票务小程序源码
Update (August 15, 2016): Fixed typos in code snippets and add "use Illuminate\Support\Facades\Auth" to "CommentsController" This is the second and concluding part of Build A Support Ticket Application With Laravel. In part 1, we setup the application and created our first ticket.
更新(2016年8月15日) :修复了代码片段中的拼写错误,并在“ CommentsController”中添加了“ use Illuminate \ Support \ Facades \ Auth”,这是使用Laravel构建支持票务应用程序的第二部分。 在第1部分中,我们设置了应用程序并创建了第一张票证。
In case you missed it, you should check Build A Support Ticket Application With Laravel - Part 1 out because we will be continuing from where we stopped.
万一您错过了它,您应该检查使用Laravel构建支持票证应用程序-第1部分 ,因为我们将从停止的地方继续。
显示用户票证 ( Displaying User Tickets )
We start off by allowing our users to see a list of all the tickets they have created and from there they can go on to view a particular ticket. Add the code below to TicketsController
首先,允许用户查看他们创建的所有票证的列表,然后从那里继续查看特定的票证。 将以下代码添加到TicketsController
// TicketsController.php
// Remember to add the lines below at the top of the controller
// use App\User;
// use App\Ticket;
// use App\Category;
// use Illuminate\Support\Facades\Auth;
public function userTickets()
{
$tickets = Ticket::where('user_id', Auth::user()->id)->paginate(10);
$categories = Category::all();
return view('tickets.user_tickets', compact('tickets', 'categories'));
}
We are getting the tickets created by the authenticated user by passing Auth::user()->id
to the where()
and getting only the 10 tickets to be displayed in a page by using Laravel paginate()
and passing 10 to it.
我们通过将Auth::user()->id
传递到where()
并通过使用Laravel paginate()
并将其传递10来获取仅10张要在页面中显示的票据,从而获得通过身份验证的用户创建的票据。
This will prevent us from showing all tickets created by a user in a single page which can be awkward if the user has lots of tickets. The tickets together with the categories are passed to a view file which will we will create shortly. Before creating the view file, let's create the routes that will handle displaying all tickets created by a user. Add the line below to routes.php
这将阻止我们在单个页面上显示用户创建的所有票证,如果用户有很多票证,这可能会很尴尬。 票证和类别一起传递到一个视图文件,我们将在不久后创建它。 在创建视图文件之前,让我们创建将处理显示用户创建的所有票证的路由。 routes.php
下行添加到routes.php
Route::get('my_tickets', 'TicketsController@userTickets');
With the routes created, lets go on and create the view. Create a new view file named user_tickets.blade.php
in the tickets
folder and paste the code below to it:
创建路线后,继续创建视图。 在tickets
文件夹中创建一个名为user_tickets.blade.php
的新视图文件,并将以下代码粘贴至该文件:
@extends('layouts.app')
@section('title', 'My Tickets')
@section('content')
<div class="row">
<div class="col-md-10 col-md-offset-1">
<div class="panel panel-default">
<div class="panel-heading">
<i class="fa fa-ticket"> My Tickets</i>
</div>
<div class="panel-body">
@if ($tickets->isEmpty())
<p>You have not created any tickets.</p>
@else
<table class="table">
<thead>
<tr>
<th>Category</th>
<th>Title</th>
<th>Status</th>
<th>Last Updated</th>
</tr>
</thead>
<tbody>
@foreach ($tickets as $ticket)
<tr>
<td>
@foreach ($categories as $category)
@if ($category->id === $ticket->category_id)
{{ $category->name }}
@endif
@endforeach
</td>
<td>
<a href="{{ url('tickets/'. $ticket->ticket_id) }}">
#{{ $ticket->ticket_id }} - {{ $ticket->title }}
</a>
</td>
<td>
@if ($ticket->status === 'Open')
<span class="label label-success">{{ $ticket->status }}</span>
@else
<span class="label label-danger">{{ $ticket->status }}</span>
@endif
</td>
<td>{{ $ticket->updated_at }}</td>
</tr>
@endforeach
</tbody>
</table>
{{ $tickets->render() }}
@endif
</div>
</div>
</div>
</div>
@endsection
First, we check if there are tickets and then display them within a table. For the ticket category, we check if the ticket category_id
is equal to the category id
then display the name of the category. Lastly the render()
will help display the pagination links.
