ajax评论系统
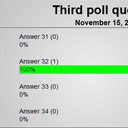
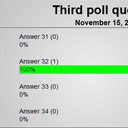
Creating own ajax poll system. Today I prepared new interesting tutorial – we will creating own Poll system (AJAX) for your projects with PHP. Polls, answers and results I going to keep in single SQL table. When we will vote for one of variants – jQuery will POST data, and then we will animate our results in realtime.
创建自己的ajax轮询系统。 今天,我准备了新的有趣的教程–我们将使用PHP为您的项目创建自己的投票系统(AJAX)。 民意测验,答案和结果我将保留在单个SQL表中。 当我们投票支持一种变体时-jQuery将发布数据,然后我们将对结果进行实时动画处理。
现场演示
[sociallocker]
[社交储物柜]
打包下载
[/sociallocker]
[/ sociallocker]
Now – download the source files and lets start coding !
现在–下载源文件并开始编码!
步骤1. SQL (Step 1. SQL)
We will need to add one table into our database:
我们将需要在数据库中添加一个表:
CREATE TABLE `s183_polls` (
`id` int(10) unsigned NOT NULL auto_increment,
`title` varchar(255) default '',
`answers` text NOT NULL,
`results` varchar(60) NOT NULL default '',
`total_votes` int(10) NOT NULL default '0',
`when` int(10) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
INSERT INTO `s183_polls` (`id`, `title`, `answers`, `results`, `total_votes`, `when`) VALUES
(NULL, 'First poll question', 'Answer 1<sep>Answer 2<sep>Answer 3<sep>Answer 4', '', 0, UNIX_TIMESTAMP()),
(NULL, 'Second poll question', 'Answer 21<sep>Answer 22<sep>Answer 23<sep>Answer 24', '', 0, UNIX_TIMESTAMP()+1),
(NULL, 'Third poll question', 'Answer 31<sep>Answer 32<sep>Answer 33<sep>Answer 34', '', 0, UNIX_TIMESTAMP()+2),
(NULL, 'Forth poll question', 'Answer 41<sep>Answer 42<sep>Answer 43<sep>Answer 44', '', 0, UNIX_TIMESTAMP()+3),
(NULL, 'Fifth poll question', 'Answer 51<sep>Answer 52<sep>Answer 53<sep>Answer 54', '', 0, UNIX_TIMESTAMP()+4);
CREATE TABLE `s183_polls` (
`id` int(10) unsigned NOT NULL auto_increment,
`title` varchar(255) default '',
`answers` text NOT NULL,
`results` varchar(60) NOT NULL default '',
`total_votes` int(10) NOT NULL default '0',
`when` int(10) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
INSERT INTO `s183_polls` (`id`, `title`, `answers`, `results`, `total_votes`, `when`) VALUES
(NULL, 'First poll question', 'Answer 1<sep>Answer 2<sep>Answer 3<sep>Answer 4', '', 0, UNIX_TIMESTAMP()),
(NULL, 'Second poll question', 'Answer 21<sep>Answer 22<sep>Answer 23<sep>Answer 24', '', 0, UNIX_TIMESTAMP()+1),
(NULL, 'Third poll question', 'Answer 31<sep>Answer 32<sep>Answer 33<sep>Answer 34', '', 0, UNIX_TIMESTAMP()+2),
(NULL, 'Forth poll question', 'Answer 41<sep>Answer 42<sep>Answer 43<sep>Answer 44', '', 0, UNIX_TIMESTAMP()+3),
(NULL, 'Fifth poll question', 'Answer 51<sep>Answer 52<sep>Answer 53<sep>Answer 54', '', 0, UNIX_TIMESTAMP()+4);
This is pur main Polls table.
