JavaScript Objects have properties, which are composed by a label associated with a value.
JavaScript对象具有属性,这些属性由与值关联的标签组成。
The object literal syntax we saw:
我们看到的对象文字语法:
const car = {
}
lets us define properties like this:
让我们定义如下属性:
const car = {
color: 'blue'
}
here we have a car
object with a property named color
, with value blue
.
在这里,我们有一个car
对象,其属性名为color
,值为blue
。
Labels can be any string. Notice that I didn’t use quotes around color
, but if I wanted to include a character not valid as a variable name in the property name, I would have had to:
标签可以是任何字符串。 请注意,我没有在color
周围使用引号,但是如果我想在属性名称中包括一个无效的字符作为变量名,则必须:
const car = {
color: 'blue',
'the color': 'blue'
}
This means spaces, hyphens, and more special characters.
这意味着空格,连字符和更多特殊字符。
As you see, we separate each property with a comma.
如您所见,我们用逗号分隔每个属性。
检索财产的价值 (Retrieving the value of a property)
We can retrieve the value of a property using 2 different syntaxes.
我们可以使用2种不同的语法来检索属性的值。
The first is dot notation:
第一个是点符号 :
car.color //'blue'
The second, which is mandatory for properties with invalid names, is to use square brackets:
第二种是使用无效名称的属性所必需的,第二种是使用方括号:
car['the color'] //'blue'
If you access an unexisting property, you get undefined
:
如果访问不存在的属性,则会得到undefined
:
car.brand //undefined
A nice way to check for a property value but default to a predefined value is to use the ||
operator:
检查属性值但默认为预定义值的一种好方法是使用||
操作员:
const brand = car.brand || 'ford'
As said, objects can have nested objects as properties:
如前所述,对象可以将嵌套对象作为属性:
const car = {
brand: {
name: 'Ford'
},
color: 'blue'
}
You can access the brand name using
您可以使用以下方式访问品牌名称
car.brand.name
or
要么
car['brand']['name']
or even mixing:
甚至混合:
car.brand['name']
car['brand'].name
设置属性的值 (Setting the value of a property)
As you saw above you can set the value of a property when you define the object.
如上所见,定义对象时可以设置属性的值。
But you can always update it later on:
但您随时可以在以后进行更新:
const car = {
color: 'blue'
}
car.color = 'yellow'
car['color'] = 'red'
And you can also add new properties to an object:
您还可以向对象添加新属性:
car.model = 'Fiesta'
car.model //'Fiesta'
如何删除财产 (How to remove a property)
Given the object
给定对象
const car = {
color: 'blue',
brand: 'Ford'
}
you can delete a property from this object using
您可以使用以下命令从此对象中删除属性
delete car.brand
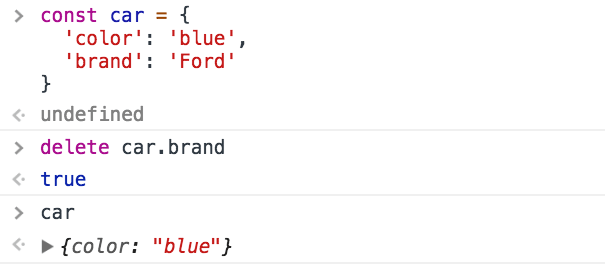
It works also expressed as:
它的工作方式还表示为:
delete car['brand']
delete car.brand
delete newCar['brand']
将属性设置为undefined (Setting a property to undefined)
If you need to perform this operation in a very optimized way, for example, when you’re operating on a large number of objects in loops, another option is to set the property to undefined
.
如果您需要以非常优化的方式执行此操作,例如,当您在循环中对大量对象进行操作时,另一种选择是将属性设置为undefined
。
Due to its nature, the performance of delete
is a lot slower than a simple reassignment to undefined
, more than 50x times slower.
由于其性质, delete
的性能要比简单地重新分配给undefined
慢很多 ,比慢得多50倍。
However, keep in mind that the property is not deleted from the object. Its value is wiped, but it’s still there if you iterate the object:
但是,请记住,该属性不会从对象中删除。 它的值被擦除了,但是如果您迭代该对象,它仍然存在:
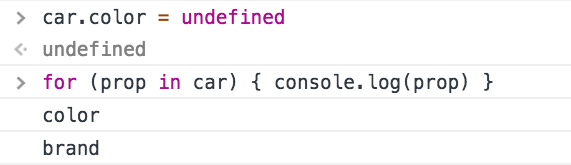
Using delete
is still very fast, you should only look into this kind of performance issues if you have a very good reason to do so, otherwise it’s always preferred to have a more clear semantic and functionality.