首先,我们检查是否有票证,然后将其显示在表格中。 对于票证类别,我们检查票证category_id
是否等于类别id
然后显示类别的名称。 最后, render()
将帮助显示分页链接。
Now if you visit the route /my_tickets
you should see a page listing the tickets (in my case, just a ticket) that has been created my the authenticated user like the image below:
现在,如果您访问/my_tickets
路线,您应该会看到一个页面,其中列出了已通过身份验证的用户创建的票证(在我的情况下,只是一张票证),如下图所示:
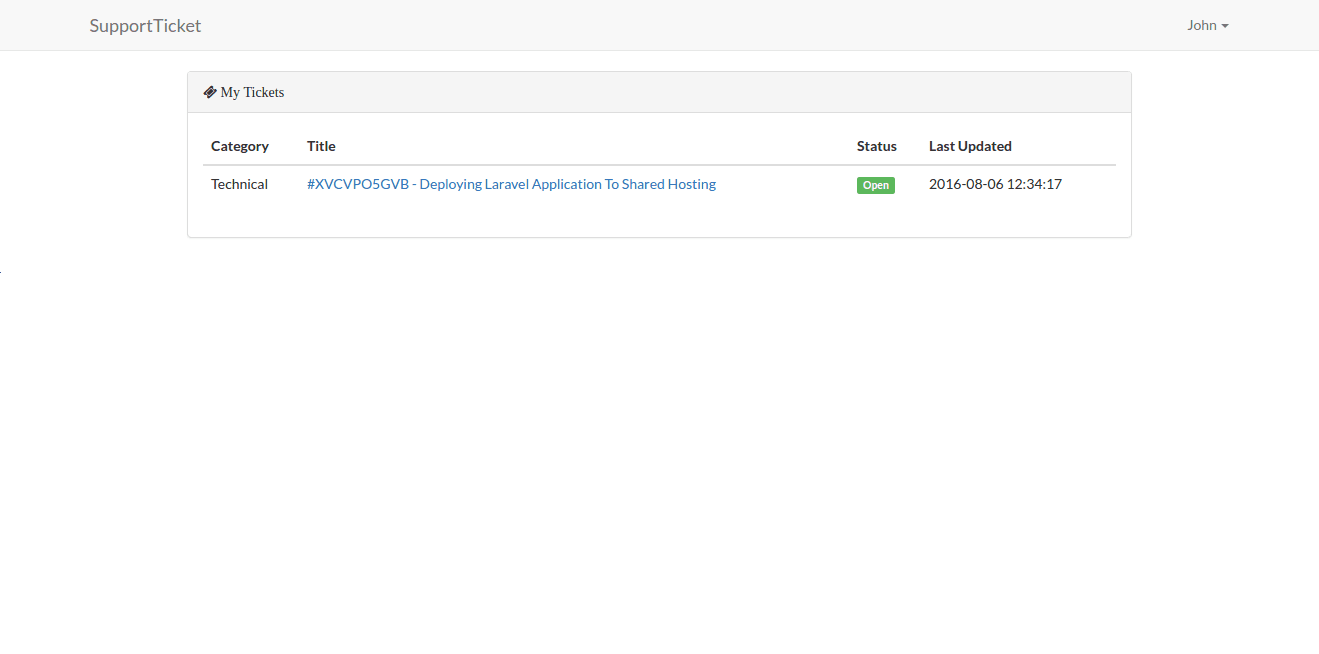
出示票 ( Showing A Ticket )
Now that a user can see a list of all the tickets he/she has created, wouldn't it be nice if the user could view a particular ticket? Of course.
现在,用户可以看到他/她创建的所有票证的列表,如果用户可以查看特定的票证,那不是很好吗? 当然。
Let's tackle that. We want the user to be able to access a particular ticket by this route tickets/ticket_id
.
