TypeScript and Visual Studio Code are two amazing products created by Microsoft, and - surprise surprise- they work amazing together! Let's take a look at how Visual Studio Code makes it a breeze to work with and configure TypeScript!
TypeScript和Visual Studio Code是Microsoft创造的两个令人惊叹的产品,而且-令人惊讶的是-它们一起工作令人惊奇! 让我们看一下Visual Studio Code如何使使用和配置TypeScript变得轻而易举!
TLDR (TLDR)
Install the TypeScript package globally and create a ".ts" file to get started. Visual Studio Code gives live TypeScript feedback to help you write better code, and provides intellisense when creating TypeScript configurations.
全局安装TypeScript软件包,并创建一个“ .ts”文件以开始使用。 Visual Studio Code提供实时TypeScript反馈以帮助您编写更好的代码,并在创建TypeScript配置时提供智能提示。
Check out Learn Visual Studio Code to learn everything you need to know about about the hottest editor in Web Development for just $10!
查看“ 学习Visual Studio Code” ,仅需10美元,即可了解有关Web开发中最热门的编辑器的所有知识。
什么是TypeScript ( What is TypeScript )
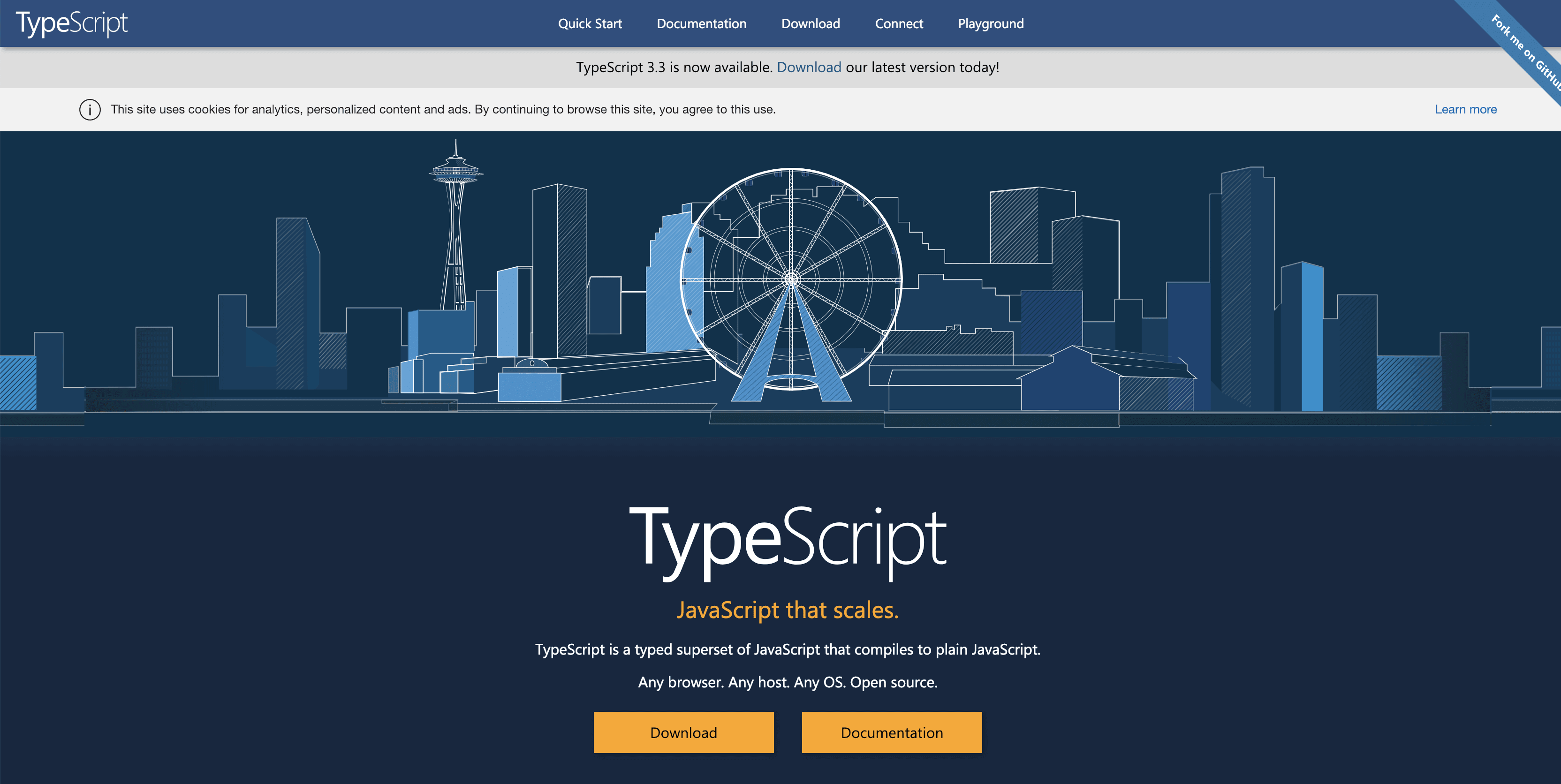
To be honest, JavaScript made me really uncomfortable for a while. Coming from a background in C# and Java, the lack of strong typing terrified me. The thought that I could pass anything to a function regardless of what it expected didn't feel right.
