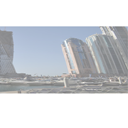
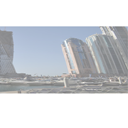
Rotate slider with easing effects tutorial. Today I decide to make another one slider (jQuery), where image is scrolled on its axis (emulating 3D). Also I will allow to use custom easing effects for switching of images. In result – this will our own jQuery plugin.
使用缓动效果教程旋转滑块。 今天,我决定制作另一个滑块(jQuery),在该滑块上图像沿其轴滚动(模拟3D)。 另外,我将允许使用自定义缓动效果来切换图像。 结果–这将是我们自己的jQuery插件。
Here are our package and demo:
这是我们的软件包和演示:
现场演示
[sociallocker]
[社交储物柜]
打包下载
[/sociallocker]
[/ sociallocker]
Lets start coding !
让我们开始编码!
步骤1. HTML (Step 1. HTML)
As usual, we start with the HTML. This is source code of our sample:
和往常一样,我们从HTML开始。 这是我们示例的源代码:
index.html (index.html)
<!DOCTYPE html>
<html lang="en"><head>
<meta http-equiv="content-type" content="text/html; charset=UTF-8">
<title>Creating rotate slider with easing effects | Script tutorials demo</title>
<link rel="stylesheet" href="css/main.css" type="text/css" />
<script type="text/javascript" src="js/jquery-1.5.2.min.js"></script>
<script type="text/javascript" src="js/jquery.easing.1.3.js"></script>
<script type="text/javascript" src="js/main.js"></script>
</head>
<body>
<div class="example">
<h3><a href="#">My Slider2 example</a></h3>
<div class="my_slider" id="slider1">
<img src="data_images/pic1.jpg" alt="" />
<img src="data_images/pic2.jpg" alt="" />
<img src="data_images/pic3.jpg" alt="" />
<img src="data_images/pic4.jpg" alt="" />
<img src="data_images/pic5.jpg" alt="" />
</div>
<div class="my_slider" id="slider2">
<img src="data_images/pic6.jpg" alt="" />
<img src="data_images/pic7.jpg" alt="" />
<img src="data_images/pic8.jpg" alt="" />
<img src="data_images/pic9.jpg" alt="" />
<img src="data_images/pic10.jpg" alt="" />
</div>
</div>
</body></html>
<!DOCTYPE html>
<html lang="en"><head>
<meta http-equiv="content-type" content="text/html; charset=UTF-8">
<title>Creating rotate slider with easing effects | Script tutorials demo</title>
<link rel="stylesheet" href="css/main.css" type="text/css" />
<script type="text/javascript" src="js/jquery-1.5.2.min.js"></script>
<script type="text/javascript" src="js/jquery.easing.1.3.js"></script>
<script type="text/javascript" src="js/main.js"></script>
</head>
<body>
<div class="example">
<h3><a href="#">My Slider2 example</a></h3>
<div class="my_slider" id="slider1">
<img src="data_images/pic1.jpg" alt="" />
<img src="data_images/pic2.jpg" alt="" />
<img src="data_images/pic3.jpg" alt="" />
<img src="data_images/pic4.jpg" alt="" />
<img src="data_images/pic5.jpg" alt="" />
</div>
<div class="my_slider" id="slider2">
<img src="data_images/pic6.jpg" alt="" />
<img src="data_images/pic7.jpg" alt="" />
<img src="data_images/pic8.jpg" alt="" />
<img src="data_images/pic9.jpg" alt="" />
<img src="data_images/pic10.jpg" alt="" />
</div>
</div>
</body></html>
Here we can see 2 defined sliders with images. Just DIV (parent) with images inside).
