花式js
Nowadays there are many Javascript libraries to make it easy the task of adding sliders to a website. Most have a host of features, to adapt the slider to the particular needs of each case. However, despite having so many functionalities, sometimes the sliders lack features that would result in a much better user experience.
如今,有许多Javascript库可以简化向网站添加滑块的任务。 大多数具有许多功能,可以使滑块适应每种情况的特殊需求。 但是,尽管功能如此之多,但有时滑块仍缺乏可带来更好用户体验的功能。
In the case of sliders that implemented the drag and drop functionality, it is hard to see how almost none (not to be absolute) implements the ability to continue the movement that the user has started, adding momentum and deceleration as necessary.
对于实现了拖放功能的滑块,很难看到几乎没有(不是绝对的)实现用户已经开始的运动的能力,并根据需要增加了动量和减速度。
In this tutorial we will introduce a new library, specifically to develop sliders with momentum in a simple way. Also we will create a fancy countdown timer, using this excellent design by Oleg Frolov as inspiration:
在本教程中,我们将介绍一个新的库,专门用于以简单的方式开发具有动量的滑块。 同样,我们将以Oleg Frolov的出色设计为灵感,创建一个精美的倒数计时器:
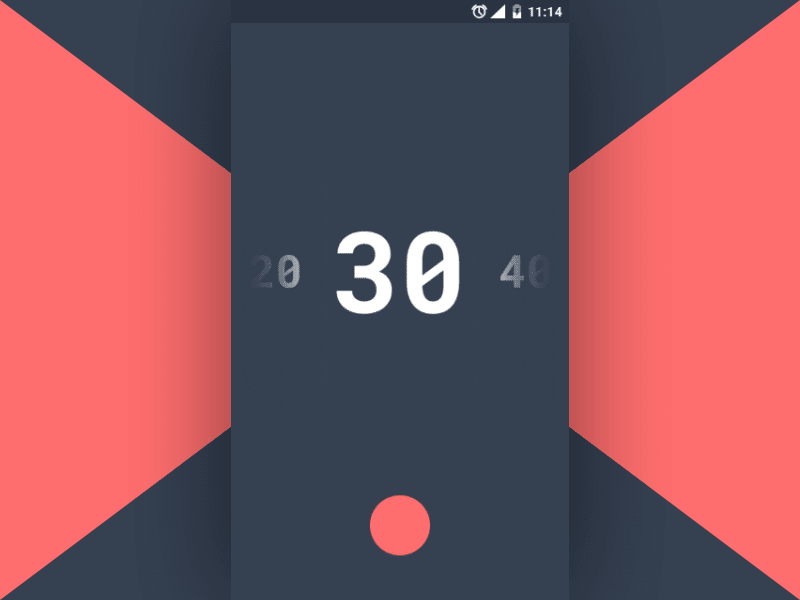
The final result will be the following:
最终结果如下:

If you want to know how to implement it, just keep reading :)
如果您想知道如何实现它,请继续阅读:)
推出MomentumSlider ( Introducing MomentumSlider )
Before starting to implement our countdown timer component, let's see what the new library MomentumSlider
can do for us.
在开始实施倒数计时器组件之前,让我们看看新的库MomentumSlider
可以为我们做什么。
As its name suggests, the main functionality of this new library is to create sliders with momentum, although it also contains the basic functionalities of a common slider.
顾名思义,这个新库的主要功能是创建具有动量的滑块,尽管它也包含常见滑块的基本功能。
It has no dependencies, so to start using it we just have to include it in the HTML and create a new instance of MomentumSlider
. Something like:
它没有依赖关系,因此要开始使用它,我们只需将其包含在HTML中并创建MomentumSlider
的新实例。 就像是:
var mySlider = new MomentumSlider({
el: '.container', // HTML element to append the slider
range: [1, 5] // Autogenerated number range
});
As you can figure out, the previous code is intended to generate a slider of numbers using the defined range, and include it within the .container
element.
如您.container
,以前的代码旨在使用定义的范围生成数字滑块,并将其包括在.container
元素内。
Of course, not all sliders are number ranges, so we can also provide custom elements for our slider, for which we must provide an HTML structure like the following:
当然,并不是所有的滑块都具有数字范围,因此我们还可以为滑块提供自定义元素,为此我们必须提供如下HTML结构:
<div class="container">
<!-- Slider container -->
<div class="ms-container ms-container--horizontal">
<!-- Slider track -->
<ul class="ms-track">
<!-- Slider slides -->
<li class="ms-slide">1</li>
<li class="ms-slide">2</li>
<li class="ms-slide">3</li>
<li class="ms-slide">4</li>
<li class="ms-slide">5</li>
</ul>
</div>
</div>
And then create a new instance of MomentumSlider
in this way:
然后以这种方式创建MomentumSlider
的新实例:
var mySlider = new MomentumSlider({
el: '.ms-container' // HTML element to init a slider
});
The final result of this second example will be exactly the same as in the first, which we have done on purpose to illustrate how HTML is generated in the first case. The advantage of this second example is that within each .ms-slide
element we can put anything :)
第二个示例的最终结果将与第一个示例完全相同,我们故意这样做是为了说明在第一种情况下如何生成HTML。 第二个示例的优点是,在每个.ms-slide
元素内,我们可以放置任何内容:)
On the other hand, the general styles and dimensions of the slider must be specified in the CSS code. The library will work using those dimensions, which gives a lot of possibilities for customization.
