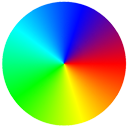
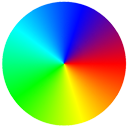
HTML5 Radial Gradient The one of our readers asked me – what if generate a radial gradient with html5 instead of using ready images (for our’s color picker which we developed several days ago). And, I decided, why not? This is not so difficult, and it would be useful for everybody. The main idea is to separate a circle into multiple sectors, and – to fill these sectors with a radial color (which depends on an angle). After working for a while – I got the desired result – canvas-based radial gradient.
HTML5径向渐变我们的一位读者问我-如果用html5而不是使用现成的图像生成径向渐变(针对我们几天前开发的颜色选择器)会怎样? 而且,我决定,为什么不呢? 这并不是那么困难,并且对每个人都有用。 主要思想是将一个圆分成多个扇区,并且–用径向颜色(取决于角度)填充这些扇区。 经过一段时间的工作–我得到了预期的结果–基于画布的径向渐变。
现场演示
If you are ready – let’s start!
如果您准备好了,那就开始吧!
步骤1. HTML (Step 1. HTML)
As usual (for all canvas-based demos) we have a very basic html markup (with a single canvas object inside):
像往常一样(对于所有基于画布的演示),我们有一个非常基本的html标记(内部有一个画布对象):
<!DOCTYPE html>
<html lang="en" >
<head>
<meta charset="utf-8" />
<meta name="author" content="Script Tutorials" />
<title>HTML5 Radial Gradient | Script Tutorials</title>
<!-- add styles -->
<link href="css/main.css" rel="stylesheet" type="text/css" />
<!-- add script -->
<script src="js/script.js"></script>
</head>
<body>
<div class="container">
<canvas id="gradient" width="500" height="500" tabindex="1"></canvas>
</div>
</body>
</html>
<!DOCTYPE html>
<html lang="en" >
<head>
<meta charset="utf-8" />
<meta name="author" content="Script Tutorials" />
<title>HTML5 Radial Gradient | Script Tutorials</title>
<!-- add styles -->
<link href="css/main.css" rel="stylesheet" type="text/css" />
<!-- add script -->
<script src="js/script.js"></script>
</head>
<body>
<div class="container">
<canvas id="gradient" width="500" height="500" tabindex="1"></canvas>
</div>
</body>
</html>
第2步:JS (Step 2. JS)
Now, please create an empty ‘script.js’ file (inside ‘js’ folder) and put this code inside (this is full JS code of our HTML5 Radial Gradient script). I’ll explain the main functionality below this code.
现在,请创建一个空的'script.js'文件(位于'js'文件夹内)并将此代码放入其中(这是我们HTML5径向渐变脚本的完整JS代码)。 我将在此代码下方解释主要功能。
js / script.js (js/script.js)
// Get angle color function
function getAngleColor(angle) {
var color, d;
if (angle < Math.PI * 2 / 5) { // angle: 0-72
d = 255 / (Math.PI * 2 / 5) * angle;
color = '255,' + Math.round(d) + ',0'; // color: 255,0,0 - 255,255,0
} else if (angle < Math.PI * 4 / 5) { // angle: 72-144
d = 255 / (Math.PI * 2 / 5) * (angle - Math.PI * 2 / 5);
color = (255 - Math.round(d)) + ',255,0'; // color: 255,255,0 - 0,255,0
} else if (angle < Math.PI * 6 / 5) { // angle: 144-216
d = 255 / (Math.PI * 2 / 5) * (angle - Math.PI * 4 / 5);
color = '0,255,' + Math.round(d); // color: 0,255,0 - 0,255,255
} else if (angle < Math.PI * 8 / 5) { // angle: 216-288
d = 255 / (Math.PI * 2 / 5) * (angle - Math.PI * 6 / 5);
color = '0,'+(255 - Math.round(d)) + ',255'; // color: 0,255,255 - 0,0,255
} else { // angle: 288-360
d = 255 / (Math.PI * 2 / 5) * (angle - Math.PI * 8 / 5);
color = Math.round(d) + ',0,' + (255 - Math.round(d)) ; // color: 0,0,255 - 255,0,0
}
return color;
}
// inner variables
var iSectors = 360;
var iSectorAngle = (360 / iSectors) / 180 * Math.PI; // in radians
// Draw radial gradient function
function drawGradient() {
// prepare canvas and context objects
var canvas = document.getElementById('gradient');
var ctx = canvas.getContext('2d');
// clear canvas
ctx.clearRect(0, 0, ctx.canvas.width, ctx.canvas.height);
// save current context
ctx.save();
// move to center
ctx.translate(canvas.width / 2, canvas.height / 2);
// draw all sectors
for (var i = 0; i < iSectors; i++) {
// start and end angles (in radians)
var startAngle = 0;
var endAngle = startAngle + iSectorAngle;
// radius for sectors
var radius = (canvas.width / 2) - 1;
// get angle color
var color = getAngleColor(iSectorAngle * i);
// draw a sector
ctx.beginPath();
ctx.moveTo(0, 0);
ctx.arc(0, 0, radius, startAngle, endAngle, false);
ctx.closePath();
// stroke a sector
