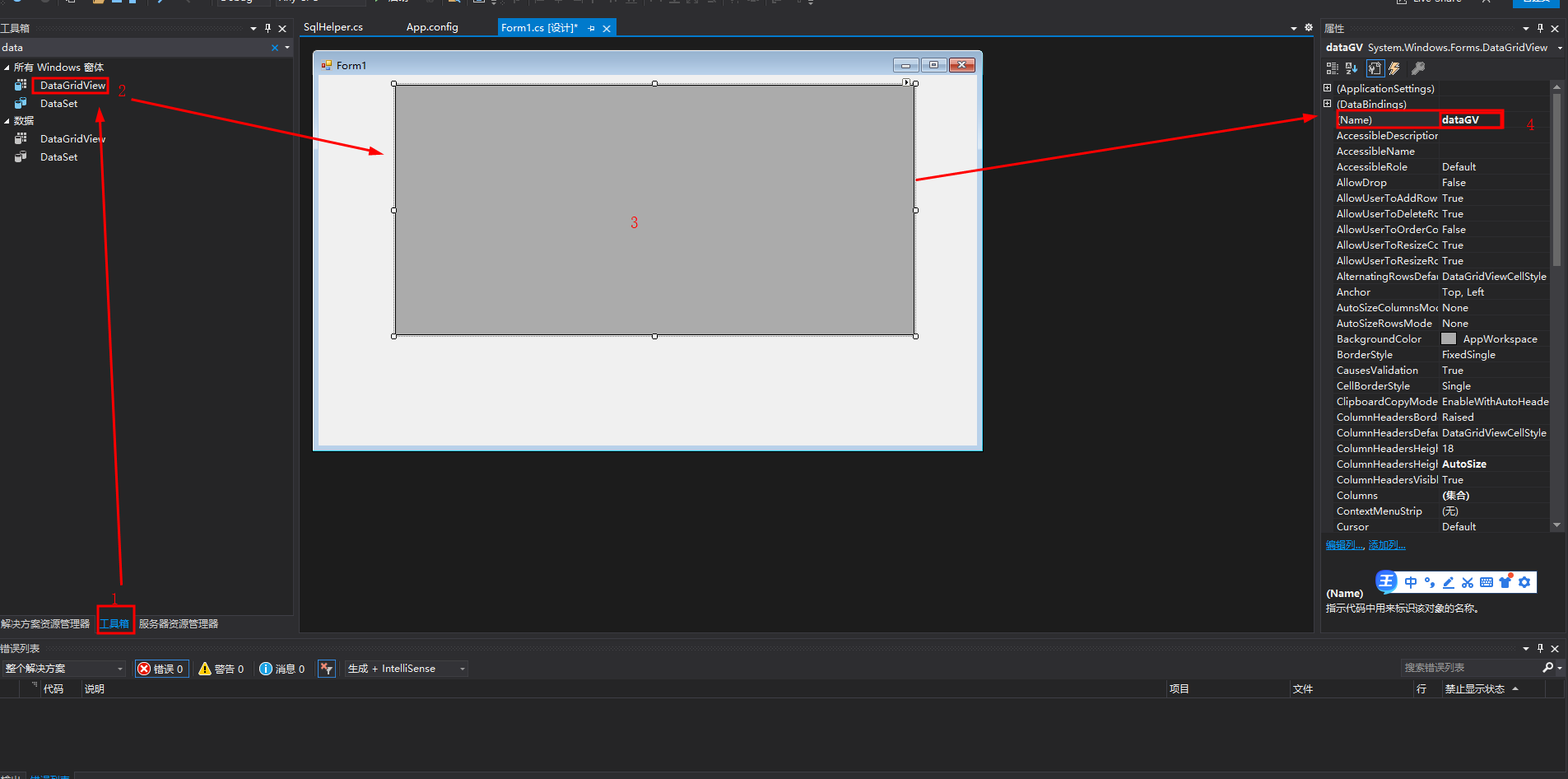
添加控件button,并命名
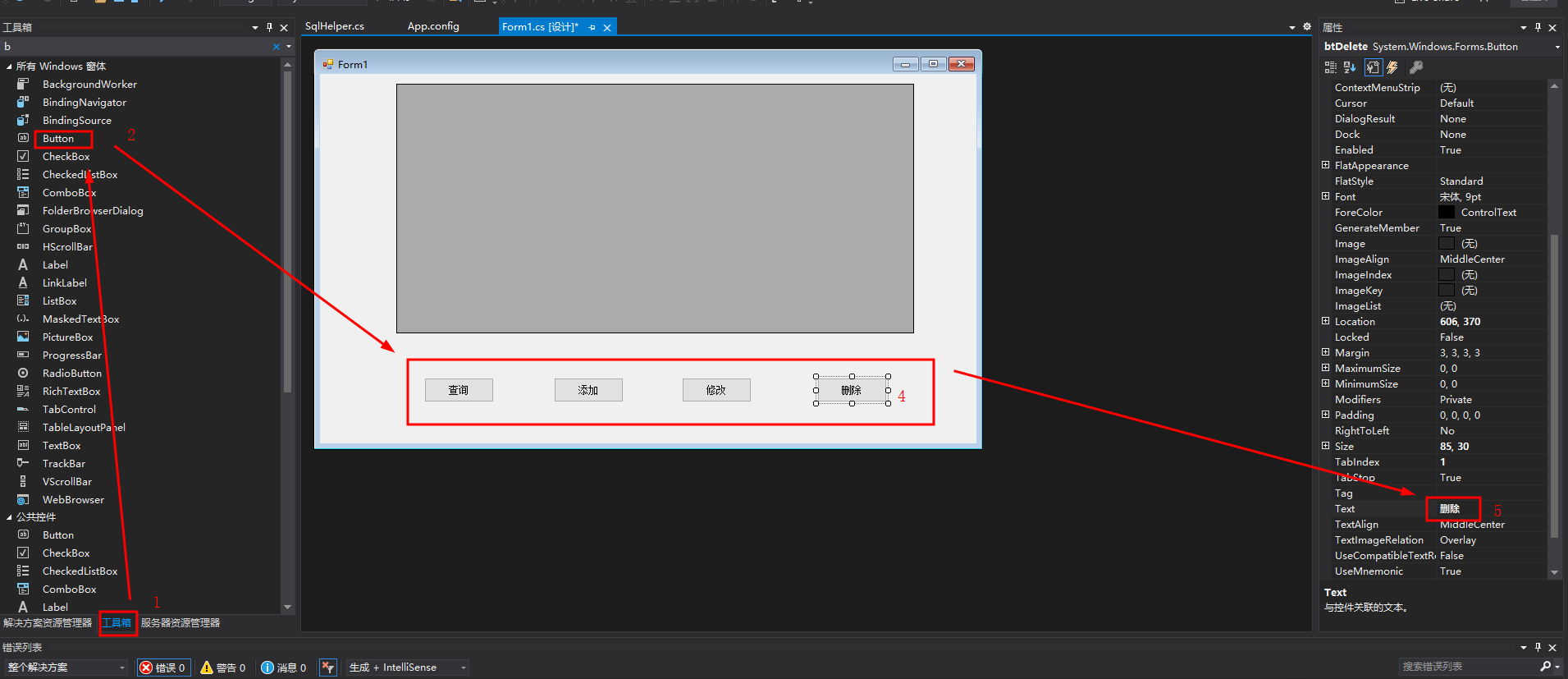
3.1.2、主窗体代码
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WindowsFormsApp1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
public void InitStudent()
{
//查询数据库中的Stuent得到的数据集ds
DataSet ds = SqlHelper.Query($" select * from Student ");
//控件datagridview命名为dataGV,将查询的数据集ds显示在网格dataGV中
dataGV.DataSource = ds.Tables[0];
}
private void Form1_Load(object sender, EventArgs e)
{
InitStudent();
}
//查询按钮