让我们解决这个问题。 我们希望用户能够通过此路线tickets/ticket_id
访问特定票证。
Okay, let's create the route in routes.php
好吧,让我们在routes.php
创建路由
Route::get('tickets/{ticket_id}', 'TicketsController@show');
As you can see, when the user hits that route, the show()
, which we have yet to create, will be triggered. Add this code below to TicketsController
:
如您所见,当用户点击该路线时,将触发我们尚未创建的show()
。 将以下代码添加到TicketsController
:
// app/Http/Controllers/TicketsController.php
public function show($ticket_id)
{
$ticket = Ticket::where('ticket_id', $ticket_id)->firstOrFail();
$category = $ticket->category;
return view('tickets.show', compact('ticket', 'category'));
}
Here show()
accepts an argument ticket_id
which will be passed from the route, and we get the ticket with that ticket_id. Remember from Part 1 where we define our Ticket to Category relationship. Using $ticket->category
will get the category which the ticket belongs to. And finally we return a view file with the ticket and category passed to it. Create a view file in the tickets
folder with the name show.blade.php
and paste the code below into it:
在这里, show()
接受一个参数ticket_id
,该参数将从路由中传递,然后我们获得带有该ticket_id的票证。 请记住,在第1部分中,我们定义了“票证与类别”关系。 使用$ticket->category
将获得票证所属的类别。 最后,我们返回一个包含票证和类别的视图文件。 在tickets
文件夹中创建一个名为show.blade.php
的视图文件,并将以下代码粘贴到其中:
// /resources/views/tickets/show.blade.php
@extends('layouts.app')
@section('title', $ticket->title)
@section('content')
<div class="row">
<div class="col-md-10 col-md-offset-1">
<div class="panel panel-default">
<div class="panel-heading">
#{{ $ticket->ticket_id }} - {{ $ticket->title }}
</div>
<div class="panel-body">
@include('includes.flash')
<div class="ticket-info">
<p>{{ $ticket->message }}</p>
<p>Categry: {{ $category->name }}</p>
<p>
@if ($ticket->status === 'Open')
Status: <span class="label label-success">{{ $ticket->status }}</span>
@else
Status: <span class="label label-danger">{{ $ticket->status }}</span>
@endif
</p>
<p>Created on: {{ $ticket->created_at->diffForHumans() }}</p>
</div>
<hr>
<div class="comment-form">
<form action="{{ url('comment') }}" method="POST" class="form">
{!! csrf_field() !!}
<input type="hidden" name="ticket_id" value="{{ $ticket->id }}">
<div class="form-group{{ $errors->has('comment') ? ' has-error' : '' }}">
<textarea rows="10" id="comment" class="form-control" name="comment"></textarea>
@if ($errors->has('comment'))
<span class="help-block">
<strong>{{ $errors->first('comment') }}</strong>
</span>
@endif
</div>
<div class="form-group">
<button type="submit" class="btn btn-primary">Submit</button>
</div>
</form>
</div>
</div>
</div>
</div>
@endsection
You should get something like the image below when you click on the link of a particular ticket.
