We have previously looked at the Carbon package by Brian Nesbitt as well as all the functionality it adds to PHP's DateTime class.
我们之前已经看过Brian Nesbitt的Carbon软件包以及它添加到PHP的DateTime类的所有功能。
In this article, we will take a look at how Laravel takes advantage of this package.
在本文中,我们将研究Laravel如何利用此软件包。
介绍 ( Introduction )
As already mentioned, The carbon class does not rebuild PHP's DateTime
class from scratch, it builds upon it.
如前所述,Carbon类不会从头开始重建PHP的DateTime
类,而是在此基础上重建的。
<?php
namespace Carbon;
class Carbon extends \DateTime
{
// code here
}
This means that you can access the default functionality of PHP's DateTime class on top of the awesomeness that is Carbon.
这意味着您可以在令人赞叹的Carbon之上访问PHP的DateTime类的默认功能。
Laravel already includes the Carbon class by default so we do not need to install it separately. To get started with Carbon in Laravel, simply create a new project using the laravel
command.
Laravel默认已经包含Carbon类,因此我们不需要单独安装它。 要开始使用Laravel中的Carbon,只需使用laravel
命令创建一个新项目。
$ laravel new scotch-dates
Laravel的碳约会 ( Carbon Dating in Laravel )
See what I did there? Turns out Brian Nesbitt had the same idea in mind while creating the Carbon package.
看看我在那里做什么? 事实证明, 布莱恩·内斯比特 ( Brian Nesbitt)在创建Carbon套件时怀有相同的想法。
Now by default, if the timestamps
variable in a Laravel model class is not explicitly set to false, then it is expected that it's corresponding table should have the created_at
and updated_at
columns.
现在默认情况下,如果未将Laravel模型类中的timestamps
变量未显式设置为false,则预期其对应的表应具有created_at
和updated_at
列。
We can however go ahead and add our own date columns such as activated_at
, dob
or any other depending on the type of application or the nature of the Laravel model we are working on.
不过,我们可以继续添加我们自己的日期列,比如activated_at
, dob
或任何其他根据应用的类型,或者我们正在处理的Laravel模式的本质。
But how does laravel know that these fields should be cast to date?
但是laravel如何知道这些字段应该强制转换为最新的?
Simple. Add all the date fields to the protected dates
variable in the model class.
简单。 将所有日期字段添加到模型类中的受保护dates
变量中。
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
/**
* The attributes that should be mutated to dates.
*
* @var array
*/
protected $dates = [
'created_at',
'updated_at',
'activated_at',
'dob'
];
}
建立 ( Setup )
In our scotch-dates application that we just created, we already have a our first migration setup for the user's table. We will need to have some user records to work with, so let's seed some data in our database with the factory helper method on tinker.
在我们刚刚创建的苏格兰日期应用程序中,我们已经为用户的表建立了第一个迁移设置。 我们将需要使用一些用户记录,因此让我们使用tinker上的factory helper方法将一些数据播种到数据库中。
$ php artisan tinker>>> factory('App\User', 10)->create()
To get started, we'll go ahead and create a new UserController
class to get all the user's from the user's table into a view and add a /users
route.
首先,我们将继续创建一个新的UserController
类,以将用户表中的所有用户添加到视图中并添加/users
路由。
/routes/web.php
/routes/web.php
Route::get('/', function () {
return view('welcome');
});
Route::get('/users', 'UserController@users');
I have gone ahead to add a few helper variables courtesy of the Carbon class to give me access to a few dates such as now
, yesterday
, today
and tomorrow
.
我继续增加了Carbon类的一些帮助变量,使我可以了解一些日期,例如now
, yesterday
, today
和tomorrow
。
/app/Http/Controllers/UserController.php
/app/Http/Controllers/UserController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\User;
use Carbon\Carbon;
class UserController extends Controller
{
public function users()
{
return view('users', [
'users' => User::all(),
'now' => Carbon::now(),
'yesterday' => Carbon::yesterday(),
'today' => Carbon::today(),
'tomorrow' => Carbon::tomorrow()
]);
}
}
Let's play around with Carbon dates on the user's
view.
让我们在user's
视图上玩转Carbon日期。
显示绝对日期 ( Displaying Absolute Dates )
Having setup our user's details and passed them on to the users
view, we can now display each of the users with the following blade template.
设置好用户的详细信息并将其传递给users
视图后,我们现在可以使用以下刀片模板显示每个用户。
<table>
<tr>
<th>Name</th>
<th>Created</th>
</tr>
@foreach($users as $user)
<tr>
<td>{{ $user->name }}</td>
<td>{{ $user->created_at }}</td>
</tr>
@endforeach
</table>
With this, we should have the following.