老实说,JavaScript使我一阵子真的不舒服。 来自C#和Java的背景,缺乏强类型输入使我感到恐惧。 不管我期望什么,我都可以将任何东西传递给函数的想法是不对的。
In comes TypeScript, a typed superset of JavaScript that compiles to plain JavaScript. Ok, a couple of key words here.
TypeScript是一种类型化JavaScript超集,可编译为普通JavaScript。 好的,这里有几个关键词。
- typed - You can define variable, parameter, and return data types 类型化 -您可以定义变量,参数和返回数据类型
- superset - TypeScript adds some additional features on top of JavaScript. All valid JavaScript is valid TypeScript, but not the other way around. 超集 -TypeScript在JavaScript之上添加了一些附加功能。 所有有效JavaScript都是有效的TypeScript,但反之则不是。
- *compiles to plain JavaScript *- TypeScript cannot be run by the browser. So available tooling takes care of compiling your TypeScript to JavaScript for the browser to understand. * 编译为普通JavaScript * -TypeScript无法由浏览器运行。 因此,可用的工具需要将您的TypeScript编译为JavaScript,以供浏览器理解。
TypeScript was created by Microsoft, who also created Visual Studio Code. As you might expect, they work pretty well together! Let's take a look.
TypeScript是由Microsoft创建的,他还创建了Visual Studio Code。 如您所料,它们可以很好地协作! 让我们来看看。
安装和编译TypeScript ( Installing and Compiling TypeScript )
The first step toward working with TypeScript is to install the package globally on your computer. install typescript package globally by running the following command.
使用TypeScript的第一步是在计算机上全局安装软件包。 通过运行以下命令全局安装Typescript软件包。
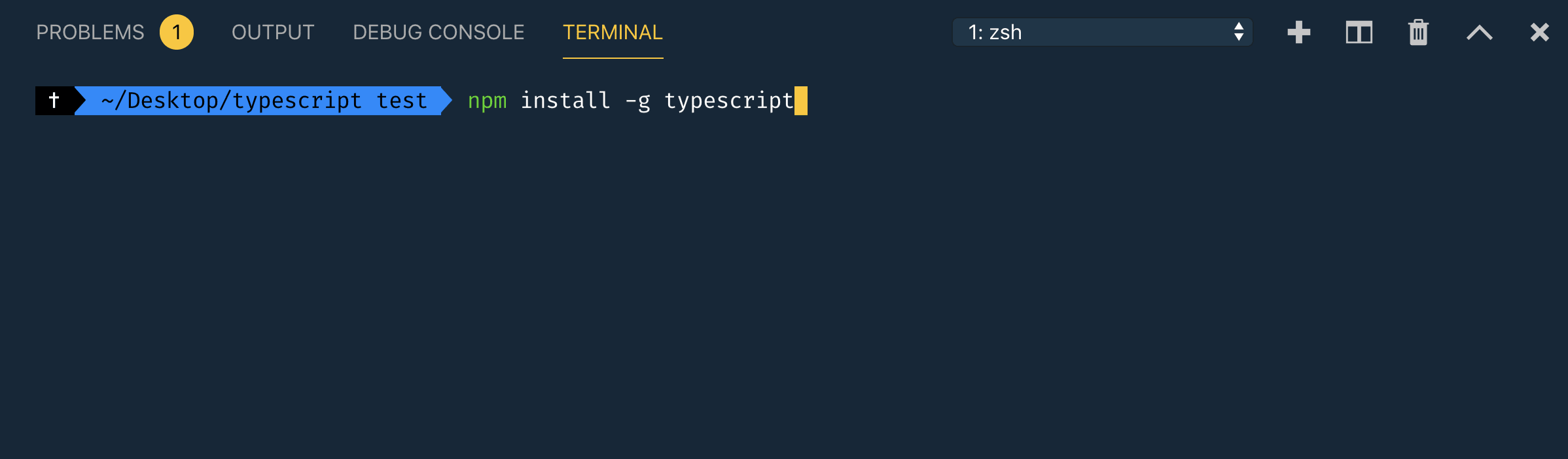
For now, you can add any valid JavaScript, but I'm going to start by creating a function that will print the first and last name from a person object. We'll build on this in a second.
