tp5使用monolog
Logging is an important part of the app development/maintenance cycle. It’s not just about the data you log, but also about how you do it. In this article, we are going to explore the Monolog package and see how it can help us take advantage of our logs.
日志记录是应用程序开发/维护周期的重要组成部分。 这不仅与您记录的数据有关,而且与您如何做有关。 在本文中,我们将探索Monolog软件包,并了解它如何帮助我们利用日志。
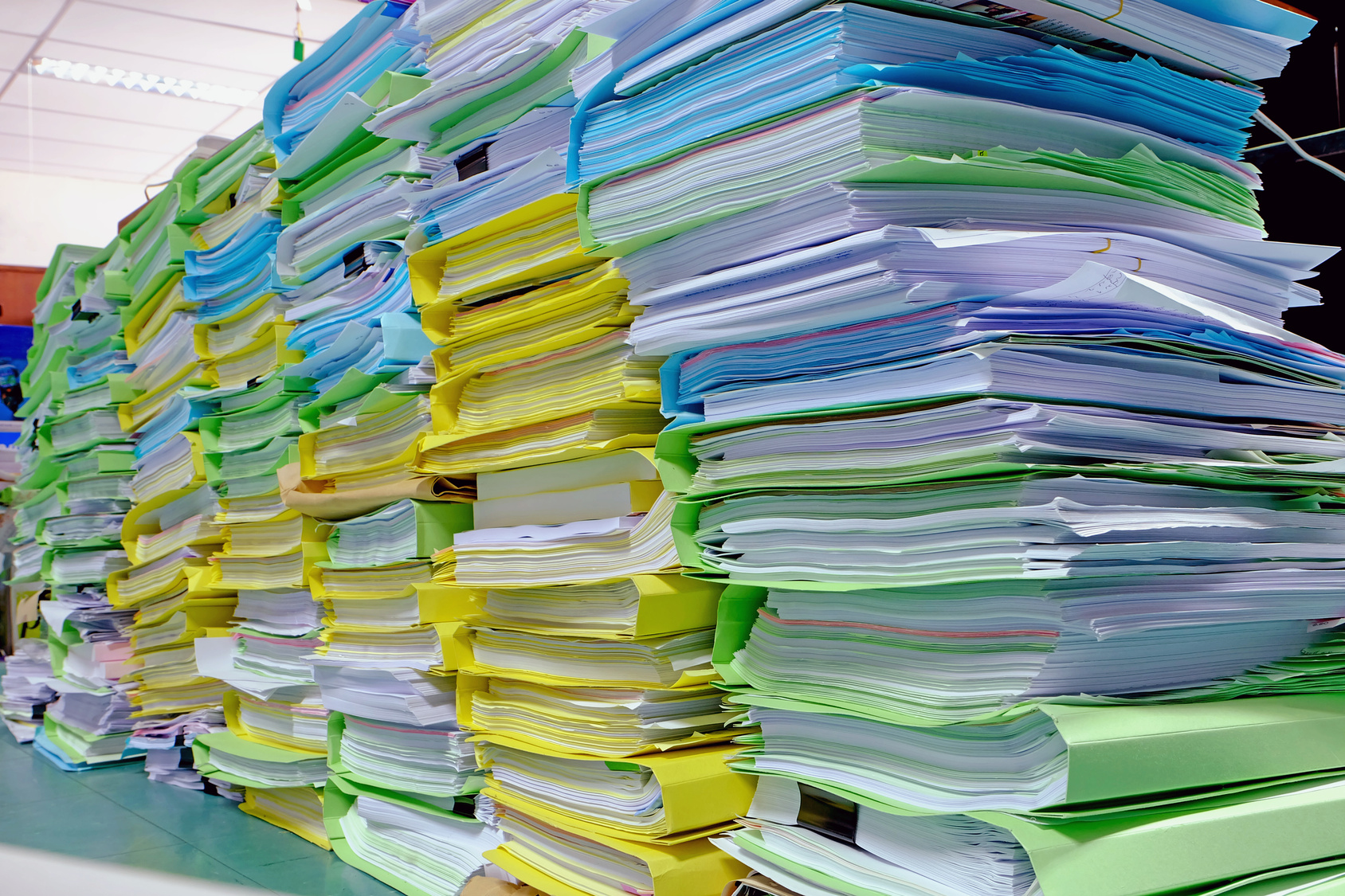
安装 (Installation)
Monolog is available on Packagist, which means that you can install it via Composer.
