1、平移动画(translate)
方法1:在XML代码中设置
1)在路径 res/anim/创建xml文件:translate_animation.xml
2)代码如下:
translate xmlns:android="http://schemas.android.com/apk/res/android"
android:duration="3000"
android:fillAfter="false"
android:fillBefore="true"
android:fillEnabled="true"
android:fromXDelta="0"
android:fromYDelta="0"
android:repeatCount="1"
android:repeatMode="restart"
android:startOffset="1000"
android:toXDelta="500"
android:toYDelta="500"
/>
<!--
duration:动画的持续时
startOffset:动画的延迟时间
fillBefore:动画播放完毕以后,视图是否停留在动画开始的状态,默认为true
fillAfter:动画播放完毕以后,视图是否停留在动画结束的状态。优先于fillBefore,默认为false
fillEnabled:是否应用fillBefore值,对fillAfter值无影响,默认为true
repeatMode:重复播放的模式。"restart":代表正序播放。"reverse":代表倒叙播放
repeatCount:重发次数
interpolator:插值器
-->
3)代码中引用动画:
// 步骤1:创建 需要设置动画的 视图View
Button mButton = (Button) findViewById(R.id.Button);
// 步骤2:创建 动画对象 并传入设置的动画效果xml文件
Animation translateAnimation = AnimationUtils.loadAnimation(this, R.anim.translate_animation);
// 步骤3:播放动画
mButton.startAnimation(translateAnimation);
方法2:直接代码设置
Animation translateAnimation = new TranslateAnimation(0, 500, 0, 500);
translateAnimation.setDuration(3000);
translateAnimation.setRepeatCount(100);
mButton.startAnimation(translateAnimation);
2、缩放动画(scale)
方式1:xmlyinyo
<scale xmlns:android="http://schemas.android.com/apk/res/android"
android:duration="3000"
android:startOffset="1000"
android:fillBefore="true"
android:fillAfter="false"
android:fillEnabled="true"
android:repeatMode="restart"
android:repeatCount="100"
android:fromXScale="1.0"
android:toXScale="3.0"
android:fromYScale="1.0"
android:toYScale="3.0"
android:pivotX="0%"
android:pivotY="0%"
>
<!--fromXScale:动画水平方向X的起始缩放倍数,0.0表示搜索到没有,1.0表示正常无伸缩。值大于1.0表示放大,小于1.0表示收缩。
pivoX:缩放轴点的x坐标
pivoY:缩放轴点的y坐标
//上面的两个值都是50%表示动画从自身中间开始。-->
</scale>
代码中引用xml动画:
Animation scaleAnimation= AnimationUtils.loadAnimation(this,R.anim.scale_animation);
mTv1.startAnimation(scaleAnimation);
方式2:直接代码设置:
//参数说明:
//参数1.fromX:水平方向X的缩放倍数
//参数2.toX
//参数3.fromY:竖直方向Y的缩放倍数
//参数4.toY
//参数5.pivoXType:缩放点x坐标模式。(即缩放的中心点在哪里)
//pivoXType:Animation.ABSOLUTE:缩放点X的坐标 = View左上角的原点 + pivoXVale数值的值
//pivoXType:Animation.RELATIVE_TO_SELF:缩放轴点的x的坐标 = View左上角的原点 + 自身宽度乘以pivoXType数值的值
//pivoXType:Animation_RELATIVE_TO_PARENT:缩放轴点 =View左上角的原点 + 父控件宽度乘以pivoXType数值的值
