一、pom.xml配置
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.8</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.9.9.3</version>
<scope>compile</scope>
</dependency>
</dependencies>
二、application.properties配置
spring.redis.host=10.6.3.148
spring.redis.port=6379
spring.redis.password=
spring.cache.redis.time-to-live=100000
三、示例代码
@SpringBootApplication
@EnableCaching
public class CacheApplication {
public static void main(String[] args) {
SpringApplication.run(CacheApplication.class, args);
}
@Bean
public RedisCacheConfiguration redisCacheConfiguration(CacheProperties cacheProperties) {
CacheProperties.Redis redisProperties = cacheProperties.getRedis();
RedisCacheConfiguration config = RedisCacheConfiguration.defaultCacheConfig();
config = config.serializeValuesWith(RedisSerializationContext.SerializationPair.fromSerializer(valueSerializer()));
if (redisProperties.getTimeToLive() != null) {
config = config.entryTtl(redisProperties.getTimeToLive());
}
if (redisProperties.getKeyPrefix() != null) {
config = config.prefixKeysWith(redisProperties.getKeyPrefix());
}
if (!redisProperties.isCacheNullValues()) {
config = config.disableCachingNullValues();
}
if (!redisProperties.isUseKeyPrefix()) {
config = config.disableKeyPrefix();
}
return config;
}
private RedisSerializer<Object> valueSerializer() {
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY);
objectMapper.enableDefaultTyping(ObjectMapper.DefaultTyping.NON_FINAL);
return new GenericJackson2JsonRedisSerializer(objectMapper);
}
}
@Data
public class User implements Serializable {
private String id;
private String name;
private String age;
private String phoneNumber;
}
@Service
public class UserManager {
@Cacheable(value = "user", key = "#id")
public User getUser(String id) {
User user = new User();
user.setId(id);
user.setName("kk_cock");
user.setAge("18");
user.setPhoneNumber("13568987450");
return user;
}
@CacheEvict(value = "user", key = "#id")
public void delete(String id) {
}
@CachePut(value = "user", key = "#result.id")
public User update(User user) {
user.setName("jj");
return user;
}
}
@RunWith(SpringRunner.class)
@SpringBootTest
public class CacheApplicationTests {
@Autowired
private UserManager userManager;
@Test
public void contextLoads() {
String id = "123456";
User user = userManager.getUser(id);
String id2 = "654321";
User user2 = userManager.getUser(id2);
userManager.update(user);
System.out.println(userManager.getUser(id) == user);
System.out.println(userManager.getUser(id2) == user2);
}
}
四、执行结果
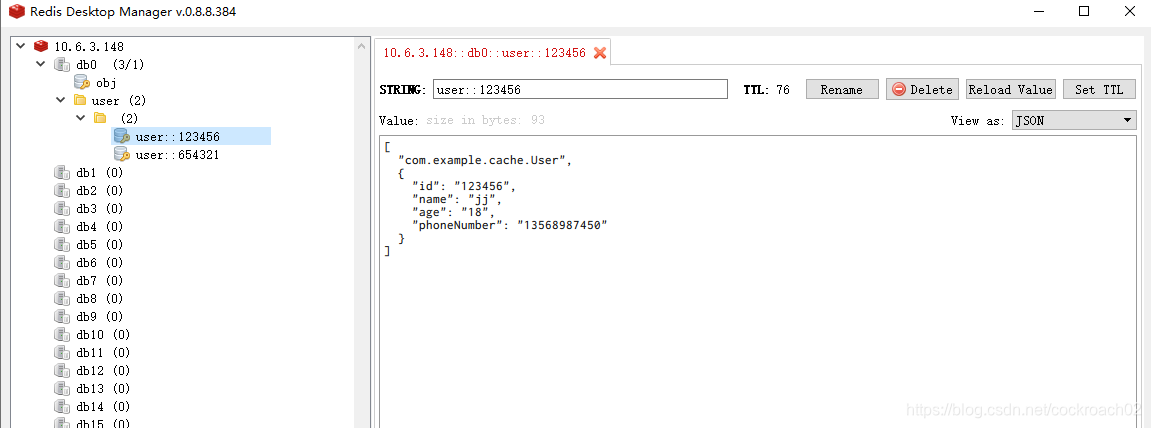
五、参考链接
- springboot使用cache缓存
- SpringBoot基础系列-SpringCache使用
- 缓存篇(三)- Spring Cache框架
- spring boot中的cache使用
- 【spring boot2】第10篇:spring boot 整合 cache
- Spring Cache介绍和使用
- 史上最全的Spring Boot Cache使用与整合
- spring-boot+redis实现缓存功能
- Spring Boot(八)集成Spring Cache 和 Redis
- Spring Cache扩展:注解失效时间+主动刷新缓存(二)
- 配置spring cache RedisCacheManager的序列化方法
- Spring Cache Redis 修改序列化方式