MongoDB is a NoSQL database. Under the NoSQL umbrella we put all those databases that do not use the SQL language for querying the data.
MongoDB是NoSQL数据库。 在NoSQL的保护下,我们放置了所有不使用SQL语言查询数据的数据库。
MongoDB的主要特征 (Key characteristics of MongoDB)
MongoDB is a very JavaScript-friendly database. It exposes a JavaScript API we can use to create databases and collections of objects (called documents).
MongoDB是一个非常友好JavaScript数据库。 它公开了一个JavaScript API,可用于创建数据库和对象(称为文档 )的集合。
It’s schemaless, which means you don’t need to pre-define a structure for the data before storing it.
它是无模式的 ,这意味着您无需在存储数据之前预先定义数据的结构。
In MongoDB you can store any object without having to worry about the particular fields that compose this object and how to store them. You tell MongoDB to store that object.
在MongoDB中,您可以存储任何对象,而不必担心组成该对象的特定字段以及如何存储它们。 您告诉MongoDB存储该对象。
Data is stored in a format similar to JSON, but enhanced to allow storing more than just basic data types.
数据以类似于JSON的格式存储,但经过增强后可以存储的不仅仅是基本数据类型。
安装 (Installation)
Let’s go ahead and install MongoDB. You could use one of the many cloud providers that offer access to a MongoDB instance, but for the sake of learning, we’ll install it ourselves.
让我们继续安装MongoDB。 您可以使用提供访问MongoDB实例的众多云提供商之一,但是为了学习起见,我们将自行安装它。
I use a Mac, so the installation instructions in this tutorial refer to that operating system.
我使用的是Mac,因此本教程中的安装说明涉及该操作系统。
Open the terminal and run:
打开终端并运行:
brew tap mongodb/brew
brew install mongodb-community
That’s it.
而已。
The instructions were not too long or complicated, assuming you know how to use the terminal and how to install Homebrew.
如果您知道如何使用终端以及如何安装Homebrew ,那么说明就不会太长或太复杂。
The installation tells us this:
安装告诉我们:
To have launchd start mongodb now and restart at login:
brew services start mongodb-community
Or, if you don't want/need a background service you can just run:
mongod --config /usr/local/etc/mongod.conf
You can choose to either launch MongoDB once and have it running forever as a background service in your computer (the thing I prefer), or you can run it just when you need it, by running the latter command.
您可以选择启动一次MongoDB并使其永久作为计算机中的后台服务运行(我喜欢这种方式),也可以通过运行后者的命令仅在需要时运行它。
The default configuration for MongoDB is this:
MongoDB的默认配置是这样的:
systemLog:
destination: file
path: /usr/local/var/log/mongodb/mongo.log
logAppend: true
storage:
dbPath: /usr/local/var/mongodb
net:
bindIp: 127.0.0.1
Logs are stored in /usr/local/var/log/mongodb/mongo.log
and the database is stored in /usr/local/var/mongodb
.
日志存储在/usr/local/var/log/mongodb/mongo.log
,数据库存储在/usr/local/var/mongodb
。
By default there is no access control, anyone can read and write to the database.
默认情况下,没有访问控制,任何人都可以读写数据库。
蒙哥壳牌 (The Mongo Shell)
The best way to experiment with MongoDB and starting to interact with it is by running the mongo
program, which starts the MongoDB shell.
