A. Calculus
Chipmunk is a math genius, and he is extraordinarily good at calculus.
Now Chipmunk gives you a formula and asks you to calculate it in one minute, or you should treat him to dinner. The formula looks like this:
∫2π0dx(1+sin2x)2−sin2x−−−−−−−−√∫02�d�(1+sin2�)2−sin2�
Obviously it is impossible to get the accurate answer, so Chipmunk only requires you to tell him the integer part of it.
Input
It can be anything, and has nothing to do with the answer.
Output
Print an integer n (1≤n≤5)� (1≤�≤5), representing the answer to the problem above.
Example
input
Copy
The probability that Awson is god
output
Copy
1
Note
Of course Chipmunk will not tell you the correct answer in the example above!
/*#include<iostream>
#include<iomanip>
#include<algorithm>
#include<cstdlib>
#include<math.h>
#include<cstring>
#include<string>
#include<map>
#include<set>
#include<vector>
#include<queue>
#include<stack>
#include<unordered_map>
#include<unordered_set>*/
#include <bits/stdc++.h>
using namespace std;
#define endl '\n'
#define ll long long
#define int long long
#define double long double
const int N = 1e6 + 5, inf = INT_MAX, mod = 998244353;
const double eps = 1e-8, pi = acos(-1);
int n;
string s;
void solve()
{
ios::sync_with_stdio(0), cin.tie(0), cout.tie(0);
getline(cin, s);
cout << 3;
}
signed main()
{
int _ = 1;
// cin >> _;
while (_--)
{
solve();
}
return 0;
}
B. Rule 110
The Rule 110 cellular automaton (often called simply Rule 110) is an elementary cellular automaton with interesting behavior on the boundary between stability and chaos. In this respect, it is similar to Conway's Game of Life. Like Life, Rule 110 is known to be Turing complete. This implies that, in principle, any calculation or computer program can be simulated using this automaton.
The Rule 110 is famous for its interesting properties. Among the 88 possible unique elementary cellular automata, Rule 110 is the only one for which Turing completeness has been proven, although proofs for several similar rules should follow as simple corollaries (e.g. Rule 124, which is the horizontal reflection of Rule 110). Rule 110 is arguably the simplest known Turing complete system.
This paragraph will introduce how Rule 110 works. When applying Rule 110 to a pattern, whether each point in the pattern will be 0 or 1 in the new generation depends on its current value, and on the values of its two neighbors. If a point is at the left/right border, then the left/right neighbor of it will be considered as 0. The rules are as follows:
Current pattern | 000 | 001 | 010 | 011 | 100 | 101 | 110 | 111 |
New state for center cell | 0 | 1 | 1 | 1 | 0 | 1 | 1 | 0 |
Chipmunk is interested in the automaton but he is not clever enough to solve it quickly. Now Chipmunk gives you the initial pattern, and you need to apply Rule 110 to the pattern once and output the result.
Input
The first line contains an integer n (1≤n≤105)� (1≤�≤105), the length of the pattern.
The next line is a string of length n� consisting of only 0s and 1s, representing the initial pattern.
Output
Output a string of length n�, the pattern after applying Rule 110.
Scoring
The problem contains several subtasks. You can get the corresponding score for each passed test case.
Subtask 1 (4040 points): n≤1000�≤1000.
Subtask 2 (6060 points): no additional constraints.
Examples
input
Copy
1
1
output
Copy
1
input
Copy
8
00111010
output
Copy
01101110
Note
In the first example, there is an only 1 in the initial pattern. The two neighbors of it are both 0, which satisfies pattern 010. According to the table above, the value of the point will stay at 1.