这是pur主投票表。
步骤2. PHP (Step 2. PHP)
Here are source code of our main file:
这是我们主文件的源代码:
index.php (index.php)
<?php
// disable warnings
if (version_compare(phpversion(), "5.3.0", ">=") == 1)
error_reporting(E_ALL & ~E_NOTICE & ~E_DEPRECATED);
else
error_reporting(E_ALL & ~E_NOTICE);
require_once('classes/CMySQL.php'); // including service class to work with database
if ($_POST['do'] == 'vote') { // in case if we submitted poll
$iPollId = (int)$_POST['id'];
$iAnswer = (int)$_POST['answer'];
if ($iPollId && $iAnswer >= 0 && ! isset($_COOKIE['av' . $iPollId])) {
// get poll info
$aPollInfo = $GLOBALS['MySQL']->getRow("SELECT * FROM `s183_polls` WHERE `id` = '{$iPollId}'");
// updating of poll results
$aAnswers = explode('<sep>', $aPollInfo['answers']);
$iCnt = count($aAnswers);
$aVotes = ($aPollInfo['results'] == '') ? array_fill(0, $iCnt, 0) : explode('<sep>', $aPollInfo['results']);
$aVotes[$iAnswer]++;
$iVotesCount = array_sum($aVotes);
$sVotes = implode('<sep>', $aVotes);
$GLOBALS['MySQL']->res("UPDATE `s183_polls` SET `results` = '{$sVotes}', `total_votes` = {$iVotesCount} WHERE `id` = {$iPollId}");
// recalculation of percents
$iVotesCnt = $aPollInfo['total_votes'] + 1;
$aPercents = array();
foreach ($aAnswers as $i => $sAnswer) {
$aPercents[$i] = round( (0 != $iVotesCnt ? (( $aVotes[$i] / $iVotesCnt ) * 100) : 0), 1);
}
setcookie('av' . $iPollId, '1', time() + 24*3600, '/'); // easy protection from duplicate votes
// return back to JS
echo json_encode($aPercents);
exit;
} else {
exit;
}
}
$sCode = '';
$iItemId = (int)$_GET['id'];
if ($iItemId) { // View item output
$aItemInfo = $GLOBALS['MySQL']->getRow("SELECT * FROM `s183_polls` WHERE `id` = '{$iItemId}'"); // get poll info
$aAnswers = explode('<sep>', $aItemInfo['answers']);
$iCnt = count($aAnswers);
$aVotes = ($aItemInfo['results'] == '') ? array_fill(0, $iCnt, 0) : explode('<sep>', $aItemInfo['results']);
$iVotesCnt = $aItemInfo['total_votes'];
$sAnswers = '';
foreach ($aAnswers as $i => $sAnswer) {
$fPercent = round((0 != $iVotesCnt ? (($aVotes[$i] / $iVotesCnt) * 100) : 0), 1);
$sAnswers .= "<div id='{$i}'><div>{$sAnswer} (<span>{$aVotes[$i]}</span>)</div><div class='row' style='width:{$fPercent}%'>{$fPercent}%</div></div>";
}
ob_start();
?>
<h1><?= $aItemInfo['title'] ?></h1>
<h3><?= date('F j, Y', $aItemInfo['when']) ?></h3><hr />
<div class="answers"><?= $sAnswers ?></div>
<hr /><h3><a href="<?= $_SERVER['PHP_SELF'] ?>">back</a></h3>
<script>
$(function(){
$('.answers > div').click(function () {
var answer = $(this).attr('id');
var $span = $(this).find('span');
$.post('<?= $_SERVER['PHP_SELF'] ?>', {id: <?= $iItemId ?>, answer: answer, do: 'vote'},
function(data){
if (data) {
var da = eval('(' + data + ')');
for (var p in da) {
$($('.answers > div .row')[p]).animate({
width: da[p] + "%"
}, 500);
$($('.answers > div .row')[p]).text(da[p] + "%");
}
$span.text(parseInt($span.text()) + 1);
}
}
);
});
});
</script>
<?
