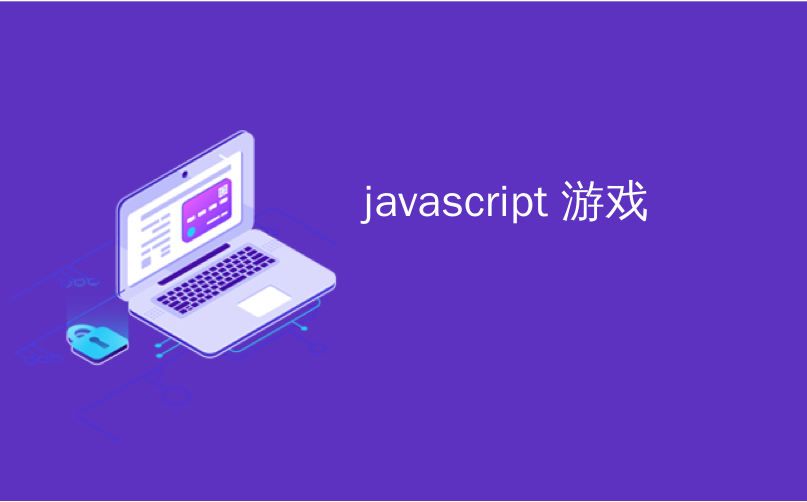
javascript 游戏
In this tutorial, I will tell you how to build a basic tic tac toe game using HTML, CSS and JavaScript.
在本教程中,我将告诉您如何使用HTML,CSS和JavaScript构建基本的井字游戏。
Most of the people know that when we were kids we used to play this game on paper. We played it many times till we win.
大多数人都知道,我们小时候曾经在纸上玩这种游戏。 我们玩了很多次,直到赢了。
Those who don’t know about this game let me give you little overview. In this game there are two players, the player who makes three of their marks in row and column or one of the two diagonals wins the game. When the board fills up and no combination making then the game is in draw state.
那些对这款游戏不了解的人让我给您一点概述。 在此游戏中,有两个玩家,在行列中赢得三个标记或两个对角线之一赢得比赛的玩家。 当棋盘填满并且没有进行组合时,游戏就处于抽签状态。
There are some rules that we are going to define here is that as we know the primary thing is move, here the player cannot undo that move. By clicking on the box he can make a move as soon as the move is done, the game proceeded to give a chance to another player.
我们要在这里定义一些规则,因为我们知道主要是移动,此处玩家无法撤消该移动。 通过单击该框,他可以在移动完成后立即进行移动,游戏继续为其他玩家提供机会。
At each move, it will show whose player moves it is either is A or it is B
每次移动时,都会显示是谁或谁是B
When the game ends it displays three outcomes:
游戏结束时,它将显示三个结果:
- winner player one 冠军玩家一
- winner player two 冠军选手二
- draw 画
It also displays the replay button if a tie happens.
如果出现平局,它还会显示重播按钮。
JavaScript井字游戏示例 (JavaScript Tic Tac Toe Game Example)
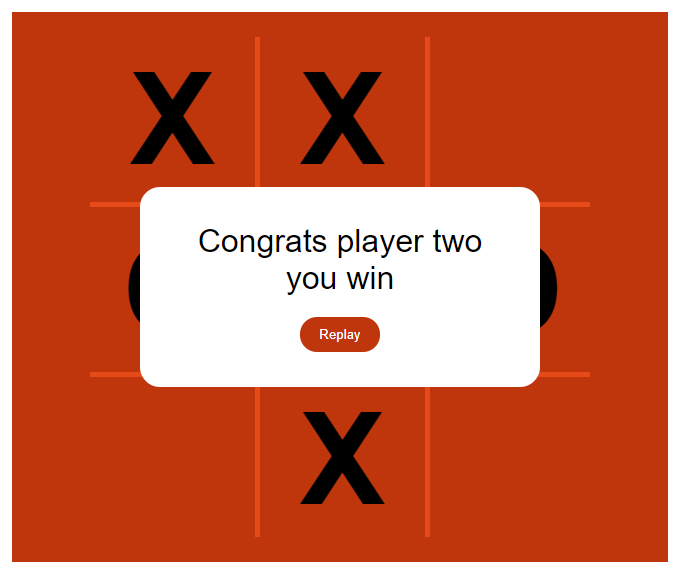
Its programming is not that simple as it looks. In this js program, we track each move for the next players move. This is quite complex when we start coding. I am sharing my simple code so that you can understand the game easily. So basically we create 3 files here or we can do all code in one file which includes all html, css and javascript.
它的编程并不像看起来那么简单。 在此js程序中,我们跟踪每个玩家的下一个动作。 当我们开始编码时,这是相当复杂的。 我正在分享我的简单代码,以便您可以轻松理解游戏。 因此,基本上我们在这里创建了3个文件,或者我们可以在一个文件中执行所有代码,其中包括所有html,css和javascript。
So firstly we make a design or UI part then start work on its functionality. You can create this by using plain javascript.
因此,首先我们制作一个设计或UI部件,然后开始对其功能进行工作。 您可以使用普通的javascript创建此文件。
So we load code on the loading of HTML document.
因此我们在加载HTML文档时加载代码。
Before writing code, we also have to check what are the winning conditions.
