zeit
I wanted to deploy a simple demo node app the other day using Now, and I got into a lot of difficulties and gotchas, with little help from the official docs. If you are in my shoes, this is for you, otherwise, read on to find out how to deploy a node app easily using the Now CLI tool.
前几天,我想使用Now部署一个简单的演示节点应用程序,但是在官方文档的帮助下,我遇到了很多困难和麻烦。 如果您不知所措,这是给您的,否则,请继续阅读以了解如何使用Now CLI工具轻松部署节点应用程序。
NodeJS is a JavaScript runtime environment that runs outside a browser. Applications and APIs can be built using NodeJS. APIs are ways by which applications share data. These apps or APIs, however, need to be hosted on the internet to be accessible by other applications on the internet.
NodeJS是在浏览器外部运行JavaScript运行时环境。 可以使用NodeJS构建应用程序和API。 API是应用程序共享数据的方式。 但是,这些应用程序或API必须托管在Internet上,以供Internet上的其他应用程序访问。
In this post, we shall deploy a simple Node app using the Now CLI service.
在本文中,我们将使用Now CLI服务部署一个简单的Node应用程序。
TL:DR ( TL:DR )
Deploy a Node app in the following steps:
通过以下步骤部署Node应用程序:
- Create a
now.json
file in your node project. 在您的节点项目中创建一个now.json
文件。 - Specify the build file and the required builder (
@now/node-server
for node apps). 指定构建文件和所需的构建器(节点应用程序的@now/node-server
)。 - Create the route for each API endpoint, in
now.json
. 在now.json
为每个API端点创建路由。 - Ensure the entry filename of your server is
index.js
else specify home route innow.json
. 确保服务器的条目文件名是index.js
否则请在now.json
指定本地路由。 - Use the
now
command to publish. 使用now
命令进行发布。
ZEIT Now is a cloud deployment and severless solution offering a seamless way to deploy both static and dynamic applications.
ZEIT Now是一种云部署和无休止的解决方案,提供了无缝方式来部署静态和动态应用程序。
安装 ( Installation )
As this is a Nodejs project, check that you have node and its accompanying package manager, npm, installed with:
由于这是一个Nodejs项目,因此请检查是否安装了node及其随附的软件包管理器npm:
node -v&& npm -v
This would print the current version numbers if installed. If you don't have it installed, go on the nodejs page to download and install it.
如果已安装,将打印当前版本号。 如果尚未安装,请进入nodejs页面下载并安装。
Next, install the Now CLI tool globally from npm with:
接下来,使用以下命令从npm全局安装Now CLI工具:
npm install -g now
Create a new now-express
project directory using:
使用以下命令创建一个新now-express
项目目录:
mkdir now-express
In the project directory, create a bare node project with this command:
在项目目录中,使用以下命令创建一个裸节点项目:
npm init -y
This creates a node project having a default configuration with an accompanying package.json
file.
这将创建一个具有默认配置以及随附package.json
文件的节点项目。
Install express in the project using:
使用以下命令在项目中安装Express:
npm install --save express
Express is a Node.js framework for building web servers efficiently.
Express是用于高效构建Web服务器的Node.js框架。
创建一个Web服务器 ( Create a Web Server )
Create a new folder in the root directory called index.js
, this can have any title, and it serves as the entry point of the application. In index.js
import express, use the built-in body parser in express and create a listener on port 5000. Do this with:
在名为index.js
的根目录中创建一个新文件夹,该文件夹可以具有任何标题,并且用作应用程序的入口点。 在index.js
import express中,在express中使用内置的主体解析器,并在端口5000上创建一个侦听器。请执行以下操作:
const express = require("express");
const app = express();
const port = 5000;
// Body parser
app.use(express.urlencoded({ extended: false }));
// Listen on port 5000
app.listen(port, () => {
console.log(`Server is booming on port 5000
Visit http://localhost:5000`);
});
In index.js
Create the home route and two simple mock API endpoints with:
在index.js
创建本地路由和两个简单的模拟API端点:
// Import Dependencies
//Specify port
// Body parser
// Home route
app.get("/", (req, res) => {
res.send("Welcome to a basic express App");
});
// Mock APIs
app.get("/users", (req, res) => {
res.json([
{ name: "William", location: "Abu Dhabi" },
{ name: "Chris", location: "Vegas" }
]);
});
app.post("/user", (req, res) => {
const { name, location } = req.body;
res.send({ status: "User created", name, location });
});
Here, we created two API endpoints to get user and to send a user through. The endpoint which posts a user to the server returns this user in its response.