private void btselect_Click(object sender, EventArgs e)
{
InitStudent();
}
//添加按钮
private void btAdd_Click(object sender, EventArgs e)
{
Add add = new Add();
add.ShowDialog(this);
InitStudent();
}
//修改按钮
private void btEdit_Click(object sender, EventArgs e)
{
//获取当前的行
int rowindex = dataGV.CurrentRow.Index;
//将获取到的当前行转为id
int id = Convert.ToInt32(dataGV.Rows[rowindex].Cells[0].Value);
Edit edit = new Edit(id.ToString());
edit.ShowDialog(this);
InitStudent();
}
//删除按钮
private void btDelete_Click(object sender, EventArgs e)
{
//获取当前的行
int rowindex = dataGV.CurrentRow.Index;
//将获取到的当前行转为id
int id = Convert.ToInt32(dataGV.Rows[rowindex].Cells[0].Value);
//进行删除操作
SqlHelper.Execut($"delete from Student where ID ='{id}'");
InitStudent();
}
}
}
3.2、附窗体设计
3.2.1、窗体Add
3.2.1.1、窗体Add设计
窗体Add中添加控件textbox、button、lable并取名、命名
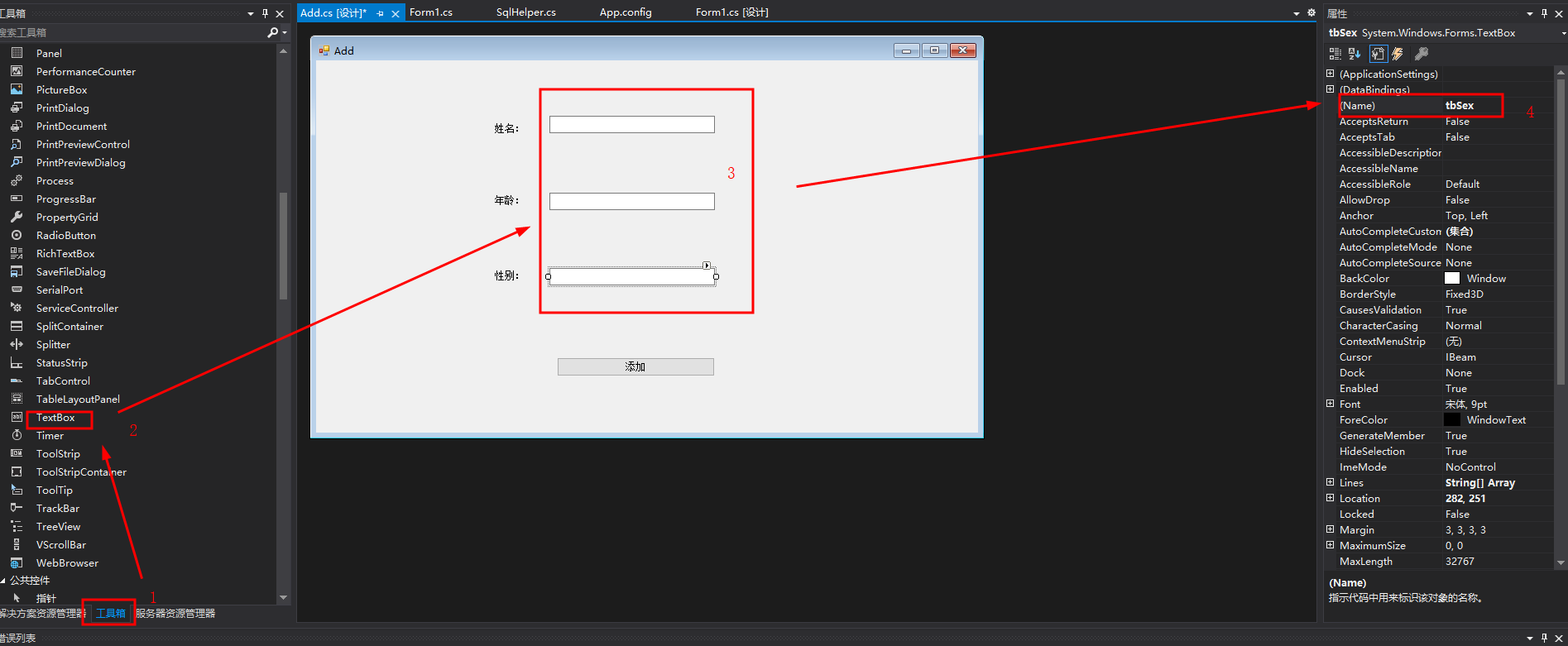
3.2.1.2、窗体Add代码
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WindowsFormsApp1
{
public partial class Add : Form
{
public Add()
{
InitializeComponent();
}
