ajax 插件
Using Ajax in your WordPress website is easier than you think. With jQuery on your side, you can submit data, as well as receive data. Communicating with WordPress is performed through a special Ajax dedicated WordPress action that is capable of handling the server side aspect of it.
在您的WordPress网站中使用Ajax比您想象的要容易。 借助jQuery,您可以提交数据,也可以接收数据。 与WordPress的通信是通过一种特殊的Ajax专用WordPress操作执行的,该操作能够处理它的服务器端方面。
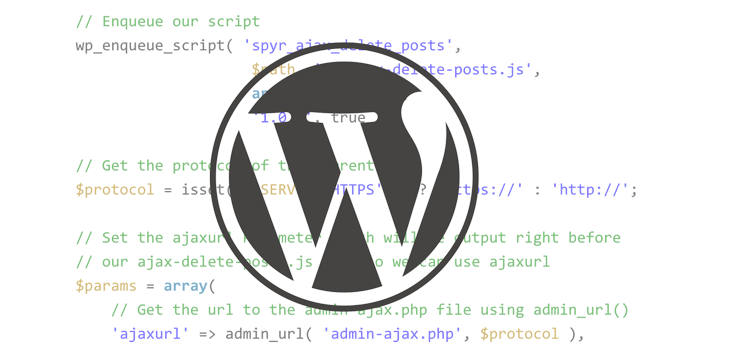
Ajax occurs usually after a form submission, or by pressing a button, enabling you to send bits of data to the server in order to be processed. As an example, please consider the following snippet of code:
Ajax通常在提交表单后或通过按下按钮来发生,使您能够将数据发送到服务器以进行处理。 例如,请考虑以下代码片段:
var data {
action: 'spyr_plugin_do_ajax_request',
var1: 'value 1',
var2: 'value 2'
}
Using our friend jQuery, we are going to POST this to the server and get a server response. In WordPress, all Ajax requests are sent to admin-ajax.php
for processing. This file resides within the /wp-admin
directory of your WordPress site. Even though the file resides in the admin area of your site, you can use it to process interactions from the front-end, as well as the back-end.
使用我们的朋友jQuery,我们将其发布到服务器并获得服务器响应。 在WordPress中,所有Ajax请求都发送到admin-ajax.php
进行处理。 该文件位于WordPress网站的/wp-admin
目录中。 即使该文件位于您网站的管理区域中,您也可以使用它来处理前端和后端的交互。
动作参数 (The Action Parameter)
In our previous example, the action parameter spyr_plugin_do_ajax_request
is responsible for making the connection between the JavaScript file and the PHP code. It’s recommended to prefix all action, including this one; so that it is unique and identifiable. Make it look like it belongs to your WordPress plugin.
在我们之前的示例中,动作参数spyr_plugin_do_ajax_request
负责在JavaScript文件和PHP代码之间建立连接。 建议给所有动作加上前缀,包括这一动作; 因此它是唯一且可识别的。 使它看起来像属于您的WordPress插件。
WordPress专用的Ajax操作 (WordPress’ Dedicated Ajax Actions)
There are two WordPress dedicated Ajax actions that we can use.
我们可以使用两个WordPress专用的Ajax操作。
The first is wp_ajax_$action
which handles the Ajax request if the user is logged in.
第一个是wp_ajax_$action
,如果用户已登录,它将处理Ajax请求。
The second is wp_ajax_nopriv_$action
which handles the Ajax request if the user is not logged in and has no privileges at all.
第二个是wp_ajax_nopriv_$action
,如果用户未登录并且根本没有特权,它将处理Ajax请求。
Following our previous examples, we would add these action hooks to our plugin like so:
在前面的示例之后,我们将这些动作钩子添加到插件中,如下所示:
add_action( 'wp_ajax_spyr_plugin_do_ajax_request', 'spyr_plugin_do_ajax_request' );
add_action( 'wp_ajax_nopriv_spyr_plugin_do_ajax_request', 'spyr_plugin_do_ajax_request' );
Now that we know the basics of how an Ajax event interacts with WordPress, let’s see a real world example. Let’s build a simple plugin which will allow administrators to delete a certain post from the front-end.
