wordpress 调试
Debugging is an essential skill for any developer. This tutorial will show you 11 powerful ways to debug WordPress and PHP errors.
调试对于任何开发人员都是必不可少的技能。 本教程将向您展示11种调试WordPress和PHP错误的强大方法。
The first item in the list is the famous WP_Debug, then we’ll jump into some more advanced methods.
列表中的第一项是著名的WP_Debug,然后我们将跳入一些更高级的方法。
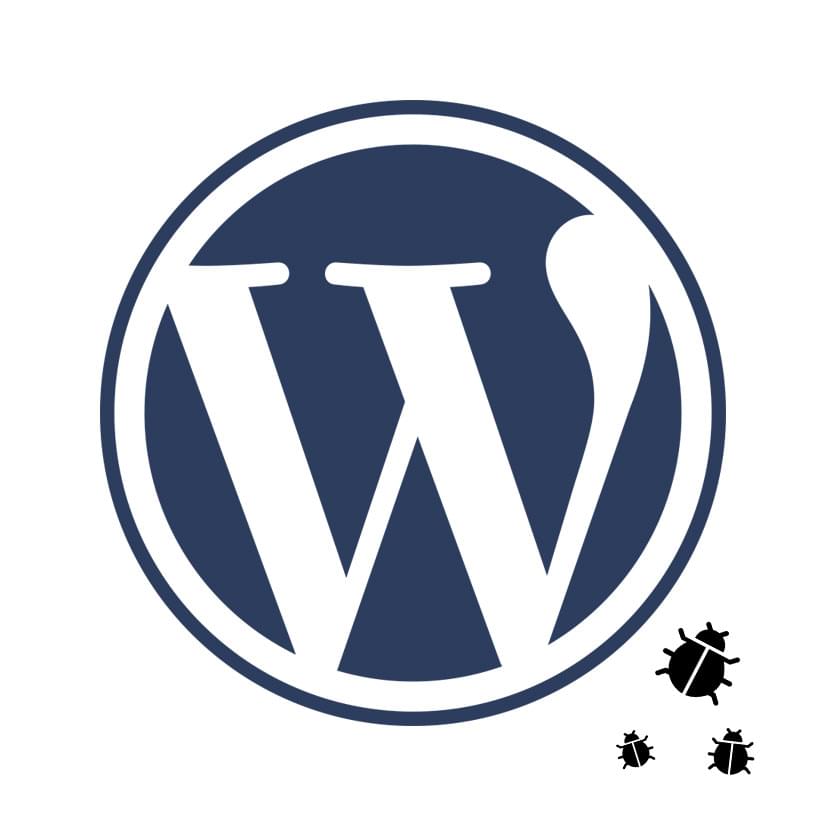
First, let’s list the types of common PHP errors:
首先,让我们列出常见PHP错误类型:
A – Notice: This is the least important error message you will see with PHP. It does not necessarily mean something is wrong, but there is possible improvement suggested.
A –注意:这是您将在PHP中看到的最不重要的错误消息。 这并不一定意味着出了什么问题,但是建议可以进行改进。
Example: null element passed to a function that expect a string.
示例:传递给期望字符串的函数的null元素。
B – Warning: It’s a more severe error, but does not cause script termination.
B –警告:这是一个更严重的错误,但不会导致脚本终止。
Example: giving include() a file that does not exist.
示例:给include()一个不存在的文件。
C – Fatal error: This is a dangerous indicator of something going really wrong, and the script terminated.
C –致命错误:这是某些错误的危险指示,脚本已终止。
Example: calling a non-existing function.
示例:调用一个不存在的函数。
1 – WP_DEBUG (1 – WP_DEBUG)
WordPress has a global constant to specify the needed level of debugging: WP_DEBUG. Along with this constant, comes two another important ones: WP_DEUBG_DISPLAY, and WP_DEBUG_LOG.
WordPress有一个全局常量来指定所需的调试级别:WP_DEBUG。 除了这个常量,还有两个重要的常量:WP_DEUBG_DISPLAY和WP_DEBUG_LOG。
WP_DEBUG is used to set the debugging mode on or off. WP_DEUBG_DISPLAY shows errors or hides them. Finally, WP_DEBUG_LOG saves your error messages in wp-content/debug.log.
WP_DEBUG用于将调试模式设置为打开或关闭。 WP_DEUBG_DISPLAY显示错误或隐藏错误。 最后,WP_DEBUG_LOG将您的错误消息保存在wp-content / debug.log中。
The three global constants can be set to true or false in wp-config.php, like this:
可以在wp-config.php中将这三个全局常量设置为true或false,如下所示:
define("WP_DEBUG&