1.引入pom
<!-- 引入天翼云sts服务 -->
<dependency>
<groupId>com.amazonaws</groupId>
<artifactId>aws-java-sdk-s3</artifactId>
<version>1.11.336</version>
</dependency>
<dependency>
<groupId>com.amazonaws</groupId>
<artifactId>aws-java-sdk-sts</artifactId>
<version>1.11.336</version>
</dependency>
2.yml配置
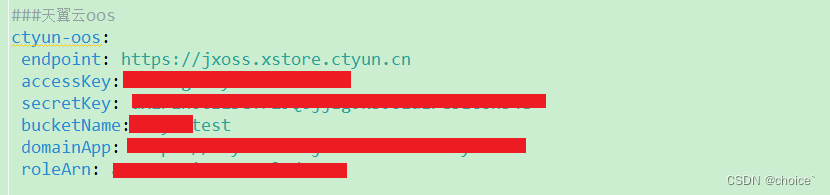
3.天翼云基础配置
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class CtyunConfig
{
@Value("${ctyun-oos.accessKey}")
private String accessKey;
@Value("${ctyun-oos.secretKey}")
private String secretKey;
@Value("${ctyun-oos.endpoint}")
private String endpoint;
@Value("${ctyun-oos.bucketName}")
private String bucketName;
@Value("${ctyun-oos.domainApp}")
private String domainApp;
@Value("${ctyun-oos.roleArn}")
private String roleArn;
public String getAccessKey()
{
return accessKey;
}
public void setAccessKey(String accessKey)
{
this.accessKey = accessKey;
}
public String getSecretKey()
{
return secretKey;
}
public void setSecretKey(String secretKey)
{
this.secretKey = secretKey;
}
public String getEndpoint()
{
return endpoint;
}
public void setEndpoint(String endpoint)
{
this.endpoint = endpoint;
}
public String getBucketName()
{
return bucketName;
}
public void setBucketName(String bucketName)
{
this.bucketName = bucketName;
}
public String getDomainApp()
{
return domainApp;
}
public void setDomainApp(String domainApp)
{
this.domainApp = domainApp;
}
public String getRoleArn()
{
return roleArn;
}
public void setRoleArn(String roleArn)
{
this.roleArn = roleArn;
}
}
4.天翼云存储工具类
import org.apache.commons.io.IOUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.ClientConfiguration;
import com.amazonaws.auth.AWSStaticCredentialsProvider;
import com.amazonaws.auth.BasicAWSCredentials;
import com.amazonaws.client.builder.AwsClientBuilder.EndpointConfiguration;
import com.amazonaws.regions.Regions;
import com.amazonaws.services.s3.AmazonS3;
import com.amazonaws.services.s3.AmazonS3ClientBuilder;
import com.amazonaws.services.s3.model.CannedAccessControlList;
import com.amazonaws.services.s3.model.PutObjectRequest;
import com.amazonaws.services.s3.model.PutObjectResult;
import com.amazonaws.services.s3.transfer.TransferManager;
import com.amazonaws.services.s3.transfer.TransferManagerBuilder;
import com.amazonaws.services.s3.transfer.Upload;
import com.amazonaws.services.s3.transfer.model.UploadResult;
import com.booway.cloud.model.OssFileModel;
import com.booway.course.file.config.CtyunConfig;
@Component
public class CtyunUtil
{
private static final Logger log = LoggerFactory.getLogger(CtyunUtil.class);
public static final String POINT = ".";
public static final String SLASH = "/";
@Autowired
private CtyunConfig ctyunConfig;
private AmazonS3 getClient()
{
BasicAWSCredentials credentials = new BasicAWSCredentials(ctyunConfig.getAccessKey(), ctyunConfig.getSecretKey());
ClientConfiguration clientConfiguration = new ClientConfiguration();
clientConfiguration.setMaxConnections(50);
clientConfiguration.setSocketTimeout(50 * 1000);
clientConfiguration.setConnectionTimeout(50 * 1000);
EndpointConfiguration endpointConfiguration = new EndpointConfiguration(ctyunConfig.getEndpoint(), Regions.DEFAULT_REGION.getName());
AmazonS3 oosClient = AmazonS3ClientBuilder.standard()