使用delete
仍然非常快,只有在您有充分理由这样做时,才应研究此类性能问题,否则始终首选具有更清晰的语义和功能。
删除属性而不更改对象 (Remove a property without mutating the object)
If mutability is a concern, you can create a completely new object by copying all the properties from the old, except the one you want to remove:
如果需要考虑可变性,则可以通过复制旧属性中的所有属性来创建一个全新的对象,但要删除的属性除外:
const car = {
color: 'blue',
brand: 'Ford'
}
const prop = 'color'
const newCar = Object.keys(car).reduce((object, key) => {
if (key !== prop) {
object[key] = car[key]
}
return object
}, {})
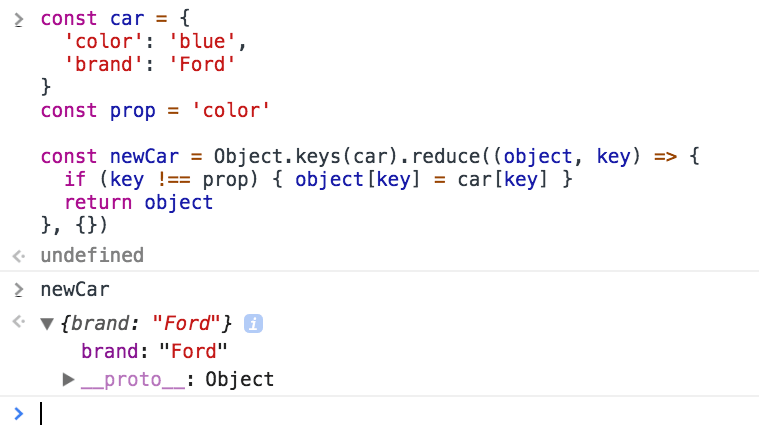
如何计算JavaScript对象中的属性数量 (How to count the number of properties in a JavaScript object)
Use the Object.keys()
method, passing the object you want to inspect, to get an array of all the (own) enumerable properties of the object.
使用Object.keys()
方法传递要检查的对象,以获得该对象的所有(自有)可枚举属性的数组。
Then calculate the length of that array by checking the length
property:
然后通过检查length
属性来计算该数组的length
:
const car = {
color: 'Blue',
brand: 'Ford',
model: 'Fiesta'
}
Object.keys(car).length
I said enumerable properties. This means their internal enumerable flag is set to true, which is the default. Check MDN for more info on this subject.
我说了无数的属性。 这意味着它们的内部可枚举标志设置为true,这是默认设置。 检查MDN以获取有关此主题的更多信息。
如何检查JavaScript对象属性是否未定义 (How to check if a JavaScript object property is undefined)
In a JavaScript program, the correct way to check if an object property is undefined is to use the typeof
operator.
在JavaScript程序中,检查对象属性是否未定义的正确方法是使用typeof
运算符。
typeof
returns a string that tells the type of the operand. It is used without parentheses, passing it any value you want to check:
typeof
返回一个字符串,该字符串告诉操作数的类型。 它不带括号使用,将要检查的任何值传递给它:
const list = []
const count = 2
typeof list //"object"
typeof count //"number"
typeof "test" //"string"
typeof color //"undefined"
If the value is not defined, typeof
returns the ‘undefined’ string.
如果未定义值,则typeof
返回'undefined' 字符串 。
Now suppose you have a car
object, with just one property:
现在假设您有一个只有一个属性的car
对象:
const car = {
model: 'Fiesta'
}
This is how you check if the color
property is defined on this object:
这是检查color
属性是否在此对象上定义的方法:
if (typeof car.color === 'undefined') {
// color is undefined
}
动态特性 (Dynamic properties)
When defining a property, its label can be an expression if wrapped in square brackets:
定义属性时,如果用方括号括起来,则其标签可以是表达式:
const car = {
['c' + 'o' + 'lor']: 'blue'
}
car.color //'blue'
语法更简单,将变量包含为对象属性 (Simpler syntax to include variables as object properties)
Instead of doing
而不是做
const something = 'y'
const x = {
something: something
}
you can do this simplified way:
您可以使用以下简化方式:
const something = 'y'
const x = {
something
}