python图像处理库
In my previous article on time-saving tips for Pythonists, I mentioned that Python is a language that can inspire love in its users.
在我以前关于Python 节省时间技巧的文章中 ,我提到Python是一种可以激发用户爱的语言。
One reason for this is the number of time-saving libraries available for this language. A nice example is the Python Imaging Library (PIL), which is the focus of this article.
造成这种情况的原因之一是可以使用这种语言的省时的库数量很多。 一个很好的例子是Python Imaging Library(PIL) ,这是本文的重点。
您可以使用PIL做什么 (What You Can Do with PIL)
PIL is a free library that adds image processing capabilities to your Python interpreter, supporting a range of image file formats such as PPM, PNG, JPEG, GIF, TIFF and BMP.
PIL是一个免费的库,它为您的Python解释器添加了图像处理功能,支持多种图像文件格式,例如PPM,PNG,JPEG,GIF,TIFF和BMP。
PIL offers several standard procedures for image processing/manipulation, such as:
PIL提供了几种用于图像处理/操纵的标准程序,例如:
- pixel-based manipulations 基于像素的操作
- masking and transparency handling 遮罩和透明处理
- filtering (for example, blurring, contouring, smoothing, edge detection) 过滤(例如,模糊,轮廓绘制,平滑,边缘检测)
- image enhancement (for example, sharpening, brightness adjustment, contrast) 图像增强(例如,锐化,亮度调整,对比度)
- geometrical, color and other transforms 几何,颜色和其他变换
- adding text to images 向图像添加文本
- cutting, pasting and merging images 剪切,粘贴和合并图像
- creating thumbnails. 创建缩略图。
PIL和枕头 (PIL and Pillow)
One issue with PIL is that its most recent version, 1.1.7, was released in 2009, and only supports Python 1.5.2–2.7. Although the PIL site promises a forthcoming version for Python 3.X, its last commit was in 2011, and it appears that development has been discontinued.
PIL的一个问题是其最新版本1.1.7于2009年发布,仅支持Python 1.5.2–2.7。 尽管PIL网站承诺即将发布适用于Python 3.X的版本,但其最后一次提交是在2011年,并且看来开发已经停止。
Fortunately, all is not lost for Python 3.X users. A project called Pillow has forked the PIL repository and added Python 3.X support. Given that most readers will probably be working with Python 3.X, I'll focus on the Pillow update in this article.
幸运的是,Python 3.X用户并不会丢失所有内容。 一个名为Pillow的项目已经分叉了PIL存储库,并添加了Python 3.X支持。 鉴于大多数读者可能会使用Python 3.X,因此本文将重点介绍Pillow更新。
安装枕 (Installing Pillow)
Since Pillow supports versions of Python back to Python 2.4, I'll only focus on installing Pillow and not the older version of PIL.
由于Pillow支持Python版本回到Python 2.4,因此我将只专注于安装Pillow,而不是较早版本的PIL。
在Mac上使用Python (Python on a Mac)
I'm currently writing this tutorial on a Mac OS X Yosemite 10.10.5, and thus will describe how to install Pillow on a Mac OS X machine. But, don't worry, I'll provide a link at the end of this section that describes how to install Pillow on other operating systems.
我目前正在Mac OS X Yosemite 10.10.5上编写本教程,因此将描述如何在Mac OS X机器上安装Pillow。 但是,不用担心,我将在本节末尾提供一个链接,该链接描述如何在其他操作系统上安装Pillow。
I just want to note here that Mac OS X comes with Python pre-installed. However, the version most likely will be prior to 3.X.
我只想在此指出Mac OS X预先安装了Python。 但是,该版本最有可能在3.X之前。
For instance, on my machine, when I run $ python --version
in the terminal, I get Python 2.7.10
.
例如,在我的机器上,当我在终端中运行$ python --version
时,我得到Python 2.7.10
。
Python和pip (Python and pip)
A very easy way to install Pillow is through pip.
安装Pillow的一种非常简单的方法是通过pip 。
If you don't have pip installed on your machine, simply type the following command in your terminal, and you're all done:
如果您的计算机上未安装pip,只需在终端中键入以下命令,即可完成操作:
$ sudo easy_install pip
Now, to install Pillow, simply type the following in your terminal:
现在,要安装Pillow,只需在终端中键入以下内容:
$ sudo pip install pillow
That was easy, wasn't it?
那很容易,不是吗?
As I promised, for installing Pillow on other operating systems, you can find the instructions for that here.
如我所承诺的,要在其他操作系统上安装Pillow,您可以在此处找到相关说明。
一些例子 (Some Examples)
In this section, I'll demonstrate a few simple things we can do with PIL.
在本节中,我将演示我们可以使用PIL进行的一些简单操作。
I'll perform these tests on the following image:
我将在以下图像上执行这些测试:
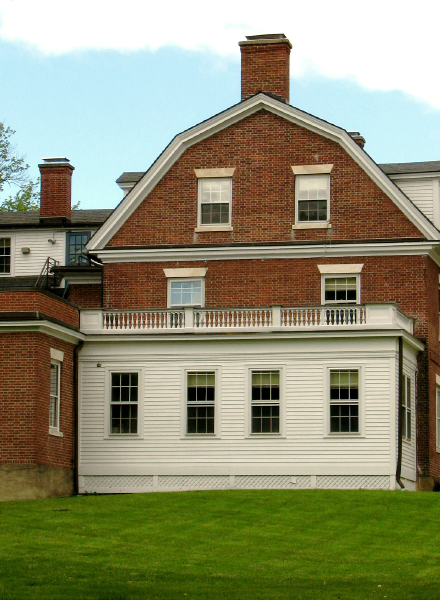
If you'd like to follow along with these examples, download the image.
