If you’ve never created a Vue.js application, I am going to guide you through the task of creating one, and understanding how it works.
如果您从未创建过Vue.js应用程序,那么我将指导您完成创建一个应用程序并了解其工作方式的任务。
第一个例子 (First example)
First I’ll use the most basic example of using Vue.
首先,我将使用使用Vue的最基本示例。
You create an HTML file which contains
您创建一个HTML文件,其中包含
<html>
<body>
<div id="example">
<p>{{ hello }}</p>
</div>
<script src="https://unpkg.com/vue"></script>
<script>
new Vue({
el: '#example',
data: { hello: 'Hello World!' }
})
</script>
</body>
</html>
and you open it in the browser. That’s your first Vue app! The page should show a “Hello World!” message.
然后在浏览器中打开它。 这是您的第一个Vue应用! 该页面应显示“ Hello World!” 信息。
I put the script tags at the end of the body so that they are executed in order after the DOM is loaded.
我将脚本标签放在正文的末尾,以便在加载DOM之后按顺序执行它们。
What this code does is, we instantiate a new Vue app, linked to the #example
element as its template (it’s defined using a CSS selector usually, but you can also pass in an HTMLElement).
该代码的作用是,我们实例化一个新的Vue应用程序,该应用程序链接到#example
元素作为其模板(通常使用CSS选择器定义,但您也可以传入HTMLElement)。
Then, it associates that template to the data
object. That is a special object that hosts the data we want Vue to render.
然后,它将该模板与data
对象相关联。 这是一个特殊的对象,用于承载我们要Vue渲染的数据。
In the template, the special {{ }}
tag indicates that’s some part of the template that’s dynamic, and its content should be looked up in the Vue app data.
在模板中,特殊的{{ }}
标签表示这是动态模板的一部分,应在Vue应用程序数据中查找其内容。
在Codepen上查看 (See on Codepen)
You can see this example on Codepen: https://codepen.io/flaviocopes/pen/YLoLOp
您可以在Codepen上看到以下示例: https ://codepen.io/flaviocopes/pen/YLoLOp
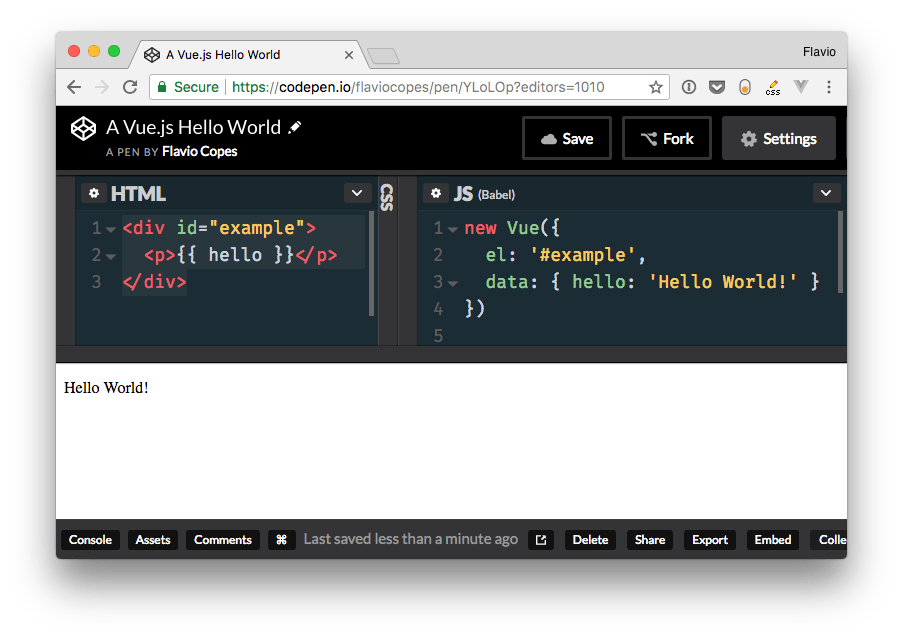
Codepen is a little different from using a plain HTML file, and you need to configure it to point to the Vue library location in the Pen settings:
Codepen与使用纯HTML文件有点不同,您需要对其进行配置以指向Pen设置中的Vue库位置:

第二个示例:Vue CLI默认应用 (Second example: the Vue CLI default app)
Let’s level up the game a little bit. The next app we’re going to build is already done, and it’s the Vue CLI default application.
