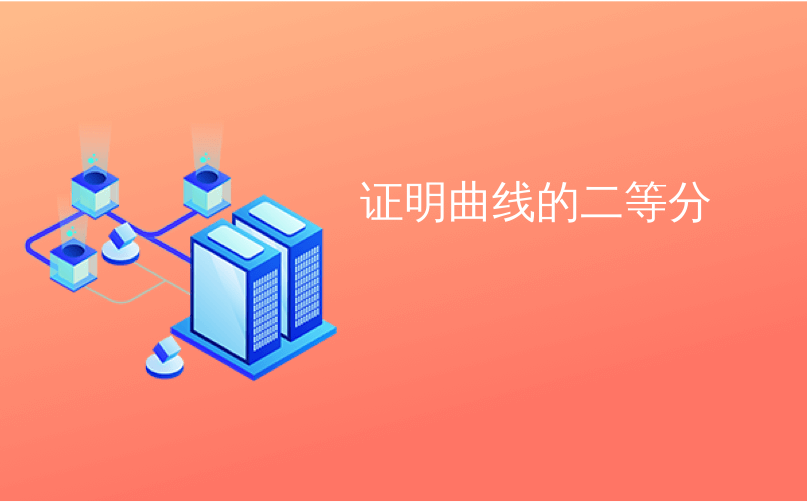
证明曲线的二等分
In this tutorial you will get program for bisection method in C and C++.
在本教程中,您将获得C和C ++中的二等分方法程序。
To find a root very accurately Bisection Method is used in Mathematics. Bisection method algorithm is very easy to program and it always converges which means it always finds root.
为了非常精确地找到根,数学中使用了二等分法。 二等分方法算法很容易编程,并且总是收敛,这意味着它总是可以找到根。
Bisection Method repeatedly bisects an interval and then selects a subinterval in which root lies. It is a very simple and robust method but slower than other methods.
二等分方法反复将一个二等分,然后选择一个根在其中的子间隔。 这是一种非常简单且健壮的方法,但比其他方法要慢。
It is also called Interval halving, binary search method and dichotomy method.
也称为间隔减半,二分搜索法和二分法。
Bisection Method calculates the root by first calculating the mid point of the given interval end points.
平分法通过首先计算计算根中点的给定间隔结束点的。
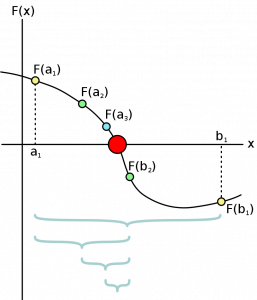
二等分法程序 (Bisection Method Procedure)
The input for the method is a continuous function f, an interval [a, b], and the function values f(a) and f(b). The function values are of opposite sign (there is at least one zero crossing within the interval). Each iteration performs these steps:
该方法的输入是一个连续函数f,一个区间[a,b]以及函数值f(a)和f(b)。 函数值的符号相反(在间隔内至少有一个零交叉)。 每次迭代执行以下步骤:
1. Calculate the midpoint c = (a + b)/2
1.计算中点c =(a + b)/ 2
2. Calculate the function value at the midpoint, function(c).
2.计算中点函数(c)的函数值。
3. If convergence is satisfactory (that is, a – c is sufficiently small, or f(c) is sufficiently small), return c and stop iterating.
3.如果收敛令人满意(即a – c足够小,或f(c)足够小),则返回c并停止迭代。
4. Examine the sign of f(c) and replace either (a, f(a)) or (b, f(b)) with (c, f(c)) so that there is a zero crossing within the new interval.
4.检查f(c)的符号,并将(a,f(a))或(b,f(b))替换为(c,f(c)),以便在新间隔内过零。
利弊 (Pros and Cons)
Advantage of the bisection method is that it is guaranteed to be converged and very easy to implement.
二等分方法的优点是可以保证收敛并且非常容易实现。
Disadvantage of bisection method is that it cannot detect multiple roots and is slower compared to other methods of calculating the roots.
对分法的缺点是它不能检测多个根,并且比其他计算根的方法要慢。
C语言二等分法程序 (Program for Bisection Method in C)
#include<stdio.h>
//function used is x^3-2x^2+3
double func(double x)
{
return x*x*x - 2*x*x + 3;
}
double e=0.01;
double c;
void bisection(double a,double b)
{
if(func(a) * func(b) >= 0)
{
printf("Incorrect a and b");
return;
}
c = a;
while ((b-a) >= e)
{
c = (a+b)/2;
if (func(c) == 0.0){
printf("Root = %lf\n",c);
break;
}
else if (func(c)*func(a) < 0){
printf("Root = %lf\n",c);
b = c;
}
else{
printf("Root = %lf\n",c);
a = c;
}
}
}
int main()
{
double a,b;
a=-10;
b=20;
printf("The function used is x^3-2x^2+3\n");
printf("a = %lf\n",a);
printf("b = %lf\n",b);
bisection(a,b);
printf("\n");
printf("Accurate Root calculated is = %lf\n",c);
return 0;
}
Output
输出量
a = -10.000000 b = 20.000000 Root = 5.000000 Root = -2.500000 Root = 1.250000 Root = -0.625000 Root = -1.562500 Root = -1.093750 Root = -0.859375 Root = -0.976563 Root = -1.035156 Root = -1.005859 Root = -0.991211 Root = -0.998535
a = -10.000000 b = 20.000000 根= 5.000000 根= -2.500000 根= 1.250000 根= -0.625000 根= -1.562500 根= -1.093750 根= -0.859375 根= -0.976563 根= -1.035156 根= -1.005859 根= -0.991211 根= -0.998535
Accurate Root calculated is = -0.998535
计算出的精确根为-0.998535
C ++中的平分法程序 (Program for Bisection Method in C++)
#include<iostream>
using namespace std;
//function used is x^3-2x^2+3
double func(double x)
{
return x*x*x - 2*x*x + 3;
}
double e=0.01;
double c;
void bisection(double a,double b)
{
if(func(a) * func(b) >= 0)
{
cout<<"Incorrect a and b";
return;
}
c = a;
while ((b-a) >= e)
{
c = (a+b)/2;
if (func(c) == 0.0){
cout << "Root = " << c<<endl;
break;
}
else if (func(c)*func(a) < 0){
cout << "Root = " << c<<endl;
b = c;
}
else{
cout << "Root = " << c<<endl;
a = c;
}
}
}
int main()
{
double a,b;
a=-10;
b=20;
cout<<"The function used is x^3-2x^2+3\n";
cout<<"a = "<<a<<endl;
cout<<"b = "<<b<<endl;
bisection(a,b);
cout<<"\n";
cout<<"Accurate Root calculated is = "<<c<<endl;
return 0;
}
This article is submitted by Rahul Maheshwari. You can connect with him on facebook.
本文由Rahul Maheshwari提交。 您可以在Facebook上与他建立联系。
Comment below if you have any queries regarding above program for bisection method in C and C++.
如果您对上述程序在C和C ++中的二分法有任何疑问,请在下面评论。
翻译自: https://www.thecrazyprogrammer.com/2017/04/bisection-method.html
证明曲线的二等分