vue文件中怎么使用php
You may well be familiar with databases such as MySQL and Access which are an ever-increasingly common means of storing data. But data is also stored in files, like Word documents, event logs, spreadsheets, image files, and so on. Databases generally require a special query language to retrieve information, whereas files are ‘flat’ and usually appear as a stream of text.
您可能非常熟悉MySQL和Access等数据库,这些数据库是存储数据的越来越常用的方法。 但是数据也存储在文件中,例如Word文档,事件日志,电子表格,图像文件等。 数据库通常需要一种特殊的查询语言来检索信息,而文件是“扁平的”文件,通常以文本流的形式出现。
Most often when working with files you’ll read from or write to them. When you want to read the contents of a file you first have to open it, then read as much of the contents as you want, then close the file when you’re finished. When writing to a file, it also needs to be opened (or perhaps created if it doesn’t already exist), then you write your data to it, and close the file when you have finished. In PHP5 there are some built-in wrapper functions that handle the opening and closing automatically for you, but it still happens under the hood.
通常,在处理文件时,您会对其进行读取或写入。 当您想读取文件的内容时,首先必须打开它,然后根据需要读取尽可能多的内容,然后在完成后关闭文件。 写入文件时,还需要将其打开(或者如果尚不存在则创建),然后将数据写入其中,并在完成后关闭文件。 在PHP5中,有一些内置的包装器函数可以自动为您处理打开和关闭操作,但仍然可以在后台进行。
You may also find it useful to find out more about the file by examining its attributes before you start working with it. For example, does the file exist? When was it last updated? When was it created?
您可能还会发现,在开始使用文件之前,通过检查文件的属性来查找有关文件的更多信息很有用。 例如,文件是否存在? 上次更新时间是什么时候? 它是何时创建的?
PHP provides a range of functions which allow you to work with files, and in this article I’ll demonstrate some of them for you.
PHP提供了一系列允许您使用文件的功能,在本文中,我将为您演示其中的一些功能。
文件属性 (File Attributes)
File attributes are the properties of a file, for example its size, the last time it was accessed, its owner, etc. Let’s look at how you find out more about the files you’re working with.
文件属性是文件的属性,例如文件的大小,上次访问的时间,文件的所有者等。让我们看一下如何查找有关正在使用的文件的更多信息。
文件大小 (File Size)
The function filesize()
retrieves the size of the file in bytes.
函数filesize()
检索filesize()
以字节为单位)。
<?php
$f = "C:\Windows\win.ini";
$size = filesize($f);
echo $f . " is " . $size . " bytes.";
When executed, the example code displays:
执行后,示例代码将显示:
C:Windowswin.ini is 510 bytes.
The use of a file on a Windows system here highlights an important point; because the backslash has special meaning as an escape character in strings, you’ll need to escape it by adding another backslash.
在Windows系统上使用文件突出了重点。 因为反斜杠作为字符串中的转义字符具有特殊含义,所以您需要通过添加另一个反斜杠来对其进行转义。
If the file doesn’t exist, the filesize()
function will return false and emit an E_WARNING
, so it’s better to check first whether the file exists using the function file_exists()
:
如果文件不存在, filesize()
函数将返回false并发出E_WARNING
,因此最好使用函数file_exists()
首先检查文件是否存在:
<?php
$f = "C:\Windows\win.ini";
if (file_exists($f)) {
$size = filesize($f);
echo $f . " is " . $size . " bytes.";
}
else {
echo $f . " does not exist.";
}
In fact many of the functions presented in this section have the same behavior, i.e., emit warnings. I’ve not included a check with file_exists()
in the rest of my examples for the sake of brevity, but you’ll want to use it when you write your own code.
实际上,本节中介绍的许多功能具有相同的行为,即发出警告。 为了简洁起见,在我的其余示例中,我并未在file_exists()
中包含检查file_exists()
,但是您在编写自己的代码时会希望使用它。
文件历史 (File History)
To determine when a file was last accessed, modified, or changed, you can use the following functions respectively: fileatime()
, filemtime()
, and filectime()
.