试用MongoDB并开始与其进行交互的最佳方法是运行mongo
程序,该程序将启动MongoDB Shell。
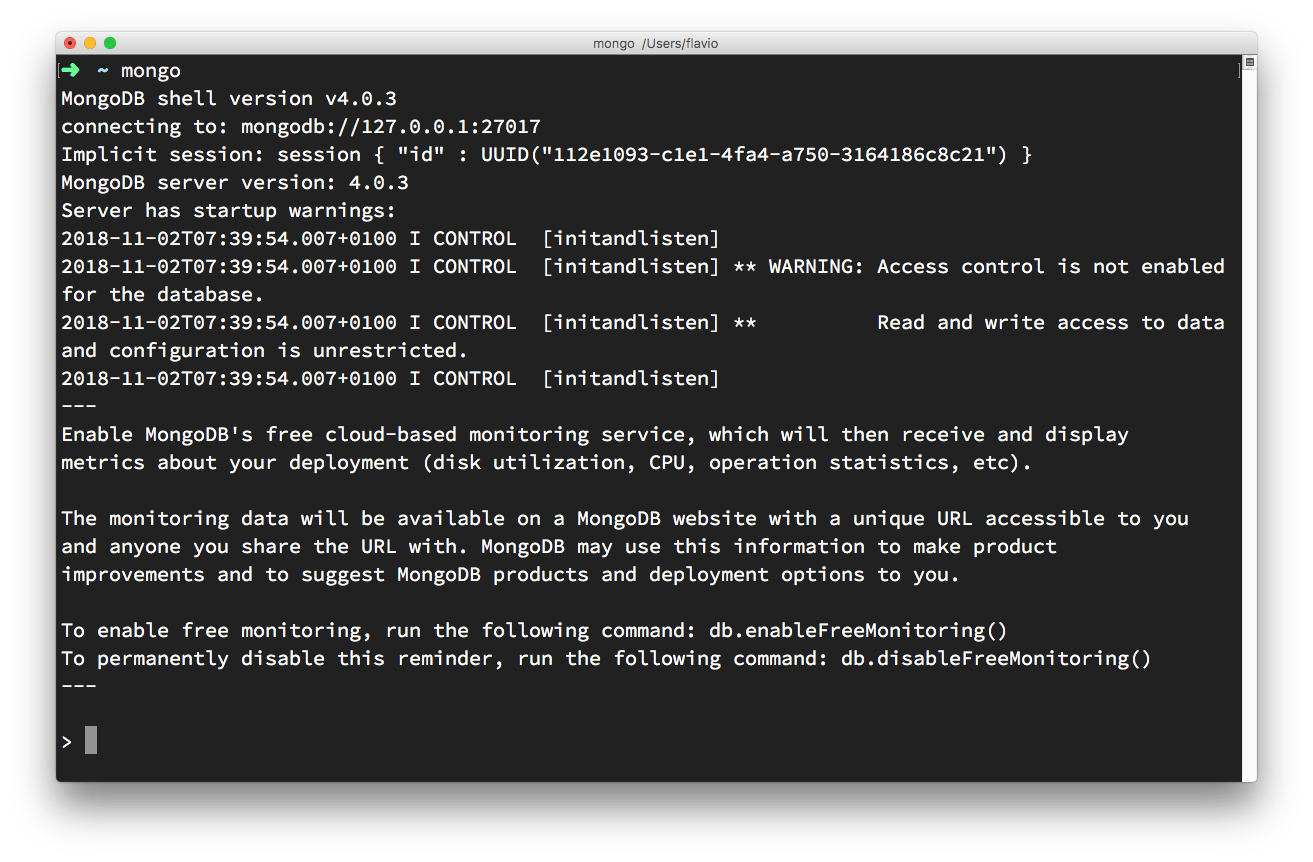
You can now enter any command that Mongo understands.
现在,您可以输入Mongo可以理解的任何命令。
建立资料库 (Create a database)
When you start, Mongo creates a database called test
. Run db
in the shell to tell you the name of the active database
开始时,Mongo将创建一个名为test
的数据库。 在外壳程序中运行db
以告诉您活动数据库的名称
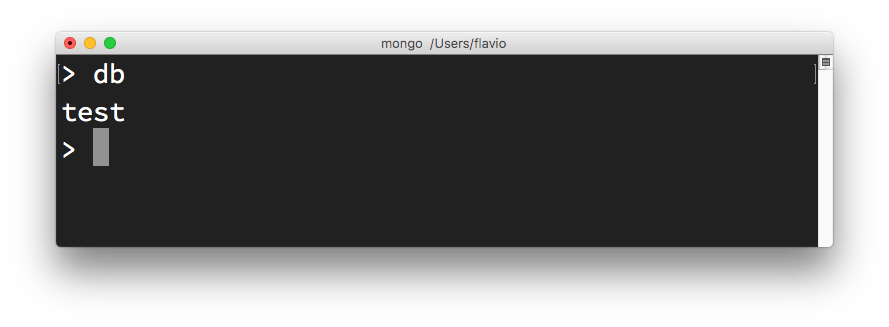
To change the database, just write use newname
and the newname
database will be instantly created and the shell switches to using that.