现在,您可以添加任何有效JavaScript,但是我将首先创建一个函数,该函数将打印person对象的名字和姓氏。 我们将在此基础上建立。
export {};
function welcomePerson(person) {
console.log(`Hey ${person.firstName} ${person.lastName}`);
return `Hey ${person.firstName} ${person.lastName}`;
}
const james = {
firstName: "James",
lastName: "Quick"
};
welcomePerson(james);
The problem with the code above is that there is no restriction on what can be passed to the welcomePerosn function. I could pass nothing, a string, etc. literally anything I want. In TypeScript, we can create interfaces which define what properties an object should have.
上面的代码的问题在于,对可以传递给welcomePerosn函数的内容没有任何限制。 我什么也不能传递,也不能传递字符串等。 在TypeScript中,我们可以创建定义对象应具有的属性的接口。
In the snippet below, I created an interface for a Person object with two properties, firstName and lastName. Then, mark the welcomePerson function to accept only person objects.
在下面的代码段中,我为具有两个属性firstName和lastName的Person对象创建了一个接口。 然后,标记welcomePerson函数以仅接受人员对象。
export {};
function welcomePerson(person: Person) {
console.log(`Hey ${person.firstName} ${person.lastName}`);
return `Hey ${person.firstName} ${person.lastName}`;
}
const james = {
firstName: "James",
lastName: "Quick"
};
welcomePerson(james);
interface Person {
firstName: string;
lastName: string;
}
The benefit of this will become clear if you try to pass a string into that function. Because we are working with a TypeScript file (and VS Code is awesome!), VS Code will immediately provide you feedback letting you know that the function expects a Person object and not a string.
如果您尝试将字符串传递给该函数,则这样做的好处将变得很明显。 因为我们正在处理TypeScript文件(而VS Code非常棒!),所以VS Code将立即为您提供反馈,让您知道该函数需要使用Person对象而不是字符串。
VS Code understands TypeScript in and out and is ready to help you improve your code and prevent errors.
VS Code可以理解TypeScript的内外,可以随时帮助您改进代码并防止错误。

Now that we have a working TypeScript file, we can compile it to JavaScript. To do this you simply need to call the function and tell it which file to compile as shown in the screenshot. I'm using the built in terminal in VS Code for reference.
现在我们有了一个有效的TypeScript文件,我们可以将其编译为JavaScript。 为此,您只需要调用函数并告诉它要编译哪个文件即可,如屏幕截图所示。 我正在使用VS Code中的内置终端作为参考。

If you didn't fix the error before, you'll see any error output.
如果您之前没有解决错误,则会看到任何错误输出。

Fix the error by passing the person object in correctly instead of a string. Then compile again, and you'll get a valid JavaScript file.
通过正确输入person对象而不是字符串来解决错误。 然后再次编译,您将获得一个有效JavaScript文件。
Notice that the template literal strings, which are an ES6 feature, were compiled simple string concatenation from ES5. We'll come back to this shortly.