.withClientConfiguration(clientConfiguration)
.withCredentials(new AWSStaticCredentialsProvider(credentials))
.withEndpointConfiguration(endpointConfiguration).build();
return oosClient;
}
public Credentials createStsToken(String bucketName) throws Exception
{
if(!doesBucketExist(bucketName)) {
throw new Exception("bucket不存在");
}
AmazonS3 oosClient = getClient();
try
{
String POLICY = "{\"Version\":\"2012-10-17\"," + "\"Statement\":" + "{\"Effect\":\"Allow\"," + "\"Action\":[\"s3:*\"],"
+ "\"Resource\":[\"arn:aws:s3:::" + bucketName + "\",\"arn:aws:s3:::" + bucketName + "/*\"]" + "}}";
AWSSecurityTokenService stsClient = buildSTSClient();
AssumeRoleRequest assumeRoleRequest = new AssumeRoleRequest();
assumeRoleRequest.setRoleArn(ctyunConfig.getRoleArn());
assumeRoleRequest.setPolicy(POLICY);
assumeRoleRequest.setRoleSessionName("session-name");
assumeRoleRequest.setDurationSeconds(43200);
AssumeRoleResult assumeRoleRes = stsClient.assumeRole(assumeRoleRequest);
Credentials stsCredentials = assumeRoleRes.getCredentials();
return stsCredentials;
}
catch (Exception e)
{
log.error(e.getMessage());
e.printStackTrace();
}
finally
{
if (oosClient != null)
{
oosClient.shutdown();
}
}
return null;
}
private AWSSecurityTokenService buildSTSClient()
{
BasicAWSCredentials credentials = new BasicAWSCredentials(ctyunConfig.getAccessKey(), ctyunConfig.getSecretKey());
AwsClientBuilder.EndpointConfiguration endpointConfiguration = new AwsClientBuilder.EndpointConfiguration(ctyunConfig.getEndpoint(), Regions.DEFAULT_REGION.getName());
return AWSSecurityTokenServiceClientBuilder.standard().withCredentials(new AWSStaticCredentialsProvider(credentials)).withEndpointConfiguration(endpointConfiguration).build();
}
public String generatePresignedUrl(String bucketName, String objectName) throws Exception
{
if(!doesBucketExist(bucketName)) {
throw new Exception("bucket不存在");
}
if(!doesObjectExist(bucketName, objectName)) {
throw new Exception("文件不存在");
}
AmazonS3 oosClient = getClient();
try
{
LocalDateTime expirationDateTime = LocalDateTime.now().plusSeconds(60 * 60);
Date expiration = Date.from(expirationDateTime.atZone(ZoneId.systemDefault()).toInstant());
GeneratePresignedUrlRequest generatePresignedUrlRequest = new GeneratePresignedUrlRequest(bucketName, objectName).withMethod(HttpMethod.GET).withExpiration(expiration);
URL url = oosClient.generatePresignedUrl(generatePresignedUrlRequest);
return url.toString();
}
catch (Exception e)
{
log.error(e.getMessage());
e.printStackTrace();
}
finally
{
if (oosClient != null)
{
oosClient.shutdown();
}
}
return null;
}
public Boolean doesBucketExist(String bucketName)
{
AmazonS3 oosClient = getClient();
try
{
return oosClient.doesBucketExistV2(bucketName);
}
catch (Exception e)
{
log.error(e.getMessage());
e.printStackTrace();
}
finally
{
if (oosClient != null)
{
oosClient.shutdown();
}
}
return false;
}
public Boolean doesObjectExist(String bucketName, String objectName)
{
AmazonS3 oosClient = getClient();
try
{
return oosClient.doesObjectExist(bucketName, objectName);
}
catch (Exception e)
{
log.error(e.getMessage());
e.printStackTrace();
}
finally
{
if (oosClient != null)
{
oosClient.shutdown();
}
}
return false;
}
