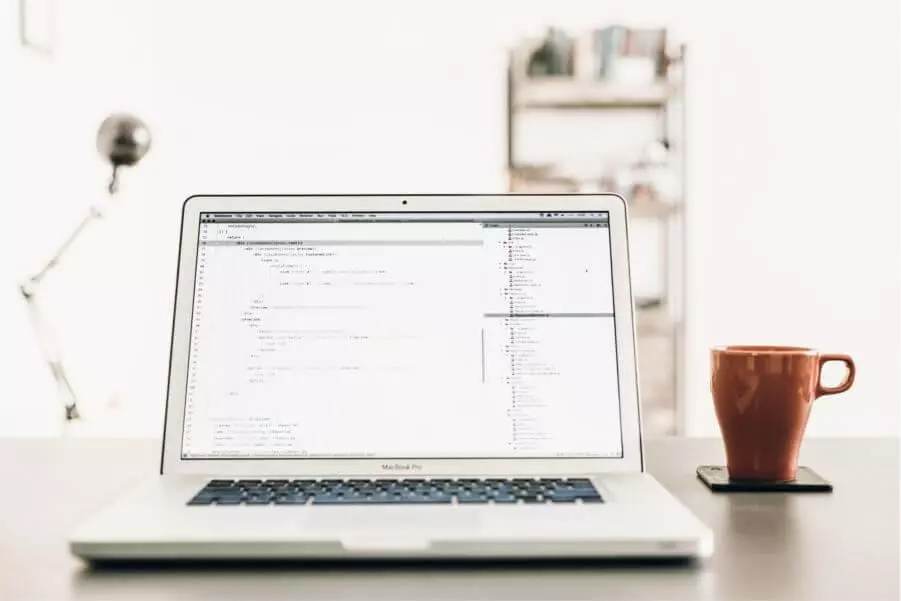
This article is part of a series created in partnership with SiteGround. Thank you for supporting the partners who make SitePoint possible.
本文是与SiteGround合作创建的系列文章的一部分。 感谢您支持使SitePoint成为可能的合作伙伴。
If your WordPress website needs a particular stylesheet or JavaScript file only in one specific page or just under a limited number of conditions, there is no need to load those files everywhere on your website.
如果您的WordPress网站仅在一个特定页面中或仅在有限的条件下需要特定的样式表或JavaScript文件,则无需将这些文件加载到网站上的任何位置。
In this article, you are going to learn how to load CSS styles and scripts only when your website needs them. This way your website will load faster and users who don’t visit the pages with the extra files won’t have to download unnecessary bytes in their browsers.
在本文中,您将学习如何仅在网站需要CSS样式和脚本时加载它们。 这样,您的网站将加载得更快,并且不访问带有多余文件的页面的用户将不必在浏览器中下载不必要的字节。
为什么您应该以WordPress方式添加样式和脚本 (Why You Should Add Styles and Scripts the WordPress Way)
If there is an anti-pattern in WordPress development, it’s adding a <link>
and <script>
tag to load stylesheets and JavaScript files respectively in the <head>
section of the HTML document and calling it a day.
如果WordPress开发中存在反模式,它会添加<link>
和<script>
标记以分别在HTML文档的<head>
部分中加载样式表和JavaScript文件,并将其命名为day。
Running a WordPress website involves using stylesheets and JavaScript code that comes from the software itself, a number of plugins, and the active theme.
运行WordPress网站需要使用样式表和来自软件本身,大量插件和活动主题JavaScript代码。
This means that theme or plugin authors don’t know in advance which styles and scripts a specific installation is going to run, or in which order those resources are needed. For instance, let’s take the jQuery library. This library can be used by WordPress itself to handle Ajax requests and other tasks, by more than one plugin, and by the active theme.
这意味着主题或插件的作者事先不知道特定安装将要运行哪种样式和脚本,或者这些资源需要按什么顺序运行。 例如,让我们来看看jQuery库。 WordPress本身可以使用该库来处理Ajax请求和其他任务,可以使用多个插件,也可以使用活动主题。
If plugin and theme authors include the stylesheets and scripts they need by adding the appropriate tags directly inside the HTML document, this could open the doors to the possibility of conflicts, of resources being loaded multiple times and/or in the wrong order.
