px2rem api
In the first part of this series we used the API to get the latest photos from 500px and filter through them, and we built a user profile with the list of associated photos. In this part, we are going to give the user the ability to vote, favorite and comment on photos, and finally, we will give the users the ability to upload their own photos. Let’s get started.
在本系列的第一部分中,我们使用API从500像素中获取最新照片并对其进行过滤,并使用相关照片列表构建了一个用户个人资料。 在这一部分中,我们将使用户能够对照片进行投票,收藏和评论,最后,我们将使用户能够上传自己的照片。 让我们开始吧。
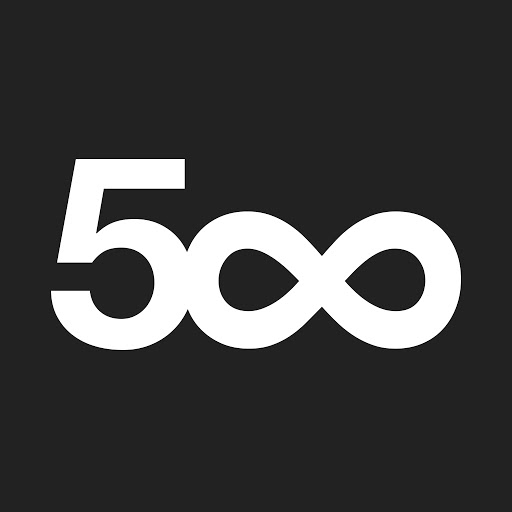
授权书 (Authorization)
When trying to access an API endpoint we need to check the authentication level required to access the resource. The API may require a consumer_key
or an OAuth.
尝试访问API端点时,我们需要检查访问资源所需的身份验证级别 。 API可能需要consumer_key
或OAuth 。
In the first part we registered a new application on 500px and received our consumer_key
and consumer_secret
. We use the Grant app to get a valid token
and token_secret
for testing.
在第一部分中,我们在500px上注册了一个新应用程序,并收到了consumer_key
和consumer_secret
。 我们使用Grant应用程序获取有效的token
和token_secret
进行测试。
认证方式 (Authentication)
After specifying your consumer_key
and consumer_secret
, you receive a token
and a token_secret
. These are going to give you the right to post and retrieve user content.
在指定了consumer_key
和consumer_secret
,您将收到一个token
和一个token_secret
。 这些将赋予您发布和检索用户内容的权利。
// bootstrap/start.php
App::singleton('pxoauth', function(){
$host = 'https://api.500px.com/v1/';
$consumer_key = 'YOUR CONSUMER KEY';
$consumer_secret = 'YOUR CONSUMER SECRET';
$token = 'GRANT TOKEN';
$token_secret = 'GRANT TOKEN SECRET';
$oauth = new PxOAuth($host, $consumer_key, $consumer_secret, $token, $token_secret);
return $oauth;
});
The PxOAuth
class wraps our communication with the 500px API.
PxOAuth
类包装了与500px API的通信。
// app/src/PxOAuth.php
class PxOAuth{
public $host;
public $client;
private $consumer_key;
private $consumer_secret;
private $token;
private $token_secret;
public function __construct($host, $consumer_key, $consumer_secret, $token, $token_secret){
$this->host = $host;
$this->consumer_key = $consumer_key;
$this->consumer_secret = $consumer_secret;
$this->token = $token;
$this->token_secret = $token_secret;
$params = [
'consumer_key' => $this->consumer_key,
'consumer_secret' => $this->consumer_secret,
'token' => $this->token,
'token_secret' => $this->token_secret
];
$oauth = new Oauth1($params);
$this->client = new Client([ 'base_url' => $this->host, 'defaults' => ['auth' => 'oauth']]);
$this->client->getEmitter()->attach($oauth);
// if app is in debug mode, we do logging
if( App::make('config')['app']['debug'] ) {
$this->addLogger();
}
}
private function addLogger(){
$log = new Logger('guzzle');
$log->pushHandler(new StreamHandler(__DIR__.'/../storage/logs/guzzle.log'));
$subscriber = new LogSubscriber($log, Formatter::DEBUG);
$this->client->getEmitter()->attach($subscriber);
}
}//class
When working with HTTP requests, it’s often useful to have some kind of logging. We add logging if the application is in debug mode.