让我们稍微升级一下游戏。 我们将要构建的下一个应用程序已经完成,它是Vue CLI默认应用程序 。
What is the Vue CLI? It’s a command line utility that helps to speed up development by scaffolding an application skeleton for you, with a sample app in place.
什么是Vue CLI? 它是一个命令行实用程序,可通过在适当位置安装示例应用程序来为您架设一个应用程序框架来帮助加快开发速度。
There are two ways you can get this application.
您可以通过两种方式获得此应用程序。
在本地使用Vue CLI (Use the Vue CLI locally)
The first is to install the Vue CLI on your computer, and run the command
首先是在计算机上安装Vue CLI ,然后运行命令
vue create <enter the app name>
使用CodeSandbox (Use CodeSandbox)
A simpler way, without having to install anything, is to go to https://codesandbox.io/s/vue.
一种无需安装任何内容的简单方法是转到https://codesandbox.io/s/vue 。
CodeSandbox is a cool code editor that allows you build apps in the cloud, which allows you to use any npm package and also easily integrate with Zeit Now for an easy deployment and GitHub to manage versioning.
CodeSandbox是一个很酷的代码编辑器,它使您可以在云中构建应用程序,从而可以使用任何npm软件包,还可以轻松地与Zeit Now集成以实现轻松部署,并通过GitHub管理版本控制。
That link I put above opens the Vue CLI default application.
我上面放置的链接打开了Vue CLI默认应用程序。
Whether you chose to use the Vue CLI locally, or through CodeSandbox, let’s inspect that Vue app in detail.
无论您是选择本地使用Vue CLI还是通过CodeSandbox使用,我们都将详细检查该Vue应用程序。
文件结构 (The files structure)
Beside package.json
, which contains the configuration, these are the files contained in the initial project structure:
在package.json
(其中包含配置)旁边,这些是初始项目结构中包含的文件:
index.html
index.html
src/App.vue
src/App.vue
src/main.js
src/main.js
src/assets/logo.png
src/assets/logo.png
src/components/HelloWorld.vue
src/components/HelloWorld.vue
index.html
(index.html
)
The index.html
file is the main app file.
index.html
文件是主应用程序文件。
In the body it includes just one simple element: <div id="app"></div>
. This is the element the Vue application will use to attach to the DOM.
在主体中,它仅包含一个简单元素: <div id="app"></div>
。 这是Vue应用程序将用于附加到DOM的元素。
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0">
<title>CodeSandbox Vue</title>
</head>
<body>
<div id="app"></div>
<!-- built files will be auto injected -->
</body>
</html>
src/main.js
(src/main.js
)
This is the main JavaScript files that drive our app.
这是驱动我们应用程序的主要JavaScript文件。
We first import the Vue library and the App component from App.vue
.
我们首先从App.vue
导入Vue库和App组件。
We set productionTip to false, just to avoid Vue to output a “you’re in development mode” tip in the console.
我们将productionTip设置为false,只是为了避免Vue在控制台中输出“ 您处于开发模式 ”提示。
Next, we create the Vue instance, by assigning it to the DOM element identified by #app
, which we defined in index.html
, and we tell it to use the App component.
接下来,我们通过将Vue实例分配给我们在index.html
定义的#app
标识的DOM元素来创建Vue实例,并告诉它使用App组件。
// The Vue build version to load with the `import` command
// (runtime-only or standalone) has been set in webpack.base.conf with an alias.
import Vue from 'vue'
import App from './App'
Vue.config.productionTip = false
/* eslint-disable no-new */
new Vue({
el: '#app',
components: { App },
template: '<App/>'
})
src/App.vue
(src/App.vue
)
App.vue
is a Single File Component. It contains 3 chunks of code: HTML, CSS and JavaScript.