在编写代码之前,我们还必须检查获胜条件。
Player 1 plays when the move is equal to 1, 3, 5, 7 and 9. Player 2 plays when the move is equal to 2, 4, 6 and 8. So, Player 1 plays when move is an odd number. Here we can write the logic this way, if ((count%2)==1), then it is player 1’s turn, otherwise it is player 2’s turn. When any player presses on a blank space, that respective player places either an X or O on the playing board.
当移动等于1、3、5、7和9时,玩家1会玩。当移动等于2、4、6和8时,玩家2会玩。因此,当移动为奇数时,玩家1会玩。 在这里,我们可以这样写逻辑,如果((count%2)== 1),则为玩家1的回合,否则为玩家2的回合。 当任何玩家按下空白处时,该玩家将X或O放在游戏板上。
In the code firstly we write the winning case scenarios at which numbers he/she can win the game. Then we make a function for each move player is playing, in the function we check that players wins or not by that move. We also make a function if no one wins the game then they can again play that game.
首先,在代码中,我们写出了获胜案例,即他/她可以赢得比赛的数字。 然后,我们为玩家正在玩的每个棋步创建一个函数,在该函数中,我们检查棋手是否获胜。 如果没有人赢得比赛,我们也会执行一项功能,然后他们可以再次玩该游戏。
Here is code to make the game.
这是制作游戏的代码。
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
<title>Tic Tac Toe Game</title>
<style>
*{
box-sizing: border-box;
font-family: sans-serif;
}
body{
margin: 0;
padding: 0;
width: 100%;
height: 100vh;
display: flex;
justify-content: center;
align-items: center;
background-color: #BF360C;
}
.container{
width: 500px;
height: 500px;
display: grid;
background-color: #E64A19;
grid-gap: 5px;
grid-template-columns: 33% 33% 33%;
grid-auto-rows: 33% 33% 33%;
}
.card{
position: relative;
background-color: #BF360C;
cursor: pointer;
}
.card::before{
position: absolute;
top: 0; right: 0; bottom: 0; left: 0;
display: flex;
justify-content: center;
align-items: center;
font-weight: bold;
font-size: 8rem;
}
.card.x::before{
content: "X";
}
.card.o::before{
content: "O";
}
.winner{
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
position: fixed;
width: 400px;
height: 200px;
padding: 20px 40px;
background-color: #fff;
font-size: 2rem;
border-radius: 20px;
text-align: center;
animation: animate .5s linear;
}
@keyframes animate{
from{
opacity: 0;
}
to{
opacity: 1;
}
}
.winner button{
margin-top: 20px;
width: 80px;
height: 35px;
line-height: 35px;
padding: 0;
border: 0;
outline: 0;
border-radius: 20px;
cursor: pointer;
color: #fff;
background-color: #BF360C;
}
</style>
</head>
<body>
<div class="container">
<div class="card" data-index="1"></div>
<div class="card" data-index="2"></div>
<div class="card" data-index="3"></div>
<div class="card" data-index="4"></div>
<div class="card" data-index="5"></div>
<div class="card" data-index="6"></div>
<div class="card" data-index="7"></div>
<div class="card" data-index="8"></div>
<div class="card" data-index="9"></div>
</div>
</body>
<script>
const cards = Array.from(document.querySelectorAll(".card"));
const winner = [[1,2,3],[4,5,6],[7,8,9],[1,5,9],[3,5,7],[1,4,7],[2,5,8],[3,6,9]];
let firstPlayer = [], secondPlayer = [], count = 0;
/*******************************************************/
function check(array){
let finalResult = false;
for(let item of winner){
let res = item.every(val => array.indexOf(val) !== -1);
if(res){
finalResult = true;
break;
}
}
return finalResult;
}
/*******************************************************/
function winnerpleyr(p){
const modal = document.createElement("div");
const player = document.createTextNode(p);
const replay = document.createElement("button");
modal.classList.add("winner");
modal.appendChild(player);
replay.appendChild(document.createTextNode("Replay"));
// replay.setAttribute("onclick","rep();");
replay.onclick = function() { rep() };
modal.appendChild(replay);
document.body.appendChild(modal);
}
/*******************************************************/
function draw(){
if(this.classList == "card"){
count++;
if(count%2 !== 0){
this.classList.add("x");
firstPlayer.push(Number(this.dataset.index));
if(check(firstPlayer)){
winnerpleyr("Congrats player one you win");
return true;
}
} else{
this.classList.add("o");
secondPlayer.push(Number(this.dataset.index));
if(check(secondPlayer)){
winnerpleyr("Congrats player two you win");
return true;
}
}
if(count === 9){
winnerpleyr("Draw");
}
}
}
/*******************************************************/
function rep(){
const w = document.querySelector(".winner");
// cards.forEach(card => card.classList = "card");
firstPlayer = [];
secondPlayer = [];
count = 0;
w.remove();
[].forEach.call(cards, function(el) {
el.classList.remove("x");
el.classList.remove("o");
});
}
/*******************************************************/
cards.forEach(card => card.addEventListener("click", draw));
</script>
</html>
You can play the game given below to test how it works.
您可以玩下面给出的游戏,以测试其工作方式。
Comment down below if you have any queries related to above JavaScript Tic Tac Toe game.
如果您对上述JavaScript Tic Tac Toe游戏有任何疑问,请在下面注释。
翻译自: https://www.thecrazyprogrammer.com/2019/09/javascript-tic-tac-toe-game.html
javascript 游戏