当您单击特定故障单的链接时,您应该会看到类似下图的内容。
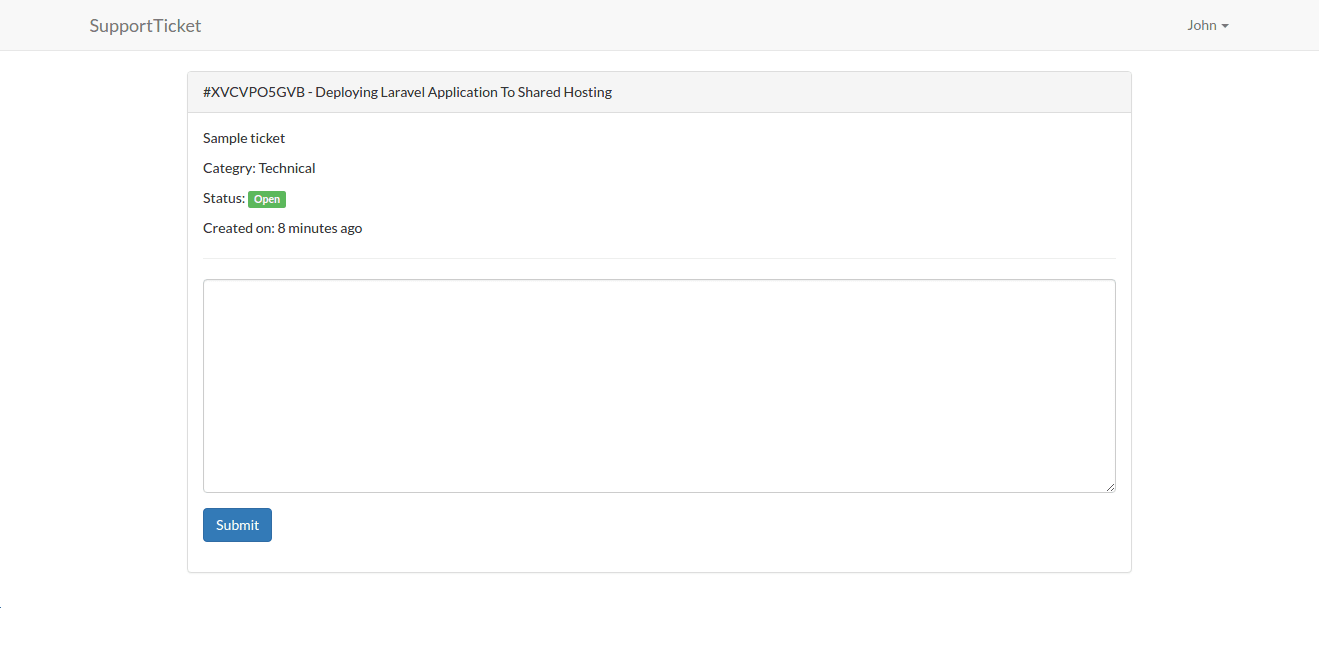
If you noticed, there is a text box which will allow the user to reply/comment on ticket. Not to worry we'll get to it shortly. We'll be updating the show view too to display the comments on the ticket.
如果您注意到,会有一个文本框,允许用户在票证上回复/评论。 不用担心,我们很快就会解决。 我们还将更新显示视图,以在票证上显示评论。
对票进行评论 ( Commenting On A Ticket )
Now let's allow the user to comment on a ticket. But first we need a place to store the comments. Create a Comment
model and a migration file with the command below:
现在,让用户对故障单发表评论。 但是首先我们需要一个地方来存储评论。 使用以下命令创建Comment
模型和迁移文件:
php artisan make:model Comment -m
Open the migration file just created and update with the code below:
打开刚刚创建的迁移文件,并使用以下代码进行更新:
Schema::create('comments', function (Blueprint $table) {
$table->increments('id');
$table->integer('ticket_id')->unsigned();
$table->integer('user_id')->unsigned();
$table->text('comment');
$table->timestamps();
});
Run the migration
运行迁移
php artisan migrate
Open Comment
model and add the following to it:
打开Comment
模型并向其中添加以下内容:
// Comment.php
protected $fillable = [
'ticket_id', 'user_id', 'comment'
];
The above will define the fields that can be mass assigned.