/*#include<iostream>
#include<iomanip>
#include<algorithm>
#include<cstdlib>
#include<math.h>
#include<cstring>
#include<string>
#include<map>
#include<set>
#include<vector>
#include<queue>
#include<stack>
#include<unordered_map>
#include<unordered_set>*/
#include <bits/stdc++.h>
using namespace std;
#define endl '\n'
#define ll long long
#define int long long
#define double long double
const int N = 1e6 + 5, inf = INT_MAX, mod = 998244353;
const double eps = 1e-8, pi = acos(-1);
int n;
string s;
map<string, char> m;
void solve()
{
m["000"] = '0';
m["001"] = '1';
m["010"] = '1';
m["011"] = '1';
m["100"] = '0';
m["101"] = '1';
m["110"] = '1';
m["111"] = '0';
cin >> n >> s;
s.insert(s.begin(), '0');
s += '0';
string ans = "";
for (int i = 1; i < s.size() - 1; ++i)
{
string t = s.substr(i - 1, 3);
ans += m[t];
}
cout << ans;
}
signed main()
{
// ios::sync_with_stdio(0), cin.tie(0), cout.tie(0);
int _ = 1;
// cin >> _;
while (_--)
{
solve();
}
return 0;
}
C. Make it in a Line
The game Tic-Tac-toe is played by two people taking turns on a 3×33×3 board, with the person winning the game when his first getting a whole horizontal or vertical or diagonal line filled with his tokens. It's easy to find that there are only 88 ways of putting tokens to win the game (only considering the tokens in the key line).
All 88 winning ways are shown in the picture below.
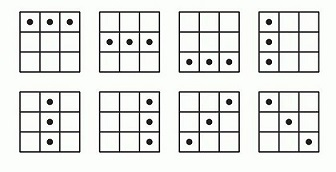
Today, Walk_alone is bored with playing the 2D Tic-Tac-Toe with Salix Leaf! He thinks the board is too small and the winning ways are too monotonous. So they decide to make a larger chess board and add a brand new dimension to the game! Now this game seems more interesting:
In a 3D n×n×n�×�×� cube, two people put their tokens by turn, and the first one whose tokens fill one horizontal or vertical or diagonal line of the cube wins the game. For instance, some winning ways in a 3D 4×4×44×4×4 cube are shown in the picture below.
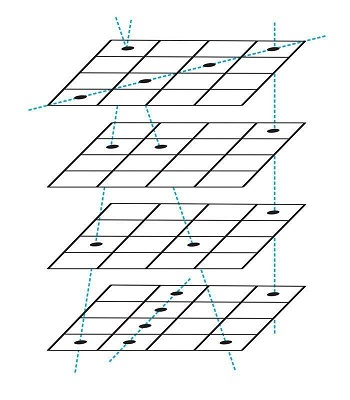
He is too lazy to do any calculation, so he wants you to tell him the number of ways to put the tokens in a line.
Input
The first and the only line of the input contains an integer n (2≤n≤1000)� (2≤�≤1000).
Output
Print only one number representing the number of ways to put the tokens in a line.
Scoring
The problem contains 2020 test cases. You can get 55 points for each passed test case.
Examples
input
Copy
2
output
Copy
28
input
Copy
3
output
Copy
49
input
Copy
4
output
Copy
76
/*#include<iostream>
#include<iomanip>
#include<algorithm>
#include<cstdlib>
#include<math.h>
#include<cstring>
#include<string>
#include<map>
#include<set>
#include<vector>
#include<queue>
#include<stack>
#include<unordered_map>
#include<unordered_set>*/
#include <bits/stdc++.h>
using namespace std;
#define endl '\n'
#define ll long long
#define int long long
#define double long double
const int N = 1e6 + 5, inf = INT_MAX, mod = 998244353;
const double eps = 1e-8, pi = acos(-1);
int n;
void solve()
{
cin >> n;
int a = 3ll * pow(n, 2ll);
int b = 6ll * n;
int c = 4ll;
cout << a + b + c;
}
signed main()
{
// ios::sync_with_stdio(0), cin.tie(0), cout.tie(0);
int _ = 1;
// cin >> _;
while (_--)
{
solve();
}
return 0;
}
D. Hard Tasks
After math class, Teacher Awson gave Sinoey n� tasks as his homework, the i�-th of which is to calculate the sum of 33 consecutive integers starting from i−1�−1, i.e. (i−1)+i+(i+1)(�−1)+�+(�+1). Note that he does not need to calculate i−1�−1 or i+1�+1.