另一方面,必须在CSS代码中指定滑块的常规样式和尺寸。 该库将使用这些尺寸进行工作,这为定制提供了很多可能性。
So, after this brief introduction to our new library to develop sliders with momentum, we can begin to develop our countdown component.
因此,在对我们的新库进行简要介绍以开发具有动力的滑块之后,我们可以开始开发倒计时组件。
HTML结构 ( HTML Structure )
Let's start with the necessary HTML code:
让我们从必要HTML代码开始:
<!-- Mobile container -->
<main class="container">
<!-- Progress bar -->
<div class="progress"></div>
<!-- Countdown counter -->
<div class="counter">1</div>
<!-- Button to toggle countdown -->
<button class="button">Toggle</button>
</main>
As we can see it is a piece of code quite simple. We have not even had to add the numbers for the slider manually, since they will be generated in the Javascript.
如我们所见,这是一段非常简单的代码。 我们甚至不必手动添加滑块的数字,因为它们将在Javascript中生成。
添加样式 ( Adding Styles )
Now we will add the general styles to match the original design:
现在,我们将添加常规样式以匹配原始设计:
body {
overflow: hidden;
// Decorative background
&:before {
content: '';
position: absolute;
left: 0;
top: 0;
width: 100%;
height: 100%;
border-left: 50vw solid #FF6E6E;
border-right: 50vw solid #FF6E6E;
border-top: 50vh solid #354051;
border-bottom: 50vh solid #354051;
z-index: -1;
}
}
// Mobile container
.container {
position: absolute;
left: 50%;
top: 50%;
width: 340px;
height: 540px;
background-color: #354051;
box-shadow: 0 0 100px rgba(0, 0, 0, 0.5);
transform: translate(-50%, -50%);
overflow: hidden;
// Header bar, just an aesthetic detail
&:before {
content: '';
position: absolute;
left: 0;
top: 0;
width: 100%;
height: 25px;
background-color: rgba(0, 0, 0, 0.2);
z-index: 3;
}
}
Then we can add the styles needed for the slider. The most important styles here are those designed to position the slider elements, as well as some other styles to achieve the look and feel we want:
然后,我们可以添加滑块所需的样式。 这里最重要的样式是用来放置滑块元素的样式,以及一些其他样式可以实现我们想要的外观:
// Slider dimmensions
$ms-container-width: 340px;
$ms-slide-width: 125px;
$ms-slide-height: 540px;
// Slider container
.ms-container {
position: relative;
top: 50%;
width: $ms-container-width;
max-width: 100%;
margin: 0 auto;
overflow: hidden;
transform: translateY(-50%);
}
// Slider track
.ms-track {
position: relative;
left: calc(50% - #{$ms-slide-width / 2}); // Centering
white-space: nowrap;
font-size: 0;
list-style: none;
padding: 0;
margin: 0;
will-change: transform;
}
// Slides
.ms-slide {
display: inline-flex;
align-items: center;
justify-content: center;
width: $ms-slide-width;
height: $ms-slide-height;
font-size: 100px;
font-family: 'Roboto Mono', monospace;
color: #FFFFFF;
user-select: none;
will-change: transform;
}
To finish with our slider we need to add the gradient effect to smoothly hide the slides adjacent to the current slide. We get that with a pair of pseudo-elements from the .ms-container
element. Let's see the code:
为了完成我们的滑块,我们需要添加渐变效果以平滑隐藏与当前幻灯片相邻的幻灯片。 我们从.ms-container
元素中获得了一对伪元素。 让我们看一下代码:
// Slider container
.ms-container {
// Gradients to hide the adjacent numbers smoothly
&:before, &:after {
content: '';
position: absolute;
top: 0;
width: 80px;
height: 100%;
z-index: 1;
pointer-events: none;
}
&:before {
left: 0;
background-image: linear-gradient(to right, rgba(53, 64, 81, 1) 25%, rgba(53, 64, 81, 0));
}
&:after {
right: 0;
background-image: linear-gradient(to left, rgba(53, 64, 81, 1) 25%, rgba(53, 64, 81, 0));
}
}
And we're done with the styles for the slider!
我们已经完成了滑块的样式!