$sCode .= ob_get_clean();
} else {
$sCode .= '<h1>List of polls:</h1>';
// taking info about all polls from database
$aItems = $GLOBALS['MySQL']->getAll("SELECT * FROM `s183_polls` ORDER by `when` ASC");
foreach ($aItems as $i => $aItemInfo) {
$sCode .= '<h2><a href="'.$_SERVER['PHP_SELF'].'?id='.$aItemInfo['id'].'">'.$aItemInfo['title'].'</a></h2>';
}
}
?>
<!DOCTYPE html>
<html lang="en" >
<head>
<meta charset="utf-8" />
<title>Creating own ajax poll system | Script Tutorials</title>
<link href="css/main.css" rel="stylesheet" type="text/css" />
<script type="text/javascript" src="js/jquery-1.5.2.min.js"></script>
</head>
<body>
<div class="container">
<?= $sCode ?>
</div>
<footer>
<h2>Creating own own ajax poll system</h2>
<a href="https://www.script-tutorials.com/creating-own-ajax-poll-system/" class="stuts">Back to original tutorial on <span>Script Tutorials</span></a>
</footer>
</body>
</html>
<?php
// disable warnings
if (version_compare(phpversion(), "5.3.0", ">=") == 1)
error_reporting(E_ALL & ~E_NOTICE & ~E_DEPRECATED);
else
error_reporting(E_ALL & ~E_NOTICE);
require_once('classes/CMySQL.php'); // including service class to work with database
if ($_POST['do'] == 'vote') { // in case if we submitted poll
$iPollId = (int)$_POST['id'];
$iAnswer = (int)$_POST['answer'];
if ($iPollId && $iAnswer >= 0 && ! isset($_COOKIE['av' . $iPollId])) {
// get poll info
$aPollInfo = $GLOBALS['MySQL']->getRow("SELECT * FROM `s183_polls` WHERE `id` = '{$iPollId}'");
// updating of poll results
$aAnswers = explode('<sep>', $aPollInfo['answers']);
$iCnt = count($aAnswers);
$aVotes = ($aPollInfo['results'] == '') ? array_fill(0, $iCnt, 0) : explode('<sep>', $aPollInfo['results']);
$aVotes[$iAnswer]++;
$iVotesCount = array_sum($aVotes);
$sVotes = implode('<sep>', $aVotes);
$GLOBALS['MySQL']->res("UPDATE `s183_polls` SET `results` = '{$sVotes}', `total_votes` = {$iVotesCount} WHERE `id` = {$iPollId}");
// recalculation of percents
$iVotesCnt = $aPollInfo['total_votes'] + 1;
$aPercents = array();
foreach ($aAnswers as $i => $sAnswer) {
$aPercents[$i] = round( (0 != $iVotesCnt ? (( $aVotes[$i] / $iVotesCnt ) * 100) : 0), 1);
}
setcookie('av' . $iPollId, '1', time() + 24*3600, '/'); // easy protection from duplicate votes
// return back to JS
echo json_encode($aPercents);
exit;
} else {
exit;
}
}
$sCode = '';
$iItemId = (int)$_GET['id'];
if ($iItemId) { // View item output
$aItemInfo = $GLOBALS['MySQL']->getRow("SELECT * FROM `s183_polls` WHERE `id` = '{$iItemId}'"); // get poll info
$aAnswers = explode('<sep>', $aItemInfo['answers']);
$iCnt = count($aAnswers);
$aVotes = ($aItemInfo['results'] == '') ? array_fill(0, $iCnt, 0) : explode('<sep>', $aItemInfo['results']);
$iVotesCnt = $aItemInfo['total_votes'];
$sAnswers = '';
foreach ($aAnswers as $i => $sAnswer) {
$fPercent = round((0 != $iVotesCnt ? (($aVotes[$i] / $iVotesCnt) * 100) : 0), 1);
$sAnswers .= "<div id='{$i}'><div>{$sAnswer} (<span>{$aVotes[$i]}</span>)</div><div class='row' style='width:{$fPercent}%'>{$fPercent}%</div></div>";
}
ob_start();
?>
<h1><?= $aItemInfo['title'] ?></h1>
<h3><?= date('F j, Y', $aItemInfo['when']) ?></h3><hr />
<div class="answers"><?= $sAnswers ?></div>
<hr /><h3><a href="<?= $_SERVER['PHP_SELF'] ?>">back</a></h3>
<script>
$(function(){
$('.answers > div').click(function () {
var answer = $(this).attr('id');
var $span = $(this).find('span');
$.post('<?= $_SERVER['PHP_SELF'] ?>', {id: <?= $iItemId ?>, answer: answer, do: 'vote'},
function(data){
if (data) {
var da = eval('(' + data + ')');
for (var p in da) {
$($('.answers > div .row')[p]).animate({
width: da[p] + "%"
}, 500);
$($('.answers > div .row')[p]).text(da[p] + "%");
}
$span.text(parseInt($span.text()) + 1);
}
}
);
});
});
</script>
<?