请注意,模板文字字符串是ES6的功能,是从ES5编译的简单字符串连接。 我们将稍后再讨论。
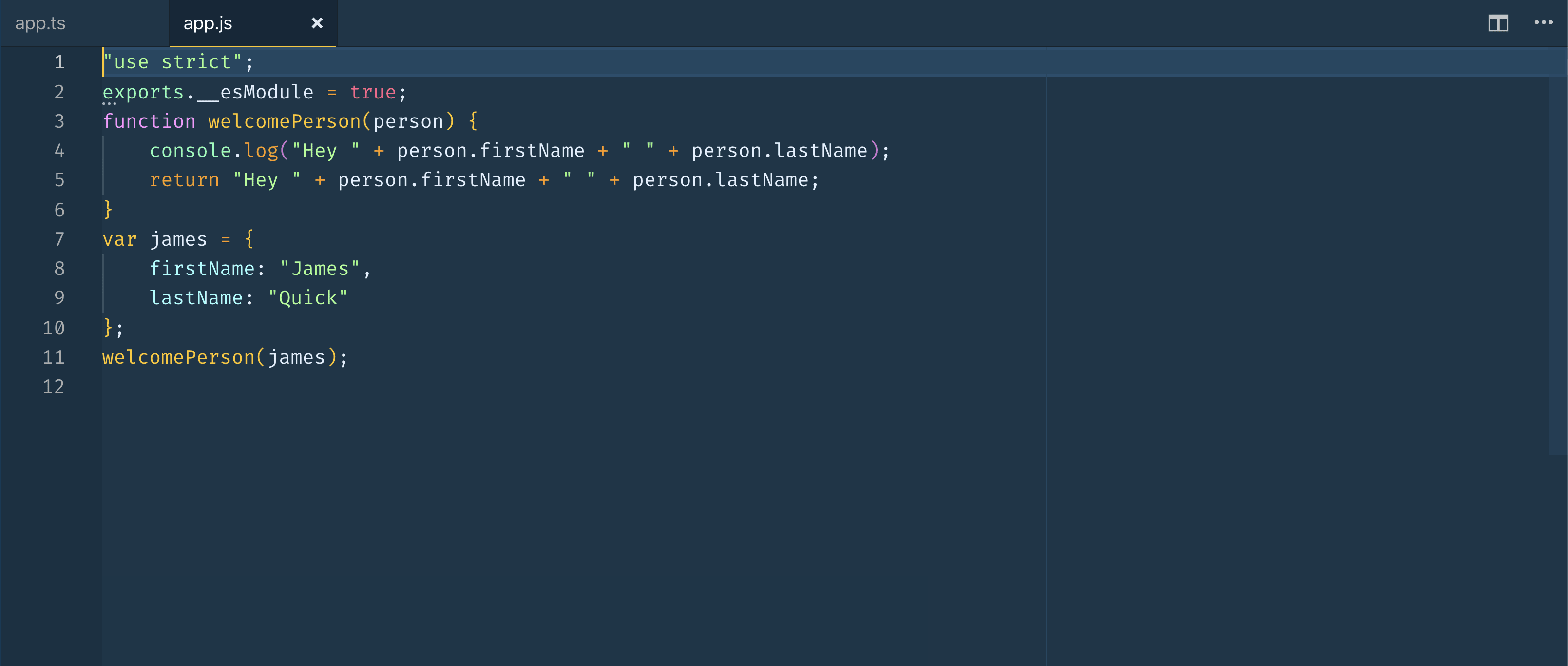
Just to prove this worked. You can now run the JavaScript directly using Node, and you should see a name printed to the console.
只是为了证明这是可行的。 现在,您可以直接使用Node运行JavaScript,并且应该在控制台上看到一个名称。

创建一个TypeScript配置文件 ( Creating a TypeScript Config File )
So far, we've compiled one file directly. This is great, but in a real world project, you might want to customize how files are compiled. For instance, you might want to have them be compiled to ES6 instead of ES5. To do this, you need to create a TypeScript configuration file.
到目前为止,我们已经直接编译了一个文件。 这很棒,但是在现实世界的项目中,您可能需要自定义文件的编译方式。 例如,您可能希望将它们编译为ES6,而不是ES5。 为此,您需要创建一个TypeScript配置文件。
To create a TypeScript configuration file, you can run the following command (similar to an npm init).
要创建TypeScript配置文件,可以运行以下命令(类似于npm init)。

Open your new config file open, and you'll see lots of different options, most of which are commented out.
打开新的配置文件,您将看到很多不同的选项,其中大多数已被注释掉。

You might have noticed there is a setting called "target" which is set to "es5". Change that setting to "es6". Since we have a TypeScript config file in our project, to compile you simple need to run "tsc". Go ahead and compile.
您可能已经注意到,有一个名为“ target”的设置被设置为“ es5”。 将该设置更改为“ es6”。 由于我们的项目中有一个TypeScript配置文件,因此只需运行“ tsc”即可进行编译。 继续编译。
Now open up the newly created JavaScript file. In the output, notice that our template literal string was left alone, proving that our TypeScript was compiled successfully to ES6.