public OssFileModel uploadFile(String fileName, InputStream fileIns, String storagePath)
{
AmazonS3 oosClient = getClient();
UploadResult partUploadFileResult = null;
PutObjectResult putObjectResult = null;
try
{
String bucketName = ctyunConfig.getBucketName();
log.info("bucketName={}",bucketName);
if (!oosClient.doesBucketExistV2(bucketName))
{
oosClient.createBucket(bucketName);
}
int fileSize = fileIns.available();
String contentType = Util.getContentType(fileName);
boolean publicRead = contentType.startsWith("image") || contentType.startsWith("application") || contentType.startsWith("text") || contentType.startsWith("ts") || contentType.startsWith("m3u8");
if (fileSize > 104857600)
{
partUploadFileResult = partUploadFile(fileIns, storagePath, publicRead);
}
else
{
PutObjectRequest req = new PutObjectRequest(bucketName, storagePath, fileIns, null);
if (publicRead)
{
req.setCannedAcl(CannedAccessControlList.PublicRead);
}
putObjectResult = oosClient.putObject(req);
}
if (partUploadFileResult != null || putObjectResult != null)
{
String suffix = fileName.substring(fileName.lastIndexOf(".") + 1);
String eTag = partUploadFileResult != null ? partUploadFileResult.getETag() : putObjectResult.getETag();
OssFileModel ossFileModel = OssFileModel.builder().fileName(fileName).fileSize(fileSize).cloudAbsolutePath(storagePath).cloudPath(ctyunConfig.getDomainApp() + SLASH + storagePath).fileSuffix(suffix).fileBucket(bucketName).md5(eTag).build();
log.info("上传成功===>>>返回对象={}",com.alibaba.fastjson.JSONObject.toJSONString(ossFileModel));
return ossFileModel;
}
}
catch (AmazonServiceException e)
{
e.printStackTrace();
}
catch (Exception e)
{
e.printStackTrace();
}
finally
{
if (oosClient != null)
{
oosClient.shutdown();
}
if (fileIns != null)
{
IOUtils.closeQuietly(fileIns);
}
}
return null;
}
private UploadResult partUploadFile(InputStream fileIns, String storagePath, boolean publicRead)
{
AmazonS3 oosClient = getClient();
try
{
TransferManager tm = TransferManagerBuilder.standard().withS3Client(oosClient).build();
PutObjectRequest request = new PutObjectRequest(ctyunConfig.getBucketName(), storagePath, fileIns, null);
if (publicRead)
{
request.withCannedAcl(CannedAccessControlList.PublicRead);
}
Upload upload = tm.upload(request);
UploadResult result = upload.waitForUploadResult();
return result;
}
catch (AmazonServiceException e)
{
e.printStackTrace();
}
catch (Exception e)
{
e.printStackTrace();
}
finally
{
if (oosClient != null)
{
oosClient.shutdown();
}
if (fileIns != null)
{
IOUtils.closeQuietly(fileIns);
}
}
return null;
}
5.补充 OssFileModel
import lombok.Builder;
import lombok.Data;
@Data
@Builder
public class OssFileModel
{
private String fileName;
private long fileSize;
private String cloudAbsolutePath;
private String cloudPath;
private String fileSuffix;
private String fileBucket;
private String md5;
public OssFileModel()
{
}
public OssFileModel(String fileName, long fileSize, String cloudAbsolutePath, String cloudPath, String fileSuffix,
String fileBucket, String MD5)
{
super();
this.fileName = fileName;
this.fileSize = fileSize;
this.cloudAbsolutePath = cloudAbsolutePath;
this.cloudPath = cloudPath;
this.fileSuffix = fileSuffix;
this.fileBucket = fileBucket;
this.md5 = MD5;
}
}