$sCode .= ob_get_clean();
} else {
$sCode .= '<h1>List of polls:</h1>';
// taking info about all polls from database
$aItems = $GLOBALS['MySQL']->getAll("SELECT * FROM `s183_polls` ORDER by `when` ASC");
foreach ($aItems as $i => $aItemInfo) {
$sCode .= '<h2><a href="'.$_SERVER['PHP_SELF'].'?id='.$aItemInfo['id'].'">'.$aItemInfo['title'].'</a></h2>';
}
}
?>
<!DOCTYPE html>
<html lang="en" >
<head>
<meta charset="utf-8" />
<title>Creating own ajax poll system | Script Tutorials</title>
<link href="css/main.css" rel="stylesheet" type="text/css" />
<script type="text/javascript" src="js/jquery-1.5.2.min.js"></script>
</head>
<body>
<div class="container">
<?= $sCode ?>
</div>
<footer>
<h2>Creating own own ajax poll system</h2>
<a href="https://www.script-tutorials.com/creating-own-ajax-poll-system/" class="stuts">Back to original tutorial on <span>Script Tutorials</span></a>
</footer>
</body>
</html>
When we open this page in first time – it will draw list of polls. Each poll unit have own page. At this page we going to display Poll title, date of adding and all possible answers. On click – script will send (POST) data to same script. In this moment we are adding this vote, and then we will send back new percentages for all variants. And jQuery will re-animate all these bars. I added my comments in most of places for better understanding.
当我们第一次打开此页面时,它将绘制民意调查列表。 每个轮询单元都有自己的页面。 在此页面上,我们将显示投票标题,添加日期和所有可能的答案。 单击时-脚本将发送(POST)数据到同一脚本。 在这一刻,我们将增加投票,然后我们将为所有变体发送新的百分比。 jQuery将为所有这些栏重新设置动画。 我在大多数地方都添加了我的评论,以便更好地理解。
I have another one PHP file in my project:
我的项目中还有一个PHP文件:
类/CMySQL.php (classes/CMySQL.php)
This is service class to work with database prepared by me. This is nice class which you can use too. Database connection details present in that class file in few variables, sure that you will able to configure this to your database. I will not publish sources of this file – this is not necessary right now. Available in package.
这是与我准备的数据库一起使用的服务类。 这是一个很好的类,您也可以使用。 该类文件中的几个变量都包含数据库连接的详细信息,请确保可以将其配置到数据库中。 我不会发布该文件的来源-现在没有必要。 封装形式。
步骤3. JS (Step 3. JS)
js / jquery-1.5.2.min.js (js/jquery-1.5.2.min.js)
This is just jQuery library. Available in package.