现在,我们了解了Ajax事件如何与WordPress交互的基础知识,让我们来看一个真实的示例。 让我们构建一个简单的插件,该插件将允许管理员从前端删除某些帖子。
Ajax Post Delete插件 (Ajax Post Delete Plugin)
We can now start building a sample plugin, which will delete Posts via Ajax. Since this article is meant to introduce you to the inner-workings of processing an Ajax request, I feel this plugin will allow you to get your hands dirty without adding any type of confusion. Our plugin will consist of:
现在,我们可以开始构建一个示例插件,该插件将通过Ajax删除帖子。 由于本文旨在向您介绍处理Ajax请求的内部工作原理,因此我认为此插件将使您不费吹灰之力,而不会增加任何混淆。 我们的插件将包括:
- Adding the JavaScript file with the Ajax script 使用Ajax脚本添加JavaScript文件
- Adding a link to every post that when clicked, it will delete the post 为每个帖子添加链接,单击该链接将删除该帖子
- Handling the Ajax request with PHP 用PHP处理Ajax请求
- Handling the button click with jQuery 使用jQuery处理按钮单击
- Enqueuing our script 排队我们的脚本
// Add script on single post only, if user has Admin rights
add_action( 'template_redirect', 'spyr_add_js_single_posts' );
function spyr_add_js_single_posts() {
// We only want to add the script if is_single()
// and can manage options (Admins)
if( is_single() && current_user_can( 'manage_options' ) ) {
// Get the Path to this plugin's folder
$path = plugin_dir_url( __FILE__ );
// Enqueue our script
wp_enqueue_script( 'spyr_ajax_delete_posts',
$path. 'js/ajax-delete-posts.js',
array( 'jquery' ),
'1.0.0', true );
// Get the protocol of the current page
$protocol = isset( $_SERVER['HTTPS'] ) ? 'https://' : 'http://';
// Set the ajaxurl Parameter which will be output right before
// our ajax-delete-posts.js file so we can use ajaxurl
$params = array(
// Get the url to the admin-ajax.php file using admin_url()
'ajaxurl' => admin_url( 'admin-ajax.php', $protocol ),
);
// Print the script to our page
wp_localize_script( 'spyr_ajax_delete_posts', 'spyr_params', $params );
}
}
Security is always important. So, we need to check whether the current user has sufficient permissions to see the ‘Delete’ link or not. We also need to ensure that we are viewing a single post, otherwise there is no point adding the ‘Delete’ link.
安全始终很重要。 因此,我们需要检查当前用户是否具有足够的权限来查看“删除”链接。 我们还需要确保我们正在查看单个帖子,否则没有必要添加“删除”链接。
If both conditions are true, proceed enqueuing the script that contains the JavaScript code to handle what happens when the link is clicked. Pay close attention to how the protocol is being used in the front-end of the website. We must do this because of the same origin policy that Ajax requests and responses are subjected to. All Ajax requests and responses have to be processed from the same domain; and the protocols must match too.
如果两个条件都成立,则使包含JavaScript代码的脚本排队,以处理单击链接时发生的情况。 请密切注意该协议在网站前端的使用方式。 我们必须这样做是因为与Ajax请求和响应所遵循的起源策略相同。 所有Ajax请求和响应都必须在同一个域中进行处理; 并且协议也必须匹配。
In WordPress, it is possible to force the https protocol in the admin section of your site by setting ‘FORCESSLADMIN’ to ‘true’ in your WordPress configuration file. Sending a request from http://example.com/sample-post/
to https://example.com/wp-admin/admin-ajax.php
won’t work. Even if we are forcing https://
on the back-end, we can make the call to admin-ajax.php
without https://
.
在WordPress中,可以通过在WordPress配置文件中将“ FORCESSLADMIN”设置为“ true”来在站点的admin部分中强制使用https协议。 从http://example.com/sample-post/
向https://example.com/wp-admin/admin-ajax.php
发送请求将不起作用。 即使我们在后端强制使用https://
,也可以在不使用https://
情况下调用admin-ajax.php
。
Finally, the last meaningful line in the code outputs our dynamic variables for us to use in ajax-delete-posts.js
.