It is a big problem for Sinoey because he does not want to carry even once! For two numbers represented as xn…x2x1¯¯¯¯¯¯¯¯¯¯¯¯¯¯¯¯¯¯¯¯¯¯¯��…�2�1¯ and yn…y2y1¯¯¯¯¯¯¯¯¯¯¯¯¯¯¯¯¯¯¯¯¯¯��…�2�1¯, carrying means there exists an integer i (1≤i≤n)� (1≤�≤�) such that xi+yi≥10��+��≥10. Take the 44th task, 3+4+53+4+5 as an example. Since 3+4+5>103+4+5>10, Sinoey will have to minus the first digit by 1010 and add 11 to the next digit in order to get the correct answer.
Carrying is awful, and Sinoey decides to do only the tasks that do not require carrying. Now you need to tell him the number of tasks he will do.
Input
The only line of the input contains an integer n (1≤n≤1018)� (1≤�≤1018), the total number of tasks.
Output
Print an integer representing the number of tasks Sinoey decides to do.
Scoring
The problem contains several subtasks. You can get the corresponding score for each passed test case.
Subtask 1 (3030 points): n≤106�≤106.
Subtask 2 (3030 points): n≤1010�≤1010.
Subtask 3 (4040 points): no additional constraints.
Examples
input
Copy
12
output
Copy
5
input
Copy
141214
output
Copy
1536
Note
In the first example, task 1,2,3,111,2,3,11 and 1212 are easy.
/*#include<iostream>
#include<iomanip>
#include<algorithm>
#include<cstdlib>
#include<math.h>
#include<cstring>
#include<string>
#include<map>
#include<set>
#include<vector>
#include<queue>
#include<stack>
#include<unordered_map>
#include<unordered_set>*/
#include <bits/stdc++.h>
using namespace std;
#define endl '\n'
#define ll long long
#define int long long
#define double long double
const int N = 1e6 + 5, inf = INT_MAX, mod = 998244353;
const double eps = 1e-8, pi = acos(-1);
int n;
void solve()
{
cin >> n;
vector<int> v;
while (n)
{
v.push_back(n % 10);
n /= 10;
}
int ans = 0;
for (int i = v.size() - 1; i >= 0; --i)
{
if (!i)
{
ans += min(v[i], 3ll);
}
if (i)
{
ans += min(v[i], 4ll) * 3 * pow(4ll, i - 1ll);
}
if (v[i] >= 4)
{
break;
}
}
cout << ans;
}
signed main()
{
// ios::sync_with_stdio(0), cin.tie(0), cout.tie(0);
int _ = 1;
// cin >> _;
while (_--)
{
solve();
}
return 0;
}
E. 2584
Getting bored with playing 2048, Awson came up with a new game called 2584, and he asks you to simulate the game for him.
There is a board with n×n�×� cells, some of which are occupied with Fibonacci numbers or obstacles. Recall that Fibonacci sequence F� is an infinite sequence of numbers in which each number (Fibonacci number) is the sum of the previous two, i.e.
Fn=⎧⎩⎨12Fn−1+Fn−2n=1n=2n≥3��={1�=12�=2��−1+��−2�≥3
You are given a sequence of Q� operations where each operation is one of "LRUD", indicating the directions: left, right, up and down, respectively. In each operation, you should
In the corresponding direction, arbitrarily take one of the foremost numbers which is not taken before. Let it be x�.
Move towards the number in that direction, until it reaches the border or a non-empty cell.
If the cell in front of x� is a number (denoted as y�) satisfying
y� is not changed in the current operation.
y� is adjacent to x� in Fibonacci sequence.
then add x� to y� (we call that y� is changed), and delete x� (x� will become an empty cell).
Repeat the instructions above until all numbers have been taken.
You need to show Awson the board after executing all operations. Note that no new numbers will be generated in this game.
Input
The first line contains two integers n (1≤n≤100)� (1≤�≤100) and Q (1≤Q≤100)� (1≤�≤100), the size of the board and the number of operations.
