wordpress插件
Introduction
介绍
WordPress, as a platform, has moved from only being a blogging platform, to a platform for a wide variety of websites. One major reason for this, is how easily WordPress can be customized and extended. This enables programmers to create far more functional sites than a normal blog. In the following tutorial we are going to create a plugin which will make wordpress an article voting site and also create a widget to display the top voted posts.
WordPress作为一个平台,已经从仅是博客平台转变为适用于各种网站的平台。 主要原因之一是WordPress的自定义和扩展容易程度。 这使程序员可以创建比普通博客更多功能的网站。 在下面的教程中,我们将创建一个插件,该插件将使wordpress成为文章投票网站,并创建一个小部件来显示获得最高投票的帖子。
创建插件 (Creating the Plugin)
To create a plugin create a file voteme.php in your wp-content/plugins/voteme folder. To create a plugin we have to add the plugin header as follows
要创建插件,请在您的wp-content / plugins / voteme文件夹中创建一个表决文件。 要创建插件,我们必须添加插件标头,如下所示
<?php
/*
Plugin Name: Vote Me
Plugin URI:
Description: This plugin to add vote in posts
Author: Abbas
Version: 0.1
Author URI:
*/
We will also define some named constants for our plugin base url, and the plugin path as follows:
我们还将为我们的插件基本URL和插件路径定义一些命名常量,如下所示:
define('VOTEMESURL', WP_PLUGIN_URL."/".dirname( plugin_basename( __FILE__ ) ) );
define('VOTEMEPATH', WP_PLUGIN_DIR."/".dirname( plugin_basename( __FILE__ ) ) );
Also, create a js folder in your voteme folder, and add a file voteme.js file in it.
另外,在您的表决文件夹中创建一个js文件夹,并在其中添加一个文件voteme.js文件。
The folder structure of the plugin would be as follows.
插件的文件夹结构如下。
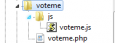
We will now enqueue the scripts by with ‘wp_enqueue_scripts’ and enqueue our JS file, and localize it to store the WP Ajax url which we will use for our ajax calls.
现在,我们将使用'wp_enqueue_scripts'将脚本排入队列,并排入我们的JS文件,并将其本地化以存储WP Ajax URL,该URL将用于我们的Ajax调用。
function voteme_enqueuescripts()
{
wp_enqueue_script('voteme', VOTEMESURL.'/js/voteme.js', array('jquery'));
wp_localize_script( 'voteme', 'votemeajax', array( 'ajaxurl' => admin_url( 'admin-ajax.php' ) ) );
}
add_action('wp_enqueue_scripts', voteme_enqueuescripts);</pre>
After this we should be able to see our plugin in the plugin list and we should activate it.
之后,我们应该能够在插件列表中看到我们的插件,并且应该激活它。
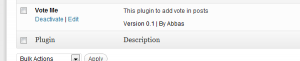
将投票链接添加到帖子 (Adding the vote link to posts)
Now we will add a link to all the posts displaying the current votes and a link to add the vote by ajax. Following is the code
现在,我们将向显示当前投票的所有帖子添加一个链接,并通过ajax添加投票。 以下是代码
function voteme_getvotelink()
{
$votemelink = "";
$post_ID = get_the_ID();
$votemecount = get_post_meta($post_ID, '_votemecount', true) != '' ? get_post_meta($post_ID, '_votemecount', true) : '0';
$link = $votemecount.' <a onclick="votemeaddvote('.$post_ID.');">'.'Vote'.'</a>';
$votemelink = '<div id="voteme-'.$post_ID.'">';
$votemelink .= '<span>'.$link.'</span>';
$votemelink .= '</div>';
return $votemelink;
}
function voteme_printvotelink($content)
{
return $content.voteme_getvotelink();
}
add_filter('the_content', voteme_printvotelink);
Here, we create a function voteme_getvote
link which first gets the current post id and then reads the post meta _votemecount
using get_post_meta
. We use the post meta _votemecount
to store the votes for a particular post. Then we create a link by adding the JavaScript function votemeaddvote
on the click of the link. The function votemeaddvote
we will see shortly.
