城市简码
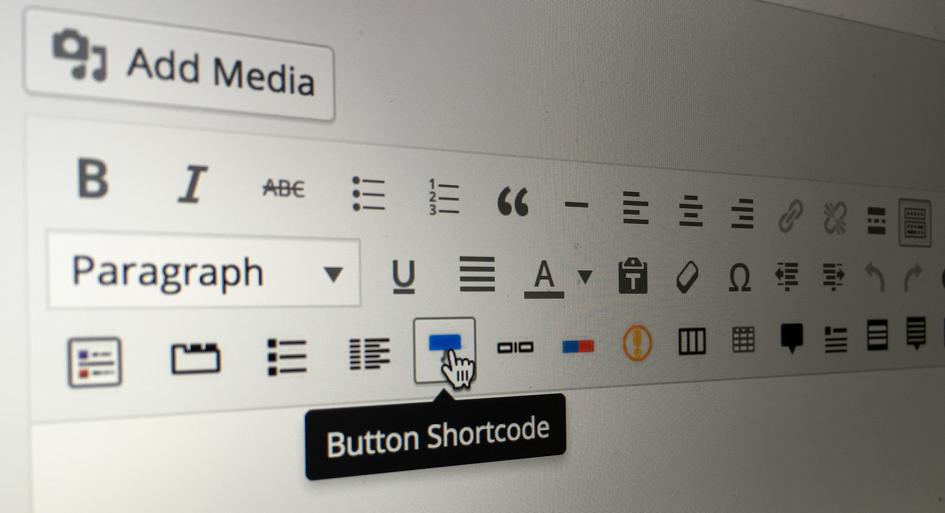
This article is part of a series created in partnership with SiteGround. Thank you for supporting the partners who make SitePoint possible.
本文是与SiteGround合作创建的系列文章的一部分。 感谢您支持使SitePoint成为可能的合作伙伴。
Ideally, WordPress authors should never need to edit raw HTML. You should enable plugins and custom meta boxes which allow the user to configure the page as necessary. Unfortunately, there are situations when it’s difficult or impractical to provide UI tools, e.g.:
理想情况下,WordPress作者永远不需要编辑原始HTML。 您应该启用插件和自定义元框,以便用户根据需要配置页面。 不幸的是,在某些情况下,提供UI工具非常困难或不切实际,例如:
- a widget provides numerous configuration options 小部件提供了许多配置选项
- similar widgets can appear multiple times in the page at any location 类似的小部件可以在页面中的任意位置多次出现
- widgets can be nested inside each other, such as a button overlaying a video within a sidebar 小部件可以彼此嵌套,例如在侧边栏中将视频覆盖的按钮
- a widget’s implementation changes, e.g. you switch from one video hosting platform to another. 小部件的实现发生了变化,例如,您从一个视频托管平台切换到另一个。
WordPress shortcodes are ideal for these situations. A shortcode allows the author to use text snippets such as [mywidget]
which places an HTML component in the rendered page without the need for coding.
WordPress短代码非常适合这些情况。 简码允许作者使用文本片段,例如[mywidget]
,它无需渲染即可在呈现的页面中放置HTML组件。
在哪里创建简码 (Where to Create Shortcodes)
Shortcodes are often created to aid custom plugin usage so you should place those within the plugin code itself. However, you can also place shortcode definitions within a theme’s functions.php
file. It’s possibly more practical to create a separate shortcodes.php
file then include that within functions.php
using the statement:
通常会创建简码来帮助自定义插件的使用,因此您应该将它们放在插件代码本身中。 但是,您也可以在主题的functions.php
文件中放置简码定义。 创建一个单独的shortcodes.php
文件,然后使用以下语句将其包含在functions.php
可能更为实用:
include('shortcodes.php');
您的第一个“ Hello World”简码 (Your First “Hello World” Shortcode)
A shortcode definition consists:
简码定义包括:
- a function which returns a string of HTML code, and 一个返回一串HTML代码的函数,以及
a call to the WordPress
add_shortcode()
hook which binds a the shortcode text definition to that function.对WordPress
add_shortcode()
挂钩的调用,该挂钩将add_shortcode()
文本定义绑定到该函数。
The most basic example:
最基本的例子:
<?php
// "Hello World" shortcode
function shortcode_HelloWorld() {
return '<p>Hello World!</p>';
}
add_shortcode('helloworld', 'shortcode_HelloWorld');
(You can omit the <?php
line if one is already present at the top of your file.)
(如果文件顶部已存在<?php
行,则可以省略<?php
行。)
Save the file then enter [helloworld]
somewhere within a page or post. Visit that page and you’ll see it’s been replaced with a “Hello World!” paragraph.
保存文件,然后在页面或帖子中的某处输入[helloworld]
。 访问该页面,您会看到它已被“ Hello World!”取代。 段。
简码参数 (Shortcode Parameters)
Shortcodes can have optional parameters, e.g.
