The following is a short extract from our book, Jump Start Sass, written by Hugo Giraudel and Miriam Suzanne. It’s the ultimate beginner’s guide to Sass. SitePoint Premium members get access with their membership, or you can buy a copy in stores worldwide.
以下是Hugo Giraudel和Miriam Suzanne所著《 Jump Start Sass 》一书的摘录。 这是Sass的终极入门指南。 SitePoint Premium成员可以使用其成员身份进行访问,或者您可以在世界各地的商店中购买副本。
Clean, beautiful code should be a goal in every project. If other developers need to make a change, they should be able to read what is there and understand it. Readable code is the core of maintainability, and the first step towards readable code is a good linter. Like a good spell-checker, the linter should catch all your small typos and formatting mistakes, so it’s not left to others to do so. It’s the first line of defense before a good code review with other developers.
干净,漂亮的代码应该成为每个项目的目标。 如果其他开发人员需要进行更改,则他们应该能够阅读并理解其中的内容。 可读代码是可维护性的核心,并朝向可读代码的第一个步骤是一个很好的棉绒 。 像一个好的拼写检查器一样,lint应该捕获所有小的拼写错误和格式错误,因此不要让别人这样做。 在与其他开发人员进行良好的代码审查之前,这是第一道防线。
There are several great linters for Sass: scss-lint is a Ruby gem, and the newer sasslint and stylelint, which are npm packages for Node. Both allow you to configure linting rules for your project, such as maximum nesting levels, leading zeros on decimals, and organization of properties in a block. You can even create your own rules as needed.
Sass有很多出色的功能: scss-lint是Ruby宝石,而较新的sasslint和stylelint是Node的npm软件包。 两者都允许您为项目配置起毛规则,例如最大嵌套级别,小数点前的零以及块中的属性组织。 您甚至可以根据需要创建自己的规则。
Sass Guidelines are handy for organizing your project, setting up your linters, establishing naming conventions, and so on. Written by Hugo, it’s an opinionated styleguide for your code; it might not all work for you, but it’s a great place to start.
Sass指南可用于组织项目,设置样机,建立命名约定等。 由Hugo撰写,它是您代码的自以为是的样式指南; 它可能并不全为您服务,但这是一个不错的起点。
If you’re using Sass variables, functions, and mixins, it’s recommended that you document how they work. Toolkit authors will find it particularly important, but anyone who has extensive tooling built into their projects should also consider documentation for their team. Another great tool from Hugo is SassDoc, an npm package that parses your Sass comments and generates a beautiful static site with your documentation.
如果您使用的是Sass变量,函数和混合,建议您记录一下它们的工作方式。 工具包作者会发现它特别重要,但是任何在项目中内置大量工具的人都应该考虑为其团队使用文档。 Hugo的另一个很棒的工具是SassDoc ,这是一个npm软件包,可以解析您的Sass注释并使用您的文档生成漂亮的静态站点。
Here’s the SassDoc comment for our tint(..)
function in Accoutrement-Colors. It starts with a general description, and then explicitly documents each parameter and the expected return:
这是Accoutrement-Colors中我们tint(..)
函数的SassDoc注释。 它以一般描述开始,然后显式记录每个参数和预期的回报:
/// Mix a color with `white` to get a lighter tint.
///
/// @param {String | list} $color -
/// The name of a color in your palette,
/// with optional adjustments in the form of `(<function-name>:<args>)`.
/// @param {Percentage} $percentage -
/// The percentage of white to mix in.
/// Higher percentages will result in a lighter tint.
///
/// @return {Color} -
/// A calculated css-ready color-value based on your global color palette.
@function tint(
$color,
$percentage
) {
/* … */
}
Using the default theme (from which there are several to choose, or you can design your own), SassDoc converts that comment into a static website, as shown below.