要更改数据库,只写use newname
和newname
数据库将立即创建和外壳切换到使用。
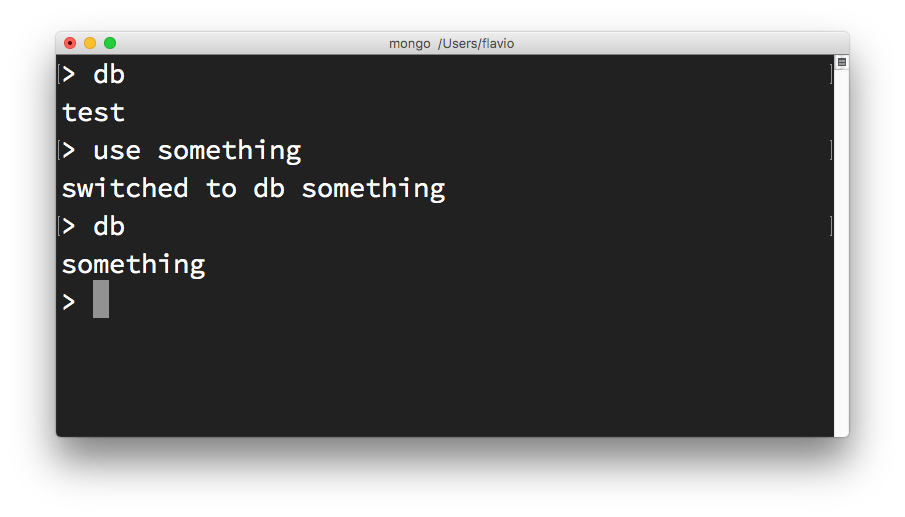
Use show databases
to list the available databases:
使用show databases
列出可用的数据库:
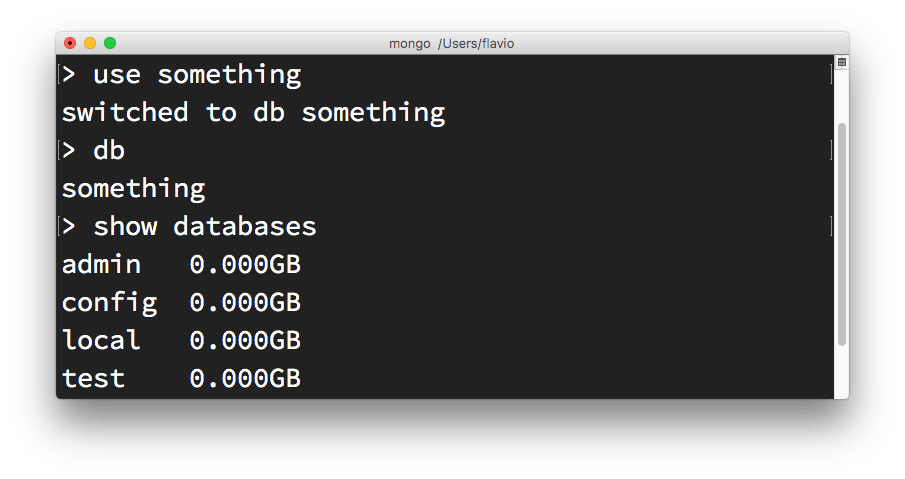
As you can see, the something
database is not listed, just because there is no collection yet in it. Let’s create one.
如您所见,未列出something
数据库,只是因为其中没有集合。 让我们创建一个。
馆藏 (Collections)
In MongoDB, a collection is the equivalent of a SQL database table.
在MongoDB中, 集合等效于SQL数据库表。
You create a collection on the current database by using the db.createCollection()
command. The first argument is the database name, and you can pass an options object as a second parameter.