现在打开新创建JavaScript文件。 在输出中,请注意我们的模板文字字符串被保留了下来,证明我们的TypeScript已成功编译到ES6。
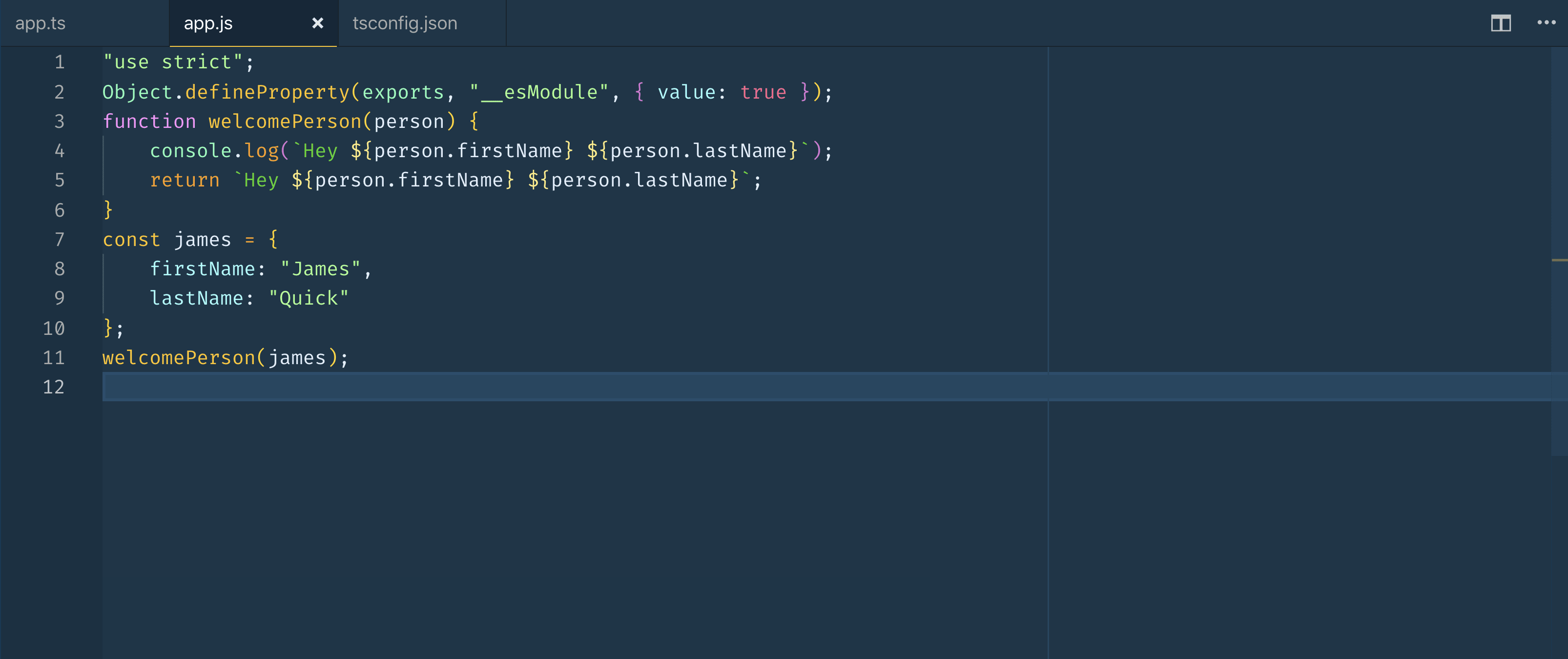
Another thing we can change is where our JavaScript files are stored after being created. This setting is "outDir".
我们可以更改的另一件事是创建JavaScript文件后将其存储在何处。 此设置为“ outDir”。
For funsies, try deleting "outDir", and then start typing it in again. Did you notice that VS Code is providing you intellisense for which properties you can set in a TypeScript config file?! How cool is that?!
对于娱乐,请尝试删除“ outDir”,然后再次开始输入。 您是否注意到VS Code为您提供了可以在TypeScript配置文件中设置哪些属性的智能感知? 多么酷啊?!
VS Code provides intellisense for TypeScript config properties.