如果您想遵循这些示例,请下载图片。
读取图像 (Read an image)
This is the most basic operation in an image processing task, since to process an image, you must read it first. With PIL, this can be easily accomplished as follows:
这是图像处理任务中最基本的操作,因为要处理图像,必须先阅读它。 使用PIL,可以很容易地完成以下操作:
from PIL import Image
img = Image.open('brick-house.png')
Notice here that img
is a PIL image object, created by the open()
function, which is part of the PIL Image
module.
注意, img
是一个由open()
函数创建的PIL图像对象,它是PIL Image
模块的一部分。
You can also read already open files, or from a string, or from a tar archive.
您也可以从字符串或tar存档中读取已打开的文件。
将图像转换为灰度,显示并保存 (Convert an image to grayscale, display it, and save it)
The file brick-house.png
is a color image. To convert it to grayscale, display it, and then save the new grayscale image, you can simply do the following:
文件brick-house.png
是彩色图像。 要将其转换为灰度 ,显示它,然后保存新的灰度图像,您只需执行以下操作:
from PIL import Image
img = Image.open('brick-house.png').convert('L')
img.show()
img.save('brick-house-gs','png')
Notice that we have used three main functions to perform this operation: convert()
, show()
, and save()
. Since we want to convert to a grayscale image, the L
parameter was used with convert()
.
注意,我们使用了三个主要函数来执行此操作: convert()
, show()
和save()
。 由于我们要转换为灰度图像,因此将L
参数与convert()
。
Here is the returned image:
这是返回的图像:
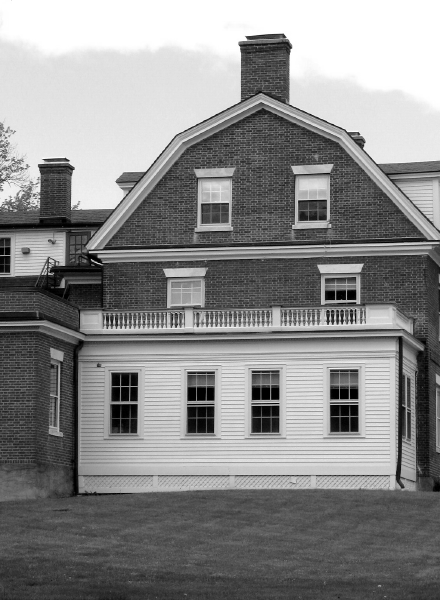
转换为其他图像类型 (Convert to another image type)
The image we are working on is of type png
. Say that you want to convert it to another image type, for instance jpg
. This operation can be done using the save()
function we used to save our result (write output to disk) in the above subsection:
我们正在处理的图像是png
类型。 假设您要将其转换为其他图像类型,例如jpg
。 可以使用上面小节中用来保存结果(将输出写入磁盘)的save()
函数来完成此操作:
from PIL import Image
img = Image.open('brick-house.png')
img.save('brick-image','jpeg')
调整图像大小 (Resize an image)
The size (dimensions) of our original image is 440 x 600px. If we want to resize it, and make it of size 256 x 256px, this can be done as follows:
我们原始图像的大小(尺寸)为440 x 600像素。 如果我们要调整大小,并使其尺寸为256 x 256像素,则可以按以下步骤进行操作:
from PIL import Image
img = Image.open('brick-house.png')
new_img = img.resize((256,256))
new_img.save('brick-house-256x256','png')
This produces a new square image:
这将产生一个新的正方形图像:
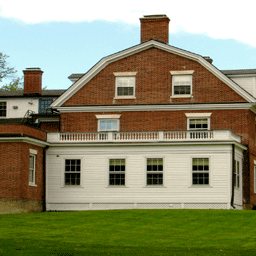
As you can see, this squeezes the image into the desired dimensions rather than cropping it, which may not be what you want. You can, of course, also crop the image while retaining the proper aspect ratio.
如您所见,这会将图像压缩到所需的尺寸,而不是裁剪图像,这可能不是您想要的。 当然,您也可以在保留适当的宽高比的同时裁剪图像。
结论 (In Conclusion)
This quick introduction is just intended to scratch the surface of PIL and to demonstrate how easily some complex image processing tasks can be accomplished in Python through the PIL library.
这份快速入门只是为了了解PIL的表面,并演示如何通过PIL库在Python中轻松完成某些复杂的图像处理任务。
The many other operations you can perform using this library are described in the comprehensive Pillow documentation, where you can read more details about the issues described above, along with handy tutorials.
全面的Pillow文档中介绍了可以使用此库执行的许多其他操作,您可以在其中阅读有关上述问题的更多详细信息以及方便的教程。
I hope this introduction has inspired you to try out image manipulation with Python. Have fun!
我希望这篇介绍会启发您尝试使用Python进行图像处理。 玩得开心!
翻译自: https://www.sitepoint.com/manipulating-images-with-the-python-imaging-library/
python图像处理库