您可以使用db.createCollection()
命令在当前数据库上创建一个集合。 第一个参数是数据库名称,您可以将options对象作为第二个参数传递。

Once you do so, show databases
will list the new database, and show collections
will list the collection.
一旦这样做, show databases
将列出新数据库,而show collections
将列出集合。
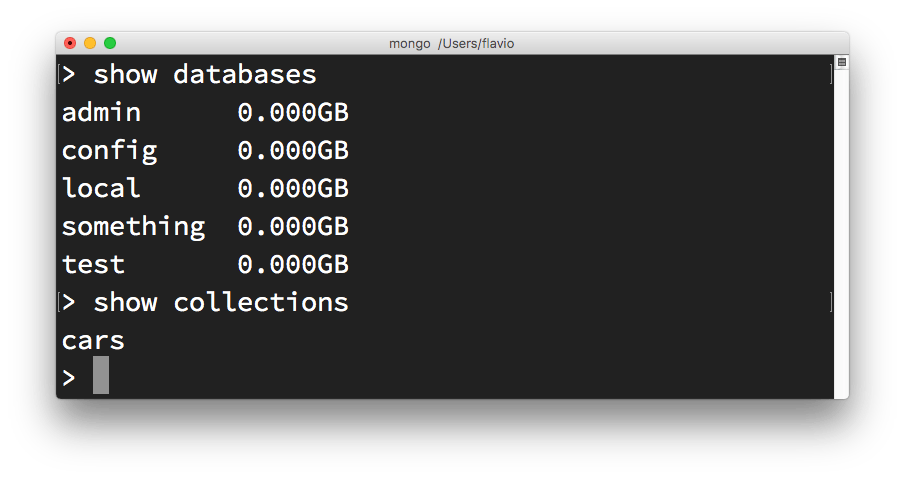
You can also create a new collection by using it as a property of the db
object, and calling insert()
to add an object to the collection:
您还可以通过将其用作db
对象的属性来创建新集合,并调用insert()
将对象添加到集合中:
db.dogs.insert({ name: 'Roger' })

列出集合中的对象 (Listing objects in a collection)
To show the objects added to a collection, use the find()
method:
要显示添加到集合中的对象,请使用find()
方法:
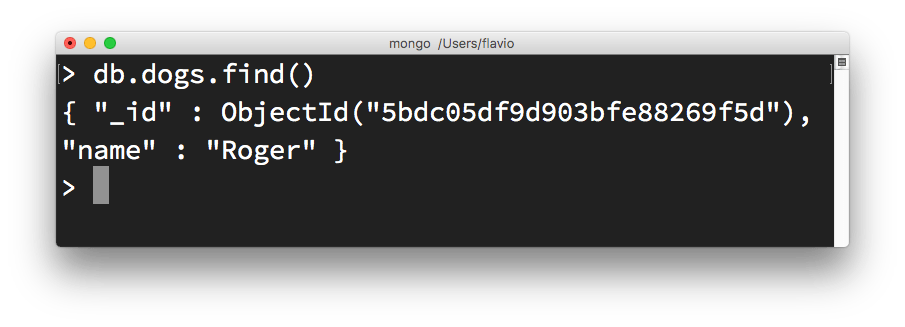
As you can see there is an additional _id
property for the record we added. That’s automatically generated for us by MongoDB.