在这里,我们创建了两个API端点来获取用户和发送用户。 将用户发布到服务器的端点在响应中返回该用户。
In the package.json
file include a script to start the server. Update package.json
to:
在package.json
文件中,包含用于启动服务器的脚本。 将package.json
更新为:
{
"name": "now-express",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"start": "node index.js",
},
"keywords": [],
"author": "William Imoh",
"license": "MIT",
"dependencies": {
"express": "^4.17.1",
"nodemon": "^1.19.1"
}
}
To launch the server, run the command:
要启动服务器,请运行以下命令:
npm run start
Navigate to http://localhost:5000 on the browser to see the simple page.
在浏览器上导航至http:// localhost:5000以查看简单页面。
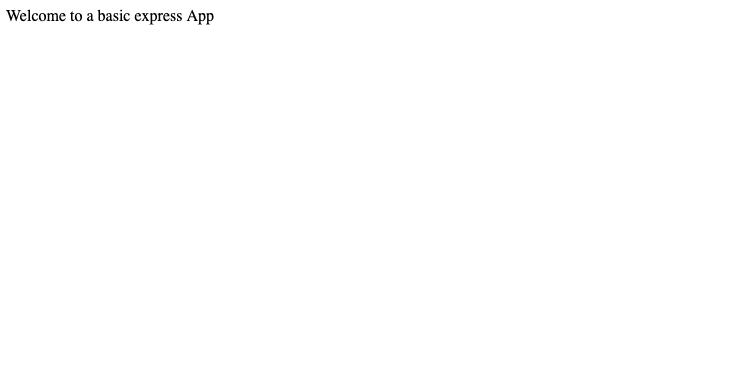
/users
route in the browser and make an API call to
/user
with data using Postman.
/users
路由,并使用Postman使用数据对/user
进行API调用。
使用Now部署应用程序 ( Deploy Application using Now )
Now requires a configuration file now.json
with which it builds a node application and creates a lambda. In the absence of the configuration file, the files are served statically. Create a file named now.json
in the root folder of the application.
现在需要一个配置文件now.json
用它来构建节点应用程序并创建一个lambda。 在没有配置文件的情况下,这些文件是静态提供的。 在应用程序的根文件夹中创建一个名为now.json
的文件。
In the configuration file, key parameters required to build the app are specified with the more important ones being the build and the builders. Create a build for the index.js
file in now.json
with:
在配置文件中,指定了构建应用所需的关键参数,其中更重要的参数是构建和构建器。 使用以下命令在now.json
为index.js
文件创建一个构建:
{
"version": 2,
"builds": [{ "src": "index.js", "use": "@now/node-server" }],
}
Here, we first specified the version of the Now platform (version 2), then we specified the source file for the node app. The @now/node-server
builder is used and recommended for node apps.
在这里,我们首先指定Now平台的版本(版本2),然后指定节点应用程序的源文件。 @now/node-server
构建器用于节点应用程序,建议将其用于节点应用程序。
Using the @now/node
builder throws an error when you access the application after deployment. @now/node
is recommended for single node serverless functions.

部署后访问应用程序时,使用@now/node
构建器将引发错误。 对于单节点无服务器功能,建议使用@now/node
。
You can read more about builds and builders.
While this setup will ship our application and provide a valid URL, we must specify the API routes else they will not be available when the app is deployed. Here's a sample error page from navigating to any route or route group when it's not specified in now.json
.
虽然此设置将交付我们的应用程序并提供有效的URL,但我们必须指定API路由,否则在部署该应用程序时它们将不可用 。 这是一个示例错误页面,当在now.json
未指定该now.json
时,它会导航到任何路由或路由组。
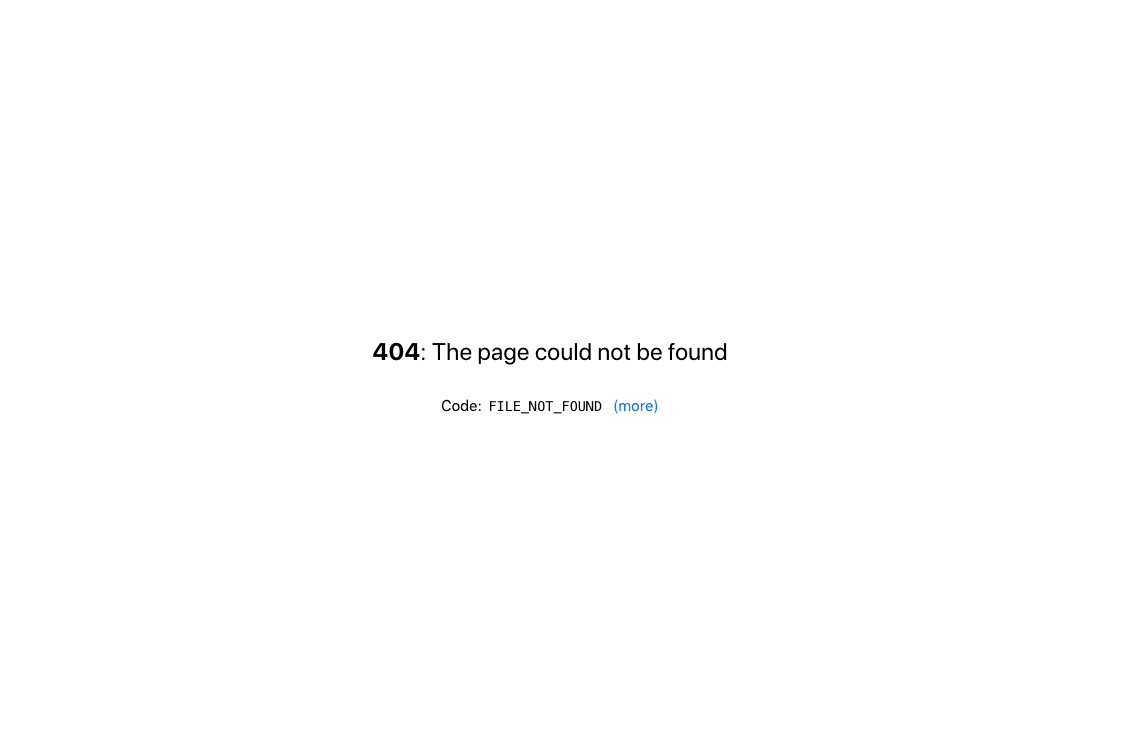
In now.json
create a key for routes, edit now.json
to:
在now.json
创建路由密钥,将now.json
编辑为:
{
"version": 2,
"builds": [{ "src": "index.js", "use": "@now/node-server" }],
"routes": [
{
"src": "/users",
"dest": "/index.js",
"methods": ["GET"]
},
{
"src": "/user",