要确定文件的最后访问,修改或更改时间,可以分别使用以下函数: fileatime()
, filemtime()
和filemtime()
filectime()
。
<?php
$dateFormat = "D d M Y g:i A";
$atime = fileatime($f);
$mtime = filemtime($f);
$ctime = filectime($f);
echo $f . " was accessed on " . date($dateFormat, $atime) . ".<br>";
echo $f . " was modified on " . date($dateFormat, $mtime) . ".<br>";
echo $f . " was changed on " . date($dateFormat, $ctime) . ".";
The code here retrieves the timestamp of the last access, modify, and change dates and displays them,
此处的代码检索上次访问的时间戳,修改和更改日期并显示它们,
C:Windowswin.ini was accessed on Tue 14 Jul 2009 4:34 AM.
C:Windowswin.ini was modified on Fri 08 Jul 2011 2:03 PM.
C:Windowswin.ini was changed on Tue 14 Jul 2009 4:34 AM.
To clarify, filemtime()
returns the time when the contents of the file was last modified, and filectime()
returns the time when information associated with the file, such as access permissions or file ownership, was changed.
为了澄清, filemtime()
返回时,文件的内容被最后一次修改的时间,和filectime()
返回时与该文件相关的信息,如访问权限或文件所有权时,被改变。
The date()
function was used to format the Unix timestamp returned by the file*time()
functions. Refer to the documentation for the date()
function for more formatting options.
date()
函数用于格式化file*time()
函数返回的Unix时间戳。 有关更多格式选项,请参考date()
函数的文档 。
档案权限 (File Permissions)
Before working with a file you may want to check whether it is readable or writeable to the process. For this you’ll use the functions is_readable()
and is_writeable()
:
在处理文件之前,您可能需要检查该文件对于该过程是可读还是可写的。 为此,您将使用is_readable()
和is_writeable()
函数:
<?php
echo $f . (is_readable($f) ? " is" : " is not") . " readable.<br>";
echo $f . (is_writable($f) ? " is" : " is not") . " writeable.";
Both functions return a Boolean value whether the operation can be performed on the file. Using the ternary operator you can tailor the display to state whether the file is or is not accessible as appropriate.
无论是否可以对文件执行操作,这两个函数均返回布尔值。 使用三元运算符,您可以定制显示以指出文件是否可以访问。
C:Windowswin.ini is readable.
C:Windowswin.ini is not writeable.
是否归档? (File or Not?)
To make absolutely sure that you’re dealing with a file you can use the is_file()
function. is_dir()
is the counterpart to check if it is a directory.
要绝对确保要处理文件,可以使用is_file()
函数。 is_dir()
是检查其是否为目录的副本。
<?php
echo $f . (is_file($f) ? " is" : " is not") . " a file.<br>";
echo $f . (is_dir($f) ? " is" : " is not") . " a directory.";
The example code outputs:
示例代码输出:
C:Windowswin.ini is a file.
C:Windowswin.ini is not a directory.
读取文件 (Reading Files)
The previous section showed how you can find out a good deal about the files you’re working with before you start reading or writing to them. Now let’s look at how you can read the contents of a file.
上一节介绍了如何在开始读取或写入文件之前找到大量有关正在使用的文件的信息。 现在让我们看一下如何读取文件内容。
The convenience function file_get_contents()
will read the entire contents of a file into a variable without the need to open or close the file yourself. This is handy when the file is relatively small, as you wouldn’t want to read in 1GB of data into memory all at once!
便捷函数file_get_contents()
会将文件的全部内容读入变量,而无需自己打开或关闭文件。 当文件相对较小时,这非常方便,因为您不想一次将1GB的数据全部读取到内存中!
<?php
$f = "c:\windows\win.ini";
$f1 = file_get_contents($f);
echo $f1;
For larger files, or just depending on the needs of your script, it’s may be wiser to handle the details yourself. This is because once a file is opened you can seek to a specific offset within it and read as little or as much data as you want at a time.
对于较大的文件,或仅取决于脚本的需求,最好自己处理这些细节。 这是因为一旦打开文件,您就可以查找其中的特定偏移量,并一次读取所需数量的数据。
The function fopen()
is used to open the file:
函数fopen()
用于打开文件:
<?php
$f = "c:\windows\win.ini";
$f1 = fopen($f, "r");
Using the function fopen()
, two arguments are needed – the file I want to open and the mode, which in this case is “r” for read. The function returns a handle or stream to the file, stored in the variable $f1
, which you use in all subsequent commands when working with the file.