如您所见,我们添加的记录还有一个附加的_id
属性。 这是MongoDB为我们自动生成的。
Now, add more dogs:
现在,添加更多的狗:
db.dogs.insert({ name: 'Buck' })
db.dogs.insert({ name: 'Togo' })
db.dogs.insert({ name: 'Balto' })
Calling db.dogs.find()
will give us all the entries, while we can pass a parameter to filter and retrieve a specific entry, for example with db.dogs.find({name: 'Roger'})
:
调用db.dogs.find()
将为我们提供所有条目,而我们可以传递参数来过滤和检索特定条目,例如使用db.dogs.find({name: 'Roger'})
:
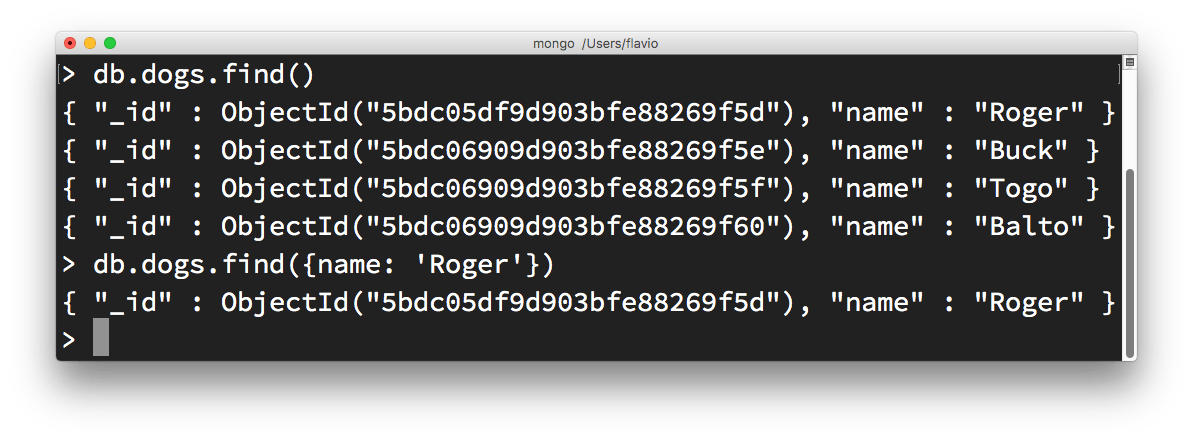
The find()
method returns a cursor you need to iterate on.
find()
方法返回需要迭代的游标。
There is another method which is handy when you know you’ll only get one record, which is findOne()
, and it’s used in the same way. If multiple records match a query, it will just return the first one.
当您知道只获得一条记录时,还有另一种方法很方便,它是findOne()
,并且以相同的方式使用。 如果多个记录与查询匹配,它将仅返回第一个。
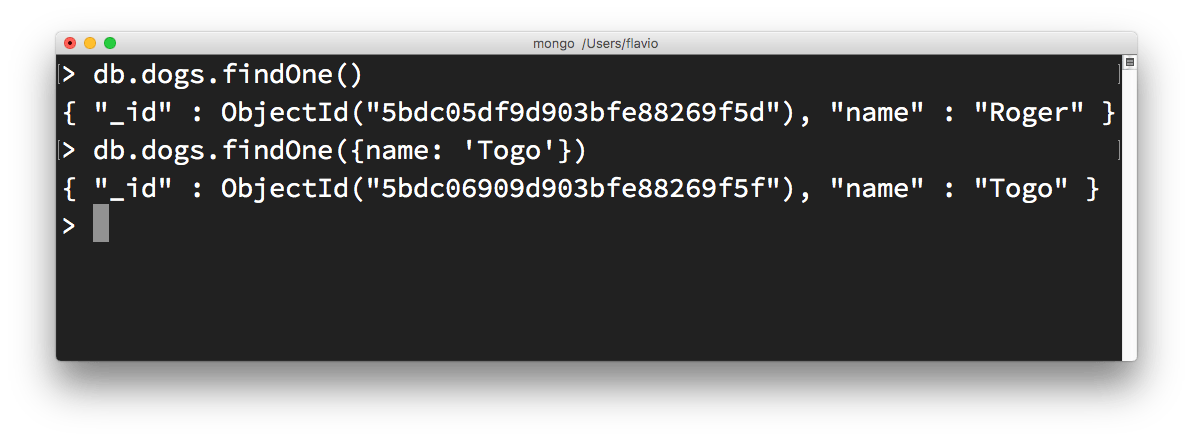
更新记录 (Updating records)
To update a record you can use the update()
method on a collection:
要更新记录,可以在集合上使用update()
方法:

删除记录 (Removing records)
You can remove a record calling the remove()
method on a collection, passing an object to help identify it:
您可以删除在集合上调用remove()
方法的记录,并传递一个对象来帮助识别它:

To remove all the entries from a collection, pass an empty object:
要从集合中删除所有条目,请传递一个空对象:
db.dogs.remove({})