react 简单还是vue
Vue动态表单 (Vue Dynamic Forms)
Easy way to dynamically create reactive forms in vue based on a varying business object model.
基于变化的业务对象模型在vue中动态创建响应式表单的简便方法。
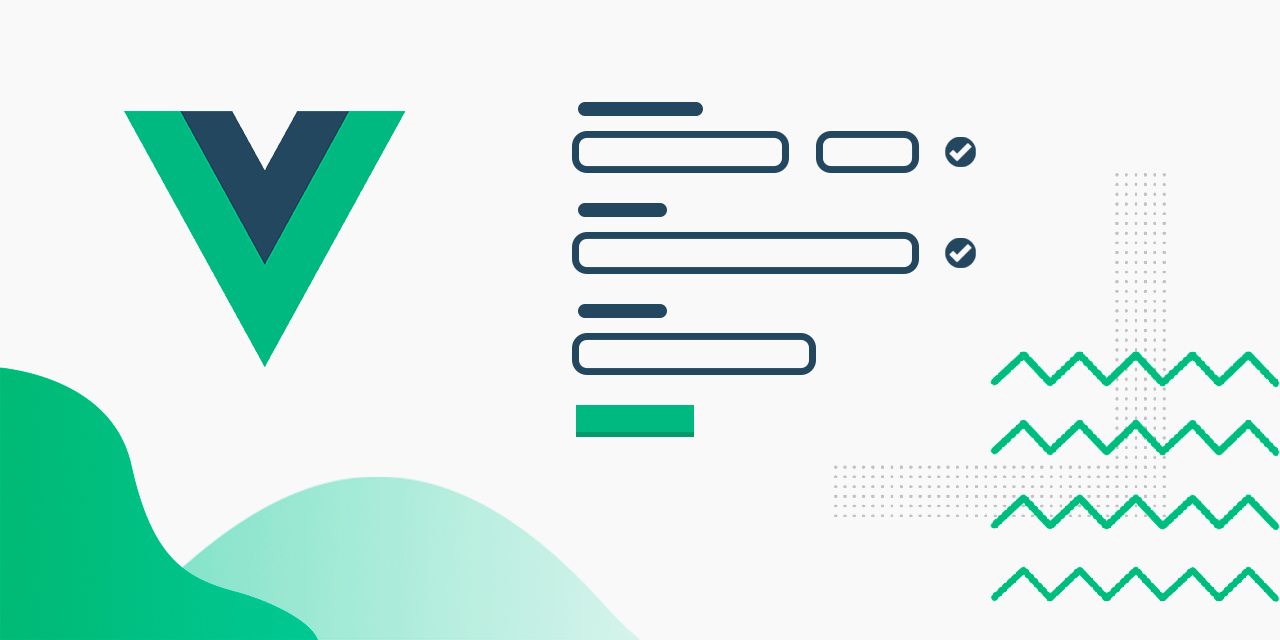
Implementing handcrafted forms can be:
实现手工制作的表单可以是:
Costly
昂贵
Time-consuming
耗时的
Specially if you need to create a very large form, in which the inputs are similar to each other, and they change frequently to meet rapidly changing business and regulatory requirements.
特别是如果您需要创建一个非常大的表单,其中输入彼此相似,并且它们会经常更改以满足快速变化的业务和法规要求。
So, will not be more economical to create the forms dynamically? Based on metadata that describes the business object model?
那么,动态创建表单会更经济吗? 基于描述业务对象模型的元数据?
That's Vue Dynamic Forms.
这就是Vue动态表单。
安装 (Installation)
$ npm install @asigloo/vue-dynamic-forms
or if you prefer yarn
或者如果您更喜欢纱线
$ yarn add @asigloo/vue-dynamic-forms
用法 (Usage)
全球 (Global)
Register the component globally in your main.js
:
在main.js
全局注册组件:
import Vue from 'vue';
import VueDynamicForms from '@asigloo/vue-dynamic-forms';
Vue.use(VueDynamicForms);
本地 (Local)
You can include the dynamic form directly to your component.