处理HTTP请求时,进行某种日志记录通常很有用。 如果应用程序处于调试模式,我们将添加日志记录。
在照片上投票 (Voting on Photos)
If you used the 500px website, you could see that the user has the ability to vote on photos to increase their rating. We can retrieve the list of users who voted for a specific photo, and you can vote it up or down.
如果您使用500px网站,则可以看到用户可以对照片进行投票以提高其评分。 我们可以检索为某张照片投票的用户列表 ,您可以对其进行上下投票 。
// app/routes.php
Route::post('/ajax/photo/vote', ['uses' => 'PXController@vote']);
// app/controllers/PXController.php
public function vote(){
$photoId = Input::get("pid");
$px = App::make('pxoauth');
$url = "photos/{$photoId}/vote";
try {
$result = $px->client->post($url, ["body" => ['vote' => '1']]);
}
catch(RequestException $e){
$response = $e->getResponse();
if($response->getStatusCode() === 403){
return (string) $response->getBody();
}
return ["status" => 500, "error" => "A serious bug occurred."];
}
return (string) $result->getBody();
}
// public/js/vote_favorite.js
$('body').on('click', '.thumb .vote', function(e){
e.preventDefault();
$this = $(this);
var pid = $this.parents(".thumb").data("photo-id");
$.ajax({
url: "/ajax/photo/vote",
type: "POST",
dataType: "json",
data: {
pid: pid
},
success: function(data){
if(data.hasOwnProperty("error")){
alert(data.error);
}
else{
$this.text(data.photo.votes_count);
alert("Photo voted successfully");
}
}
});
});
The best way to implement the voting action is through AJAX. We are going to send an AJAX post request with the photo ID, and get back a JSON response, which either contains an error message or a refreshed photo. After retrieving the photo ID, we send a post request to the photos/photo_id/vote
endpoint along with a vote
parameter (0 or 1). If the request wasn’t successful we can catch the exception and test $response->getStatusCode()
. You can visit the docs to see the list of errors.
实施投票行动的最佳方法是通过AJAX。 我们将发送带有照片ID的AJAX发布请求,并获取JSON响应,其中包含错误消息或刷新的照片。 检索完照片ID后,我们将发布请求和vote
参数(0或1)发送到photos/photo_id/vote
端点。 如果请求不成功,我们可以捕获异常并测试$response->getStatusCode()
。 您可以访问文档以查看错误列表。
将照片标记为收藏 (Marking Photos as Favorite)
Marking photos as favorites is almost the same as upvoting them, except that you don’t pass any extra parameters in the body. You can see the docs for more details.
将照片标记为收藏与将照片标记为收藏几乎相同,只是您不会在正文中传递任何其他参数。 您可以查看文档以了解更多详细信息。
// app/routes.php
Route::post('/ajax/photo/favorite', ['uses' => 'PXController@favorite']);
// app/controllers/PXController.php
public function favorite(){
$photoId = Input::get("pid");
$px = App::make('pxoauth');
$url = "photos/{$photoId}/favorite";
try {
$result = $px->client->post($url);
}
catch(RequestException $e){
$response = $e->getResponse();
if($response->getStatusCode() === 403){
return (string) $response->getBody();
}
return ["status" => 500, "error" => "A serious bug occurred."];
}
return (string) $result->getBody();
}
// public/js/vote_favorite.js
$('body').on('click', '.thumb .favorite', function(e){
e.preventDefault();
$this = $(this);
var pid = $this.parents(".thumb").data("photo-id");
$.ajax({
url: "/ajax/photo/favorite",
type: "POST",
dataType: "json",
data: {
pid: pid
},
success: function(data){
if(data.hasOwnProperty("error")){
alert(data.error);
}
else{
$this.text(data.photo.favorites_count);
alert("Photo favourited successfully");
}
}
});
});
If you already voted or favorited a photo, you’ll get an error saying (Sorry, you have already voted for this Photo
). You can avoid that by disabling the link if the voted
or favorited
attribute inside a photo object is set to true
.