最后,代码中最后一行有意义的行输出我们的动态变量,供我们在ajax-delete-posts.js
。
<script type='text/javascript'>
/* <![CDATA[ */
var spyr_params = {"ajaxurl":"http:\/\/example.com\/wp-admin\/admin-ajax.php"};
/* ]]> */
</script>
添加删除链接到内容 (Add Delete Link to Content)
// Add an admin link to each post
add_filter( 'the_content', 'spyr_add_content_delete_link' );
function spyr_add_content_delete_link( $content ) {
if( is_single() && current_user_can( 'manage_options' ) ) {
// Get current post
global $post;
// Create a nonce for this action
$nonce = wp_create_nonce( 'spyr_adp-delete-' . $post->ID );
// Get link to adminn page to trash the post and add nonces to it
$link = '<a href="'. admin_url( 'post.php?post='. $post->ID .'&action=trash&nonce=' .$nonce ) .'"
class="spyr_adp_link" data-nonce="'. $nonce .'" data-id="'. $post->ID .'">Delete Post</a>';
// Append link to content
return $content . '<p>'. $link .'</p>';
}
}
Short and simple. Check for permissions again, and make sure you are viewing a single post. These security measures are necessary and it’s something you are going to have to get used to. Next, create your nonce and tie it to an action; note the the special format. It uses the following structure, prefix_plugin-action-identifier
, where spyr
is the prefix, adp = Ajax Delete Post, delete is our action, and the post ID is the identifier. This is not necessary but it’s recommended when writing plugins. Note that we are also adding the Post’s ID and nonce value to the link using HTML5 data attributes. The last step is to attach your formatted link to the content.
简短而简单。 再次检查权限,并确保您正在查看单个帖子。 这些安全措施是必需的,这是您必须习惯的。 接下来,创建您的随机数并将其与动作关联; 请注意特殊格式。 它使用以下结构prefix_plugin-action-identifier
,其中spyr
是前缀,adp = Ajax Delete Post,delete是我们的操作,post ID是标识符。 这不是必需的,但是在编写插件时建议这样做。 请注意,我们还将使用HTML5数据属性将帖子的ID和随机数添加到链接中。 最后一步是将格式化的链接附加到内容。
使用PHP和WordPress处理Ajax请求 (Handling the Ajax Request with PHP and WordPress)
We are now half way through the article. What follows is the code that actually handles the submission of your Ajax Request. Pay close attention since it relies on how you name the data submitted by the jQuery request to get the post ID (pid) and nonce value.
我们现在到文章的一半了。 以下是实际处理提交Ajax请求的代码。 请密切注意,因为它取决于您如何命名jQuery请求提交的数据以获取帖子ID(pid)和现时值。
// Ajax Handler
add_action( 'wp_ajax_spyr_adp_ajax_delete_post', 'spyr_adp_ajax_delete_post' );
function spyr_adp_ajax_delete_post() {
// Get the Post ID from the URL
$pid = $_REQUEST['pid'];
// Instantiate WP_Ajax_Response
$response = new WP_Ajax_Response;
// Proceed, again we are checking for permissions
if( current_user_can( 'manage_options' ) &&
// Verify Nonces
wp_verify_nonce( $_REQUEST['nonce'], 'spyr_adp-delete-' . $pid ) &&
// Delete the post
wp_delete_post( $pid ) ) {
// Build the response if successful
$response->add( array(
'data' => 'success',
'supplemental' => array(
'pid' => $pid,
'message' => 'This post has been deleted',
),
) );
} else {
// Build the response if an error occurred
$response->add( array(
'data' => 'error',
'supplemental' => array(
'pid' => $pid,
'message' => 'Error deleting this post ('. $pid .')',
),
) );
}
// Whatever the outcome, send the Response back
$response->send();
// Always exit when doing Ajax
exit();
}
spyr_adp_ajax_delete_post
is the name of the action being passed on from the request of your JavaScript file. With this in mind, the full name for your action would be wp_ajax_spyr_adp_ajax_delete_post
. The Post ID and nonce values are also passed on via the JavaScript file, and we can get them from $_REQUEST
.
spyr_adp_ajax_delete_post
是从JavaScript文件的请求传递过来的操作的名称。 考虑到这一点,您的操作的全名将为wp_ajax_spyr_adp_ajax_delete_post
。 Post ID和nonce值也通过JavaScript文件传递,我们可以从$_REQUEST
获得它们。
We are going to be instantiating WP_Ajax_Response
which will allow us to return a nice XML response. You can then read and act upon what’s returned via jQuery. Once again, you need to verify that the user is an Administrator, and try to match nonce values before deleting the post. You need to build different Responses for each scenario so that you know for sure if the Post was deleted or not. You can then attach a brief and concise message describing the result so that you can provide extra feedback to the user. Finally, you send the Response back to the browser and exit. Always remember to exit when you are doing Ajax Requests.