Packagist上提供了Monolog,这意味着您可以通过Composer安装它。
composer require 'monolog/monolog:1.13.*'
However, there is a great chance that you’re using a framework for your app. Monolog provides a list of integrations for most popular frameworks. I’m using Silex for this demo, but I won’t be using the provided integration to show how you can configure Monolog for any of your apps.
但是,您很有可能正在为您的应用程序使用框架。 Monolog提供了大多数流行框架的集成列表 。 我在此演示中使用Silex,但不会使用提供的集成来展示如何为任何应用程序配置Monolog。
记录仪 (The Logger)
When you create a new logger, you should give it a channel name. This is how you distinguish your list of loggers. I will bind my logger to the app container.
创建新的记录器时,应为其指定一个频道名称。 这是您区分记录器列表的方式。 我将记录器绑定到应用程序容器。
// app/bootstrap/container.php
$logger = new \Monolog\Logger('general');
$app->container->logger = $logger;
Because Monolog is PSR-3, you can be sure that you are adhering to the PHP-FIG standards. This will allow you to switch to any other implementation if you don’t feel comfortable with Monolog (I don’t see any reason why you wouldn’t). You can now start logging using one of the following methods, depending on your log level. (log
, debug
, info
, warning
, error
, critical
, alert
, emergency
).
由于Monolog是PSR-3 ,因此可以确定您遵循PHP-FIG标准。 如果您对Monolog不满意,这将允许您切换到其他任何实现(我看不出为什么不这样做)。 现在,根据您的日志级别 ,可以使用以下方法之一开始日志记录。 ( log
, debug
, info
, warning
, error
, critical
, alert
, emergency
)。
$app->container->logger->info("Just an INFO message.");
处理程序 (Handlers)
When you log something, it goes through the list of registered handlers. A handler needs to specify a log level it will be handling and a bubble status deciding wether to propagate the message or not. You can see that we didn’t register any handlers for our logger so far. By default, Monolog will use the \Monolog\Handler\StreamHandler
to log to the standard error output. You can see the list of available handlers in the documentation.
当您记录某些内容时,它将遍历已注册处理程序的列表。 处理程序需要指定将要处理的日志级别和气泡状态,以决定是否传播消息。 您可以看到,到目前为止,我们尚未为记录器注册任何处理程序。 默认情况下,Monolog将使用\Monolog\Handler\StreamHandler
记录到标准错误输出。 您可以在文档中看到可用处理程序的列表。
To illustrate the use of multiple handlers and bubbling, let’s assume that we want to log our info messages to the browser console and error message to the terminal output.
为了说明多个处理程序和冒泡的用法,我们假设我们要将信息消息记录到浏览器控制台,将错误消息记录到终端输出。
// app/bootstrap/container.php
$logger = new \Monolog\Logger('general');
$browserHanlder = new \Monolog\Handler\BrowserConsoleHandler(\Monolog\Logger::INFO);
$streamHandler = new \Monolog\Handler\StreamHandler('php://stderr', \Monolog\Logger::ERROR);
$logger->pushHandler($browserHanlder);
$logger->pushHandler($streamHandler);
$app->container->logger = $logger;
// app/routes.php
$app->get('/admin/users', function () use ($app) {
$app->container->logger->info("Another INFO message");
$app->container->logger->error("just another ERROR message");
// ...
});
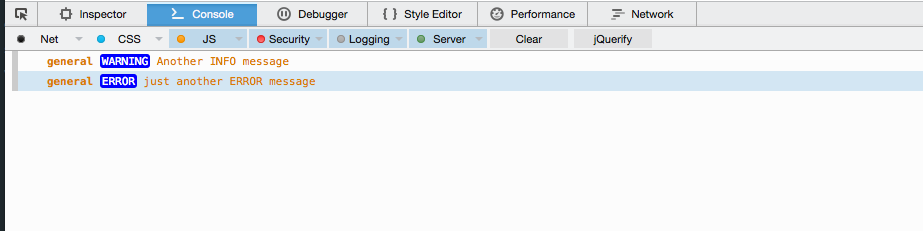

The error message was logged to the terminal console as expected, but why does it appear on the browser console? This is the bubbling option that Monolog provides. The error message was logged to the terminal first, and as long as the bubbling option is set to true, it will continue its way to the top of the handlers queue. Now, lets set the bubbling option to false on the stream handler.