Each of the next n� lines contains n� integers, where the j�-th number of the i�-th line is ai,j (−1≤ai,j≤2584)��,� (−1≤��,�≤2584). The cell on line i� column j� is empty if ai,j=0��,�=0, an obstacle if ai,j=−1��,�=−1, or a Fibonacci number otherwise. It is guaranteed that ai,j��,� is either 00, −1−1 or a Fibonacci number.
The last line is a string of length Q� consisting of "LRUD", representing the direction of operations.
Output
Output n� lines, each line containing n� integers separated by spaces, representing the board after all operations. The output format should be the same with input.
Scoring
The problem contains several subtasks. You can get the corresponding score for each passed test case.
Subtask 1 (4040 points): for all i� and j�, ai,j≥0��,�≥0.
Subtask 2 (6060 points): no additional constraints.
Examples
input
Copy
4 1
2 3 2 3
3 -1 0 2
1 0 0 1
3 2 3 5
R
output
Copy
0 0 5 5
3 -1 0 2
0 0 1 1
0 0 5 8
input
Copy
2 6
2584 1597
1597 987
LLDRLD
output
Copy
0 0
6765 0
input
Copy
5 2
2 3 5 3 8
3 5 -1 -1 2
1 0 0 2 3
-1 -1 0 2 3
1 1 1 1 3
LU
output
Copy
13 8 8 0 5
3 3 -1 -1 0
0 0 5 1 0
-1 -1 1 0 0
1 1 0 0 0
Note
Take operation R and sequence [2,3,2,3][2,3,2,3] as an example (the first line in example 1). The operation contains the following 44 steps.
Step 11. The rightmost number 33 is taken. Since 33 is already at the border, the sequence will remain the same.
Step 22. Now the rightmost number is 22, because 33 is already taken. The number in front of 22 is 33. Merge the two numbers and it becomes [2,3,0,5][2,3,0,5].
Step 33. Move 33 to the right and it becomes [2,0,3,5][2,0,3,5]. Though 33 and 55 are adjacent in Fibonacci sequence, 55 is merged in step 22, so 33 cannot merge with 55.
Step 44. Move 22 to the right and merge with 33. Finally, the sequence becomes [0,0,5,5][0,0,5,5].
/*#include<iostream>
#include<iomanip>
#include<algorithm>
#include<cstdlib>
#include<math.h>
#include<cstring>
#include<string>
#include<map>
#include<set>
#include<vector>
#include<queue>
#include<stack>
#include<unordered_map>
#include<unordered_set>*/
#include <bits/stdc++.h>
using namespace std;
#define endl '\n'
#define ll long long
#define int long long
#define double long double
const int N = 1e2 + 5, inf = INT_MAX, mod = 998244353;
const double eps = 1e-8, pi = acos(-1);
int n, m, a[N][N], f[N];
bool vis[N][N];
map<int, int> mp;
bool work(int x1, int y1, int x2, int y2)
{
if (x1 < 1 || y1 < 1 || x2 < 1 || y2 < 1 || x1 > n || y1 > n || x2 > n || y2 > n || !a[x1][y1] || a[x1][y1] == -1)
{
return 0;
}
if (!a[x2][y2])
{
a[x2][y2] = a[x1][y1];
a[x1][y1] = 0;
return 1;
}
if (a[x2][y2] == -1 || vis[x2][y2])
{
return 0;
}
if (mp[a[x2][y2]] == mp[a[x1][y1]] - 1 || mp[a[x2][y2]] == mp[a[x1][y1]] + 1)
{
a[x2][y2] += a[x1][y1];
a[x1][y1] = 0;
vis[x2][y2] = 1;
return 0;
}
return 0;
}
void solve()
{
f[1] = 1, f[2] = 1;
mp[f[2]] = 2;
for (int i = 3; i <= 32; ++i)
{
f[i] = f[i - 1] + f[i - 2];
mp[f[i]] = i;
}
cin >> n >> m;
for (int i = 1; i <= n; ++i)
{
for (int j = 1; j <= n; ++j)
{
cin >> a[i][j];
}
}
for (int i = 1; i <= m; ++i)
{
memset(vis, 0, sizeof vis);
char c;
cin >> c;
if (c == 'L')
{
for (int j = 1; j <= n; ++j)
{
for (int k = 2; k <= n; ++k)
{
int x = j, y = k;
while (work(x, y, x, y - 1))
{
--y;
}
}
}
}
if (c == 'R')
{
for (int j = 1; j <= n; ++j)
{
for (int k = n - 1; k >= 1; --k)
{
int x = j, y = k;
while (work(x, y, x, y + 1))
{
++y;
}
}
}
}
if (c == 'U')
{
for (int j = 1; j <= n; ++j)
{
for (int k = 2; k <= n; ++k)
{