使用函数fopen()
,需要两个参数–我要打开的文件和模式,在这种情况下为“ r”以供读取。 该函数将文件的句柄或流返回到文件中,该句柄或流存储在变量$f1
,您在处理文件时在所有后续命令中使用该句柄或流。
The most common mode values are:
最常见的模式值为:
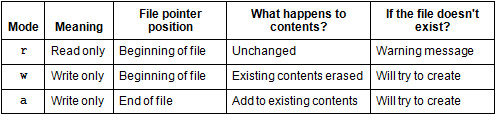
For other values, refer to the list in PHP’s fopen()
page.
有关其他值,请参考PHP的fopen()
页面中的列表。
To read from the opened file one line at a time, use the function fgets()
:
要一次从打开的文件中读取一行,请使用函数fgets()
:
<?php
$f = "c:\windows\win.ini";
$f1 = fopen($f, "r");
do {
echo fgets($f1) . "<br>";
}
while (!feof($f1));
fclose($f1);
Using a do
–while
loop is a good option because you may not know in advance how many lines are in the file. The function feof()
checks whether the file has reached its end – the loop continues until the end of file condition is reached.
使用do
– while
循环是一个不错的选择,因为您可能事先不知道文件中有多少行。 feof()
函数检查文件是否已到达末尾–循环继续进行,直到达到文件末尾条件为止。
To tidy up after I’ve finished reading the file, the function fclose()
is used to close the file.
为了在我读完文件后整理一下,使用函数fclose()
关闭文件。
写文件 (Writing Files)
Two commonly used modes when writing to a file using the function fwrite()
are “w” and “a” – “w” indicates you want to write to the file but it will remove any of the existing file contents beforehand, while “a” means that you will append any new data to what already exists in the file. You need to be sure you are using the correct option!
使用功能fwrite()
写入文件时,两种常用模式是“ w”和“ a” –“ w”表示您要写入文件,但是它将预先删除任何现有文件内容,而“ a” ”表示您将任何新数据追加到文件中已存在的数据上。 您需要确保使用正确的选项!
In this example I’ll use “a” to append:
在此示例中,我将使用“ a”附加:
<?php
$file = "add_emp.txt";
$f1 = fopen($file, "a");
$output = "banana" . PHP_EOL;
fwrite($f1, $output);
$output = "cheese" . PHP_EOL;
fwrite($f1, $output);
fclose($f1);
First the file name is assigned to a variable, then the file is opened in mode “a” for append. The data to be written is assigned to a variable, $output
, and fwrite()
adds the data to the file. The process is repeated to add another line, then the file is closed using fclose()
. The pre-defined constant PHP_EOL
adds the newline character specific to the platform PHP is running on.
首先,将文件名分配给变量,然后以“ a”模式打开文件以进行追加。 将要写入的数据分配给变量$output
,并且fwrite()
将数据添加到文件中。 重复此过程以添加另一行,然后使用fclose()
关闭文件。 预定义常量PHP_EOL
添加了特定于PHP运行平台的换行符。
The file contents after executing the above code should look like this:
执行以上代码后,文件内容应如下所示:
banana
cheese
The convenience function file_put_contents()
can also write to a file. It accepts the file name, the data to be written to the file, and the constant FILE_APPEND
if it should append the data (it will overwrite the file’s contents by default).
便捷函数file_put_contents()
也可以写入文件。 它接受文件名,要写入文件的数据以及常量FILE_APPEND
如果它应该附加数据)(默认情况下,它将覆盖文件的内容)。
Here’s the same example as above, but this time using file_put_contents()
:
这是与上面相同的示例,但是这次使用file_put_contents()
:
<?php
$file = "add_emp.txt";
file_put_contents($file, "banana" . PHP_EOL);
file_put_contents($file, "cheese" . PHP_EOL, FILE_APPEND);
使用CSV文件 (Working with CSV Files)