Animation scaleAnimation=new ScaleAnimation(0,3,0,3,Animation.RELATIVE_TO_SELF,0.5f,Animation.RELATIVE_TO_SELF,0.5f);
scaleAnimation.setDuration(300);
scaleAnimation.setRepeatCount(100);
mTv1.startAnimation(scaleAnimation);
3、旋转动画(rotate)
方式1:在xml中设置
<rotate xmlns:android="http://schemas.android.com/apk/res/android"
android:duration="3000"
android:repeatCount="100"
android:fromDegrees="0"
android:toDegrees="720"
android:pivotX="50%"
android:pivotY="50%"/>
代码中引用:
Animation rorateAnimation= AnimationUtils.loadAnimation(this,R.anim.ratate_animation);
mTv1.startAnimation(rorateAnimation);
方式2:直接代码设置
Animation rotateAnimation=new RotateAnimation(0,360,Animation.RELATIVE_TO_SELF,0.5f,Animation.RELATIVE_TO_SELF,0.5f);
rotateAnimation.setDuration(3000);
rotateAnimation.setRepeatCount(100);
mTv1.startAnimation(rotateAnimation);
4、透明动画(Alpha)
方式1:xml中设置
<alpha xmlns:android="http://schemas.android.com/apk/res/android"
android:duration="3000"
android:repeatCount="100"
android:fromAlpha="0.0"
android:toAlpha="1.0">
</alpha>
代码中引用动画:
Animation alphaAnimation= AnimationUtils.loadAnimation(this,R.anim.alpha_animation);
mTv1.startAnimation(alphaAnimation);
方式2:代码中设置动画
Animation alphaAnimation=new AlphaAnimation(1.0f,0.0f);
alphaAnimation.setDuration(3000);
alphaAnimation.setRepeatCount(100);
mTv1.startAnimation(alphaAnimation);
5、动画集
方式1:xml中设置
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:duration="3000"
android:repeatCount="100"
android:shareInterpolator="true">
<!--
写在 set标签里面的,表示动画具备的公共属性
shareInterpolator:表示组合动画中的动画是否和集合共享同一个插值器,如果集合不指定插值器,那么子动画需要单独设置
-->
<alpha android:fromAlpha="1.0"
android:toAlpha="0.5"/>
<translate android:fromXDelta="0"
android:toXDelta="100"
android:fromYDelta="0"
android:toYDelta="100"/>
<scale android:fromXScale="1.0"
android:toXScale="3.0"
android:fromYScale="1.0"
android:toYScale="3.0"
android:pivotX="50%"
android:pivotY="50%"/>
<rotate android:fromDegrees="0"
android:toDegrees="360"
android:pivotX="50%"
android:pivotY="50%"/>
</set>
代码中引用xml动画:
Animation setAnimation= AnimationUtils.loadAnimation(this,R.anim.set_animation);
mTv1.startAnimation(setAnimation);
方式2:代码直接设置
//组合动画
AnimationSet setAnimation=new AnimationSet(true);
setAnimation.setDuration(3000);