如果插件和主题作者通过在HTML文档中直接添加适当的标签来包含所需的样式表和脚本,则可能会引发冲突,多次加载资源和/或加载顺序错误的可能性。
This is why you should always load styles and scripts following best practices as recommended by WordPress.org coding standards:
这就是为什么您应该始终按照WordPress.org编码标准建议的最佳做法加载样式和脚本的原因:
So that everything works harmoniously, it’s important that theme and plugins load scripts and stylesheets using the standard WordPress method. This will ensure the site remains efficient and that there are no incompatibility issues.
为了使所有内容和谐地工作,使用标准WordPress方法加载主题和插件很重要。 这将确保站点保持高效,并且不存在不兼容问题。
Including CSS & JavaScript — Theme Handbook
如何使用wp_enqueue_style和wp_enqueue_script (How to Use wp_enqueue_style and wp_enqueue_script)
The barebones function to include your custom stylesheet into a theme looks like this:
将自定义样式表包含到主题中的准系统功能如下所示:
wp_enqueue_style( $handle, $src, $deps, $ver, $media );
Only the first two parameters are obligatory, the others are optional.
仅前两个参数为必填项,其他为可选参数。
The
$handle
parameter is the name of your stylesheet. You can call itmain
,portfolio
, etc., depending on what the file is for. If you’re including stylesheets from a JavaScript plugin, just use the plugin’s name$handle
参数是样式表的名称。 您可以将其称为main
,portfolio
等,具体取决于文件的用途。 如果要包含来自JavaScript插件的样式表,只需使用插件的名称$src
stands for the URL where the stylesheet is located$src
代表样式表所在的URLThe
$deps
parameter specifies if this stylesheet depends on another stylesheet to work correctly$deps
参数指定此样式表是否依赖于另一个样式表才能正常工作$ver
determines the stylesheet’s version number$ver
确定样式表的版本号$media
stands for the media type the stylesheet is for, e.g., all, screen, print, etc.$media
代表样式表用于的媒体类型,例如,所有,屏幕,印刷等。
As an example, the code to add a stylesheet named portfolio.css
to your WordPress website could look like this:
例如,向您的WordPress网站添加名为portfolio.css
的样式表的代码如下所示:
wp_enqueue_style( 'portfolio', get_template_directory_uri() . '/css/portfolio.css',false,null,'screen');
To include a JavaScript file, you should use:
要包含JavaScript文件,您应该使用:
wp_enqueue_script($handle, $src, $deps, $ver, $in_footer)
The parameters are similar to those in wp_enqueue_style
, except for the last one, which sets a true or false value according to whether you want to place the <script>
tag in the <footer>
or <header>
section of your document.
参数与wp_enqueue_style
的参数相似,除了最后一个参数外,该参数根据要在文档的<footer>
或<header>
部分中放置<script>
标记来设置true或false值。
Let’s say you’d like to add portfolio.js
to your website, here’s what the code would look like:
假设您想将portfolio.js
添加到您的网站中,代码如下所示:
wp_enqueue_script( 'portfolio', get_template_directory_uri() . '/js/portfolio.js', array ( 'jquery' ), null, true);
This code tells WordPress that:
此代码告诉WordPress:
You want to use the
portfolio
file, which is located in thejs
subfolder inside the active theme’s directory您要使用
portfolio
文件,该文件位于活动主题目录下的js
子文件夹中WordPress needs to download jQuery before loading
portfolio.js
otherwise the latter won’t work correctly (you don’t need to import jQuery because it already comes bundled with WordPress by default)WordPress的需要下载的jQuery加载之前
portfolio.js
否则后者将无法正常工作(你不需要导入jQuery的,因为它已经自带的WordPress捆绑默认)WordPress needs to add this file in the
<footer>
section of the HTML document.WordPress需要将此文件添加到HTML文档的
<footer>
部分中。
It’s likely you want to include more than one stylesheet or script file, in this case wrap all the calls to wp_enqueue_style()
and wp_enqueue_script()
inside a function and then hook this function to the appropriate WordPress action hook.