添加与Javascript的交互性 ( Adding Interactivity with Javascript )
We already have our slider looking great, we just need to use our library MomentumSlider
to add the necessary functionality. Let's see how we can do it in the following piece of code:
我们已经使滑块看起来很棒,我们只需要使用我们的库MomentumSlider
即可添加必要的功能。 让我们看看如何在下面的代码中做到这一点:
// Initializing the slider
var ms = new MomentumSlider({
el: '.container', // HTML element to append the slider
range: [1, 60], // Generate the elements of the slider using the range of numbers defined
loop: 2, // Make the slider infinite, adding 2 extra elements at each end
style: {
// Styles to interpolate as we move the slider
// The first value corresponds to the adjacent elements
// The second value corresponds to the current element
transform: [{scale: [0.4, 1]}],
opacity: [0.3, 1]
}
});
Surely you have noticed that the previous code is simple, except perhaps for the style
option. That is why we are going to explain a bit more about what this option is for.
当然,您已经注意到,前面的代码很简单,除了style
选项。 这就是为什么我们将解释更多有关此选项的用途的原因。
The style
option allows you to interpolate CSS styles as the slider is moved. Each property to be interpolated receives an Array
value, in which the first value corresponds to the previous adjacent slide, the second value corresponds to the current slide, and the third value corresponds to the subsequent adjacent slide. In the absence of a third value (as in our case), the first value will be used instead.
style
选项允许您在移动滑块时插入CSS样式。 每个要插值的属性都接收一个Array
值,其中第一个值对应于先前的相邻幻灯片,第二个值对应于当前幻灯片,第三个值对应于后续的相邻幻灯片。 如果没有第三个值(如本例所示),则将使用第一个值。
In this way, you can achieve very nice effects, like the one we have achieved in our countdown timer :)
这样,您可以达到非常好的效果,就像我们在倒数计时器中实现的效果一样:)
实施倒计时 (Implementing the Countdown)
So far we have seen how to create our slider from scratch. Now let's see how to add a simple countdown functionality.
到目前为止,我们已经看到了如何从头开始创建滑块。 现在,让我们看看如何添加简单的倒计时功能。
In order to avoid making the tutorial too long, we are going to skip the necessary CSS code to achieve the final result we want to show. However, we think it is important to highlight how we have implemented the countdown timer functionality.
为了避免使教程太长,我们将跳过必要CSS代码以实现我们想要显示的最终结果。 但是,我们认为重要的是要突出我们如何实现倒数计时器功能。
First we will listen the click
event on the Toggle button
, and we will start or stop the countdown accordingly:
首先,我们将监听Toggle button
上的click
事件,然后我们将相应地开始或停止倒计时:
// Simple toggle functionality
button.addEventListener('click', function () {
if (running) {
stop();
} else {
start();
}
running = !running;
});
Of course, for everything to work properly we must implement the start
and stop
functions respectively. Let's see the code, commented in detail for a better understanding:
当然,为了使一切正常工作,我们必须分别实现start
和stop
功能。 让我们看一下代码,对它进行详细注释以更好地理解:
// Start the countdown
function start() {
// Disable the slider during countdown
ms.disable();
// Get current slide index, and set initial values
seconds = ms.getCurrentIndex() + 1;
counter.innerText = secondsInitial = seconds;
root.style.setProperty('--progress', 0);
// Add class to trigger CSS transitions for `running` state
container.classList.add('container--running');
// Set interval to update the component every second
timer = setInterval(function () {
// Update values
counter.innerText = --seconds;
root.style.setProperty('--progress', (secondsInitial - seconds) / secondsInitial * 100);
// Stop countdown if it's finished
if (!seconds) {
stop();
running = false;
}
}, 1000);
}
// Stop the countdown
function stop() {
// Enable slider
ms.enable();
// Clear interval
clearInterval(timer);
// Reset progress
root.style.setProperty('--progress', 100);
// Remove `running` state
container.classList.remove('container--running');
}
精加工 ( Finishing )
And we have finished the essential parts of our fancy component! Check it out :)
至此,我们已经完成了花式组件的基本部分! 签出 :)

In this tutorial we saw how to use the new MomentumSlider
library to create amazing sliders. Please note that this library is still in development, so if you find an error or want to contribute, you can do so through the Github repository. Stay tunned!
在本教程中,我们看到了如何使用新的MomentumSlider
库创建令人惊叹的滑块。 请注意,该库仍在开发中,因此,如果您发现错误或想要提供帮助,可以通过Github存储库进行 。 敬请期待!
And remember that in this tutorial we only explain the essential parts of our countdown timer. We invite you to check the full code on Github, or modify it and try new things in the Codepen demo.
请记住,在本教程中,我们仅说明了倒数计时器的基本组成部分。 我们邀请您检查Github上的完整代码 ,或对其进行修改,然后在Codepen演示中尝试新功能。
We sincerely hope that you have enjoyed the tutorial and that it has been useful!
我们衷心希望您喜欢本教程并且对您有所帮助!
翻译自: https://scotch.io/tutorials/building-a-fancy-countdown-timer-with-momentumsliderjs
花式js