App.vue
是单个文件组件。 它包含3个代码块:HTML,CSS和JavaScript。
This might seem weird at first, but Single File Components are a great way to create self-contained components that have all they need in a single file.
乍一看似乎很奇怪,但是“单个文件组件”是一种创建独立组件的好方法,这些组件可以在单个文件中包含所有必需的组件。
We have the markup, the JavaScript that is going to interact with it, and style that’s applied to it, which can be scoped, or not. In this case, it’s not scoped, and it’s just outputting that CSS which is applied like regular CSS to the page.
我们有标记,将要与之交互JavaScript以及应用于它的样式,无论该样式是否可以作用域。 在这种情况下,它没有作用域,只是输出了像常规CSS一样应用到页面CSS。
The interesting part lies in the script
tag.
有趣的部分在于script
标签。
We import a component from the components/HelloWorld.vue
file, which we’ll describe later.
我们从components/HelloWorld.vue
文件导入一个组件 ,稍后将对其进行描述。
This component is going to be referenced in our component. It’s a dependency. We are going to output this code:
该组件将在我们的组件中引用。 这是一个依赖关系。 我们将输出以下代码:
<div id="app">
<img width="25%" src="./assets/logo.png">
<HelloWorld/>
</div>
from this component, which you see references the HelloWorld component. Vue will automatically insert that component inside this placeholder.
在该组件中,您会看到它引用了HelloWorld组件。 Vue会自动将该组件插入此占位符。
<template>
<div id="app">
<img width="25%" src="./assets/logo.png">
<HelloWorld/>
</div>
</template>
<script>
import HelloWorld from './components/HelloWorld'
export default {
name: 'App',
components: {
HelloWorld
}
}
</script>
<style>
#app {
font-family: 'Avenir', Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
src/components/HelloWorld.vue
(src/components/HelloWorld.vue
)
Here’s the HelloWorld component, which is included by the App component.
这是HelloWorld组件,它包含在App组件中。
This component outputs a set of links, along with a message.
该组件输出一组链接以及一条消息。
Remember above we talked about CSS in App.vue, which was not scoped? The HelloWorld component has scoped CSS.
还记得上面我们讨论过App.vue中CSS,但没有限定范围吗? HelloWorld组件的作用域为CSS。
You can easily determine it by looking at the style
tag. If it has the scoped
attribute, then it’s scoped: <style scoped>
您可以通过查看style
标签轻松确定它。 如果它具有scoped
属性,则它的作用域为: <style scoped>
This means that the generated CSS will be targeting the component uniquely, via a class that’s applied by Vue transparently. You don’t need to worry about this, and you know the CSS won’t leak to other parts of the page.
这意味着生成CSS将通过Vue透明应用的类来唯一地定位组件。 您无需为此担心,并且知道CSS不会泄漏到页面的其他部分。
The message the component outputs is stored in the data
property of the Vue instance, and outputted in the template as {{ msg }}
.
组件输出的消息存储在Vue实例的data
属性中,并在模板中以{{ msg }}
。
Anything that’s stored in data
is reachable directly in the template via its own name. We didn’t need to say data.msg
, just msg
.