VS Code为TypeScript配置属性提供了智能感知。
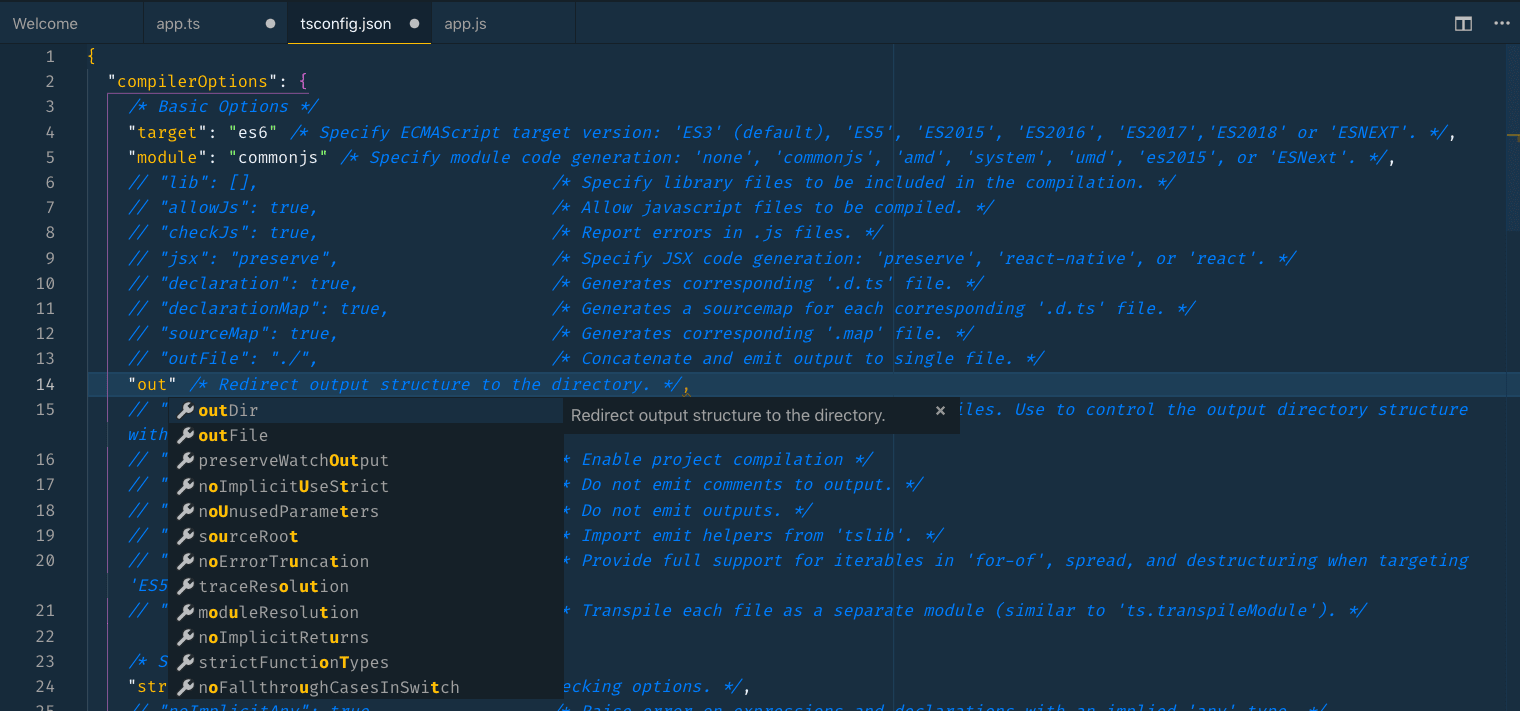
I changed my out directory to a dist folder like so.