如果您已经投票或收藏了照片,则会出现一条错误消息( Sorry, you have already voted for this Photo
)。 如果将照片对象内的voted
或favorited
属性设置为true
则可以通过禁用链接来避免这种true
。
注释 (Comments)
Commenting systems are mandatory for users to give their opinions. The 500px API provides endpoints for dealing with comments. We split the process into three main parts.
评论系统是用户发表意见所必须的。 500px API提供了用于处理注释的端点。 我们将过程分为三个主要部分。
单张照片 (Single Photos)
In the first part, when the user clicked on a photo we opened the link on the 500px website. Now, we are going to implement our own single photo page.
在第一部分中,当用户单击照片时,我们打开了500px网站上的链接。 现在,我们将实现自己的单张照片页面。
The photos/:photo_id
endpoint accepts a list of parameters, and returns the selected photo or a not found error.
photos/:photo_id
端点接受参数列表,并返回所选照片或未找到错误。
// app/routes.php
Route::get('/photo/{id}', ['uses' => 'PXController@show']);
// app/controllers/PXController.php
public function show($id){
$px = App::make('pxoauth');
try {
$photo = $px->client->get("photos/{$id}?image_size=4")->json();
return View::make('single', ['photo' => $photo['photo']]);
}
catch(RequestException $e){
$response = $e->getResponse();
if($response->getStatusCode() === 404){
// handle 404: photo not found
}
}
}
// app/views/single.blade.php
...
<h1>{{ $photo['name'] }}</h1>
<p class="lead">
by <a href="/user/{{ $photo['user']['id'] }}">{{ $photo['user']['username'] }}</a>
</p>
<hr>
<p><span class="glyphicon glyphicon-time"></span> Posted on {{ $photo['created_at'] }}</p>
<hr>
<img class="img-responsive" src="{{ $photo['images'][0]['url'] }}" alt="">
<hr>
@if($photo['description'])
<p class="lead">{{ $photo['description'] }}</p>
@endif
<hr>
...
If the request was successful, we pass the photo object to the view, otherwise we catch the exception and handle the 404 error. You can visit the docs for more details.
如果请求成功,则将照片对象传递给视图,否则我们将捕获异常并处理404错误。 您可以访问文档以获取更多详细信息。
获得评论 (Getting Comments)
Retrieving comments can be done in two different ways. We can pass the comments
parameter to the single photo endpoint (photos/:id?comments
), or we can use the photos/:id/comments
endpoint. The second way allows us to retrieve nested comments, which makes it easier for us to print them. The API limits the number of comments per request to 20 and you can use the page
parameter to get the complete list.
可以两种不同方式检索评论。 我们可以将comments
参数传递到单个photo端点( photos/:id?comments
),也可以使用photos/:id/comments
端点。 第二种方法使我们能够检索嵌套的注释,这使我们更容易打印它们。 API将每个请求的评论数限制为20,并且您可以使用page
参数来获取完整列表。
// app/controllers/PXController.php
public function show($id){
$px = App::make('pxoauth');
try {
$photo = $px->client->get("photos/{$id}?image_size=4")->json();
$comments = $px->client->get("photos/{$id}/comments?nested=true")->json();
return View::make('single', ['photo' => $photo['photo'], 'comments' => $comments['comments']]);
}
catch(RequestException $e){
$response = $e->getResponse();
if($response->getStatusCode() === 404){
// handle 404: photo not found
}
}
}
// app/views/single.blade.php
@foreach($comments as $comment)
<div class="media">
<a class="pull-left" href="#">
<img class="media-object" src="{{ $comment['user']['userpic_url'] }}" alt="">
</a>
<div class="media-body">
<h4 class="media-heading">{{ $comment['user']['username'] }}
<small>{{ $comment['created_at'] }}</small>
</h4>
{{ $comment['body'] }}
@if(count($comment['replies']))
@foreach($comment['replies'] as $ncomment)
<div class="media">
<a class="pull-left" href="#">
<img class="media-object" src="{{ $ncomment['user']['userpic_url'] }}" alt="">
</a>
<div class="media-body">
<h4 class="media-heading">{{ $ncomment['user']['username'] }}
<small>{{ $comment['created_at'] }}</small>
</h4>
{{ $comment['body'] }}
</div>
</div>
@endforeach
@endif
</div>
</div>
@endforeach
Nested comments are inserted on the replies
array, and we can paginate comments using the total_pages
attribute. You can visit the docs to see the list of available parameters.
