效果图
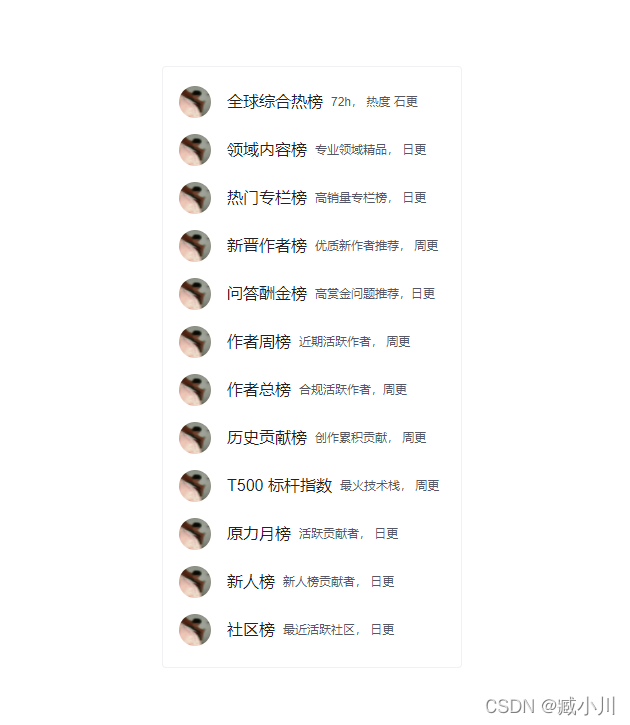
JS
import React, { useState } from 'react'
import styles from './style.less'
const contentStyle = {
color: '#fc5531'
}
const yus = 'https://www.dataojocloud.com/dataeye/v1/data/image/get?imageid=617a5596fd73380b818dc300'
const gy = 'https://www.dataojocloud.com/dataeye/v1/data/image/get?imageid=617a559d2ee1bd5a04779569'
const contentData = [
{ name: '全球综合热榜', text: '72h, 热度 石更', id: 1 },
{ name: '领域内容榜', text: '专业领域精品, 日更', id: 2 },
{ name: '热门专栏榜', text: '高销量专栏榜, 日更', id: 3 },
{ name: '新晋作者榜', text: '优质新作者推荐, 周更', id: 4 },
{ name: '问答酬金榜', text: '高赏金问题推荐,日更', id: 5 },
{ name: '作者周榜', text: '近期活跃作者, 周更', id: 6 },
{ name: '作者总榜', text: '合规活跃作者,周更', id: 7 },
{ name: '历史贡献榜', text: '创作累积贡献, 周更', id: 8 },
{ name: 'T500 标杆指数', text: '最火技术栈, 周更', id: 9 },
{ name: '原力月榜', text: '活跃贡献者, 日更', id: 10 },
{ name: '新人榜', text: '新人榜贡献者, 日更', id: 11 },
{ name: '社区榜', text: '最近活跃社区, 日更', id: 12 },
]
const index = () =>
{
const [active, setActive] = useState(null)
const [activeClick, setActiveClick] = useState(null)
const enterActive = (id) =>
{
setActive(id)
}
const leaveActive = () =>
{
setActive(false)
}
const clickActive = (id) =>
{
setActiveClick(id)
}
return (
<div className={styles.container}>
<ul onMouseLeave={leaveActive}>
{contentData.map((data, index) => (
<li className={styles.listItem} key={index} onClick={() => clickActive(data.id)} onMouseOver={() => enterActive(data.id)}>
<div className={styles.itemBox}>
<img src={active === data.id ? gy : activeClick === data.id ? gy : yus} alt="" />
</div>
<div className={styles.itemContent}>
<div style={active === data.id ? contentStyle : activeClick === data.id ? contentStyle : null} className={styles.text}>{data.name}</div>
<div className={styles.dec}>{data.text}</div>
</div>
</li>
))
}
</ul>
</div >
)
}
export default index
CSS
body {
margin: 0;
padding: 0;
-webkit-box-sizing: border-box;
box-sizing: border-box;
font-family: PingFang SC, Hiragino Sans GB, Arial, Microsoft YaHei, Verdana, Roboto, Noto, Helvetica Neue, sans-serif;
}
ul {
padding: 0;
margin: 0;
list-style-type: none;
}
.container {
margin: 100px auto;
width: 300px;
img {
width: 100%;
height: 100%;
}
ul {
background: #fff;
border-radius: 4px;
padding-top: 8px;
padding-bottom: 16px;
border: 1px solid #f0f0f5;
}
li {
display: flex;
align-items: center;
-webkit-box-align: center;
}
.listItem {
clear: both;
margin-top: 6px;
cursor: pointer;
}
}
.itemBox {
margin: 0 16px;
width: 32px;
height: 32px;
border-radius: 50%;
overflow: hidden;
}
.itemContent {
display: flex;
align-items: center;
flex: 1;
padding-top: 10px;
padding-bottom: 10px;
margin-right: 14px;
.text {
font-size: 16px;
font-weight: 500;
color: #222226;
line-height: 22px;
margin-right: 8px;
}
.dec {
font-size: 12px;
color: #555666;
}
}