上面将定义可以批量分配的字段。
票证评论关系 ( Ticket To Comment Relationship )
A Comment can belong to a Ticket, while a Ticket can have many Comments. This is a one to many relationship and we will use Eloquent to setup the relationship. Open the Comment
model and paste the code below into it:
注释可以属于故障单,而故障单可以具有许多注释。 这是一对多的关系,我们将使用Eloquent来建立关系。 打开Comment
模型并将以下代码粘贴到其中:
// Comment.php
public function ticket()
{
return $this->belongsTo(Ticket::class);
}
Now let's edit the Ticket
model in the same manner to add the inverse relationship:
现在,以相同的方式编辑Ticket
模型以添加逆关系:
// Ticket.php
public function comments()
{
return $this->hasMany(Comment::class);
}
用户评论关系 ( User To Comment Relationship )
A Comment can belong to a User, while a User can have many Comments. This is a one to many relationship and we will use Eloquent to setup the relationship. Open the Comment
model and paste the code below into it:
一个注释可以属于一个用户,而一个用户可以具有多个注释。 这是一对多的关系,我们将使用Eloquent来建立关系。 打开Comment
模型并将以下代码粘贴到其中:
// Comment.php
public function user()
{
return $this->belongsTo(User::class);
}
Now let's edit the User
model in the same manner to add the inverse relationship:
现在,让我们以相同的方式编辑User
模型以添加逆关系:
// User.php
public function comments()
{
return $this->hasMany(Comment::class);
}
用户到票证关系 ( User To Ticket Relationship )
A Ticket can belong to a User, while a User can have many Ticket. This is a one to many relationship and we will use Eloquent to setup the relationship. Open the User
model and paste the code below into it:
一个票证可以属于一个用户,而一个用户可以拥有多个票证。 这是一对多的关系,我们将使用Eloquent来建立关系。 打开User
模型并将以下代码粘贴到其中:
// User.php
public function tickets()
{
return $this->hasMany(Ticket::class);
}
Now let's edit the Ticket
model in the same manner to add the inverse relationship:
现在,以相同的方式编辑Ticket
模型以添加逆关系:
// Ticket.php
public function user()
{
return $this->belongsTo(User::class);
}
评论控制器 ( Comment Controller )
We need to create a new controller that will handle all comments specific logics. Run the code below to create a CommentsController
.
我们需要创建一个新的控制器来处理所有注释特定的逻辑。 运行以下代码以创建CommentsController
。
php artisan make:controller CommentsController
The CommentsController
will have only one method called postComment()
.
CommentsController
将只有一个称为postComment()
方法。
// Remember to add the lines below at the top of the controller
// use App\User;
// use App\Ticket;
// use App\Comment;
// use App\Mailers\AppMailer;
// use Illuminate\Support\Facades\Auth;
public function postComment(Request $request, AppMailer $mailer)
{
$this->validate($request, [
'comment' => 'required'
]);
$comment = Comment::create([
'ticket_id' => $request->input('ticket_id'),
'user_id' => Auth::user()->id,
'comment' => $request->input('comment'),
]);
// send mail if the user commenting is not the ticket owner
if ($comment->ticket->user->id !== Auth::user()->id) {
$mailer->sendTicketComments($comment->ticket->user, Auth::user(), $comment->ticket, $comment);
}
return redirect()->back()->with("status", "Your comment has be submitted.");
}
What the postComment()
does is simple; make sure the comment box is filled, store a comment in the comments table with the ticket_id
, user_id
and the actually comment
. Then send an email, if the user commenting is not the ticket owner (that is, if the comment was made by an admin, an email will be sent to the ticket owner). And finally display a status message.
postComment()
作用很简单; 确保已填充评论框,将注释与ticket_id
, user_id
和实际comment
一起存储在注释表中。 然后,如果用户评论不是票证所有者,则发送电子邮件(即,如果评论是由管理员进行的,则电子邮件将发送给票证所有者)。 最后显示状态信息。
You will notice that I used the relationships defined above extensively. Let me further explain the code snippet.
您会注意到,我广泛使用了上面定义的关系。 让我进一步解释代码片段。
// send mail if the user commenting is not the ticket owner
if ($comment->ticket->user->id !== Auth::user()->id) {
$mailer->sendTicketComments($comment->ticket->user, Auth::user(), $comment->ticket, $comment);
}
I check to see if the user id of the ticket that was commentted on is not equal to the authenticated user id. If it is (that is, ticket owner made the comment) there is no point sending an email to the user since he/she is the one that made the comment. If it is not (that is, an admin made the comment) an email is sent to the ticket owner.
我检查是否已注释的票证的用户ID不等于已验证的用户ID。 如果是(即,票证拥有者发表了评论),就没有必要向用户发送电子邮件,因为他/她是发表评论的人。 如果不是(即由管理员发表评论),则会将电子邮件发送给票证所有者。
评论表格 ( Comment Form )
Let's take a closer look at the comment box included in the show ticket view.