int x = k, y = j;
while (work(x, y, x - 1, y))
{
--x;
}
}
}
}
if (c == 'D')
{
for (int j = 1; j <= n; ++j)
{
for (int k = n - 1; k >= 1; --k)
{
int x = k, y = j;
while (work(x, y, x + 1, y))
{
++x;
}
}
}
}
}
for (int i = 1; i <= n; ++i)
{
for (int j = 1; j <= n; ++j)
{
cout << a[i][j] << " ";
}
cout << endl;
}
}
signed main()
{
ios::sync_with_stdio(0), cin.tie(0), cout.tie(0);
int _ = 1;
// cin >> _;
while (_--)
{
solve();
}
return 0;
}
F. Do Not Play Nim
Yjmeimei likes game theory, but she is not smart enough to solve any related problem.
Now Zygege gives her a new problem.
There are n� piles of stones. Alice and Bob take turns removing them. On Alice's turn, she can remove an arbitrary number of stones (at least one) from a single pile. On Bob's turn, he can remove some stones from a single pile, and the number of stones he chooses should not be less than the number of stones Alice has removed in total. Alice plays first, and the player who cannot make a move loses.
Now Yjmeimei needs to figure out who will win the game if both Alice and Bob play the game optimally. Of course it is still too hard for her, so she asks you for help.
Since the problem is a bit like Nim, Yjmeimei names it "Do Not Play Nim".
Input
The input contains several test cases.
The first line of the input contains an integer T (1≤T≤10)� (1≤�≤10), the number of test cases.
For each test case, the first line contains an integer n (1≤n≤2⋅105)� (1≤�≤2⋅105) indicating the number of piles of stones. The second line of contains n� integers. The i�-th integer ai (1≤ai≤109)�� (1≤��≤109) represents the number of stones in the i�-th pile.
It is guaranteed that the sum of n� in all test cases does not exceed 2⋅1052⋅105.
Output
For each test case, print "Alice" or "Bob" (without quotes) in one line indicating the winner. If the result is not deterministic, print "Awson is God!".
Scoring
The problem contains several subtasks. You can get the corresponding score for each passed test case.
Subtask 1 (2020 points): n≤2�≤2.
Subtask 2 (8080 points): no additional constraints.
Example
input
Copy
311021 161 1 4 5 1 4
output
Copy
Alice
Bob
Alice
Note
In the first case, Alice can take 66 stones, and it will be impossible for Bob to take no less than 66 stones, so Alice wins.
In the second case, Alice will remove the first pile, and Bob will remove the second pile. Alice cannot move after that for all stones are removed, so Bob wins.
The third case is too smelly for Yjmeimei to elaborate on!
/*#include<iostream>
#include<iomanip>
#include<algorithm>
#include<cstdlib>
#include<math.h>
#include<cstring>
#include<string>
#include<map>
#include<set>
#include<vector>
#include<queue>
#include<stack>
#include<unordered_map>
#include<unordered_set>*/
#include <bits/stdc++.h>
using namespace std;
#define endl '\n'
#define ll long long
#define int long long
#define double long double
const int N = 1e6 + 5, inf = INT_MAX, mod = 998244353;
const double eps = 1e-8, pi = acos(-1);
int n;
void solve()
{
cin >> n;
vector<int> v(n), vt(2, 1);
for (auto &x : v)
{
cin >> x;
}
if (v == vt)
{
cout << "Bob" << endl;
}
else
{
cout << "Alice" << endl;
}
}
signed main()
{
ios::sync_with_stdio(0), cin.tie(0), cout.tie(0);
int _ = 1;
cin >> _;
while (_--)
{
solve();
}
return 0;
}
G. Awson Loves Chipmunks
Awson loves chipmunks, especially the k�-th one among all n� chipmunks! He wants to keep a consecutive interval of chipmunks in his home, and the interval should include the k�-th chipmunk.