错误消息已按预期记录到终端控制台,但是为什么它会出现在浏览器控制台上? 这是Monolog提供的冒泡选项。 该错误消息首先被记录到终端,并且只要冒泡选项设置为true,它就会继续前进到处理程序队列的顶部。 现在,让我们在流处理程序上将冒泡选项设置为false。
// app/bootstrap/container.php
// ...
$streamHandler = new \Monolog\Handler\StreamHandler('php://stderr', \Monolog\Logger::ERROR, false);
// ...
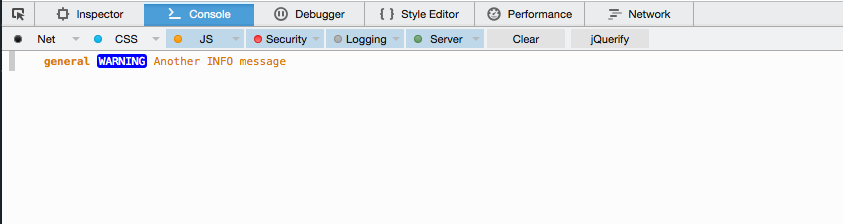

Now you can see that the error log didn’t show on the browser console, and that’s how you can separate your log levels.
现在您可以看到错误日志没有显示在浏览器控制台上,这就是您分隔日志级别的方法。
You may also use external services such as HipChat, Slack, etc, as message recipients. Let’s assume that we have a developers channel on Slack, and we want to let everybody know that an error occurred with the necessary details.
您还可以将外部服务(例如HipChat,Slack等)用作邮件收件人。 假设我们在Slack上有一个开发人员频道,并且想让所有人都知道发生了必要的细节错误。
松弛处理程序 (Slack Handler)
Before pushing the Slack handler to the queue, you need to get a token to give the handler the authorization to post to your Slack channel. Go to the authorization page and generate a new token.
在将Slack处理程序推送到队列之前,您需要获取一个令牌以赋予处理程序授权发布到您的Slack通道的权限。 转到授权页面并生成一个新令牌。
// app/bootstrap/container.php
// ...
$slackHandler = new \Monolog\Handler\SlackHandler('xoxp-5156076911-5156636951-6084570483-7b4fb8', '#general', 'ChhiwatBot');
$logger->pushHandler($slackHandler);
// ...
You only need to specify your Slack token and the channel name, and the third optional parameter is the robot name. The log level for Slack is CRITICAL
, but you can change it using the setLevel
method.
您只需指定Slack令牌和通道名称,第三个可选参数是机械手名称。 Slack的日志级别为CRITICAL
,但是您可以使用setLevel
方法更改它。
$slackHandler->setLevel(\Monolog\Logger::ERROR);
After sending the log message, you can visit your channel to see the logged error message.
发送日志消息后,您可以访问您的频道以查看记录的错误消息。
$app->container->logger->error("just an ERROR message");
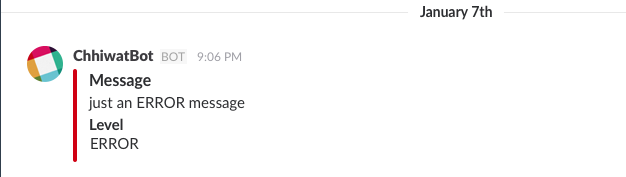
格式化程序 (Formatters)
Until now, all of our logs were pre-formatted and we didn’t get the chance to specify the logging format. This is what formatters are for. Every handler has a default formatter, and if you don’t specify one you’ll get the LineFormatter
. For every formatter you can always switch to another one, like the HtmlFormatter
. Some handlers have their own formatters like the ChromePHPHandler
, LogglyHandler
, etc.
到目前为止,我们所有的日志都是预先格式化的,因此我们没有机会指定日志格式。 这就是格式化程序的用途。 每个处理程序都有一个默认的格式化程序,如果您不指定默认格式化程序,则会得到LineFormatter
。 对于每种格式程序,您都可以随时切换到另一种格式程序,例如HtmlFormatter
。 一些处理程序具有自己的格式化程序,例如ChromePHPHandler
, LogglyHandler
等。
// app/bootstrap/container.php
$browserHanlder = new \Monolog\Handler\BrowserConsoleHandler(\Monolog\Logger::INFO);
$browserHanlder->setFormatter(new \Monolog\Formatter\HtmlFormatter);
//...