在这里我们可以看到2个带有图像的已定义滑块。 只是DIV(父级),里面有图片)。
步骤2. CSS (Step 2. CSS)
Here are used CSS file with styles of our demo:
以下是带有我们演示样式CSS文件:
css / main.css (css/main.css)
body{background:#eee;margin:0;padding:0}
.example{background:#FFF;width:500px;overflow:hidden;border:1px #000 solid;margin:20px auto;padding:15px;-moz-border-radius: 3px;-webkit-border-radius: 3px}
.my_slider {float:left}
/*My slider2 styles*/
.my_slider {
width:500px;
height:340px;
overflow: hidden;
position:relative;
list-style: none outside none;
padding:0;
margin:0;
}
.my_slider img {
position: absolute;
top: 0px;
left: 0px;
opacity:0;
width:500px;
}
.my_slider img:first-child {
opacity:1;
}
body{background:#eee;margin:0;padding:0}
.example{background:#FFF;width:500px;overflow:hidden;border:1px #000 solid;margin:20px auto;padding:15px;-moz-border-radius: 3px;-webkit-border-radius: 3px}
.my_slider {float:left}
/*My slider2 styles*/
.my_slider {
width:500px;
height:340px;
overflow: hidden;
position:relative;
list-style: none outside none;
padding:0;
margin:0;
}
.my_slider img {
position: absolute;
top: 0px;
left: 0px;
opacity:0;
width:500px;
}
.my_slider img:first-child {
opacity:1;
}
步骤3. JS (Step 3. JS)
Here are our main JS file:
这是我们的主要JS文件:
js / main.js (js/main.js)
(function($){
$.fn.MySlider = function(interval, e, d) {
var slides = $(this.selector).children();
var amount = slides.length;
var w = slides[0].width;
var h = slides[0].height;
var hw = h / 2;
var eas = e;
var dur = d;
var iCur=0;
var iNext=1;
// we will animate opacity, height and top property of current and next slides
function run() {
$(slides[iNext]).animate({
opacity: 0,
height: 0,
top: hw,
}, {
duration: dur,
specialEasing: {
height: eas
}
});
$(slides[iCur]).animate({
opacity: 0,
height: 0,
top: hw,
}, {
duration: dur,
specialEasing: {
height: eas
},
complete: function() {
$(slides[iCur]).animate({
opacity: 0,
height: h,
top:0,
}, {
duration: dur,
specialEasing: {
height: eas
}
});
$(slides[iNext]).animate({
opacity: 1,
height: h,
top:0,
}, {
duration: dur,
specialEasing: {
height: eas
}
});
iCur++;
iNext++;
if (iCur >= amount) {
iCur = 0;
}
if (iNext >= amount) {
iNext = 0;
}
}
});
// loop
setTimeout(run, interval);
}
// first run
if (amount > 1)
setTimeout(run, dur / 2);
};
})(jQuery);
// custom initialization
jQuery(window).load(function() {
$('#slider1').MySlider(5000, 'linear', 1000);
$('#slider2').MySlider(5000, 'easeOutCirc', 1000);
});
(function($){
$.fn.MySlider = function(interval, e, d) {
var slides = $(this.selector).children();
var amount = slides.length;
var w = slides[0].width;
var h = slides[0].height;
var hw = h / 2;
var eas = e;
var dur = d;
var iCur=0;
var iNext=1;
// we will animate opacity, height and top property of current and next slides
function run() {
$(slides[iNext]).animate({
opacity: 0,
height: 0,
top: hw,
}, {
duration: dur,
specialEasing: {
height: eas
}
});
$(slides[iCur]).animate({
opacity: 0,
height: 0,
top: hw,
}, {
duration: dur,
specialEasing: {
height: eas
},
complete: function() {
$(slides[iCur]).animate({
opacity: 0,
height: h,
top:0,
}, {
duration: dur,
specialEasing: {
height: eas
}
});
$(slides[iNext]).animate({
opacity: 1,
height: h,
top:0,
}, {
duration: dur,
specialEasing: {
height: eas
}
});
iCur++;
iNext++;
if (iCur >= amount) {
iCur = 0;
}
if (iNext >= amount) {
iNext = 0;
}
}
});
// loop
setTimeout(run, interval);
}
// first run
if (amount > 1)
setTimeout(run, dur / 2);
};
})(jQuery);
// custom initialization
jQuery(window).load(function() {
$('#slider1').MySlider(5000, 'linear', 1000);
$('#slider2').MySlider(5000, 'easeOutCirc', 1000);
});
Our new slider accepting 3 variables: interval between sliding, easing method, and duration of switching photos effect. Here are sample: $(‘#slider1’).MySlider(5000, ‘linear’, 1000);
我们的新滑块接受3个变量:滑动间隔,缓动方法和切换照片效果的持续时间。 以下是示例:$('#slider1')。MySlider(5000,'linear',1000);
js / jquery-1.5.2.min.js和js / jquery.easing.1.3.js (js/jquery-1.5.2.min.js and js/jquery.easing.1.3.js)
This is default jQuery library and easing addon. Available in our package.
这是默认的jQuery库和缓动插件。 在我们的包装中可用。
现场演示
结论 (Conclusion)
Today we have learned how to create another slider with scrolling images on its axis. Hope that you will like that effect and you will use this in your own projects. Good luck!
今天,我们已经学习了如何创建另一个在其轴上滚动图像的滑块。 希望您会喜欢这种效果,并且可以在自己的项目中使用它。 祝好运!
翻译自: https://www.script-tutorials.com/creating-rotate-slider-with-easing-effects/