我们将实例化WP_Ajax_Response
,它将允许我们返回一个不错的XML响应。 然后,您可以阅读并执行通过jQuery返回的内容。 再一次,您需要确认用户是管理员,并在删除帖子之前尝试匹配现时值。 您需要为每种情况构建不同的响应,以便确定是否删除了该帖子。 然后,您可以附上简短简洁的消息,描述结果,以便向用户提供额外的反馈。 最后,您将响应发送回浏览器并退出。 始终记得在执行Ajax请求时退出。
The Request, as it will be sent to the server for processing, is as simple as this:
发送到服务器进行处理的请求非常简单:
action=spyr_adp_ajax_delete_post&pid=403&nonce=878727e18a
action=spyr_adp_ajax_delete_post&pid=403&nonce=878727e18a
An example of the formatted XML file returned in the Response can be explored below:
可以在下面的示例中找到响应中返回的格式化XML文件的示例:
<wp_ajax>
<response action="spyr_adp_ajax_delete_post_0">
<object id="0" position="1">
<response_data>success</response_data>
<supplemental>
<pid>403</pid>
<message>This post has been deleted</message>
</supplemental>
</object>
</response>
</wp_ajax>
使用jQuery处理按钮单击 (Handling the Button Click with jQuery)
The only thing left to do now is write the JavaScript code, which will send the request and parse the response. The code itself is pretty self explanatory but I’ve gone ahead and added inline comments to make it easier to understand.
现在剩下要做的就是编写JavaScript代码,它将发送请求并解析响应。 代码本身是很容易解释的,但我已经继续进行并添加了内联注释,以使其更易于理解。
jQuery( document ).ready( function() {
jQuery( '.spyr_adp_link' ).click( function( e ) {
var link = this;
var id = jQuery( link ).attr( 'data-id' );
var nonce = jQuery( link ).attr( 'data-nonce' );
// This is what we are sending the server
var data = {
action: 'spyr_adp_ajax_delete_post',
pid: id,
nonce: nonce
}
// Change the anchor text of the link
// To provide the user some immediate feedback
jQuery( link ).text( 'Trying to delete post' );
// Post to the server
jQuery.post( spyr_params.ajaxurl, data, function( data ) {
// Parse the XML response with jQuery
// Get the Status
var status = jQuery( data ).find( 'response_data' ).text();
// Get the Message
var message = jQuery( data ).find( 'supplemental message' ).text();
// If we are successful, add the success message and remove the link
if( status == 'success' ) {
jQuery( link ).parent().after( '<p><strong>' + message + '</strong></p>').remove();
} else {
// An error occurred, alert an error message
alert( message );
}
});
// Prevent the default behavior for the link
e.preventDefault();
});
});
Parsing the XML with jQuery is straightforward using jQuery.find();
look through the XML for an element as if you were trying to find an element in an HTML document.
使用jQuery.find();
可以很jQuery.find();
用jQuery解析XML jQuery.find();
在XML中查找元素,就好像您试图在HTML文档中查找元素一样。
结论 (Conclusion)
As you can see, performing Ajax requests in your plugins and themes is a very straightforward process in WordPress. This is notably easier to achieve, thanks to the two dedicated actions: wp_ajax_$action
and wp_ajax_nopriv_$action
. This is just the beginning of a path of awesome code writing that will save page re-loads and add interactivity to your WordPress theme or plugin. What will you do next?
如您所见,在WordPress和插件中执行Ajax请求是一个非常简单的过程。 这要归功于两个专用操作: wp_ajax_$action
和wp_ajax_nopriv_$action
,它更容易实现。 这只是很棒的代码编写路径的开始,它将节省页面重新加载并为您的WordPress主题或插件增加交互性。 接下来您要做什么?
If you’re looking for more information on this topic, make sure you check out the WordPress Codex page on Ajax and Ajax in Plugins.
如果您正在寻找有关此主题的更多信息,请确保检查出Ajax和插件中的Ajax 上的WordPress Codex页面 。
翻译自: https://www.sitepoint.com/adding-ajax-to-your-wordpress-plugin/
ajax 插件