您可以将动态表单直接包含到组件中。
import Vue from 'vue';
import { DynamicForm } from '@asigloo/vue-dynamic-forms';
const components = { DynamicForm };
export {
...
components,
...
}
表格组成 (Form Composition)
The dynamic form component is really easy to use, you will only need to add it to your template like this:
动态表单组件非常易于使用,您只需要像这样将其添加到模板中:
<template>
<dynamic-form :id="testForm.id" :fields="testForm.fields" />
<button type="submit" :form="testForm.id">Submit</button>
</template>
And then create the fields on your component's data structure, to create the fields you can import the factory function FormField
from the library core:
然后在组件的数据结构上创建字段,要创建这些字段,可以从库核心导入工厂函数FormField
:
import { FormField } from '@asigloo/vue-dynamic-forms';
const yourAwesomeComponent = {
name: 'your-awesome',
data() {
return {
testForm: {
id: 'test-form',
fields: [
new FormField({
type: 'text',
label: 'Name',
name: 'name',
}),
new FormField({
type: 'email',
label: 'Email',
name: 'email',
}),
],
},
};
},
};
export default yourAwesomeComponent;
Each FormField
has a set of properties to customize your input field:
每个FormField
都有一组属性以自定义您的输入字段:
Property | Type | Default | Description |
---|---|---|---|
type | String | text | Define the nature of the field, can be text|email|url|password|number|radio|checkbox|textarea|select |
label | String | null | Defines the text in the input label |
placeholder | String | null | Defines short hint that describes the expected value of an input field |
name | String | null | Name property for the field inside of the form data |
value | any | null | Initial value of the input field |
disabled | Boolean | false | Whenever the input field is disabled or not |
customClass | String | null | Allows the user to set custom classes to the input for styling purpouses |
options | Array of Objects | [] | Options for input type select |
inline | Boolean | false | Specifies if options for radio|checkbox should be inline |
validations | Array of Objects | [] | Array of FormValidation objects, specify validations and messages for the input |
属性 | 类型 | 默认 | 描述 |
---|---|---|---|
类型 | 串 | 文本 | 定义字段的性质,可以是text|email|url|password|number|radio|checkbox|textarea|select |
标签 | 串 | 空值 | 定义输入标签中的文本 |
占位符 | 串 | 空值 | 定义简短提示,以描述输入字段的期望值 |
名称 | 串 | 空值 | 表单数据中字段的名称属性 |
值 | 任何 | 空值 | 输入字段的初始值 |
残障人士 | 布尔型 | 假 | 无论何时禁用输入字段 |
customClass | 串 | 空值 | 允许用户将自定义类设置为用于设计目的的输入 |
选项 | 对象数组 | [] | 输入类型select 选项 |
排队 | 布尔型 | 假 | 指定radio|checkbox 选项是否应该内联 |
验证 | 对象数组 | [] | FormValidation 对象的数组,为输入指定验证和消息 |
活动和提交 (Events and Submit)
Event | Type | Emitter | Description |
---|---|---|---|
submit | DynamicForm | Emits whenever the submit button has been clicked and the form is valid (no errors), returns all the form values in an Object | |
form-error | DynamicForm | Emits whenever the submit button has been clicked and the form is invalid (with errors), returns all errors | |
change | DynamicForm | Emits every time there is a value changed on the form, returns all the form values in an Object without the need ob submitting |
事件 | 类型 | 发射极 | 描述 |
---|---|---|---|
submit | DynamicForm | 每当单击“提交”按钮且表单有效(无错误)时,将退出,并返回对象中的所有表单值 | |
form-error | DynamicForm | 每当单击“提交”按钮并且表单无效(有错误)时,都会退出,并返回所有错误 | |
change | DynamicForm | 每次在表单上更改值时发出,在不需要提交的情况下返回对象中的所有表单值 |
A small example of a form without submit button
一个没有提交按钮的表单的小例子
<template>
<dynamic-form
:id="testForm.id"
:fields="testForm.fields"
@change="valuesChanged"
/>
</template>
...
const yourAwesomeComponent = {
name: 'your-awesome',
methods: {
valuesChanged(values) {
this.formData = {
...this.formData,
...values,
};
},
}
}
...