setAnimation.setRepeatCount(100);
setAnimation.setRepeatMode(Animation.RESTART);
//创建子动画(位移动画,每次位移父控件的宽度*0.5的宽度)
Animation translteAnimation=new TranslateAnimation(TranslateAnimation.RELATIVE_TO_PARENT,0.0f,
TranslateAnimation.RELATIVE_TO_PARENT,0.5f,
TranslateAnimation.RELATIVE_TO_PARENT,0.0f,
TranslateAnimation.RELATIVE_TO_PARENT,0.0f);
//缩放动画(xy分别放大3倍,放大的中心点为控件自身的中心点)
Animation scaleAnimation=new ScaleAnimation(1.0f,3.0f,1.0f,3.0f,Animation.RELATIVE_TO_SELF,0.5f,Animation.RELATIVE_TO_SELF,0.5f);
//透明动画(从不透明 到 半透明)
Animation alphaAnimation=new AlphaAnimation(1.0f,0.5f);
//旋转动画(从0 旋转到 360,旋转的点为控件的中心点)
Animation rotateAnimation=new RotateAnimation(0,360,Animation.RELATIVE_TO_SELF,0.5f,Animation.RELATIVE_TO_SELF,0.5f);
setAnimation.addAnimation(alphaAnimation);
setAnimation.addAnimation(scaleAnimation);
setAnimation.addAnimation(rotateAnimation);
setAnimation.addAnimation(translteAnimation);
mTv1.startAnimation(setAnimation);
6、插值器
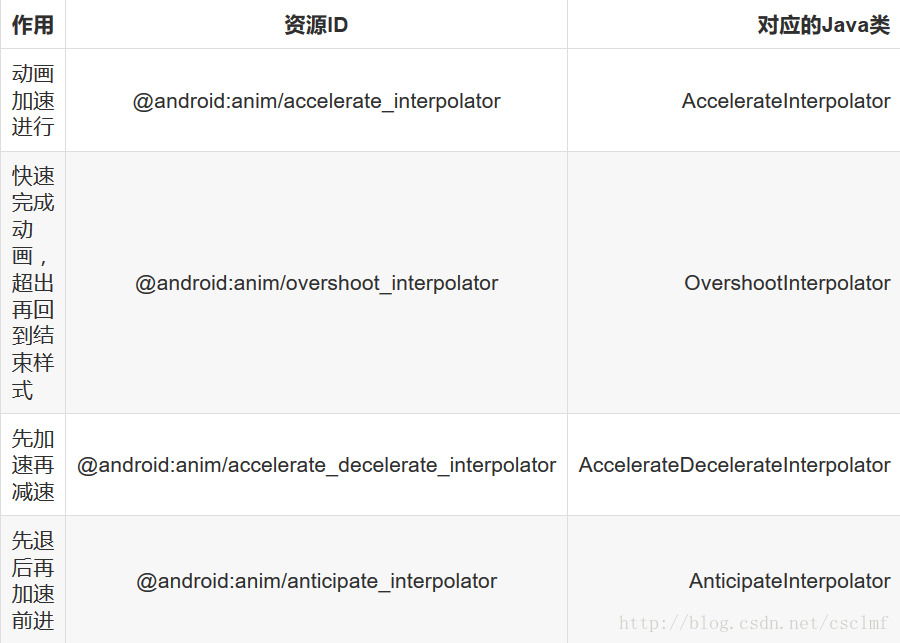
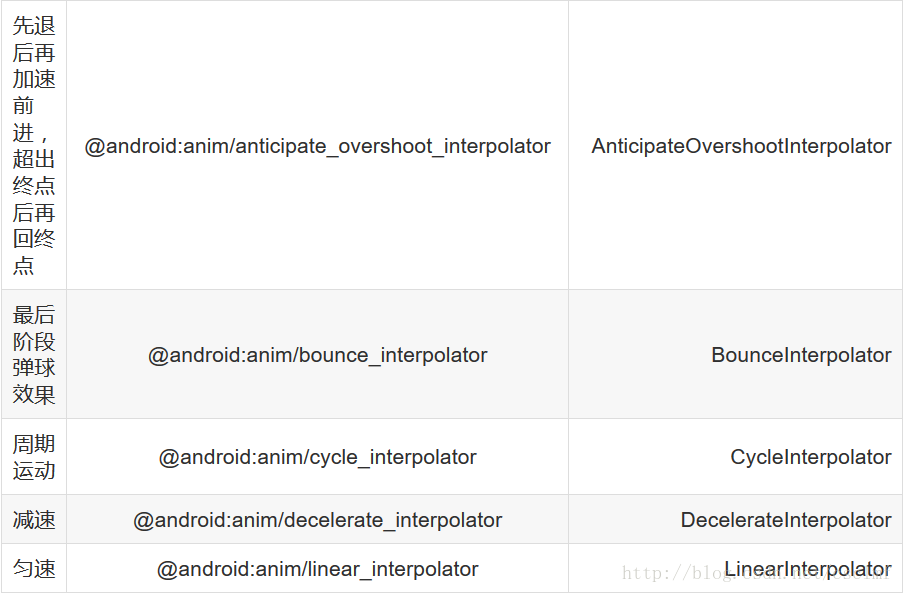
使用方式1:在xml中设置
方式2:直接代码设置
7、动画监听
setAnimation.setAnimationListener(new Animation.AnimationListener() {
@Override
public void onAnimationStart(Animation animation) {
//动画开始执行
System.out.println("MainActivity.onAnimationStart");
}
@Override
public void onAnimationEnd(Animation animation) {
//动画结束时执行
System.out.println("MainActivity.onAnimationEnd");
}
@Override
public void onAnimationRepeat(Animation animation) {
//动画重复的时候执行
System.out.println("MainActivity.onAnimationRepeat");
}
});
Button mButton = (Button) findViewById(R.id.Button);
// 步骤1:创建 需要设置动画的 视图View Animation translateAnimation = AnimationUtils.loadAnimation(
this, R.anim.view_animation);
// 步骤2:创建 动画对象 并传入设置的动画效果xml文件 mButton.startAnimation(translateAnimation);
// 步骤3:播放动画
作者:Carson_Ho
链接:https://www.jianshu.com/p/733532041f46
來源:简书
著作权归作者所有。商业转载请联系作者获得授权,非商业转载请注明出处。
Button mButton = (Button) findViewById(R.id.Button);
// 步骤1:创建 需要设置动画的 视图View Animation translateAnimation = AnimationUtils.loadAnimation(
this, R.anim.view_animation);
// 步骤2:创建 动画对象 并传入设置的动画效果xml文件 mButton.startAnimation(translateAnimation);
// 步骤3:播放动画
作者:Carson_Ho
链接:https://www.jianshu.com/p/733532041f46
來源:简书
著作权归作者所有。商业转载请联系作者获得授权,非商业转载请注明出处。