ctx.strokeStyle = 'rgb('+color+')';
ctx.stroke();
// fill a sector
ctx.fillStyle = 'rgb('+color+')';
ctx.fill();
// rotate to the next sector
ctx.rotate(iSectorAngle);
}
// restore context
ctx.restore();
}
// initialization
if(window.attachEvent) {
window.attachEvent('onload', drawGradient);
} else {
if(window.onload) {
var curronload = window.onload;
var newonload = function() {
curronload();
drawGradient();
};
window.onload = newonload;
} else {
window.onload = drawGradient;
}
}
// Get angle color function
function getAngleColor(angle) {
var color, d;
if (angle < Math.PI * 2 / 5) { // angle: 0-72
d = 255 / (Math.PI * 2 / 5) * angle;
color = '255,' + Math.round(d) + ',0'; // color: 255,0,0 - 255,255,0
} else if (angle < Math.PI * 4 / 5) { // angle: 72-144
d = 255 / (Math.PI * 2 / 5) * (angle - Math.PI * 2 / 5);
color = (255 - Math.round(d)) + ',255,0'; // color: 255,255,0 - 0,255,0
} else if (angle < Math.PI * 6 / 5) { // angle: 144-216
d = 255 / (Math.PI * 2 / 5) * (angle - Math.PI * 4 / 5);
color = '0,255,' + Math.round(d); // color: 0,255,0 - 0,255,255
} else if (angle < Math.PI * 8 / 5) { // angle: 216-288
d = 255 / (Math.PI * 2 / 5) * (angle - Math.PI * 6 / 5);
color = '0,'+(255 - Math.round(d)) + ',255'; // color: 0,255,255 - 0,0,255
} else { // angle: 288-360
d = 255 / (Math.PI * 2 / 5) * (angle - Math.PI * 8 / 5);
color = Math.round(d) + ',0,' + (255 - Math.round(d)) ; // color: 0,0,255 - 255,0,0
}
return color;
}
// inner variables
var iSectors = 360;
var iSectorAngle = (360 / iSectors) / 180 * Math.PI; // in radians
// Draw radial gradient function
function drawGradient() {
// prepare canvas and context objects
var canvas = document.getElementById('gradient');
var ctx = canvas.getContext('2d');
// clear canvas
ctx.clearRect(0, 0, ctx.canvas.width, ctx.canvas.height);
// save current context
ctx.save();
// move to center
ctx.translate(canvas.width / 2, canvas.height / 2);
// draw all sectors
for (var i = 0; i < iSectors; i++) {
// start and end angles (in radians)
var startAngle = 0;
var endAngle = startAngle + iSectorAngle;
// radius for sectors
var radius = (canvas.width / 2) - 1;
// get angle color
var color = getAngleColor(iSectorAngle * i);
// draw a sector
ctx.beginPath();
ctx.moveTo(0, 0);
ctx.arc(0, 0, radius, startAngle, endAngle, false);
ctx.closePath();
// stroke a sector
ctx.strokeStyle = 'rgb('+color+')';
ctx.stroke();
// fill a sector
ctx.fillStyle = 'rgb('+color+')';
ctx.fill();
// rotate to the next sector
ctx.rotate(iSectorAngle);
}
// restore context
ctx.restore();
}
// initialization
if(window.attachEvent) {
window.attachEvent('onload', drawGradient);
} else {
if(window.onload) {
var curronload = window.onload;
var newonload = function() {
curronload();
drawGradient();
};
window.onload = newonload;
} else {
window.onload = drawGradient;
}
}
Once our window has loaded (onload event) we draw our radial gradient (drawGradient function). In the beginning we prepare canvas and context objects (as usual), after – more interesting: as I told, we are going to split whole circle (360 degrees) into sectors (I decided to use 360 sectors for more smooth gradient). In order to draw every sector we should jump to the center, draw an arc, and then – we need to fill this area (sector) with a certain color. To generate radial color we have to return a certain color depending on an angle. I defined ‘getAngleColor’ function for this purpose. Once we have drawn a sector – we draw next sector (until we finish with all of them to build whole radial circle).
加载窗口(onload事件)后,我们绘制径向渐变(drawGradient函数)。 首先,我们准备画布和上下文对象(通常),然后–更加有趣:正如我所告诉的,我们将把整个圆(360度)划分为多个扇区(我决定使用360个扇区来获得更平滑的渐变)。 为了绘制每个扇区,我们应该跳到中心,画一条弧,然后–我们需要用某种颜色填充该区域(扇区)。 要生成径向颜色,我们必须根据角度返回某种颜色。 为此,我定义了“ getAngleColor”函数。 一旦绘制了一个扇形,便绘制了下一个扇形(直到我们完成所有这些绘制,以建立整个径向圆)。
现场演示
[sociallocker]
[社交储物柜]
打包下载
[/sociallocker]
[/ sociallocker]
结论 (Conclusion)
That’s all for today, we have just finished building own html5 radial gradient element. See you next time, good luck!
今天就这些,我们已经完成了构建自己的html5径向渐变元素的工作。 下次见,祝你好运!
翻译自: https://www.script-tutorials.com/html5-radial-gradient/