选择输入 (Select input)
...
new FormField({
type: 'select',
label: 'Category',
name: 'category',
options: [
{ value: null, text: 'Please select an option' },
{ value: 'arduino', text: 'Arduino' },
{ value: 'transistors', text: 'Transistors' },
],
}),
...
单选和复选框 (Radio and Checkboxes)
...
new FormField({
type: 'checkbox',
label: 'Read the conditions',
name: 'conditions',
inline: false,
}),
new FormField({
type: 'radio',
label: 'Prefered Animal',
name: 'animal',
inline: true,
options: [
{ text: 'Dogs', value: 'dogs' },
{ text: 'Cats', value: 'cats' },
{ text: 'Others', value: 'others' },
],
}),
...
验证方式 (Validation)
This library offers a simple validation system using the property validations
as an array of FormValidation
objects containing the validation function and the text in case of error.
该库提供了一个简单的验证系统,该系统使用属性validations
作为FormValidation
对象的数组, FormValidation
包含验证函数和文本(如果发生错误)。
To use it you need the following code:
要使用它,您需要以下代码:
import {
FormField,
FormValidation,
required,
email,
pattern,
} from '@asigloo/vue-dynamic-forms';
const yourAwesomeComponent = {
name: 'your-awesome',
data() {
return {
testForm: {
id: 'test-form',
fields: [
new FormField({
type: 'text',
label: 'Name',
name: 'name',
}),
new FormField({
type: 'email',
label: 'Email',
name: 'email',
validations: [
new FormValidation(required, 'This field is required'),
new FormValidation(email, 'Format of email is incorrect'),
],
}),
new FormField({
type: 'password',
label: 'Password',
name: 'password',
validations: [
new FormValidation(required, 'This field is required'),
new FormValidation(
pattern(
'^(?=.*[a-z])(?=.*[A-Z])(?=.*d)(?=.*[#$^+=!*()@%&]).{8,10}$',
),
'Password must contain at least 1 Uppercase, 1 Lowercase, 1 number, 1 special character and min 8 characters max 10',
),
],
}),
],
},
};
},
};
export default yourAwesomeComponent;
造型主题 (Styling themes)
The components are unstyled by default, so you can customize them with your own styles. If you want a more "ready to go" solution you can import on of the themes we have included in src/styles/themes/
组件默认情况下未设置样式,因此您可以使用自己的样式自定义它们。 如果您想要一个更“准备就绪”的解决方案,则可以导入src/styles/themes/
包含的src/styles/themes/
@import '[email protected]/vue-dynamic-forms/src/styles/themes/default.scss';
发展历程 (Development)
项目设置 (Project setup)
yarn install
编译和热重装 (Compiles and hot-reloads)
yarn run serve
编译并最小化生产 (Compiles and minifies for production)
yarn run build
运行测试 (Run your tests)
yarn run test
整理和修复文件 (Lints and fixes files)
yarn run lint
运行单元测试 (Run your unit tests)
yarn run test:unit
贡献 (Contributing)
If you find this library useful and you want to help improve it, maintain it or just want a new feature, feel free to contact me, or feel free to do a PR 😁.
如果您发现此库有用,并且想要帮助改进,维护它或只是想要一个新功能,请随时与我联系,或者随时进行PR😁。
待办事项清单 (Todolist)
This are the features I have planned for next versions of this library
这是我为该库的下一版本计划的功能
[ ] Material theme
[]素材主题
[ ] Form Mixins for fields manipulation (for example, change values of a field depending of other)
[] Form Mixins用于字段操作(例如,根据其他字段更改字段的值)
[ ] More complex input types.
[]更复杂的输入类型。
[ ] Nuxt plugin istall
[] Nuxt插件istall
[ ] Better docs & online examples
[]更好的文档和在线示例
[ ] Storybook
[]故事书
翻译自: https://vuejsexamples.com/easy-way-to-dynamically-create-reactive-forms-in-vue/
react 简单还是vue