"dest": "/index.js",
"methods": ["POST"]
}
]
}
This points the two available endpoints to destination pathnames as well as specifying the HTTP verbs available on each endpoint. Failure to state the applicable methods leaves the endpoint open to any kind of HTTP request.
这会将两个可用端点指向目标路径名,并指定每个端点上可用的HTTP动词。 无法说明适用的方法会使端点对任何类型的HTTP请求开放。
The routes also take keys specifying applicable headers and status codes for each route. You can find more about handling routes in deployment here.
路由还带有指定适用于每个路由的标头和状态代码的密钥。 您可以在此处找到有关在部署中处理路由的更多信息。
In the command line, deploy your application using:
在命令行中,使用以下命令部署应用程序:
now
This deploys your application and provides a valid URL, in my case, I have:
这将部署您的应用程序并提供有效的URL,就我而言,我有:

You can find the app on https://now-express.williamimoh.now.sh Navigate to /users
to see an object with data from the server.
您可以在https://now-express.williamimoh.now.sh上找到该应用程序。导航到/users
以查看包含服务器数据的对象。
Gotcha ( Gotcha )
One key thing to note, while you may have a different name for your server file other than index.js
, Now requires that the entry file name is index
to be recognised, as well as the correct file extension. Failure to adhere to this naming convention will have your app being rendered using Now's directory listing.
需要注意的一件事是,尽管服务器文件的名称可能不同于index.js
,但现在要求输入文件名是可识别的index
,以及正确的文件扩展名。 如果不遵守此命名约定,则会使用Now的目录清单呈现您的应用程序。
Now requires the entry node file to be named index.js.
现在需要将入口节点文件命名为index.js。
A workaround to this is to specify the home route in the now.json
and point the destination to the server file. Even at this, the build source file specified in now.json
will direct to the server file.
一种解决方法是在now.json
指定本地路由,然后将目标指向服务器文件。 即使这样, now.json
指定的构建源文件now.json
将直接定向到服务器文件。
Assuming we rename the entry file of this project from index.js
to server.js
, now.json
becomes:
假设我们将该项目的入口文件从index.js
重命名为server.js
, now.json
变为:
{
"version": 2,
"builds": [{ "src": "server.js", "use": "@now/node-server" }],
"routes": [
{
"src": "/",
"dest": "/server.js",
"methods": ["GET"]
},
{
"src": "/users",
"dest": "/server.js",
"methods": ["GET"]
},
{
"src": "/user",
"dest": "/server.js",
"methods": ["POST"]
}
]
}
You can find the completed project on Github: https://github.com/Chuloo/now-express
您可以在Github上找到完成的项目: https : //github.com/Chuloo/now-express
结论 ( Conclusion )
In this post, we deployed a simple node app using Now and obtained a live URL. We also saw some gotchas with deploying an app using Now.
在本文中,我们使用Now部署了一个简单的节点应用程序,并获得了实时URL。 我们还看到了一些使用Now部署应用程序的陷阱。
Feel free to comment on this post if you have any questions or suggestions. Happy keyboard slapping.
如果您有任何疑问或建议,请随时评论此帖子。 快乐的键盘掌声。
翻译自: https://scotch.io/tutorials/easily-deploy-a-serverless-node-app-with-zeit-now
zeit