让我们仔细看一下显示票证视图中包含的评论框。
<div class="comment-form">
<form action="{{ url('comment') }}" method="POST" class="form">
{!! csrf_field() !!}
<input type="hidden" name="ticket_id" value="{{ $ticket->id }}">
<div class="form-group{{ $errors->has('comment') ? ' has-error' : '' }}">
<textarea rows="10" id="comment" class="form-control" name="comment"></textarea>
@if ($errors->has('comment'))
<span class="help-block">
<strong>{{ $errors->first('comment') }}</strong>
</span>
@endif
</div>
<div class="form-group">
<button type="submit" class="btn btn-primary">Submit</button>
</div>
</form>
</div>
The form will be POST
ed to comment
route which we are yet to create. Go on and add this to routes.php
该表格将被过POST
以comment
我们尚未创建的路线。 继续并将其添加到routes.php
Route::post('comment', 'CommentsController@postComment');
Now you should be able to comment on a ticket but even after commenting, you won't see the comment yet because we haven't updated the show view to display comments. First we need to update the show()
to also fetch comments and pass it to the view.
现在您应该可以对故障单发表评论了,但是即使发表评论,您也不会看到评论,因为我们还没有更新显示视图以显示评论。 首先,我们需要更新show()
来获取注释并将其传递给视图。
// TicketsController.php
public function show($ticket_id)
{
$ticket = Ticket::where('ticket_id', $ticket_id)->firstOrFail();
$comments = $ticket->comments;
$category = $ticket->category;
return view('tickets.show', compact('ticket', 'category', 'comments'));
}
Also update the show view, add the code below just after <hr>
to do just that.
还要更新显示视图,在<hr>
之后添加以下代码即可。
// resources/views/tickets/show.blade.php
<div class="comments">
@foreach ($comments as $comment)
<div class="panel panel-@if($ticket->user->id === $comment->user_id) {{"default"}}@else{{"success"}}@endif">
<div class="panel panel-heading">
{{ $comment->user->name }}
<span class="pull-right">{{ $comment->created_at->format('Y-m-d') }}</span>
</div>
<div class="panel panel-body">
{{ $comment->comment }}
</div>
</div>
@endforeach
</div>
发送评论电子邮件 ( Sending Comment Email )
Remember from part 1, we created a dedicated class called AppMailler
that will handle all emails that we will be sending? From the postComment()
in the CommentsController
, I called a sendTicketComments()
which does not exist yet, passing along some arguments. Now we will create this method. Open app/Mailers/AppMailer.php
and we will add the sendTicketComments()
just above the deliver()
.
还记得在第1部分中,我们创建了一个名为AppMailler
的专用类,该类将处理我们将发送的所有电子邮件吗? 从CommentsController
的postComment()
,我sendTicketComments()
尚不存在的sendTicketComments()
,并传递了一些参数。 现在,我们将创建此方法。 打开app/Mailers/AppMailer.php
,我们将在sendTicketComments()
上方添加sendTicketComments()
deliver()
。
// app/Mailers/AppMailer.php
public function sendTicketComments($ticketOwner, $user, Ticket $ticket, $comment)
{
$this->to = $ticketOwner->email;
$this->subject = "RE: $ticket->title (Ticket ID: $ticket->ticket_id)";
$this->view = 'emails.ticket_comments';
$this->data = compact('ticketOwner', 'user', 'ticket', 'comment');
return $this->deliver();
}
We need one more view file. This view file will be inside theemails
folder in the view directory. Go on and create ticket_comments.blade.php and add:
我们还需要一个视图文件。 该视图文件将位于视图目录中的emails
文件夹内。 继续并创建ticket_comments.blade.php并添加:
// resources/views/emails/ticket_comments.blade.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Suppor Ticket</title>
</head>
<body>
<p>
{{ $comment->comment }}
</p>
---
<p>Replied by: {{ $user->name }}</p>
<p>Title: {{ $ticket->title }}</p>
<p>Title: {{ $ticket->ticket_id }}</p>
<p>Status: {{ $ticket->status }}</p>
<p>
You can view the ticket at any time at {{ url('tickets/'. $ticket->ticket_id) }}
</p>
</body>
</html>
将票证标记为已关闭 ( Marking Ticket As Closed )
The last feature of the our Support Ticket application is the ability to mark ticket as closed. But a ticket can only be marked as closed by an admin. So we need to setup an admin. Remember from part 1, that when we created a our users