您可能想包含多个样式表或脚本文件,在这种情况下, wp_enqueue_style()
和wp_enqueue_script()
所有调用包装在一个函数中,然后将此函数挂接到相应的WordPress action hook上 。
Here’s an example. If you’re developing a WordPress theme, you can add this to your functions.php
file.
这是一个例子。 如果您正在开发WordPress主题,则可以将其添加到functions.php
文件中。
function mytheme_styles_scripts() {
//main stylesheet, style.css
wp_enqueue_style( 'style', get_stylesheet_uri() );
//stylesheet for portfolio section
//(different from the rest of the site)
wp_enqueue_style( 'portfolio', get_template_directory_uri() . '/css/portfolio.css',false,null,'screen');
//JS for portfolio section
wp_enqueue_script( 'portfolio', get_template_directory_uri() . '/js/portfolio.js', array ( 'jquery' ), null, true);
}
//hook the function to wp_enqueue_scripts action hook
add_action( 'wp_enqueue_scripts', 'mytheme_styles_scripts' );
Now you can rest assured that WordPress will find all the required stylesheets and scripts and download them in the correct order.
现在您可以放心,WordPress将找到所有必需的样式表和脚本,并以正确的顺序下载它们。
有条件地加载样式表和脚本 (Conditional Loading of Stylesheets and Scripts)
The code above works great and follows WordPress best practices. However, WordPress will download portfolio.css
and portfolio.js
everywhere on your website. If your visitors never go to your porfolio page, which is unfortunate but can happen, the browser would have loaded two unnecessary files.
上面的代码效果很好,并遵循WordPress最佳实践。 但是,WordPress会在您网站上的任何地方下载portfolio.css
和portfolio.js
。 如果您的访问者从未访问过您的porfolio页面,这是不幸的,但是这种情况可能发生,那么浏览器将加载两个不必要的文件。
Now, let’s take it up a notch and make sure WordPress includes portfolio.css
and portfolio.js
only when visitors land on your portfolio section.
现在,让我们提高一个档次,确保仅当访问者登陆您的投资组合部分时,WordPress才包含portfolio.css
和portfolio.js
。
WordPress条件标签 (WordPress Conditional Tags)
WordPress offers conditional tags, which in combination with regular if...else
statements, make it very easy to choose what code to apply under certain conditions.
WordPress提供了条件标签 ,该标签与常规if...else
语句结合使用,可以很容易地选择在某些条件下要应用的代码。
For example, if you want to execute some code only on the home page of your website and another bit of code everywhere else, then you’ll write something like this:
例如,如果您只想在网站首页上执行某些代码,而在其他地方执行其他代码,那么您将编写如下代码:
if ( is_front_page() ):
//your code here
else:
//alternative code here
endif;
Conversely, if you want to execute some code everywhere except on the home page of your website, you’ll write:
相反,如果您想在网站首页以外的任何地方执行一些代码,则将编写:
if ( !is_front_page() ):
//your code here;
endif;
WordPress offers tons of conditional tags, you can check out a complete list on the Conditional Tags chapter of the Theme Handbook.
WordPress提供了大量的条件标签,您可以在主题手册的“ 条件标签”一章中查看完整列表。
There’s a way you can leverage conditional tags to ensure WordPress downloads both porfolio.css
and portfolio.js
only if visitors access the portfolio page of your website.
有一种方法可以利用的条件标记,以确保WordPress的下载都porfolio.css
和portfolio.js
只有当访问者访问你的网站的投资组合页面。
Let’s examine some of the ways you can do just that.
让我们研究一些可以做到这一点的方法。
使用页面ID (Using the Page ID)
You can use conditional tags to check if users are on the portfolio page of your website using the portfolio page ID.