嵌套注释将插入到replies
数组中,我们可以使用total_pages
属性对注释进行分页。 您可以访问文档以查看可用参数列表。
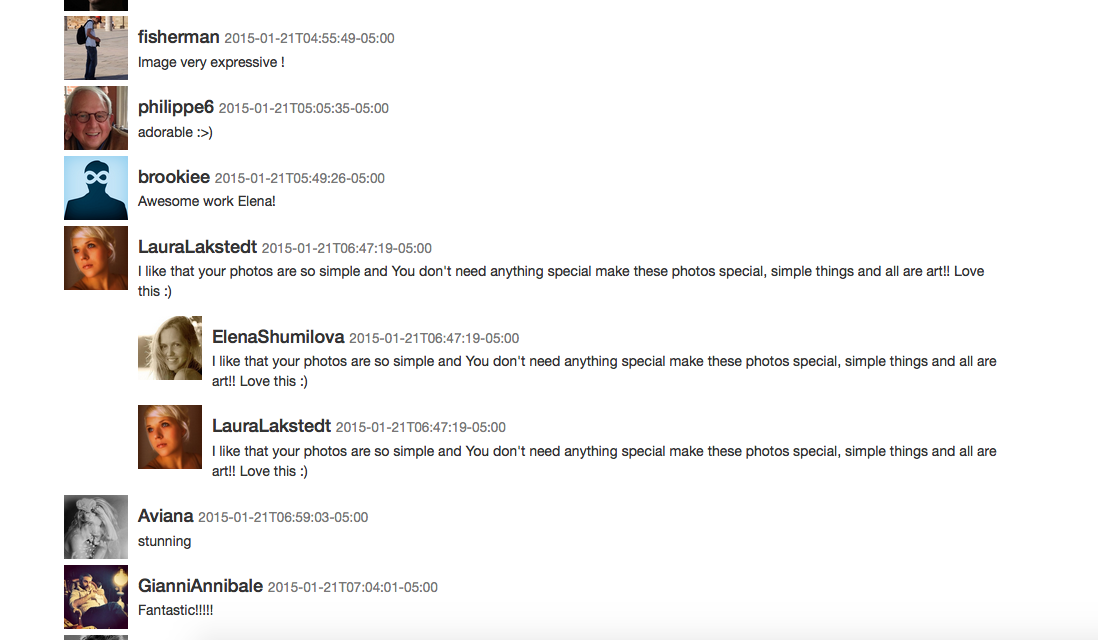
新评论 (New Comment)
When the user is viewing a photo, he can comment on the photo to express his emotions and opinion. In this tutorial, we won’t allow replying on comments, we can only post a new comment, but it’s basically the same.
当用户查看照片时,他可以在照片上发表评论以表达自己的情感和见解。 在本教程中,我们将不允许回复评论 ,我们只能发布新评论,但基本相同。
// app/views/single.blade.php
<div class="well">
<h4>Leave a Comment:</h4>
{{ Form::open(['url' => '/photo/comment', 'method' => 'post']) }}
<input type="hidden" name="pid" value="{{ $photo['id'] }}"/>
<div class="form-group">
<textarea name="comment" class="form-control" rows="3"></textarea>
</div>
<button type="submit" class="btn btn-primary">Submit</button>
{{ Form::close() }}
</div>
// app/routes.php
Route::post('/photo/comment', ['uses' => 'PXController@comment']);
// app/controllers/PXController.php
public function comment(){
$photoId = Input::get('pid');
$comment = Input::get('comment');
$px = App::make('pxoauth');
$result = $px->client->post("photos/{$photoId}/comments", ['body' => ['body' => $comment]])->json();
if($result['status'] != 200){
// handle 400: Bad request.
