python初学者
Are you in the process of learning Python? It’s a fantastic language to learn, but as with any language, it does present challenges that can seem overwhelming at times, especially if you’re teaching yourself.
您正在学习Python吗? 这是一门很棒的语言,但是与其他任何语言一样,它确实带来了有时似乎难以克服的挑战,尤其是当您自学时。
Given all the different ways of doing things in Python, we decided to compile a helpful list of issues that beginners often face — along with their solutions.
考虑到Python的所有不同处理方式,我们决定汇编一份初学者经常面临的有用问题列表及其解决方案。
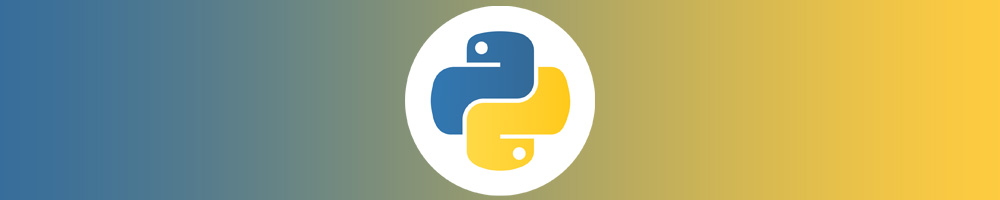
1.从终端读取 (1. Reading from the Terminal)
If you’re running a program on the terminal and you need user input, you may need to get it through the terminal itself. (Other alternatives include reading a file to get inputs.)
如果您正在终端上运行程序,并且需要用户输入,则可能需要通过终端本身来获取它。 (其他替代方法包括读取文件以获取输入。)
In Python, the raw_input
function facilitates this. The only argument the function takes is the prompt. Let’s look at a small program to see the result:
在Python中, raw_input
函数可简化此操作。 该函数采用的唯一参数是提示。 让我们看一个小程序以查看结果:
name = raw_input('Type your name and press enter: ')
print 'Hi ' + name
If you save those lines in a file (with a .py
extension) and execute it, you get the following result:
如果将这些行保存在文件中(扩展名为.py
)并执行它,则会得到以下结果:
Type your name and press enter: Shaumik
Hi Shaumik
One important thing to note here is that the variable is a string, and you need to filter and convert it to use it in a different form (like an input for the number of iterations or the length of a matrix):
这里要注意的重要一件事是变量是一个字符串,您需要对其进行过滤和转换以以不同的形式使用它(例如输入迭代次数或矩阵长度):
name = raw_input('Type your name and press enter: ')
print 'Hi ' + name
num = raw_input('How many times do you want to print your name: ')
for i in range(int(num)):
print name
2.用Python枚举 (2. Enumerating in Python)
Python often presents ways of doing things different from other popular programming languages like C++ and Java. When you traverse an array in other languages, you increment an integer from 0
and access the corresponding elements of the array. The following shows a crude way of doing the same:
Python经常提供与其他流行的编程语言(例如C ++和Java)不同的处理方式。 当您使用其他语言遍历数组时,可以从0
增加整数并访问数组的相应元素。 下面显示了执行此操作的粗略方法:
for (int i = 0; i < array_length; ++i)
cout << array[i];
However, in Python, you can simply traverse each element of the array without using the indices at all:
但是,在Python中,您可以简单地遍历数组的每个元素而根本不使用索引:
for item in array:
print item
What if there’s a need to access the indices too? The enumerate
function helps you do so. Enumeration of an array (or a list
, as it’s known in Python) creates pairs of the items in an array and their indices. The same can be demonstrated as follows:
如果也需要访问索引怎么办? enumerate
功能可以帮助您做到这一点。 数组(或列表
,如Python中所知)的枚举创建数组中的项目对及其索引。 可以证明如下:
>>> x = [10, 11, 12, 13, 14]
>>> for item in enumerate(x):
... print item
...
(0, 10)
(1, 11)
(2, 12)
(3, 13)
(4, 14)
Imagine a situation where you need to print every alternate item in an array. One way of doing so is as follows:
设想一种情况,您需要打印阵列中的每个备用项目。 一种方法如下:
>>> for index, item in enumerate(x):
... if index % 2 == 0:
... print item
...
10
12
14
3.通过Python执行外部命令 (3. Executing an External Command through Python)
At some point, you may need to execute a terminal command within a Python script. This can be achieved through the call
function under the subprocess
module. There are many ways to do this, one of which is shown below:
在某些时候,您可能需要在Python脚本中执行终端命令。 这可以通过subprocess
模块下的call
功能来实现。 有很多方法可以做到这一点,如下所示:
>>> from subprocess import call
>>> call('cal')
March 2016
Su Mo Tu We Th Fr Sa
1 2 3 4 5
6 7 8 9 10 11 12
13 14 15 16 17 18 19
20 21 22 23 24 25 26
27 28 29 30 31
0
The last zero in the output shows that the subprocess we created within our script ended normally. In other words, there were no issues in running the command.
