laravel5.2.15
In Laravel 5.1, the framework includes functionality called broadcasting events that makes it easy to create real-time apps in PHP. With this new functionality, an app can publish events to various cloud-based real-time PubSub solutions, like Pusher, or to Redis.
在Laravel 5.1中,该框架包含称为广播事件的功能,该功能使使用PHP创建实时应用程序变得容易。 借助此新功能,应用程序可以将事件发布到各种基于云的实时PubSub解决方案,例如Pusher或Redis。
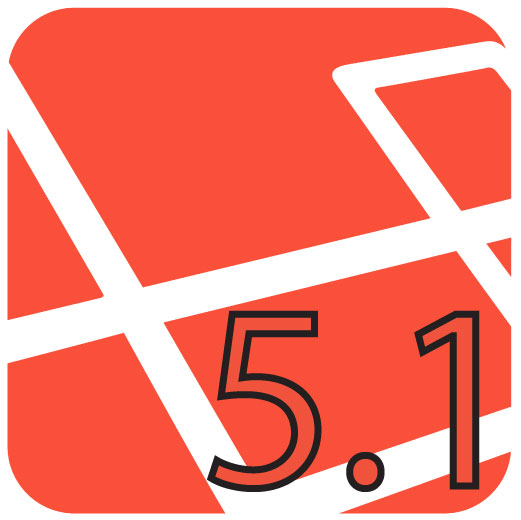
In this article, we examine a simple todo app and turn it into a real-time app using Laravel’s broadcasting events.
在本文中,我们研究了一个简单的待办事项应用程序,并使用Laravel的广播事件将其转换为实时应用程序。
下载并安装应用 (Download and Install App)
The easiest way to get started is to spin up an instance of Homestead Improved. If you don’t want to use Homestead Improved, you must have git and composer installed on your system.
最简单的入门方法是启动Homestead Improvement的实例。 如果您不想使用Homestead Enhanced,则必须在系统上安装git和composer 。
We will put the starter code in a directory called todo-app
by cloning a git repo.
我们将通过克隆git repo将入门代码放在名为todo-app
的目录中。
git clone https://github.com/cwt137/l51-todo-app todo-app
After the cloning is finished, go into the todo-app
directory. We need to install all the app dependencies by running the following command (depending on how your composer is set up, the command might be slightly different):
克隆完成后,进入todo-app
目录。 我们需要通过运行以下命令来安装所有应用程序依赖项(取决于您的作曲者的设置方式,该命令可能会略有不同):
composer install
After all the dependencies are installed, we must set up our database.
安装所有依赖项之后,我们必须设置数据库。
php artisan migrate
测试非实时应用 (Testing the Non Real-time App)
This app is now functional, but without the real-time functionality provided by Laravel’s broadcasting events. Open up the homepage in two browsers and put them side by side so you can do a comparison. If you do not have two different browsers, you can use two browser windows.
该应用程序现在可以运行,但是没有Laravel广播事件提供的实时功能。 在两个浏览器中打开主页,并将它们并排放置,以便进行比较。 如果没有两个不同的浏览器,则可以使用两个浏览器窗口。
Manipulate the todo list in the first browser window. Then do something in the second browser window. You will notice that the other browser window that you were not in does not update without hitting the refresh button. This is because there is no real-time functionality. Let’s add some.
在第一个浏览器窗口中操作待办事项列表。 然后在第二个浏览器窗口中执行某些操作。 您会注意到,您所不在的其他浏览器窗口在未单击刷新按钮的情况下不会更新。 这是因为没有实时功能。 让我们添加一些。
向应用程序添加实时功能 (Adding Real-time Capabilities to the App)
Adding real-time capabilities will allow both browser windows to update their content without waiting for a hit of the refresh button.
添加实时功能将允许两个浏览器窗口更新其内容,而无需等待按下刷新按钮。
In this example, we will define three Laravel event classes that get triggered at various times in our app. One event is the ItemCreated
event that is triggered when a new item is created. The second event is the ItemUpdated
event that is triggered when an item is updated (is marked completed or uncompleted). The last is the ItemDeleted
event that is triggered when an item is removed from the todo list.