Now when you log a new message it will be formatted in HTML and then logged to the browser console.
现在,当您记录新消息时,它将以HTML格式设置,然后记录到浏览器控制台中。
$app->container->logger->info("Another INFO message");
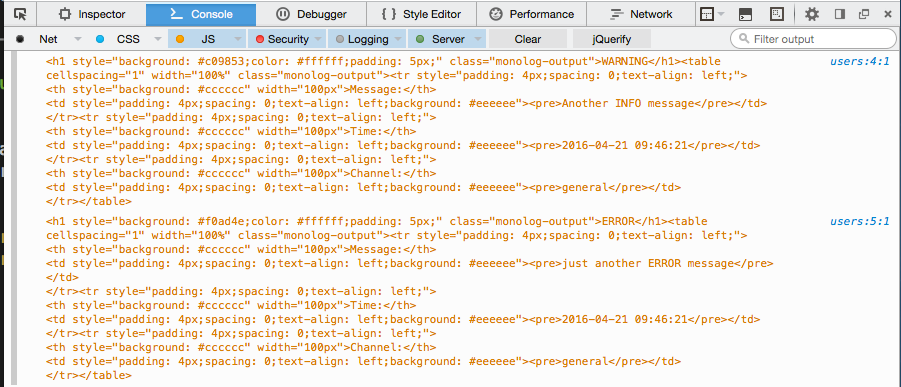
You can also extend the LineFormatter
to include more details on the message. The available variables are:
您还可以扩展LineFormatter
以包括有关消息的更多详细信息。 可用变量为:
message
: Log message.message
:日志消息。context
: list of data passed when creating the logger. (new Logger('channelName', ['user' => 'adam'])
).context
:创建记录器时传递的数据列表。 (new Logger('channelName', ['user' => 'adam'])
)。level
: Error level code.level
:错误级别代码。level_name
: Error level name.level_name
:错误级别名称。channel
: Logger channel name.channel
:记录器通道名称。datetime
: Current date time.datetime
:当前日期时间。extra
: Data pushed by preprocessors.extra
:预处理器推送的数据。
预处理器 (Preprocessors)
Preprocessors are useful for adding more details to your log. For example, the WebProcessor
adds more details about the request like (url
, ip
, etc). When creating your logger instance you set the list of handlers and processors, but you can also use the pushProcessor
method to add them later.
预处理程序对于将更多详细信息添加到日志中很有用。 例如, WebProcessor
添加有关请求的更多详细信息,例如( url
, ip
等)。 在创建记录器实例时,可以设置处理程序和处理器的列表,但也可以在pushProcessor
使用pushProcessor
方法添加它们。
// app/bootstrap/container.php
$logger = new \Monolog\Logger('general');
$logger->pushProcessor(new \Monolog\Processor\WebProcessor);
$browserHanlder = new \Monolog\Handler\BrowserConsoleHandler(\Monolog\Logger::INFO);
$logger->pushHandler($browserHanlder);
//...
When logging anything, the log record will be passed through the list of registered processors, and you’ll get something like the following.
在记录任何内容时,日志记录将通过已注册处理器的列表传递,您将获得类似以下内容的信息。
$app->container->logger->info("Another INFO message");
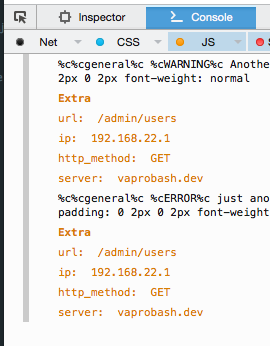
Monolog provides a list of useful preprocessor for including details about memory peaks, user id, etc. You can check the list of available preprocessors in the documentation.
Monolog提供了有用的预处理器列表,其中包括有关内存峰值,用户ID等的详细信息。您可以在文档中查看可用的预处理器列表。
结语 (Wrap Up)
Monolog is one of the best available logging libraries out there, and it’s also integrated into most of the popular frameworks like Symfony, Laravel, Silex, Slim, etc. If you have any comments or questions, you can post them below and I’ll do my best to answer them.
Monolog是目前最好的日志记录库之一,它也已集成到大多数流行的框架中,例如Symfony,Laravel,Silex,Slim等。如果您有任何意见或疑问,可以将其发布在下面,我将尽我所能回答他们。
翻译自: https://www.sitepoint.com/logging-with-monolog-from-devtools-to-slack/
tp5使用monolog