简码可以具有可选参数,例如
[sitemap title='Web pages', depth=3]
Parameters are passed as an array to the shortcode function as the first argument. The full code to generate a page hierarchy sitemap:
参数作为第一个参数作为数组传递给shortcode函数。 生成页面层次结构站点地图的完整代码:
// sitemap shortcode
function shortcode_GenerateSitemap($params = array()) {
// default parameters
extract(shortcode_atts(array(
'title' => 'Site map',
'id' => 'sitemap',
'depth' => 2
), $params));
// create sitemap
$sitemap = wp_list_pages("title_li=&depth=$depth&sort_column=menu_order&echo=0");
if ($sitemap) {
$sitemap =
($title ? "<h2>$title</h2>" : '') .
'<ul' . ($id? '' : " id="$id"") . ">$sitemap</ul>";
}
return $sitemap;
}
add_shortcode('sitemap', 'shortcode_GenerateSitemap');
The shortcode_atts()
function assigns default values to parameters when required. The PHP extract()
function then converts each array value into the real variables $title
, $id
and $depth
.
shortcode_atts()
函数在需要时将默认值分配给参数。 然后, PHP extract()
函数将每个数组值转换为实数变量$title
, $id
和$depth
。
(Note array(...)
can be replaced with the shorter [...]
syntax if you are using PHP5.4 or above.)
(注array(...)
可以被带有较短的取代[...]
语法,如果使用的是PHP5.4或以上)。
Add a [sitemap]
shortcode to any post or page and optionally change the parameters, e.g. [sitemap depth=5]
.
在任何帖子或页面上添加[sitemap]
简码,并可选地更改参数,例如[sitemap depth=5]
。
嵌套BBCode简码 (Nested BBCode Shortcodes)
BBCode (Bulletin Board Code) is a lightweight markup format which, like standard shortcodes, uses [square brackets] to denote commands. This permits shortcodes to contain text content or be nested within each other.
BBCode (公告板代码)是一种轻巧的标记格式,与标准的短代码一样,使用[方括号]表示命令。 这允许简码包含文本内容或彼此嵌套。
Presume your pages require pull quotes and general purpose call-to-action buttons. It would be impractical to create a single shortcode especially when a button could be used on its own or embedded within a quote. We could require HTML such as:
假设您的页面需要引号和通用号召性用语按钮。 创建单个短代码是不切实际的,尤其是当按钮可以单独使用或嵌入在引号中时。 我们可能需要HTML,例如:
<blockquote>
<p>Everything we do is amazing!</p>
<p><a href="/contact-us/" class="cta button main">Call us today</a></p>
</blockquote>
It would could get this wrong when the editor was intimately familiar with HTML. Fortunately, shortcodes provide an easier route, e.g.
当编辑者非常熟悉HTML时,可能会出错。 幸运的是,短代码提供了一条更简单的途径,例如
[quote]
Everything we do is amazing!
[cta type='main']Call us today[/cta]
[/quote]
The content between the tags is passed to the shortcode function as the second argument. We can create two shortcodes functions:
标签之间的内容作为第二个参数传递给shortcode函数。 我们可以创建两个简码函数:
// [quote] shortcode
function shortcode_Quote($params = array(), $content) {
// default parameters
extract(shortcode_atts(array(
'type' => ''
), $params));
// create quote
return
'<blockquote' .
($type ? " class=\"$type\"" : '') .
'>' .
do_shortcode($content) .
'</blockquote>';
}
add_shortcode('quote', 'shortcode_Quote');
// [cta] shortcode
function shortcode_CallToAction($params = array(), $content) {
// default parameters
extract(shortcode_atts(array(
'href' => '/contact-us/',
'type' => ''
), $params));
// create link in button style
return
'<a class="cta button' .
($type ? " $type" : '') .
'">' .
do_shortcode($content) .
'</a>';
}
add_shortcode('cta', 'shortcode_CallToAction');
Note the use of do_shortcode($content)
function which applies further shortcodes to the content when they exist.
请注意do_shortcode($content)
函数的使用, do_shortcode($content)
函数将进一步的短代码应用于内容(如果存在)。
Shortcodes are easy to implement and can be changed or enhanced quickly. I recommend creating a shortcode cheatsheet with examples so editors have a reference when complex functionality is required.
简码易于实现,可以快速更改或增强。 我建议使用示例创建简短代码备忘单,以便在需要复杂功能时供编辑者参考。
If you’re looking for somewhere to host your WordPress site after you’ve got your shortcodes all figured out, take a look at our partner, SiteGround. They offer managed WordPress hosting, with one-click installation, staging environments, a WP-CLI interface, pre-installed Git, autoupdates, and more!
如果所有短代码都弄清楚了,如果您正在寻找一个托管WordPress网站的地方,请查看我们的合作伙伴SiteGround 。 他们提供托管的WordPress托管,一键安装,暂存环境,WP-CLI界面,预安装的Git,自动更新等!
翻译自: https://www.sitepoint.com/create-custom-wordpress-shortcodes/
城市简码