However, chipmunks eat a lot and it is a heavy burden for Awson to buy food for all chipmunks. Specifically, the i�-th chipmunk has a hunger value ai��. If Awson decides to raise the chipmunks from index l� to r (1≤l≤k≤r≤n)� (1≤�≤�≤�≤�), then the burden brought to Awson will be f(l,r)=∑l≤i≤rai�(�,�)=∑�≤�≤���.
Awson does not want to worry too much about chipmunks' food, so he will choose the interval with the m�-th smallest burden. Now you are required to tell Awson the burden he will carry.
Input
The first line contains three integers n (1≤n≤106)� (1≤�≤106), k (1≤k≤n)� (1≤�≤�) and m (1≤m≤k(n−k+1))� (1≤�≤�(�−�+1)) as described above.
The second line contains n� integers. The i�-th integer ai (|ai|≤109)�� (|��|≤109) represents the hunger value of the i�-th chipmunk.
Output
Print an integer representing the m�-th smallest burden.
Scoring
The problem contains several subtasks. You can get the corresponding score for each passed test case.
Subtask 1 (3030 points): n≤103�≤103.
Subtask 2 (7070 points): no additional constraints.
Examples
input
Copy
4 2 3
1 2 4 8
output
Copy
6
input
Copy
4 2 2
-1 2 -4 8
output
Copy
-2
Note
In the first example, the second chipmunk must be chosen, and there are 66 ways to choose the interval in total. They are f(2,2)=2,�(2,2)=2, f(1,2)=3,�(1,2)=3, f(2,3)=6,�(2,3)=6, f(1,3)=7,�(1,3)=7, f(2,4)=14,�(2,4)=14, and f(1,4)=15�(1,4)=15. Among them, f(2,3)=6�(2,3)=6 is the third smallest, so the answer is 66.
/*#include<iostream>
#include<iomanip>
#include<algorithm>
#include<cstdlib>
#include<math.h>
#include<cstring>
#include<string>
#include<map>
#include<set>
#include<vector>
#include<queue>
#include<stack>
#include<unordered_map>
#include<unordered_set>*/
#include <bits/stdc++.h>
using namespace std;
#define endl '\n'
#define ll long long
#define int long long
#define double long double
const int N = 1e6 + 5, inf = INT_MAX, mod = 998244353;
const double eps = 1e-8, pi = acos(-1);
int n, k, m, a[N];
vector<int> L, R;
bool check(int mid)
{
int res = 0;
for (int i = 0, j = L.size() - 1; i < R.size(); ++i)
{
if (R[i] <= mid)
{
++res;
}
while (j >= 0 && L[j] + R[i] > mid)
{
--j;
}
if (j >= 0 && L[j] + R[i] <= mid)
{
res += j + 1;
}
}
return res < m;
}
void solve()
{
cin >> n >> k >> m;
for (int i = 1; i <= n; ++i)
{
cin >> a[i];
}
int sum = 0;
for (int i = k - 1; i >= 1; --i)
{
sum += a[i];
L.push_back(sum);
}
sum = 0;
for (int i = k; i <= n; ++i)
{
sum += a[i];
R.push_back(sum);
}
sort(L.begin(), L.end());
sort(R.begin(), R.end());
int l = -1e18, r = 1e18;
while (l < r)
{
int mid = (l + r + 1) >> 1;
if (check(mid))
{
l = mid;
}
else
{
r = mid - 1;
}
}
cout << l + 1;
}
signed main()
{
ios::sync_with_stdio(0), cin.tie(0), cout.tie(0);
int _ = 1;
// cin >> _;
while (_--)
{
solve();
}
return 0;
}