在这里,我们创建一个功能voteme_getvote
链接,该链接首先获取当前帖子ID,然后使用get_post_meta
读取帖子元_votemecount
。 我们使用post meta _votemecount
存储特定帖子的投票。 然后,通过单击链接添加JavaScript函数votemeaddvote
来创建链接。 我们很快会看到功能votemeaddvote
。
Next, we use the WordPress filter the_content
to hook our function to add this link after each post. You will be able to see the number of votes and vote link below each post now.
接下来,我们使用WordPress过滤器the_content
来挂钩我们的函数,以便在每个帖子之后添加此链接。 您现在将可以看到每个帖子下方的投票数和投票链接。
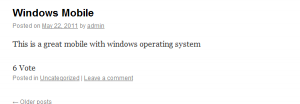
使用Ajax添加投票 (Adding votes using Ajax)
As we have added the vote link to every posts its time we make it functional to actually add votes. The JavaScript function which does the AJAX vote posting is below
当我们将投票链接添加到每个帖子时,我们就可以实际添加投票了。 下面是进行AJAX投票发布JavaScript函数
function votemeaddvote(postId)
{
jQuery.ajax({
type: 'POST',
url: votemeajax.ajaxurl,
data: {
action: 'voteme_addvote',
postid: postId
},
success:function(data, textStatus, XMLHttpRequest){
var linkid = '#voteme-' + postId;
jQuery(linkid).html('');
jQuery(linkid).append(data);
},
error: function(MLHttpRequest, textStatus, errorThrown){
alert(errorThrown);
}
});
}
This sends an AJAX request to WordPress, and sends the action as voteme_addvote
and the post ID. If the AJAX call is successful it just adds the data in the div for that post. If there is an error it will just display the error.
这会将AJAX请求发送到WordPress,并将操作作为voteme_addvote
和帖子ID发送。 如果AJAX调用成功,它只会在该帖子的div中添加数据。 如果有错误,它将仅显示错误。
To handle the AJAX request we will have to create a function as follows
为了处理AJAX请求,我们将必须创建一个如下函数
function voteme_addvote()
{
$results = '';
global $wpdb;
$post_ID = $_POST['postid'];
$votemecount = get_post_meta($post_ID, '_votemecount', true) != '' ? get_post_meta($post_ID, '_votemecount', true) : '0';
$votemecountNew = $votemecount + 1;
update_post_meta($post_ID, '_votemecount', $votemecountNew);
$results.='<div class="votescore" >'.$votemecountNew.'</div>';
// Return the String
die($results);
}
// creating Ajax call for WordPress
add_action( 'wp_ajax_nopriv_voteme_addvote', 'voteme_addvote' );
add_action( 'wp_ajax_voteme_addvote', 'voteme_addvote' );
In function voteme_addvote we get the post ID from the posted data and then get the current vote count for that post. Then we increment the vote by 1 and update the post meta again. Then we create a div with the new vote details and sent that back by using die($results);
在功能voteme_addvote中,我们从发布的数据中获取发布ID,然后获取该发布的当前投票计数。 然后我们将投票增加1并再次更新post meta。 然后,我们使用新的投票详细信息创建一个div,然后使用die($ results)将其发送回去;
To register this function to handle AJAX request for action: ‘voteme_addvote’ using the following wordpress hooks
要注册此功能以处理AJAX动作请求,请使用以下wordpress挂钩:“ voteme_addvote”
// creating Ajax call for WordPress
add_action( 'wp_ajax_nopriv_voteme_addvote', 'voteme_addvote' );
add_action( 'wp_ajax_voteme_addvote', 'voteme_addvote' );</pre>
Now we’ll be able to click the vote link to add the vote on the post with AJAX.
现在,我们将能够单击投票链接以使用AJAX在帖子上添加投票。
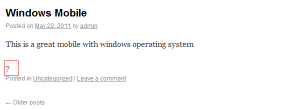
自定义WordPress管理员以显示帖子投票 (Customizing WordPress admin to show post votes)
It would be very convenient for the admin to be able to see the votes on the post edit page. Then from there he would be able to see the post details, and also the vote details on that page.
