linux实现一个线程间的合作处理。
对于线程c,p。。。。c是消费者,p是生产者。
生产一批数据然后消费。每次得有阻塞等待线程锁被唤醒。这里的参数就不是数据了而是一个执行流
代码实现如下:
Task.hpp代码
1 #include <iostream>
2 using namespace std;
3
4
5 class Task
6 {
7 public:
8 Task(int x,int y,char op)
9 :_x(x)
10 ,_y(y)
11 ,_op(op)
12 {}
13 Task()
14 {}
15
16 void Run()
17 {
18 char p=_op;
19 int ret=0;
20 switch(p)
21 {
22 case '+':
23 ret=_x+_y;
24 break;
25 case '-':
26 ret=_x-_y;
27 break;
28 case '*':
29 ret=_x*_y;
30 break;
31 case '/':
32 if(_y==0)
33 {
34 cout<<"除数为0,错误"<<endl;
35 ret=-1;
36 }
37 else
38 ret=_x/_y;
39 break;
40 default:
41 break;
42 }
43 cout<<_x<<p<<_y<<"="<<ret<<endl;
44 }
45
46 ~Task()
47 {}
48
49 private:
50 int _x;
51 int _y;
52 char _op;
53 };
BlockQueue.hpp不变
main.cc代码
1 #include "BlockQueue.hpp"
2 #include "task.hpp"
3
4 void* Constum(void* arg) //消费数据
5 {
6 auto bq = (BlockQueue<Task>*)arg;
7 while(1)
8 {
9 sleep(2);
10 Task t;
11 bq->Pop(t);
12 t.Run();
13 cout<<"constum: "<< "消费数据了" <<endl;
14 }
15 }
16
17 void* Producer(void* arg)//生产数据
18 {
19 auto bq = (BlockQueue<Task>*)arg;
20 char arr[]="+-*/";
21 while(1)
22 {
23 int x =rand()%100+1;
24 int y = rand()%50;
25 char op=arr[rand()%4];
26 Task t(x,y,op);
27 bq->Push(t);
28 cout<<"producter: "<< "生产了数据" << endl;
29 }
30 }
31
32 int main()
33 {
34 srand((unsigned int)time(nullptr));
35 pthread_t c,p;
linux实现一个线程间的合作处理。
对于线程c,p。。。。c是消费者,p是生产者。
生产一批数据然后消费。每次得有阻塞等待线程锁被唤醒。
代码实现如下:
BlockQueue.hpp代码
1 #pragma once
2
3 #include <iostream>
4 using namespace std;
5 #include <pthread.h>
6 #include <cstdio>
7 #include <queue>
8 #include <ctime>
9 #include <cstdlib>
10 #include <unistd.h>
11
12 #define NUM 10
13
14 template <class T>
15 class BlockQueue
16 {
17 private:
18 bool IsFull()
19 {
20 if(q.size()==cap)
21 {
22 return true;
23 }
24 else
25 return false;
26 }
27 bool IsEmpty()
28 {
29 return q.size()==0;
30 }