data
存储的任何内容都可以通过自己的名称直接在模板中访问。 我们不需要说data.msg
,只需要说msg
。
<template>
<div class="hello">
<h1>{{ msg }}</h1>
<h2>Essential Links</h2>
<ul>
<li>
<a
href="https://vuejs.org"
target="_blank"
>
Core Docs
</a>
</li>
<li>
<a
href="https://forum.vuejs.org"
target="_blank"
>
Forum
</a>
</li>
<li>
<a
href="https://chat.vuejs.org"
target="_blank"
>
Community Chat
</a>
</li>
<li>
<a
href="https://twitter.com/vuejs"
target="_blank"
>
Twitter
</a>
</li>
<br>
<li>
<a
href="http://vuejs-templates.github.io/webpack/"
target="_blank"
>
Docs for This Template
</a>
</li>
</ul>
<h2>Ecosystem</h2>
<ul>
<li>
<a
href="http://router.vuejs.org/"
target="_blank"
>
vue-router
</a>
</li>
<li>
<a
href="http://vuex.vuejs.org/"
target="_blank"
>
vuex
</a>
</li>
<li>
<a
href="http://vue-loader.vuejs.org/"
target="_blank"
>
vue-loader
</a>
</li>
<li>
<a
href="https://github.com/vuejs/awesome-vue"
target="_blank"
>
awesome-vue
</a>
</li>
</ul>
</div>
</template>
<script>
export default {
name: 'HelloWorld',
data() {
return {
msg: 'Welcome to Your Vue.js App'
}
}
}
</script>
<!-- Add "scoped" attribute to limit CSS to this component only -->
<style scoped>
h1,
h2 {
font-weight: normal;
}
ul {
list-style-type: none;
padding: 0;
}
li {
display: inline-block;
margin: 0 10px;
}
a {
color: #42b983;
}
</style>
运行应用 (Run the app)
CodeSandbox has a cool preview functionality. You can run the app and edit anything in the source to have it immediately reflected in the preview.
CodeSandbox具有很酷的预览功能。 您可以运行该应用程序并编辑源代码中的任何内容,以使其立即反映在预览中。
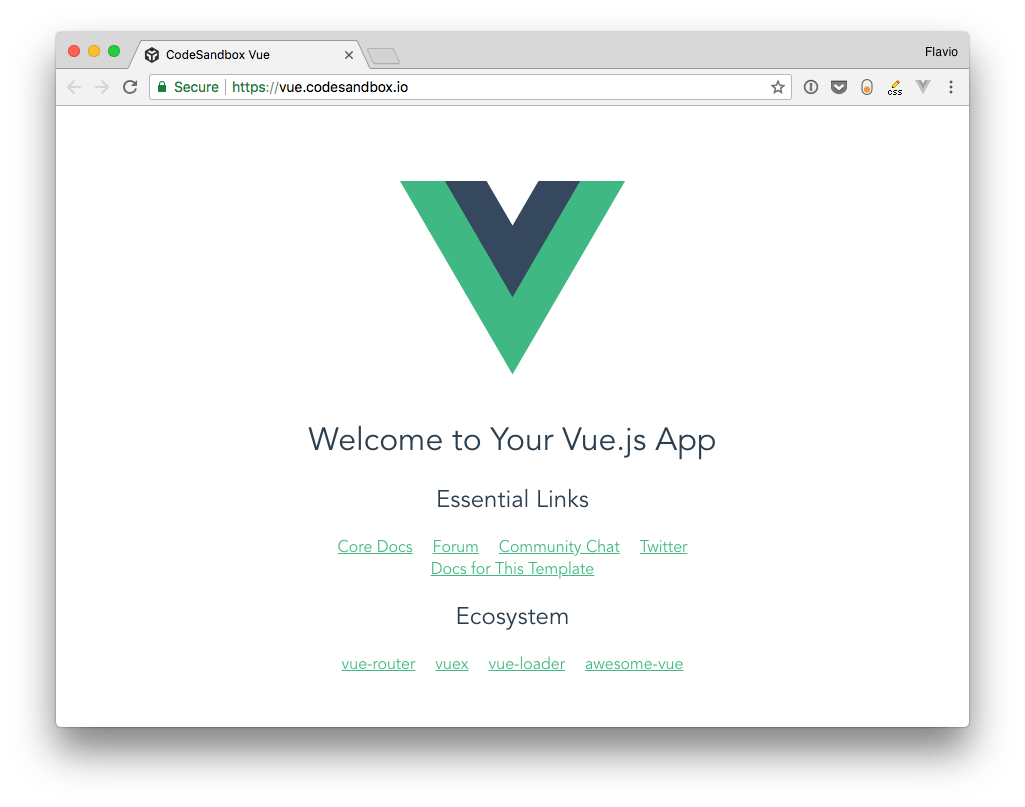