我将出目录更改为dist文件夹,如下所示。
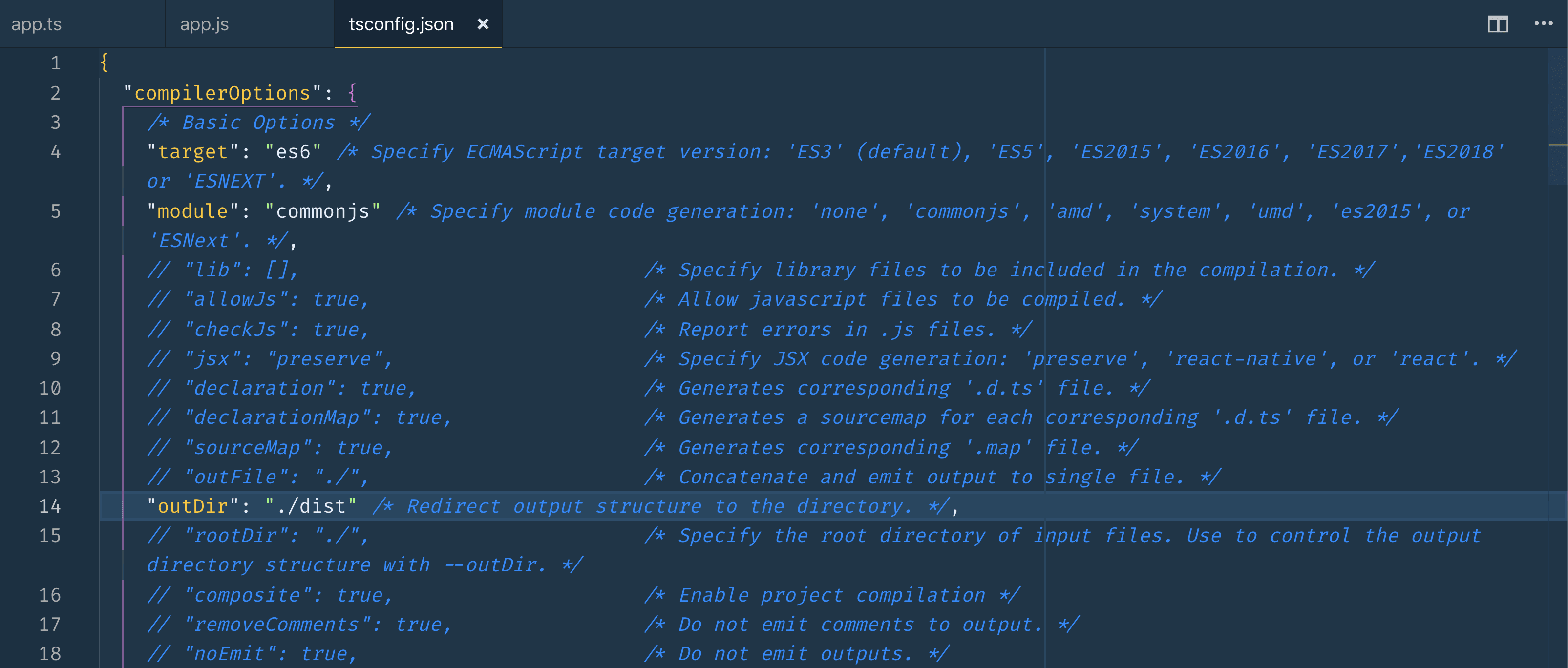
After compiling again, notice that my output JavaScript file is indeed located inside of a "dist" folder.
再次编译后,请注意,我的输出JavaScript文件确实位于“ dist”文件夹内。

使用的TypeScript ( TypeScript in Use )
TypeScript has gained more and more popularity over the last couple of years, and I think it will continue to do so. Here's a couple of examples of how they are used in modern front-end frameworks.
在过去的几年中,TypeScript越来越流行,我认为它将继续如此。 这是几个如何在现代前端框架中使用它们的示例。
角度CLI (Angular CLI)

Angular CLI projects come preconfigured with TypeScript. All of the configuration, linting, etc. is built in by default, which is pretty sweet! Create an Angular CLI project and take a look around. This is a great way to see what TypeScript looks like in a real app.