在此示例中,我们将定义三个Laravel事件类,它们在应用程序中的不同时间触发。 一个事件是在创建新项目时触发的ItemCreated
事件。 第二个事件是ItemUpdated
事件,该事件在更新项目(标记为已完成或未完成)时触发。 最后一个是ItemDeleted
事件,当从待办事项列表中删除一个项目时会触发该事件。
广播活动 (Broadcasting Events)
To do broadcasting events, we will create a regular Laravel event, but we will implement an interface called ShouldBroadcast
. If Laravel sees that an event class implements ShouldBroadcast
it knows that it is a broadcasting event. This interface requires us to define a method inside our event class called broadcastOn
. It should return an array of strings which are the channels that this event should be broadcasted on.
为了进行广播事件,我们将创建一个常规的Laravel事件,但是我们将实现一个名为ShouldBroadcast
的接口。 如果Laravel看到事件类实现了ShouldBroadcast
则它知道这是一个广播事件。 此接口要求我们在事件类中定义一个名为broadcastOn
。 它应返回一个字符串数组,这些字符串是应广播此事件的频道。
To create the event classes we need, run some artisan commands
要创建我们需要的事件类,请运行一些artisan命令
php artisan make:event ItemCreated
php artisan make:event ItemUpdated
php artisan make:event ItemDeleted
Open up app/Events/ItemCreated.php
and replace the contents with the code below:
打开app/Events/ItemCreated.php
并将内容替换为以下代码:
<?php
namespace App\Events;
use App\Item;
use App\Events\Event;
use Illuminate\Queue\SerializesModels;
use Illuminate\Contracts\Broadcasting\ShouldBroadcast;
class ItemCreated extends Event implements ShouldBroadcast
{
use SerializesModels;
public $id;
/**
* Create a new event instance.
*
* @param Item $item
* @return void
*/
public function __construct(Item $item)
{
$this->id = $item->id;
}
/**
* Get the channels the event should be broadcast on.
*
* @return array
*/
public function broadcastOn()
{
return ['itemAction'];
}
}
Laravel’s event system will serialize this object and broadcast it out on the real-time cloud system using the itemAction
channel. The next couple of events are similar to the first.
Laravel的事件系统将序列化该对象,并使用itemAction
通道在实时云系统上广播该对象。 接下来的几个事件与第一个类似。
Open up app/Events/ItemUpdated.php
and replace the contents with the code below:
打开app/Events/ItemUpdated.php
并将内容替换为以下代码:
<?php
namespace App\Events;
use App\Item;
use App\Events\Event;
use Illuminate\Queue\SerializesModels;
use Illuminate\Contracts\Broadcasting\ShouldBroadcast;
class ItemUpdated extends Event implements ShouldBroadcast
{
use SerializesModels;
public $id;
public $isCompleted;
/**
* Create a new event instance.
*
* @param Item $item
* @return void
*/
public function __construct(Item $item)
{
$this->id = $item->id;
$this->isCompleted = (bool) $item->isCompleted;
}
/**
* Get the channels the event should be broadcast on.
*
* @return array
*/
public function broadcastOn()
{
return ['itemAction'];
}
}
Open up app/Events/ItemDeleted.php
and replace the contents with the code below:
打开app/Events/ItemDeleted.php
并将内容替换为以下代码:
<?php
namespace App\Events;
use App\Item;
use App\Events\Event;
use Illuminate\Queue\SerializesModels;
use Illuminate\Contracts\Broadcasting\ShouldBroadcast;
class ItemDeleted extends Event implements ShouldBroadcast
{
use SerializesModels;
public $id;
/**
* Create a new event instance.
*
* @param Item $item
* @return void
*/
public function __construct(Item $item)
{
$this->id = $item->id;
}
/**
* Get the channels the event should be broadcast on.
*
* @return array
*/
public function broadcastOn()
{
return ['itemAction'];
}
}
数据库事件 (Database Events)
There are a few places in which we could fire events for our project. We could do it inside the controller, or inside the database event triggers. In this example we will use the database triggers because it feels more natural to me and doesn’t fill up the controller with extra stuff. Also, by putting the event firing logic inside the database layer, it makes it possible to fire events when a command-line program is built or when an app is built as a cron job.