这只是jQuery库。 封装形式。
步骤4. CSS (Step 4. CSS)
Now – all used CSS styles:
现在-所有使用CSS样式:
css / main.css (css/main.css)
*{
margin:0;
padding:0;
}
body {
background-repeat:no-repeat;
background-color:#bababa;
background-image: -webkit-radial-gradient(600px 200px, circle, #eee, #bababa 40%);
background-image: -moz-radial-gradient(600px 200px, circle, #eee, #bababa 40%);
background-image: -o-radial-gradient(600px 200px, circle, #eee, #bababa 40%);
background-image: radial-gradient(600px 200px, circle, #eee, #bababa 40%);
color:#fff;
font:14px/1.3 Arial,sans-serif;
min-height:600px;
}
footer {
background-color:#212121;
bottom:0;
box-shadow: 0 -1px 2px #111111;
display:block;
height:70px;
left:0;
position:fixed;
width:100%;
z-index:100;
}
footer h2{
font-size:22px;
font-weight:normal;
left:50%;
margin-left:-400px;
padding:22px 0;
position:absolute;
width:540px;
}
footer a.stuts,a.stuts:visited{
border:none;
text-decoration:none;
color:#fcfcfc;
font-size:14px;
left:50%;
line-height:31px;
margin:23px 0 0 110px;
position:absolute;
top:0;
}
footer .stuts span {
font-size:22px;
font-weight:bold;
margin-left:5px;
}
.container {
border:3px #111 solid;
color:#000;
margin:20px auto;
padding:15px;
position:relative;
text-align:center;
width:500px;
border-radius:15px;
-moz-border-radius:15px;
-webkit-border-radius:15px;
}
.answers > div {
cursor:pointer;
margin:0 0 0 40px;
padding:10px;
text-align:left;
}
.answers > div:hover {
background-color: rgba(255, 255, 255, 0.4);
}
.answers div .row {
background-color:#0f0;
}
*{
margin:0;
padding:0;
}
body {
background-repeat:no-repeat;
background-color:#bababa;
background-image: -webkit-radial-gradient(600px 200px, circle, #eee, #bababa 40%);
background-image: -moz-radial-gradient(600px 200px, circle, #eee, #bababa 40%);
background-image: -o-radial-gradient(600px 200px, circle, #eee, #bababa 40%);
background-image: radial-gradient(600px 200px, circle, #eee, #bababa 40%);
color:#fff;
font:14px/1.3 Arial,sans-serif;
min-height:600px;
}
footer {
background-color:#212121;
bottom:0;
box-shadow: 0 -1px 2px #111111;
display:block;
height:70px;
left:0;
position:fixed;
width:100%;
z-index:100;
}
footer h2{
font-size:22px;
font-weight:normal;
left:50%;
margin-left:-400px;
padding:22px 0;
position:absolute;
width:540px;
}
footer a.stuts,a.stuts:visited{
border:none;
text-decoration:none;
color:#fcfcfc;
font-size:14px;
left:50%;
line-height:31px;
margin:23px 0 0 110px;
position:absolute;
top:0;
}
footer .stuts span {
font-size:22px;
font-weight:bold;
margin-left:5px;
}
.container {
border:3px #111 solid;
color:#000;
margin:20px auto;
padding:15px;
position:relative;
text-align:center;
width:500px;
border-radius:15px;
-moz-border-radius:15px;
-webkit-border-radius:15px;
}
.answers > div {
cursor:pointer;
margin:0 0 0 40px;
padding:10px;
text-align:left;
}
.answers > div:hover {
background-color: rgba(255, 255, 255, 0.4);
}
.answers div .row {
background-color:#0f0;
}
现场演示
结论 (Conclusion)
Today we built the great AJAX Poll system for your website. Sure that this material will be useful for your own projects. Good luck in your work!
今天,我们为您的网站构建了出色的AJAX投票系统。 确保该材料将对您自己的项目有用。 祝您工作顺利!
翻译自: https://www.script-tutorials.com/creating-own-ajax-poll-system/
ajax评论系统