管理员能够在帖子编辑页面上看到投票将非常方便。 然后,从那里他将可以看到该职位的详细信息,以及该页面上的投票详细信息。
To add the vote details we need to add a hook on the filter ‘manage_edit-post_columns’ to add the Votes as a column on the post edit page as follows.
要添加投票详细信息,我们需要在过滤器“ manage_edit-post_columns”上添加一个挂钩,以将“投票”添加为帖子编辑页面上的一栏,如下所示。
add_filter( 'manage_edit-post_columns', 'voteme_extra_post_columns' );
function voteme_extra_post_columns( $columns ) {
$columns[ 'votemecount' ] = __( 'Votes' );
return $columns;
}
Now we need to provide the value to display for this column. To do this we need to hook into the filter manage_posts_custom_column
. When we hook into this filter our function voteme_post_column_row
is called and with the post column name. Here we only process out column votemecount
and give the value for it. We read the value from our custom column _votemecount
and echo it. The complete code for this is as follows.
现在,我们需要提供此列要显示的值。 为此,我们需要加入过滤器manage_posts_custom_column
。 当我们连接到该过滤器时,将调用函数voteme_post_column_row
并使用发布列名称。 在这里,我们只处理votemecount
列并votemecount
提供值。 我们从自定义列_votemecount
读取值并回显它。 完整的代码如下。
function voteme_post_column_row( $column ) {
if ( $column != 'votemecount' )
return;
global $post;
$post_id = $post->ID;
$votemecount = get_post_meta($post_id, '_votemecount', true) != '' ? get_post_meta($post_id, '_votemecount', true) : '0';
echo $votemecount;
}
add_action( 'manage_posts_custom_column', 'voteme_post_column_row', 10, 2 );
On the post edit page you should be able to see the vote details column.
在帖子编辑页面上,您应该能够看到投票详细信息列。
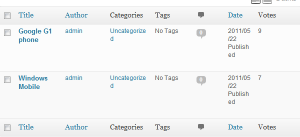
在WordPress管理中对投票基础进行排序 (Sorting post on basics of votes in WordPress admin)
It would be convenient for the admin if we make the vote column sortable. He would be able to see the most voted posts and also the least voted posts. To do this first we must make the Vote column clickable for sorting. To do this we hook into the filter manage_edit-post_sortable_columns
and add the vote column to it as follows.
如果我们将投票栏设为可排序的,对管理员来说将很方便。 他将能够看到投票最多的帖子以及投票最少的帖子。 首先,我们必须使“投票”列可单击以进行排序。 为此,我们将过滤器插入manage_edit-post_sortable_columns
过滤器,并按如下所示向其中添加投票栏。
add_filter( 'manage_edit-post_sortable_columns', 'voteme_post_sortable_columns' );
function voteme_post_sortable_columns( $columns )
{
$columns[ 'votemecount' ] = votemecount;
return $columns;
}
Then we add a hook onto the load-edit.php
hook when we have the order by request for votemecount
we merge the sort parameters with
然后,当我们通过对votemecount
的请求获得订单时,将钩子添加到load-edit.php
钩子上,将排序参数与
'meta_key' => '_votemecount',
'orderby' => 'meta_value_num'
So that it sorts on the basics of custom column and considers that column as numeric rather than as a string. The code for it is as follows.
因此,它基于自定义列的基础进行排序,并将该列视为数字而不是字符串。 它的代码如下。
add_action( 'load-edit.php', 'voteme_post_edit' );
function voteme_post_edit()
{
add_filter( 'request', 'voteme_sort_posts' );
}
function voteme_sort_posts( $vars )
{
if ( isset( $vars['post_type'] ) && 'post' == $vars['post_type'] )
{
if ( isset( $vars['orderby'] ) && 'votemecount' == $vars['orderby'] )
{
$vars = array_merge(
$vars,
array(
'meta_key' => '_votemecount',
'orderby' => 'meta_value_num'
)
);
}
}
return $vars;
}
Now in the admin page the vote column will be clickable and clicking on it will sort the post on the basics of votes.