在一些地方,我们可以为我们的项目引发事件。 我们可以在控制器内部或数据库事件触发器内部进行操作。 在此示例中,我们将使用数据库触发器,因为它对我来说更自然,不会为控制器增加多余的东西。 另外,通过将事件触发逻辑放在数据库层中,可以在构建命令行程序或将应用程序作为cron作业构建时触发事件。
Eloquent, the database library, fires events every time a model is created, saved after an update, or deleted. We will listen to those events so we can fire our own broadcasting events. This will be done through a service provider.
雄辩的是,数据库模型每次创建,更新后保存或删除模型时都会触发事件。 我们将收听这些事件,以便触发自己的广播事件。 这将通过服务提供商来完成。
Open the file at app/Providers/AppServiceProvider.php
and replace the contents with the following code:
在app/Providers/AppServiceProvider.php
打开文件,然后将内容替换为以下代码:
<?php
namespace App\Providers;
use Event;
use App\Item;
use App\Events\ItemCreated;
use App\Events\ItemUpdated;
use App\Events\ItemDeleted;
use Illuminate\Support\ServiceProvider;
class AppServiceProvider extends ServiceProvider
{
/**
* Bootstrap any application services.
*
* @return void
*/
public function boot()
{
Item::created(function ($item) {
Event::fire(new ItemCreated($item));
});
Item::updated(function ($item) {
Event::fire(new ItemUpdated($item));
});
Item::deleted(function ($item) {
Event::fire(new ItemDeleted($item));
});
}
/**
* Register any application services.
*
* @return void
*/
public function register()
{
//
}
}
推杆 (Pusher)
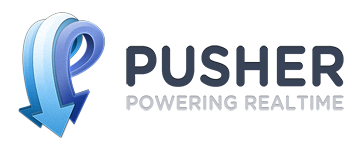
The real-time cloud service we will use for this example is Pusher. Support for this service is built into Laravel and is the easiest to set up.
在此示例中,我们将使用实时云服务Pusher。 Laravel内置了对此服务的支持,并且最容易设置。
注册 (Registration)
We must register for an account to get a set of credentials. After registering on the Pusher website, go to the admin screen and create a new app called todo-app
. Take note of the app_id
, key
and secret
. We will need those later.
我们必须注册一个帐户才能获得一组凭据。 在Pusher网站上注册后,进入管理屏幕并创建一个名为todo-app
的新todo-app
。 注意app_id
, key
和secret
。 我们稍后将需要这些。
Pusher PHP服务器库 (Pusher PHP Server Library)
For our app to use Pusher, we must add the server library to our project. This is done via composer.
为了使我们的应用程序使用Pusher,我们必须将服务器库添加到我们的项目中。 这是通过作曲家完成的。
composer require 'pusher/pusher-php-server:2.2.1'
JavaScript (JavaScript)
We will add some JavaScript to the bottom of our homepage. Open up resources/views/index.blade.php
, the blade template for the homepage, and put the following code just before the closing body tag
我们将在主页底部添加一些JavaScript。 打开resources/views/index.blade.php
(此页面的刀片模板),并将以下代码放在结束body标签之前
<script src="//js.pusher.com/2.2/pusher.min.js"></script>
<script>
var pusher = new Pusher("{{ env(PUSHER_KEY) }}");
</script>
<script src="js/pusher.js"></script>
The code above loads the Pusher Javascript client library, instantiates the Pusher object by passing our key into the constructor, and loads our Pusher specific app logic.
上面的代码加载Pusher Javascript客户端库,通过将我们的密钥传递给构造函数来实例化Pusher对象,并加载特定于Pusher的应用逻辑。
In the starter app, items were deleted and added based on events happening on the page (form submit, delete icon clicked, etc). This is why when we opened two browser windows, both did not get updated. One browser window can’t see what is going on in the other browser window. To make the app real-time, we will add and delete items based upon the events coming from Pusher. But first, open up public/js/app.js
and comment out all the calls to the addItem()
and removeItem()
functions. Be sure not to delete the two function declarations at the top of the file.
在入门应用程序中,根据页面上发生的事件(表单提交,单击的删除图标等)删除和添加了项目。 这就是为什么当我们打开两个浏览器窗口时,两个窗口都没有更新。 一个浏览器窗口看不到另一浏览器窗口中正在发生的事情。 为了使应用程序实时,我们将根据来自Pusher的事件添加和删除项目。 但是首先,打开public/js/app.js
并注释掉对addItem()
和removeItem()
函数的所有调用。 确保不要删除文件顶部的两个函数声明。
Create the file public/js/pusher.js
and put the following code into it.