您可以使用投资组合页面ID使用条件标签来检查用户是否在您网站的投资组合页面上。
To know the page ID do the following:
要了解页面ID,请执行以下操作:
- Login to your WordPress website’s Admin panel 登录到WordPress网站的管理面板
Click on Pages to access the list of all the pages on your website
单击页面以访问您网站上所有页面的列表
Click on Pages to access the list of all the pages on your website
单击页面以访问您网站上所有页面的列表
Click on Pages to access the list of all the pages on your website
单击页面以访问您网站上所有页面的列表
Click on the title of the page you’re interested in to open it in the editor
单击您感兴趣的页面的标题以在编辑器中将其打开
Click on the title of the page you’re interested in to open it in the editor
单击您感兴趣的页面的标题以在编辑器中将其打开
Now that the page is open in the editor, look at the URL in your browser’s address bar. You should see something like
post=
plus a number. That number is the page ID:现在,页面已在编辑器中打开,然后在浏览器的地址栏中查看URL。 您应该看到类似于
post=
的数字。 该数字是页面ID:Now that the page is open in the editor, look at the URL in your browser’s address bar. You should see something like
post=
plus a number. That number is the page ID:现在,页面已在编辑器中打开,然后在浏览器的地址栏中查看URL。 您应该看到类似于
post=
的数字。 该数字是页面ID:Now that the page is open in the editor, look at the URL in your browser’s address bar. You should see something like
post=
plus a number. That number is the page ID:现在,页面已在编辑器中打开,然后在浏览器的地址栏中查看URL。 您应该看到类似于
post=
的数字。 该数字是页面ID:
Now that you know your page ID, you can modify the previous code that included your theme’s stylesheets and scripts with the use of conditional tags as follows:
现在您已经知道了页面ID,可以使用条件标签修改包含主题主题的样式表和脚本的先前代码,如下所示:
function mytheme_styles_scripts() {
//main stylesheet, style.css
wp_enqueue_style( 'style', get_stylesheet_uri() );
//check we are on page with ID 1
if ( is_page('1') ):
//if we are, load styles and script
//for portfolio page
wp_enqueue_style( 'portfolio', get_template_directory_uri() . '/css/portfolio.css',false,null,'screen');
//JS for portfolio section
wp_enqueue_script( 'portfolio', get_template_directory_uri() . '/js/portfolio.js', array ( 'jquery' ), null, true);
endif;
}
//hook the function to wp_enqueue_scripts action hook
add_action( 'wp_enqueue_scripts', 'mytheme_styles_scripts' );
使用页面标题 (Using the Page Title)
Conditional tags for pages work with the page title too. If your portfolio page has a title of My Portfolio, the code could look like this (I just add the conditional part for brevity’s sake):
页面的条件标签也可以与页面标题一起使用。 如果您的投资组合页面的标题为我的投资组合 ,则代码可能如下所示(为简便起见,我仅添加条件部分):
if ( is_page('My Portfolio') ):
//rest of the code here
endif;
使用页面弹头 (Using the Page Slug)
You can use conditional tags with the page slug, which is the user friendly URL for that page. Here’s what it would look like in practice:
您可以在页面slug中使用条件标签,slug是该页面的用户友好URL。 这是实际的样子:
if ( is_page('my-portfolio') ):
//rest of the code here
endif;
结论 (Conclusion)
In this article I discussed how you can include stylesheets and script files conditionally in your WordPress website. There are circumstances when the extra styles are so few in number that it doesn’t make much sense to have a dedicated stylesheet for them. This would only generate one more HTTP request your website could perhaps do without.
在本文中,我讨论了如何在WordPress网站中有条件地包括样式表和脚本文件。 在某些情况下,多余的样式数量很少,以至于没有专用的样式表是没有意义的。 这只会再产生一个HTTP请求,您的网站可能无法做到。
However, under appropriate conditions, e.g., a page or topic area with a different design from the rest of the website, selective loading of stylesheets and scripts follows WordPress best practices and ensures your visitors’ browsers only download the amount of data needed to display the requested content.
但是,在适当的条件下(例如,页面或主题区域的设计与网站的其余部分不同),选择性加载样式表和脚本将遵循WordPress的最佳做法,并确保访问者的浏览器仅下载显示内容所需的数据量。要求的内容。
How do you include custom scripts and styles into your WordPress website? Hit the comment box below to share.
您如何在WordPress网站中包含自定义脚本和样式? 点击下面的评论框分享。
翻译自: https://www.sitepoint.com/conditional-tags-load-styles-and-scripts-in-wordpress/