31 public:
32 BlockQueue(int _cap=NUM):cap(_cap)
33 {
34 pthread_mutex_init(&lock,nullptr);
35 pthread_cond_init(&_full,nullptr);
36 pthread_cond_init(&_empty,nullptr);
37 }
38
39 ~BlockQueue()
40 {
41 pthread_mutex_destroy(&lock);
42 pthread_cond_destroy(&_full);
43 pthread_cond_destroy(&_empty);
44 }
45
46 void Push(const T& pu)
47 {
48 pthread_mutex_lock(&lock);
49 while(IsFull()) //万一是多线程调用,如果两个及其多个同时觉得满了,只有一个竞争上就会有伪唤醒,所以得while
50 {
51 pthread_cond_wait(&_full,&lock);
52 }
53 q.push(pu);
54 pthread_mutex_unlock(&lock);
55
56 //走到这里就是已经有数据了,唤醒pop
57 if(q.size()>=cap/2)
58 {
59 cout<<"数据已经很多了,快来消费"<<endl;
60 pthread_cond_signal(&_empty);
61 }
62 }
63
64 void Pop(T& po)
65 {
66 pthread_mutex_lock(&lock);
67 if(IsEmpty())
68 {
69 pthread_cond_wait(&_empty,&lock);
70
71 }
72 po=q.front();
73 q.pop();
74 pthread_mutex_unlock(&lock);
75 while(q.size()<=cap/2)
76 {
77 cout<<"数据已经很少了,快来生产"<<endl;
78 pthread_cond_signal(&_full);
79 }
80 }
81
82 private:
83 queue<T> q;
84 int cap; //队列数据个数
85 pthread_mutex_t lock;
86 pthread_cond_t _full;
87 pthread_cond_t _empty;
88
89 };
main代码
1 #include "BlockQueue.hpp"
2
3 void* Constum(void* arg) //消费数据
4 {
5 auto bq = (BlockQueue<int>*)arg;
6 while(1)
7 {
8 sleep(2);
9 int data=0;
10 bq->Pop(data);
11 cout<<"constum: "<< data <<endl;
12 }
13 }
14
15 void* Producer(void* arg)//生产数据
16 {
17 auto bq = (BlockQueue<int>*)arg;
18 while(1)
19 {
20 int data=rand()%100+1;
21 bq->Push(data);
22 cout<<"producter: "<< data << endl;
23 }
24 }
25
26 int main()
27 {
28 srand((unsigned int)time(nullptr));
29 pthread_t c,p;
30 BlockQueue<int> *bq = new BlockQueue<int>();
31 pthread_create(&c,nullptr,Constum,bq);
32 pthread_create(&p,nullptr,Producer,bq);
33
34 pthread_join(c,nullptr);
35 pthread_join(p,nullptr);
36
37 return 0;
38 }
39
``''
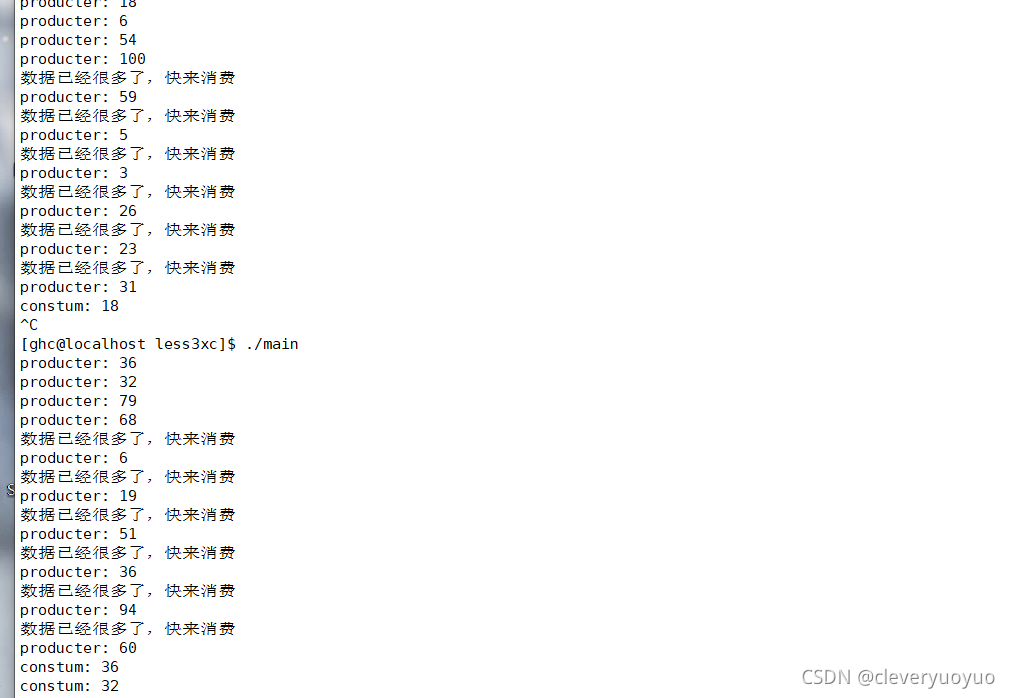