table, we created a column called is_admin
with a default value of 0 which indicate not an admin. A quick refreshener
我们的支持票证应用程序的最后一个功能是能够将票证标记为已关闭。 但是票证只能由管理员标记为已关闭。 因此,我们需要设置一个管理员。 请记住,从第1部分开始,当我们创建一个users
表时,我们创建了一个名为is_admin
的列,其默认值为0,该列表示不是admin。 快速恢复
$table->integer('is_admin')->unsigned()->default(0);
AdminMiddleware ( AdminMiddleware )
We are going to create an AdminMiddleware
which will allow only an admin access to an admin only area. Run the command below to create AdminMiddleware
我们将创建一个AdminMiddleware
,它将仅允许管理员访问仅管理员区域。 运行以下命令以创建AdminMiddleware
php artisan make:middleware AdminMiddleware
Update the handle()
with:
使用以下命令更新handle()
:
// AdminMiddleware.php
// Remember to add the line below at the top
// use Illuminate\Support\Facades\Auth;
public function handle($request, Closure $next)
{
if (Auth::user()->is_admin !== 1) {
return redirect('home');
}
return $next($request);
}
We need to register our newly created middleware. Open app/Http/Kernel.php
under the $routeMiddleware
array, and add the line below to it
我们需要注册我们新创建的中间件。 打开$routeMiddleware
数组下的app/Http/Kernel.php
,并在其下面添加以下行
'admin' => \App\Http\Middleware\AdminMiddleware::class,
Now that the admin
middleware has been registered, lets go on and create our admin specific routes. Add the following to routes.php
现在已经注册了admin
中间件,让我们继续创建我们的管理员专用路由。 将以下内容添加到routes.php
Route::group(['prefix' => 'admin', 'middleware' => 'admin'], function() {
Route::get('tickets', 'TicketsController@index');
Route::post('close_ticket/{ticket_id}', 'TicketsController@close');
});
The code above is pretty straightforward, define some routes not only an authenticated user can access but must also be an admin.
上面的代码非常简单,定义了一些路由,不仅经过身份验证的用户可以访问,而且还必须是管理员。
// TicketsController.php
public function index()
{
$tickets = Ticket::paginate(10);
$categories = Category::all();
return view('tickets.index', compact('tickets', 'categories'));
}
The first route /admin/tickets
will trigger the index()
on the TicketsController
which will get all the tickets that have been created. For the view file, create a file index.blade.php
in the tickets
folder and paste the code into it.
第一个路由/admin/tickets
将触发TicketsController
上的index()
,该TicketsController
将获取所有已创建的票证。 对于视图文件,在tickets
文件夹中创建一个文件index.blade.php
,并将代码粘贴到其中。
// resources/views/tickets/index.blade.php
@extends('layouts.app')
@section('title', 'All Tickets')
@section('content')
<div class="row">
<div class="col-md-10 col-md-offset-1">
<div class="panel panel-default">
<div class="panel-heading">
<i class="fa fa-ticket"> Tickets</i>
</div>
<div class="panel-body">
@if ($tickets->isEmpty())
<p>There are currently no tickets.</p>
@else
<table class="table">
<thead>
<tr>
<th>Category</th>
<th>Title</th>
<th>Status</th>
<th>Last Updated</th>
<th style="text-align:center" colspan="2">Actions</th>
</tr>
</thead>
<tbody>
@foreach ($tickets as $ticket)
<tr>
<td>
@foreach ($categories as $category)
@if ($category->id === $ticket->category_id)
{{ $category->name }}
@endif
@endforeach
</td>
<td>
<a href="{{ url('tickets/'. $ticket->ticket_id) }}">
#{{ $ticket->ticket_id }} - {{ $ticket->title }}
</a>
</td>
<td>
@if ($ticket->status === 'Open')
<span class="label label-success">{{ $ticket->status }}</span>
@else
<span class="label label-danger">{{ $ticket->status }}</span>
@endif
</td>
<td>{{ $ticket->updated_at }}</td>
<td>
<a href="{{ url('tickets/' . $ticket->ticket_id) }}" class="btn btn-primary">Comment</a>
</td>
<td>
<form action="{{ url('admin/close_ticket/' . $ticket->ticket_id) }}" method="POST">
{!! csrf_field() !!}
<button type="submit" class="btn btn-danger">Close</button>
</form>
</td>
</tr>
@endforeach
</tbody>
</table>
{{ $tickets->render() }}
@endif
</div>
</div>
</div>
</div>
@endsection
The second route close_ticket/ticket_id
will trigger the close()
on the ticketsController
. The method will handle closing a particular ticket.