创建文件public/js/pusher.js
并将以下代码放入其中。
( function( $, pusher, addItem, removeItem ) {
var itemActionChannel = pusher.subscribe( 'itemAction' );
itemActionChannel.bind( "App\\Events\\ItemCreated", function( data ) {
addItem( data.id, false );
} );
itemActionChannel.bind( "App\\Events\\ItemUpdated", function( data ) {
removeItem( data.id );
addItem( data.id, data.isCompleted );
} );
itemActionChannel.bind( "App\\Events\\ItemDeleted", function( data ) {
removeItem( data.id );
} );
} )( jQuery, pusher, addItem, removeItem);
The app subscribes to the itemAction
channel. By default, Lavavel uses the qualified class name of the event object as the Pusher event name. The Javascript code above sets up listeners for the three events App\Events\ItemCreated
, App\Events\ItemUpdated
, and App\Events\ItemDeleted
. There are callbacks that handle what happens when these events are triggered. As you can see the calls to getItem
and removeItem
were moved into the callbacks for the various events. So now, items are added or removed based on a Pusher event instead of a user event.
该应用程序订阅了itemAction
频道。 默认情况下,Lavavel使用事件对象的合格类名称作为Pusher事件名称。 上面的Javascript代码为三个事件App\Events\ItemCreated
, App\Events\ItemUpdated
和App\Events\ItemDeleted
设置了侦听器。 有回调处理这些事件被触发时发生的情况。 如您所见,对getItem
和removeItem
的调用已移至各种事件的回调中。 因此,现在,根据Pusher事件而不是用户事件来添加或删除项目。
测试实时应用 (Testing the Realtime App)
To test out this app, you need to set the keys for Pusher. By default, Laravel looks at environment variables for the Pusher information. Open up the .env
file and put the following lines at the bottom.
要测试此应用,您需要设置Pusher的键。 默认情况下,Laravel在环境变量中查找Pusher信息。 打开.env
文件,并将以下行放在底部。
PUSHER_KEY=YOUR_PUSHER_KEY
PUSHER_SECRET=YOUR_PUSHER_SECRET
PUSHER_APP_ID=YOUR_PUSHER_APP_ID
This will set up the variables. Where YOUR_PUSHER_KEY
, YOUR_PUSHER_SECRET
, YOUR_PUSHER_APP_ID
are your Pusher assigned key
, secret
and app_id
, respectively.
这将设置变量。 其中YOUR_PUSHER_KEY
, YOUR_PUSHER_SECRET
, YOUR_PUSHER_APP_ID
分别是您的Pusher分配的key
, secret
和app_id
。
Open up two browser windows again and manipulate the todo list in one of them. You will see the list automatically update in the other browser window without having to hit the refresh button.
再次打开两个浏览器窗口,并在其中一个窗口中操作待办事项列表。 您将在另一个浏览器窗口中看到列表自动更新,而无需单击刷新按钮。
最后的想法 (Final Thoughts)
Although not covered in this article, the framework used is extensible and if Laravel does not support your real-time solution, there might be a composer package with the appropriate broadcast driver for it already. Or you can create your own broadcast driver along with a service provider to load it.
尽管本文未涉及,但所使用的框架是可扩展的,并且如果Laravel不支持您的实时解决方案,则可能已经有一个composer程序包,带有适当的广播驱动程序。 或者,您可以创建自己的广播驱动程序以及服务提供商以进行加载。
With the new broadcasting events functionality built into Laravel 5.1, it is now easier for PHP developers to create real-time apps. This new real-time capability unlocks many possibilities that were only available to apps written for other platforms like Node.js.
通过Laravel 5.1中内置的新广播事件功能,PHP开发人员现在可以更轻松地创建实时应用程序。 这项新的实时功能释放了许多可能性,这些可能性仅适用于为其他平台(如Node.js)编写的应用程序。
翻译自: https://www.sitepoint.com/real-time-apps-laravel-5-1-event-broadcasting/
laravel5.2.15