}
return Redirect::back();
}
The method retrieves the photo ID and the comment body. We then post a request to the photos/:photo_id/comments
endpoint which only accepts those two parameters, and returns a 200 status code if the comment was successfully posted. Otherwise, we handle the 400 or 404 error. You can check the docs for more details.
该方法检索照片ID和评论正文。 然后,我们将请求发布到photos/:photo_id/comments
端点,该请求仅接受这两个参数,如果注释成功发布,则返回200状态代码。 否则,我们将处理400或404错误。 您可以检查文档以了解更多详细信息。
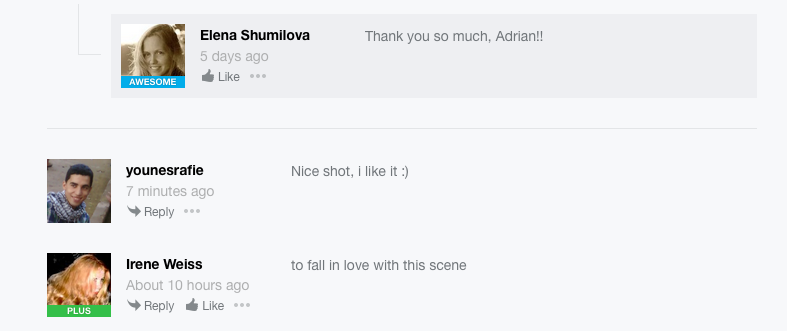
上载新照片 (Uploading New Photos)
When using a package like Guzzle, uploading files become a straight forward process. The API accepts many parameters, but we are only going to use a name
, description
and a photo file. You can visit the docs for the full list of parameters.
当使用像Guzzle这样的软件包时 ,上传文件成为简单的过程。 该API接受许多参数,但是我们将仅使用name
, description
和一个图片文件。 您可以访问文档以获取完整的参数列表。
// app/routes.php
Route::get('/upload', ['uses' => 'PagesController@photoUpload']);
Route::post('/photo/upload', ['as' => 'photo.upload', 'uses' => 'PXController@upload']);
// app/views/upload.blade.php
<div class="row">
{{ Form::open(['route' => 'photo.upload', 'files' => true]) }}
<div class="form-group">
{{ Form::label('name', 'Name: ') }}
{{ Form::text('name', null, ['class' => 'form-control']) }}
</div>
<div class="form-group">
{{ Form::label('description', 'Description: ') }}
{{ Form::textarea('description', null, ['class' => 'form-control']) }}
</div>
<div class="form-group">
{{ Form::label('photo', 'Upload a photo') }}
{{ Form::file('photo', null, ['class' => 'form-control']) }}
</div>
{{ Form::submit('Upload', ['class' => 'btn btn-primary']) }}
{{ Form::close() }}
</div>
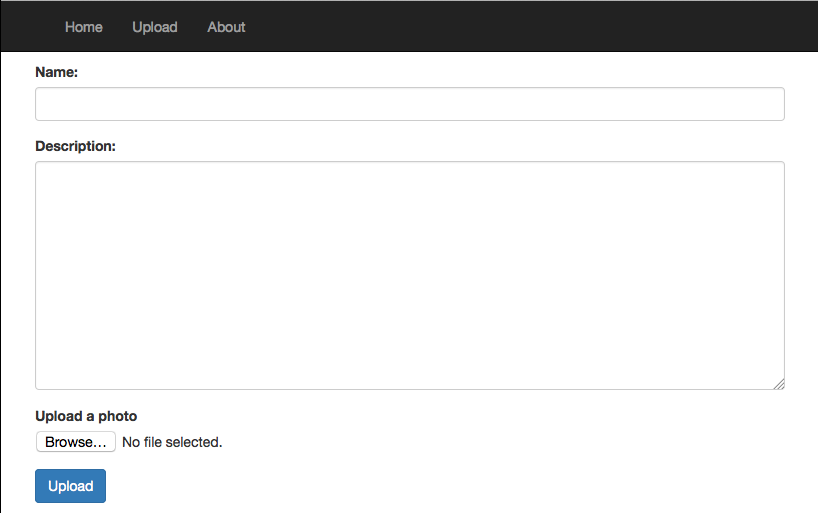
// app/controllers/PXController.php
public function upload(){
try {
$px = App::make('pxoauth');
$result = $px->client->post('photos/upload', [
'body' => [
'name' => Input::get('name'),
'description' => Input::get('description'),
'file' => fopen(Input::file('photo')->getPathname(), 'r'),
]
])->json();
// you may want to pass a success message
return Redirect::to("/photo/{$result['photo']['id']}");
}
catch(RequestException $e){
$response = $e->getResponse();
if($response->getStatusCode() === 422){
// handle 422: Server error
}
}
}//upload
When you want to upload some data through HTTP you need to deal with multipart/form-data
headers and reading file content and adding it to the request. You can read more about sending files with Guzzle here.