现在,在管理页面中,“投票”列将是可单击的,单击该列将对投票的基础进行排序。
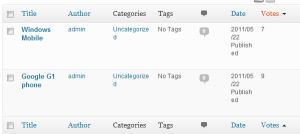
只允许注册用户投票 (Allowing only Registered users to vote)
We might want that not anyone can vote on the post. We might want to check that only users who are registered on our site will be able to vote. We’ll control this via creating a setting page for our plugin as follows
我们可能希望没有人可以对此职位进行投票。 我们可能要检查,只有在我们网站上注册的用户才可以投票。 我们将通过为插件创建一个设置页面来控制它,如下所示
// Settings
add_action('admin_menu', 'voteme_create_menu');
function voteme_create_menu() {
add_submenu_page('options-general.php','Vote Me','Vote Me','manage_options', __FILE__.'voteme_settings_page','voteme_settings_page');
}
function voteme_settings_page() {
?>
<div class="wrap">
<?php
global $blog_id;
if( isset( $_POST['votemeoptionssubmit'] ) )
{
update_option( 'votemelogincompulsory' , $_POST[ 'votemelogincompulsory' ] );
}
?>
<div id="settingsform">
<form id='votemesettingform' method="post" action="">
<h1><?php echo 'Vote Me Settings'; ?></h1>
<Input type = 'Radio' Name ='votemelogincompulsory' value= 'yes' <?php if( get_option('votemelogincompulsory') == 'yes' ) echo 'checked';?> >User Must be logged in for voting
<br/>
<Input type = 'Radio' Name ='votemelogincompulsory' value= 'no' <?php if( get_option('votemelogincompulsory') != 'yes' ) echo 'checked';?> >User might not be logged in for voting
<br/><br/>
<p class="submit">
<input type="submit" id="votemeoptionssubmit" name="votemeoptionssubmit" class="button-primary" value="<?php echo 'Save'; ?>" />
</p>
</form>
</div>
</div>
<?php }
Here we hook onto admin_menu
and create our setting page to show radio buttons for whether to allow voting for registered users only. Then based on the option selected by the admin we update the option votemelogincompulsory
. The settings page will look as follows.
在这里,我们进入admin_menu
并创建我们的设置页面,以显示单选按钮,以决定是否仅允许注册用户投票。 然后根据管理员选择的选项,我们更新votemelogincompulsory
选项。 设置页面如下所示。
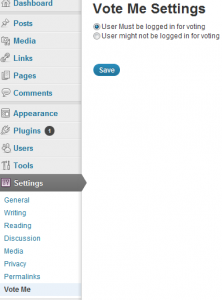
Then, update the voteme_getvotelink
function to read the option votemelogincompulsory
and to show the votelink or the login link depending on the option selected by the user and wthere the user is logged in or no. The code for it is as follows
然后,更新voteme_getvotelink
函数以读取votemelogincompulsory
选项votemelogincompulsory
并根据用户选择的选项显示投票链接或登录链接,无论用户是否登录。 它的代码如下
function voteme_getvotelink()
{
$votemelink = "";
if( get_option('votemelogincompulsory') != 'yes' || is_user_logged_in() )
{
$post_ID = get_the_ID();
$votemecount = get_post_meta($post_ID, '_votemecount', true) != '' ? get_post_meta($post_ID, '_votemecount', true) : '0';
$link = $votemecount.' <a onclick="votemeaddvote('.$post_ID.');">'.'Vote'.'</a>';
$votemelink = '<div id="voteme-'.$post_ID.'">';
$votemelink .= '<span>'.$link.'</span>';
$votemelink .= '</div>';
}
else
{
$register_link = site_url('wp-login.php', 'login') ;
$votemelink = '<div class="votelink" >'." <a href=".$register_link.">"."Vote"."</a>".'</div>';
}
return $votemelink;
}
创建一个小部件以显示投票最多的帖子。 (Creating a widget to display Top voted posts.)
Now we will create a widget to display the top voted posts. First we create a function called voteme_get_highest_voted_posts
which takes the number of posts and then displays those many post in order of the highest voted posts. It also displays the number of vote for each post.