使用默认主题(可以从中选择多个主题,也可以设计自己的主题),SassDoc将该评论转换为静态网站,如下所示。
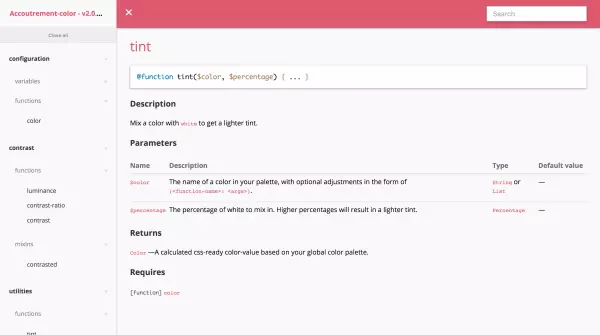
Testing is also important if you are doing anything complex with functions or mixins. It’s a good way to ensure your code won’t break any time you make adjustments, but it can also be helpful in developing new features. If you write the tests first, you’ll know exactly if the feature works correctly when your tests pass!
如果您要对函数或mixin做任何复杂的事情,测试也很重要。 这是确保代码在进行任何调整时都不会中断的好方法,但对开发新功能也有帮助。 如果您首先编写测试,则在测试通过时,您将确切知道该功能是否正常运行!
True is a unit-testing toolkit from yours truly, written in pure Sass so that it works anywhere Sass is compiled. The core testing happens in assertion functions: assert-equal(..)
, assert-unequal(..)
, assert-true(..)
, and assert-false(..)
. These are organized into tests, and can be grouped in test modules. Here’s an example of True testing our tint(..)
function:
True是使用纯Sass编写的真正的单元测试工具包,因此可以在编译Sass的任何地方使用。 核心测试发生在断言函数中: assert-equal(..)
, assert-unequal(..)
, assert-true(..)
和assert-false(..)
。 这些被组织成测试,并且可以分组在测试模块中。 这是True测试我们的tint(..)
函数的示例:
@include test-module('Tint [function]') {
@include test('Adjusts the tint of a color') {
@include assert-equal(
tint('primary', 25%),
mix(#fff, color('primary'), 25%),
'Returns a color mixed with white at a given weight.');
}
}
When compiled, True will output CSS comments with detailed results, and warn you in the console if any tests fail:
编译后,True将输出带有详细结果CSS注释,如果任何测试失败,则在控制台中警告您:
/* # Module: Tint [function] */
/* ------------------------- */
/* Test: Adjusts the tint of a color */
/* ✔ Returns a color mixed with white at a given weight. */
/* … */
/* # SUMMARY ---------- */
/* 16 Tests: */
/* - 14 Passed */
/* - 0 Failed */
/* - 2 Output to CSS */
/* -------------------- */
What does it mean that two tests were “output to CSS” in this example? Those tests aren’t shown, but they are testing mixin output. Using pure CSS, True can only confirm the results of function tests, so mixin tests are simply output to the CSS where they can be compared manually (gross) or with a CSS parser (better!). To make that easy, True integrates with JavaScript test runners such as Mocha, and has a Ruby command line interface written by Scott Davis. Either one will parse the CSS output completely, including the output from mixins, and give you full results for both function and mixin tests:
在此示例中,两个测试“输出到CSS”是什么意思? 这些测试没有显示,但是它们正在测试mixin输出。 使用纯CSS,True只能确认功能测试的结果,因此混合测试仅输出到CSS,可以手动比较(粗略)或使用CSS解析器比较(更好!)。 为了简化这一过程,True与JavaScript测试运行程序(例如Mocha)集成在一起,并具有由Scott Davis编写的Ruby命令行界面。 任何一种都将完全解析CSS输出,包括mixins的输出,并为您提供功能和mixin测试的完整结果:
Luminance [function] ✓ Returns luminance of a color Contrast Ratio [function] ✓ Returns contrast ratio between two colors Contrast [function] ✓ Dark on light ✓ Light on dark ✓ Default light fallback ✓ Default dark fallback ✓ Multiple contrast options contrasted [mixin] ✓ Dark on light ✓ Light on dark Tint [function] ✓ Adjusts the tint of a color Shade [function] ✓ Adjusts the shade of a color Color [function] ✓ Named color ✓ Referenced color ✓ Adjusted color ✓ Complex nesting of colors ✓ Multiple adjustment function arguments 16 passing (11ms)