当您想通过HTTP上传一些数据时,您需要处理multipart/form-data
标头并读取文件内容并将其添加到请求中。 您可以在此处阅读有关使用Guzzle发送文件的更多信息。
The API returns a 200 status code along with the new photo on success, otherwise we get a 422 error if the file format is not supported, etc. We redirect the user to the new photo page.
如果成功,API将返回200状态代码以及新照片,否则,如果不支持文件格式,则会收到422错误。等等。我们将用户重定向到新照片页面。
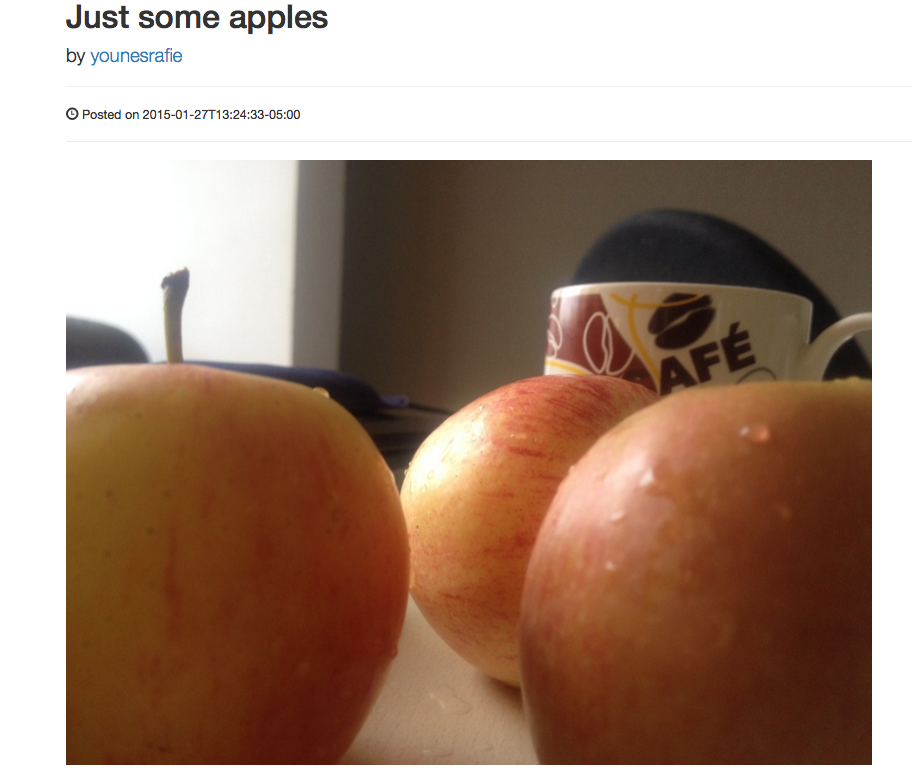
结论 (Conclusion)
The 500px API has more functionality than what we showed in this tutorial. You can check other API hack apps to get an idea of what is possible, and you can check the Github repository to test the final result. Let me know what you think in the comments below.
500px API比我们在本教程中展示的功能更多。 您可以检查其他API hack应用程序以了解可能的解决方案,还可以检查Github存储库以测试最终结果。 让我知道您在以下评论中的想法。
翻译自: https://www.sitepoint.com/commenting-upvoting-uploading-photos-500px-api/
px2rem api