输出中的最后一个零表示我们在脚本中创建的子进程正常结束。 换句话说,运行命令没有问题。
If you need to use arguments for the command, you need to append them to the main command as a list. For instance, to run the command ls -l
, the following needs to be done:
如果需要为命令使用参数,则需要将它们作为列表附加到主命令。 例如,要运行命令ls -l
,需要完成以下工作:
>>> from subprocess import call
>>> call(['ls', '-l'])
total 16
-rw-------@ 1 donny staff 439 Oct 21 16:06 chess.csv
-rw-r--r-- 1 donny staff 72 Mar 1 17:28 read.py
0
To check what happens to the 0
when something wrong happens, we can run a git command in a non git repository:
要检查发生错误时0
会发生什么,我们可以在非git存储库中运行git命令:
>>> from subprocess import call
>>> call(['git', 'status'])
fatal: Not a git repository (or any of the parent directories): .git
128
In the output, the second line is the output of the command, whereas 128
is the exit code.
在输出中,第二行是命令的输出,而128
是退出代码。
4.处理异常 (4. Working with Exceptions)
Python is an interpreted language, which means that the code is executed line by line. If an error is encountered on a line, further execution of the code stops. However, you can handle
known exceptions in Python using the try-except block. Let’s look at a simple example, by generating a runtime error of dividing by 0
:
Python是一种解释型语言,这意味着代码是逐行执行的。 如果在一行上遇到错误,则代码的进一步执行将停止。 但是,您可以使用try-except块处理
Python中的已知异常。 让我们看一个简单的示例,它生成一个运行时错误除以0
:
>>> x = 1/0
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ZeroDivisionError: integer division or modulo by zero
When the interpreter reaches this line, your program execution stops completely! However, using the try-except block can help avoiding the same.
当解释器到达此行时,您的程序执行将完全停止! 但是,使用try-except块可以帮助避免这种情况。
>>> try:
... x = 1/0
... except:
... print "Some error occurred"
...
Some error occurred
When such an error occurs within the try
block, the interpreter just executes the except
block. The except
block can further be extended by catching individual errors:
当try
块中发生此类错误时,解释器仅执行except
块。 通过捕获单个错误,可以进一步扩展except
块:
>>> try:
... x = 1/0
... except ZeroDivisionError:
... print "You tried to divide by zero"
... except:
... print "Some unknown error occurred"
...
You tried to divide by zero
You can go one step ahead, catch the exception and further process it (like log the error somewhere) by modifying the except
block:
您可以前进一步,捕获异常并通过修改except代码
块进一步处理该异常(例如将错误记录在某处):
>>> try:
... x = 1/0
... except Exception as e:
... print "Exception occurred: " + str(e)
...
Exception occurred: integer division or modulo by zero
5.使用模块 (5. Working with Modules)
While looking at the code of others, you’ll often encounter this block of code:
在查看其他人的代码时,您经常会遇到以下代码块:
def some_function():
...
if __name__ == '__main__':
...
This is used frequently when you create modules in Python. Ideally, the code in the last block usually demonstrates some basic usage of the function(s) above. When you run the file in the terminal, this code is executed. However, if you use this module in a different file for the purpose of using the function, this block is not executed. Confusing? Let’s understand this better through an example:
当您在Python中创建模块时,经常会使用它。 理想情况下,最后一块中的代码通常演示上述功能的一些基本用法。 在终端中运行文件时,将执行此代码。 但是,如果出于使用功能的目的在另一个文件中使用此模块,则不会执行该块。 令人困惑? 让我们通过一个示例更好地理解这一点:
# File print.py
def print_me():
print "me"
# demonstrating use of print_me
print_me()
Now, if I import this file to use the function in a new file, watch what happens:
现在,如果我导入此文件以在新文件中使用该功能,请注意会发生什么:
>>> import print
me
>>>
The function gets executed as soon as you import the file. To avoid this, we put the function under a if __name__ == '__main__':
block:
导入文件后立即执行该函数。 为了避免这种情况,我们将函数放在if __name__ == '__main__':
块:
# File print.py
def print_me():
print "me"
if __name__ == '__main__':
# demonstrating use of print_me
print_me()
When we modify the code, the print_me()
function is executed only when you run it as a file, but not when you import it as a module:
当我们修改代码时,仅在将其作为文件运行时才执行print_me()
函数,而在将其作为模块导入时则不会执行:
>>> import print
>>>
最后的想法 (Final Thoughts)
If Python isn’t your first programming language, it’s going to take some time to adjust to its way of doing things. I recommend you watch an interesting YouTube video of Raymond Hettinger explaining this Pythonic Way of programming. In this post, I’ve attempted to cover a few of these Pythonic issues.
如果Python不是您的第一门编程语言,它将需要一些时间来适应其工作方式。 我建议您观看雷蒙德·海廷格(Raymond Hettinger)的一段有趣的YouTube视频,其中介绍了这种Python编程方式 。 在本文中,我尝试介绍了其中一些Pythonic问题。
How was your Pythonic journey, or how is it still going? Do you prefer doing these tasks differently? Do let us know in the comments below!
您的Python之旅如何,或者进展如何? 您喜欢以不同的方式完成这些任务吗? 请在下面的评论中告诉我们!
翻译自: https://www.sitepoint.com/5-common-problems-faced-by-python-beginners/
python初学者