有了这个,我们应该具备以下条件。
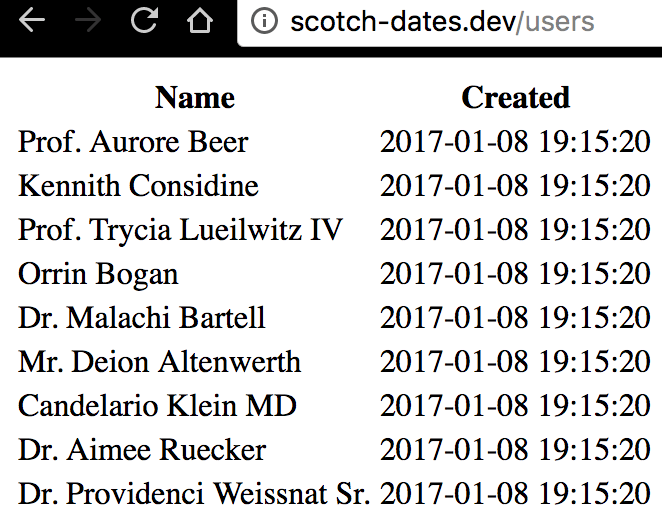
相对显示日期 ( Displaying Dates Relatively )
Displaying dates relatively is quite popular since it is easier for humans to read out a post as created 30 minutes ago as opposed to 2017-01-08 19:15:20.
相对显示日期非常受欢迎,因为相对于2017-01-08 19:15:20 ,人类更容易读出30分钟前创建的帖子 。
Let's play around with the Carbon class to see how we can display the dates relatively in different ways.
让我们玩转Carbon类,看看如何以不同的方式相对显示日期。
将过去的值与现在的默认值进行比较时: (When comparing a value in the past to default now:)
This comes in handy when you want to display a date in the past with reference to the current time. This would be something like:
当您想参考当前时间显示过去的日期时,这非常方便。 就像这样:
- A few seconds ago 几秒钟前
- 30 minutes ago 30分钟前
- 2 days ago 2天前
- 1 year ago 1年以前
To achieve this, we simply use the diffForHumans
method.
为此,我们只需使用diffForHumans
方法。
$user->created_at->diffForHumans()
// 1 hour ago
将将来的值与现在的默认值进行比较时: (When comparing a value in the future to default now:)
You'd probably want to use this in cases where you need to publish a post in the future or show an expiration date.
如果您以后需要发布帖子或显示有效日期,则可能需要使用此功能。
- 1 hour from now 从现在起1小时
- 5 months from now 从现在起5个月
$user->created_at->addDays(5)->diffForHumans()
//5 days from now
将过去的值与另一个值进行比较时: (When comparing a value in the past to another value:)
- 1 hour before 1小时前
- 5 months before 5个月前
$yesterday->diffForHumans($today)
//1 day before
将将来的值与另一个值进行比较时: (When comparing a value in the future to another value:)
- 1 hour after 1小时后
- 5 months after 5个月后
$tomorrow->diffForHumans($today)
//1 day after
有关差异的更多信息 ( More About Diffs )
While it may be nice to display to the user fully quallified diffs such as 2 hours ago, you may at times simply want to show the user the value without the text.
虽然最好向用户显示完全类似的差异(例如2小时前) ,但有时您可能只是想向用户显示没有文本的值。
This may come in handy where you have many comments coming in and the text is just too repetitive. diffInSeconds()
in particular can be used in cases where the difference in time between two entities, say, lap times, is of significance.
这可能会派上用场,因为您会收到很多评论,并且文本过于重复。 特别是在两个实体之间的时间差(例如圈速)很重要的情况下,可以使用diffInSeconds()
。
This can achieved by the diffInYears
, diffInMonths()
, diffInWeeks()
, diffInDays()
, diffInWeekdays()
, diffInWeekendDays()
diffInHours()
, diffInMinutes()
and diffInSeconds()
methods.
这可以通过diffInYears
, diffInMonths()
, diffInWeeks()
, diffInDays()
, diffInWeekdays()
, diffInWeekendDays()
diffInHours()
, diffInMinutes()
和diffInSeconds()
方法来实现。
$user->created_at->diffInHours(); //2
$user->created_at->diffInMinutes(); //134
$user->created_at->diffInSeconds(); //8082
The carbon class also come with methods that return the number of seconds since midnight or to the end of the day (midnight) which can be used to create a countdown, say, for a product sale.
carbon类还提供了一些方法,这些方法返回从午夜到一天结束(午夜)的秒数,这些秒数可用于创建倒计时,例如用于产品销售。
$now->secondsSinceMidnight() //77825
$now->secondsUntilEndOfDay() //8574
结论 ( Conclusion )
There's alot you can achieve with the Carbon class and sometimes you will not find out about a functionality that it provides until you need it.
Carbon类有很多可以实现的功能,有时直到您需要它时,才会发现它提供的功能。
Take a look at the documentation here. Happy Carbon dating!
在这里查看文档。 快乐碳约会!
翻译自: https://scotch.io/tutorials/quick-tip-display-dates-relatively-in-laravel