现在,我们将创建一个小部件以显示投票最多的帖子。 首先,我们创建一个名为voteme_get_highest_voted_posts
的函数,该函数获取帖子数,然后按投票数最高的顺序显示很多帖子。 它还显示每个帖子的投票数。
function voteme_get_highest_voted_posts($numberofpost)
{
$output = '';
$the_query = new WP_Query( 'meta_key=_votemecount&orderby=meta_value_num&order=DESC&posts_per_page='.$numberofpost );
// The Loop
while ( $the_query->have_posts() ) : $the_query->the_post();
$output .= '<li>';
$output .= '<a href="'.get_permalink(). '" rel="bookmark">'.get_the_title().'('.get_post_meta(get_the_ID(), '_votemecount', true).')'.'</a> ';
$output .= '</li>';
endwhile;
wp_reset_postdata();
return $output;
}
Then we create a widget which takes the number of post and the title from the user and uses the abpve function to display the top voted posts.
然后,我们创建一个小部件,该小部件将获取用户的帖子数量和标题,并使用abpve函数显示投票最多的帖子。
class VoteMeTopVotedWidget extends WP_Widget {
function VoteMeTopVotedWidget() {
// widget actual processes
$widget_ops = array('classname' => 'VoteMeTopVotedWidget', 'description' => 'Widget for top voted Posts.' );
$this->WP_Widget('VoteMeTopVotedWidget','VoteMeTopVotedWidget', $widget_ops);
}
function form($instance) {
// outputs the options form on admin
$defaults = array( 'title' => 'Top Voted Posts', 'numberofposts' => '5' );
$instance = wp_parse_args( (array) $instance, $defaults );
?>
<p>
<label for="<?php echo $this->get_field_id( 'title' ); ?>"><?php echo 'Title:'; ?></label>
<input id="<?php echo $this->get_field_id( 'title' ); ?>" name="<?php echo $this->get_field_name( 'title' ); ?>" value="<?php echo $instance['title']; ?>" class="widefat" />
</p>
<p>
<label for="<?php echo $this->get_field_id( 'numberofposts' ); ?>"><?php echo 'Number of Posts'; ?></label>
<input id="<?php echo $this->get_field_id( 'numberofposts' ); ?>" name="<?php echo $this->get_field_name( 'numberofposts' ); ?>" value="<?php echo $instance['numberofposts']; ?>" class="widefat" />
</p>
<?php
}
function update($new_instance, $old_instance) {
// processes widget options to be saved
$instance = $old_instance;
$instance['title'] = strip_tags( $new_instance['title'] );
$instance['numberofposts'] = $new_instance['numberofposts'];
return $instance;
}
function widget($args, $instance) {
// outputs the content of the widget
extract( $args );
$title = apply_filters('widget_title', $instance['title'] );
echo $before_widget;
if ( $title )
echo $before_title . $title . $after_title;
echo '<ul>';
echo voteme_get_highest_voted_posts($instance['numberofposts']);
echo '</ul>';
echo $after_widget;
}
}
function voteme_widget_init() {
// Check for the required API functions
if ( !function_exists('register_widget') )
return;
register_widget('VoteMeTopVotedWidget');
}
add_action('widgets_init', 'voteme_widget_init');</pre>
The widget will look as follows
小部件如下所示
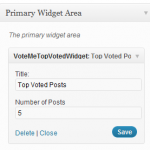
On the front, the widget will look as follows
在前面,小部件将如下所示
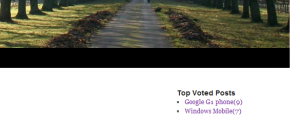
结论。 (Conclusion.)
With custom fields, WordPress makes it easy for us to extend it to use for different purposes. WordPress has good support for AJAX as we have seen in this tutorial. So, happy WordPress development!
通过自定义字段,WordPress使我们可以轻松地将其扩展以用于不同目的。 正如我们在本教程中看到的那样,WordPress对AJAX具有良好的支持。 因此,WordPress开发愉快!
翻译自: https://www.sitepoint.com/create-a-voting-plugin-for-wordpress/
wordpress插件