private void button4_Click(object sender, EventArgs e)
{
string name = tbName.Text.ToString();
int age = Convert.ToInt32(tbAge.Text);
string sex = tbSex.Text.ToString();
SqlHelper.Execut($"insert into Student(Sname,Sage,Ssex) values('{name}',{age},'{sex}')");
this.Close();
}
}
}
3.2.2、窗体Edit
3.2.2.1、窗体Edit设计
窗体Edit中添加控件textbox、button、lable并取名、命名
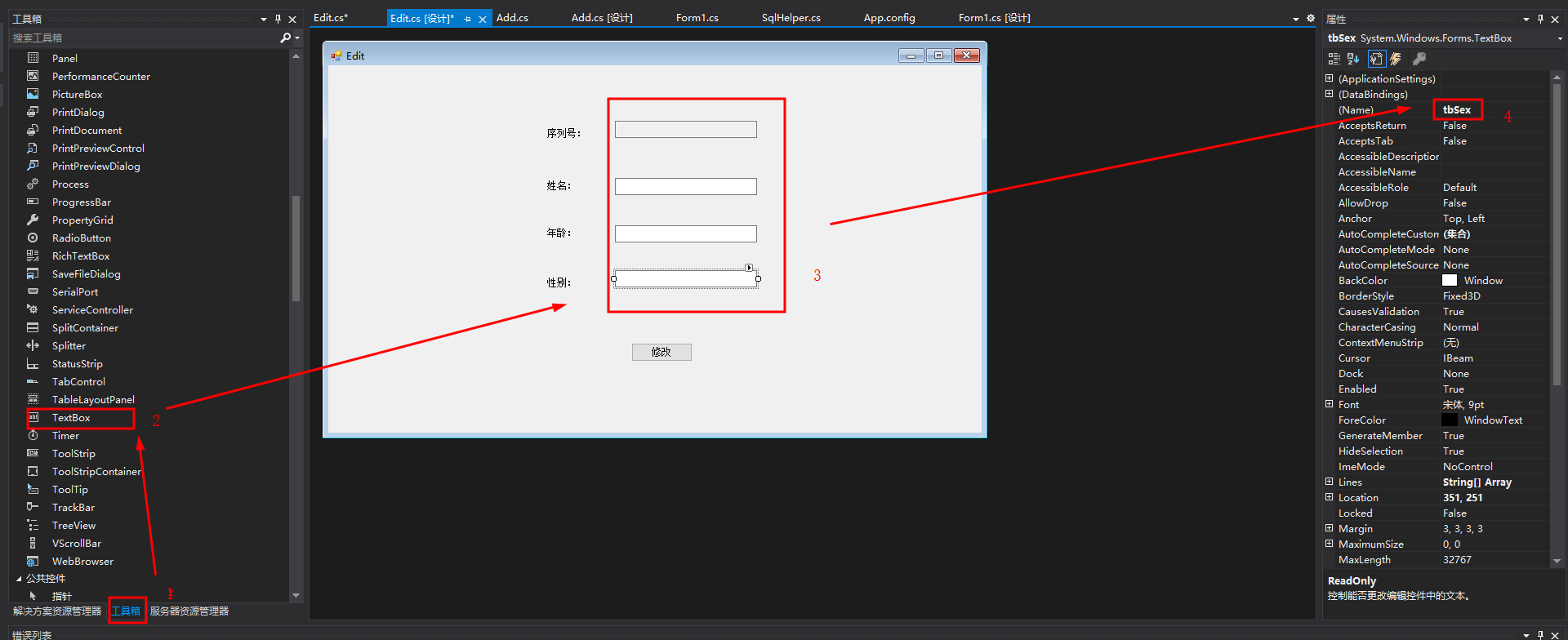
3.2.2.2、窗体Edit代码
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WindowsFormsApp1
{
public partial class Edit : Form
{
//有参构造,传递参数id