Angular CLI项目预先配置了TypeScript。 默认情况下,所有配置,棉绒等都是内置的! 创建一个Angular CLI项目并四处看看。 这是查看真实应用中TypeScript外观的好方法。
创建React App 2 (Create React App 2)
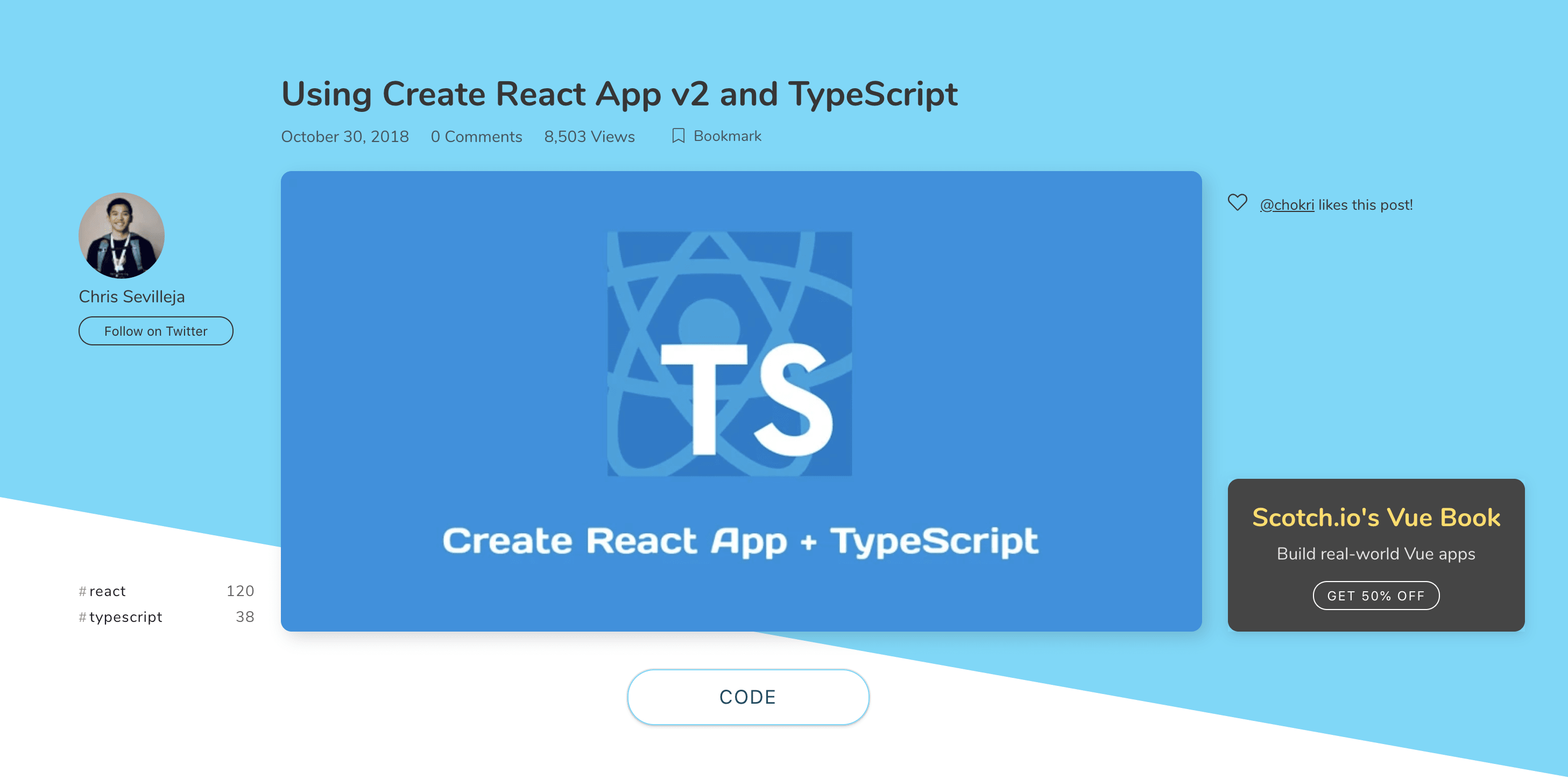
https://scotch.io/tutorials/using-create-react-app-v2和打字稿 )!
Vue CLI 3 (Vue CLI 3)

Vue CLI projects can be configured to use TypeScript when creating a new project. For more details, you can check out the [Vue Docs](https://vuejs.org/v2/guide/typescript.html).
可以将Vue CLI项目配置为在创建新项目时使用TypeScript。 有关更多详细信息,您可以查看[Vue Docs]( https://vuejs.org/v2/guide/typescript.html )。
回顾 ( Recap )
TypeScript is awesome! It allows you to generate higher quality JavaScript that can you feel more comfortable about when shipping to production. As you can tell, VS Code is excited and well equipt to helping you write TypeScript, generating configurations, etc.
TypeScript很棒! 它允许您生成更高质量JavaScript,使您在交付生产时会感到更加自在。 如您所知,VS Code很激动,并且可以帮助您编写TypeScript,生成配置等。
If you haven't already, give TypeScript a try. Let us know in the comments below what you think!
如果您还没有尝试过TypeScript,请尝试一下。 在下面的评论中让我们知道您的想法!
翻译自: https://scotch.io/tutorials/working-with-typescript-in-visual-studio-code