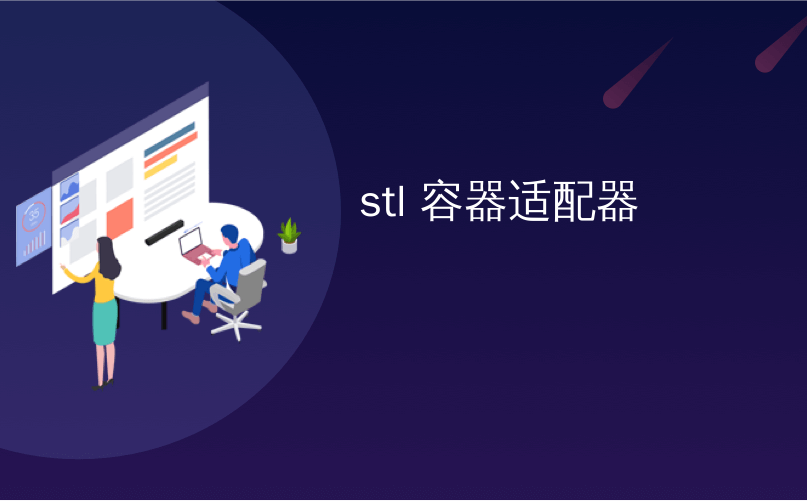
stl 容器适配器
In this tutorial you will learn about STL stack container adaptor in C++ i.e. std::stack and all functions which it provides.
在本教程中,您将学习C ++中的STL堆栈容器适配器,即std :: stack及其提供的所有功能。
std::stack is a container adaptor. We know that container adaptors are not containers. They provide specific interfaces. Elements manipulated in container adaptors by encapsulated functions of specific classes.
std :: stack是容器适配器。 我们知道容器适配器不是容器。 它们提供特定的接口。 通过特定类的封装函数在容器适配器中操作的元素。
Stack operates in Last in First out (LIFO) type of arrangement. Always elements will be inserted and also deleted at same side of the stack.
堆栈采用后进先出(LIFO)类型的安排。 总是在堆栈的同一侧插入和删除元素。
Working with direct operations on stack/queue and other container adaptors, will so much useful in competitive programming. It saves time and also encapsulated functions of object implemented in best complexity way. When program size too large, instead of writing entire code if we use direct functions from library that gives unambiguity while working.
在堆栈/队列和其他容器适配器上直接操作,在竞争性编程中将非常有用。 它节省了时间,并且还以最佳复杂性方式实现了对象的封装功能。 当程序太大时,如果我们使用库中的直接函数而不是编写整个代码,而直接函数会在工作时提供明确的信息。
C ++ STL堆栈容器适配器– std :: stack (C++ STL Stack Container Adaptor – std::stack)
To work with stl container, we first need to include stack header file.
要使用stl容器,我们首先需要包括堆栈头文件。
#include<stack>
#include <stack>
The functions associated with stack are:
与堆栈相关的功能是:
push(element): Inserting elements into stack is called “push” operation.
push(element):将元素插入堆栈称为“推”操作。
pop(element): Removing elements into stack is called “pop” operation.
pop(element):将元素移到堆栈中称为“ pop”操作。
top(element): Displays the top element of the stack.
top(element):显示堆栈的顶部元素。
size(element): Returns the size of the stack
size(element):返回堆栈的大小
empty(): This is Boolean operation which returns whether the stack is empty or not.
empty():这是布尔运算,它返回堆栈是否为空。
Program to show the above functions on stack:
程序以在堆栈上显示上述功能:
#include<iostream>
#include<stack>
using namespace std;
int main()
{
stack <int> stk; // declearing stack
for (int i=0; i<5; i++){
// pushing elements into stack
stk.push(i);
}
cout << "size of the stack is ";
cout << stk.size() << endl;
cout << "top of the stack is ";
cout << stk.top() << endl;
// to show all elements we should pop each time.
// Since we can only access top of the stack.
cout << "elements of the stack are " << endl ;
for (int i=0; i<5; i++){
cout << stk.top() << " ";
stk.pop(); // popping element after showing
}
cout << endl;
if (stk.empty() == 1) {
cout << "finally stack is empty " << endl;
}
else {
cout << "stack is not empty " << endl;
}
return 0;
}
Output
输出量
size of the stack is 5 top of the stack is 4 elements of the stack are 4 3 2 1 0 finally stack is empty
堆栈的大小是5堆栈的 顶部是堆栈的4个 元素是 4 3 2 1 0 最后堆栈是空的
One other operations is:
另一项操作是:
swap(): Swap function swaps the elements in one stack to other.
swap():交换函数将一个堆栈中的元素交换到另一个。
#include <iostream>
#include <stack>
using namespace std;
int main(){
stack <int> stk1, stk2;
for (int i=1; i<6; i++){
stk1.push(i+10);
}
cout << "elements 11, 12, 13, 14, 15 pushed into stack 1" << endl;
for (int i=1; i<6; i++){
stk2.push(i*10);
}
cout << "elements 10, 20, 30, 40, 50 pushed into stack 2" << endl;
cout << "doing swapping operation..... " << endl;
stk1.swap(stk2);
cout << "after swapping " << endl;
cout << "elements of stack 1 are " ;
for (int i=0; i<5; i++){
cout << stk1.top() << " ";
stk1.pop();
}
cout << endl;
cout << "elements of stack 2 are " ;
for (int i=0; i<5; i++){
cout << stk2.top() << " ";
stk2.pop();
}
return 0;
}
Output
输出量
elements 11, 12, 13, 14, 15 pushed into stack 1 elements 10, 20, 30, 40, 50 pushed into stack 2 doing swapping operation….. after swapping elements of stack 1 are 50 40 30 20 10 elements of stack 2 are 15 14 13 12 11
元素11、12、13、14、15推入堆栈1的 元素10、20、30、40、50推入堆栈2 进行交换操作….. 交换 堆栈1的元素 之后 是50 40 30 20 10 堆栈2的元素是15 14 13 12 11
Comment below if you have any queries related to above tutorial for stl stack or std::stack.
如果您对stl stack或std :: stack有任何与以上教程相关的查询,请在下面评论。
翻译自: https://www.thecrazyprogrammer.com/2017/09/stl-stack.html
stl 容器适配器