public Edit(string id)
{
InitializeComponent();
//窗体的参数id赋值给控件(textbox)tbID,该赋值必须放在 InitializeComponent()初始化语句之后;
this.tbID.Text = id;
}
private void Edit_Load(object sender, EventArgs e)
{
int id = Convert.ToInt32(this.tbID.Text);
DataSet data = SqlHelper.Query($"select * from Student where ID ={id}");
this.tbID.Text = data.Tables[0].Rows[0]["ID"].ToString();
this.tbName.Text = data.Tables[0].Rows[0]["Sname"].ToString();
this.tbAge.Text = data.Tables[0].Rows[0]["Sage"].ToString();
this.tbSex.Text = data.Tables[0].Rows[0]["Ssex"].ToString();
}
private void btEdit_Click(object sender, EventArgs e)
{
int ID = int.Parse(this.tbID.Text);
string name = this.tbName.Text;
int age = int.Parse(this.tbAge.Text);
string sex = this.tbSex.Text;
SqlHelper.Execut($"update Student set Sname ='{name}', Sage={age} , Ssex = '{sex}' where ID = {ID} ");
this.Close();
}
}
}
四,数据库
数据库中Student表设计
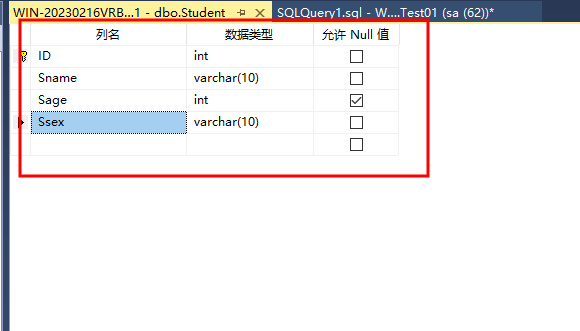
五,整体结构及其效果展示
5.1、整体结构
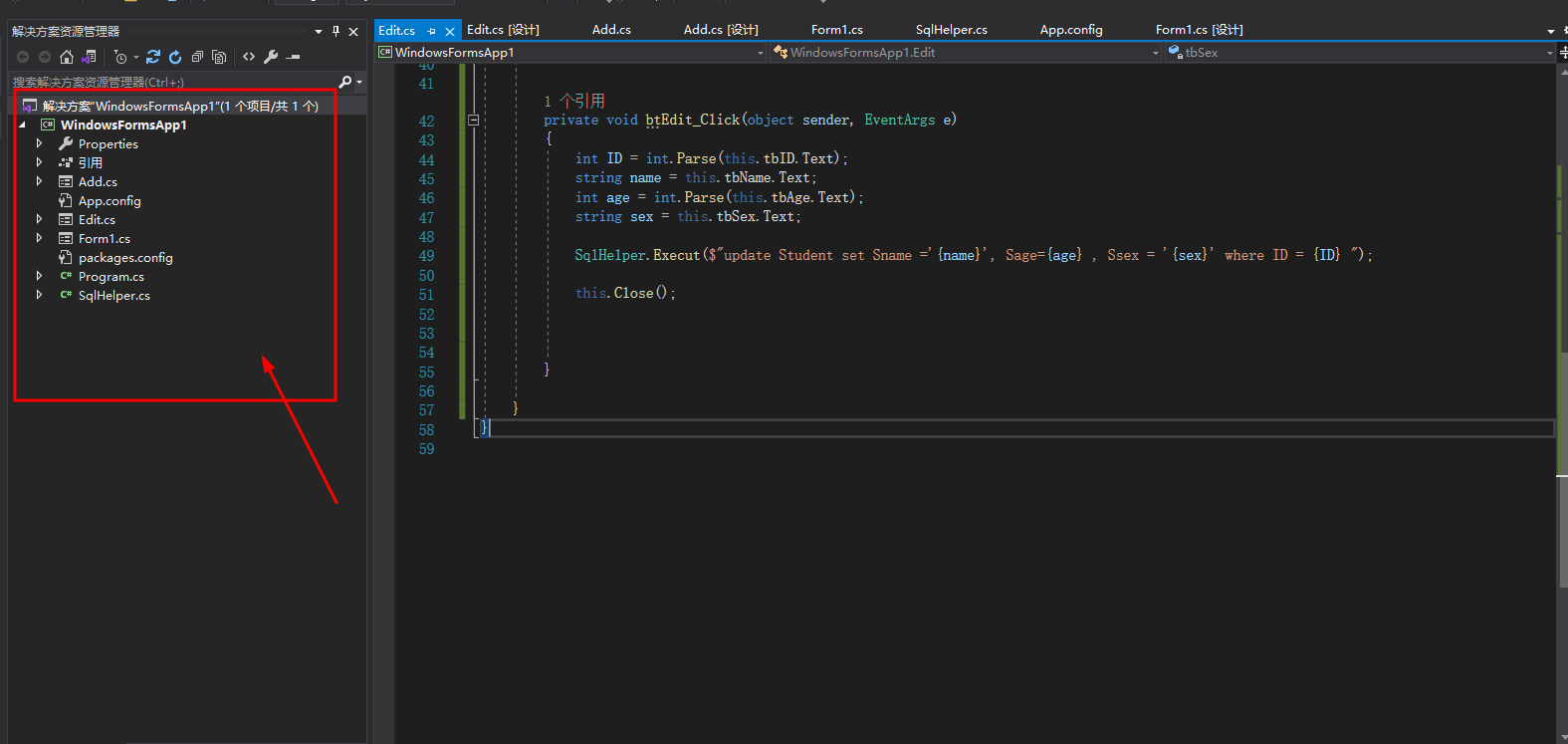
5.2、效果展示
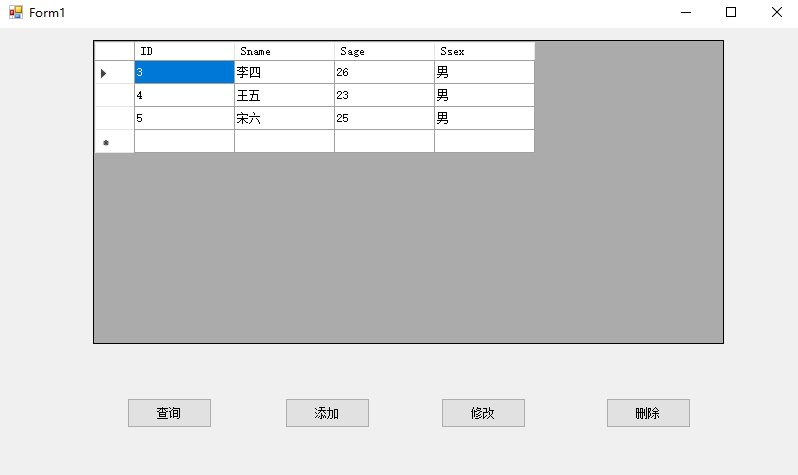