CSV stands for comma separated variable and indicates the file contains data delimited with a comma. Each line is a record, and each record is made up of fields, very much like a spreadsheet. In fact, software such as Excel provides the means to save files in this format. Here is an example as displayed in Excel 2007 (which doesn’t show the commas):
CSV代表逗号分隔的变量,表示文件包含用逗号定界的数据。 每行都是一条记录,每条记录都由字段组成,非常类似于电子表格。 实际上,诸如Excel之类的软件提供了以这种格式保存文件的方法。 这是在Excel 2007中显示的示例(不显示逗号):
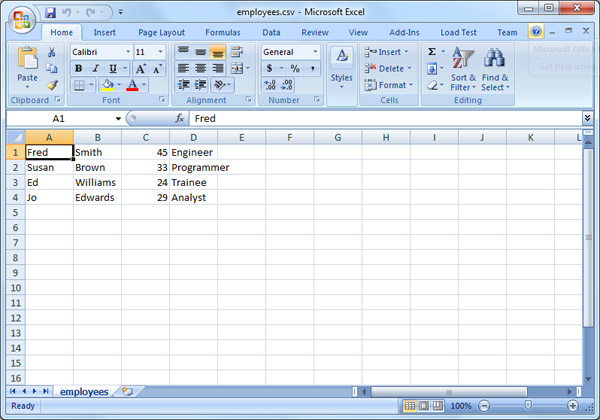
There are 4 lines of data, or records, in the file containing first name, last name, age and job title. To read the file and extract the individual fields, start by opening the file in read mode:
文件中有4行数据或记录,其中包含名字,姓氏,年龄和职务。 要读取文件并提取各个字段,请首先以读取模式打开文件:
<?php
$file = "employees.csv";
$f = fopen($file, "r");
Now I need to use a function specifically to read the CSV formatted file, fgetcsv()
; a while loop is used to cater for the fact that the number of records is expected to vary:
现在,我需要使用专门用于读取CSV格式文件的函数fgetcsv()
; 使用while循环来满足以下事实:记录数预计会有所变化:
<?php
$file = "employees.csv";
$f = fopen($file, "r");
while ($record = fgetcsv($f)) {
foreach($record as $field) {
echo $field . "<br>";
}
}
fclose($f);
This will produce output with a field on each line:
这将在每行上产生一个带有字段的输出:
Fred
Smith
45
Engineer
Susan
Brown
33
Programmer
Ed
Williams
24
Trainee
Jo
Edwards
29
Analyst
Let’s examine the loop. First, fgetcsv()
has one argument only – the file handle; the comma delimiter is assumed. fgetcsv()
returns data in an array which is then processed using a foreach
loop. When all records have been read the file is closed using fclose()
.
让我们研究一下循环。 首先, fgetcsv()
仅具有一个参数–文件句柄; 假定使用逗号分隔符。 fgetcsv()
返回数组中的数据,然后使用foreach
循环对其进行处理。 读取所有记录后,使用fclose()
关闭文件。
Let’s now look at how you can update or write to a CSV file, this time using the function fputcsv()
. Because I am using the same file that I used above, I will open it in append mode, and the data to be added to the file has been defined in an array.
现在让我们看一下如何使用函数fputcsv()
来更新或写入CSV文件。 因为我使用的是与上面相同的文件,所以将以附加模式打开它,并且要添加到文件中的数据已在数组中定义。
<?php
$file = "employees.csv";
$f = fopen($file, "a");
$newFields = array(
array('Tom', 'Jones', 36, 'Accountant'),
array('Freda', 'Williams', 45, 'Analyst'),
array('Brenda', 'Collins', 34, 'Engineer'));
foreach($newFields as $fields) {
fputcsv($f, $fields);
}
fclose($f);
The contents of the file as displayed in Excel now look like this:
现在,在Excel中显示的文件内容如下所示:
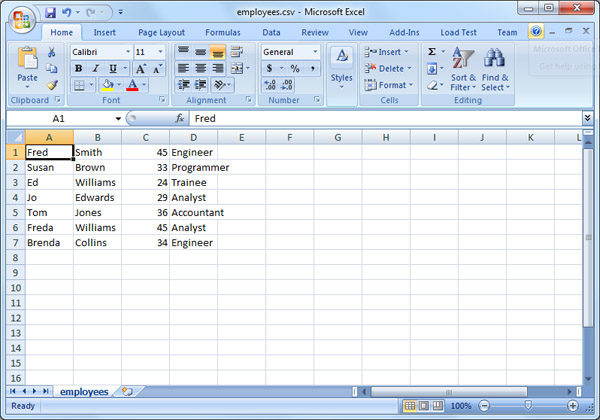
摘要 (Summary)
This article has given you an introduction into working with files and shown how you can find information about files, read from and write to files. There are many other functions you can use when working with files – this article has covered some of the most commonly used. You’ll find more information on PHP’s file system functions page.
本文向您介绍了如何使用文件,并说明了如何查找有关文件的信息,如何读取和写入文件。 处理文件时,您还可以使用其他许多功能-本文介绍了一些最常用的功能。 您可以在PHP的文件系统功能页面上找到更多信息。
Image via Marco Rullkoetter / Shutterstock
图片来自Marco Rullkoetter / Shutterstock
vue文件中怎么使用php