第二路线close_ticket/ticket_id
将触发close()
在ticketsController
。 该方法将处理关闭特定票证。
// TicketsController.php
public function close($ticket_id, AppMailer $mailer)
{
$ticket = Ticket::where('ticket_id', $ticket_id)->firstOrFail();
$ticket->status = 'Closed';
$ticket->save();
$ticketOwner = $ticket->user;
$mailer->sendTicketStatusNotification($ticketOwner, $ticket);
return redirect()->back()->with("status", "The ticket has been closed.");
}
We get the ticket with the ticket_id passed from the route, and set it status to Closed
and save it. Then send an email by calling sendTicketStatusNotification()
(which we will create shortly) on the AppMailer
class. And finally display a status message.
我们获得从路线传递的具有ticket_id的票证,并将其状态设置为“ Closed
并保存。 然后通过在AppMailer
类上调用sendTicketStatusNotification()
(稍后将创建)来发送电子邮件。 最后显示状态信息。
发送故障单状态通知 ( Sending Ticket Status Notification )
Add the code below to app/Mailers/AppMailer.php
just above the deliver()
将以下代码添加到app/Mailers/AppMailer.php
deliver()
上方
// app/Mailers/AppMailer.php
public function sendTicketStatusNotification($ticketOwner, Ticket $ticket)
{
$this->to = $ticketOwner->email;
$this->subject = "RE: $ticket->title (Ticket ID: $ticket->ticket_id)";
$this->view = 'emails.ticket_status';
$this->data = compact('ticketOwner', 'ticket');
return $this->deliver();
}
We need one more view file. This view file will be inside theemails
folder in the view directory. Go on and create ticket_status.blade.php and add:
我们还需要一个视图文件。 该视图文件将位于视图目录中的emails
文件夹内。 继续并创建ticket_status.blade.php并添加:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Suppor Ticket Status</title>
</head>
<body>
<p>
Hello {{ ucfirst($ticketOwner->name) }},
</p>
<p>
Your support ticket with ID #{{ $ticket->ticket_id }} has been marked has resolved and closed.
</p>
</body>
</html>
只允许经过身份验证的用户访问 ( Allowing Only Authenticated Users Access )
Only authenticated users can use our Support Ticket Application. Laravel has us covered by providing Authenticate
middleware which will allow only authenticated users access to the routes in which the auth
middleware is added to. We will be add the auth
middleware to TicketsController
contructor:
只有经过身份验证的用户才能使用我们的支持票证申请。 Laravel通过提供Authenticate
中间件为我们提供了覆盖,该中间件将仅允许经过身份验证的用户访问将auth
中间件添加到的路由。 我们将auth
中间件添加到TicketsController
构造器中:
// TicketsController.php
public function __construct()
{
$this->middleware('auth');
}
This way, all the methods in TicketsController
will only be accessible by authenticated users.
这样, TicketsController
所有方法将仅由经过身份验证的用户访问。
画龙点睛 ( Finishing Touches )
When we ran the make:auth
command in part 1, Laravel created some scaffolding for us which we are going to make use of. A HomeController
and a home.
view. We'll be making some few changes to the home
view. Update the home
view with:
当我们在第1部分中运行make:auth
命令时,Laravel为我们创建了一些脚手架,我们将利用它们。 一个HomeController
和一个home.
视图。 我们将对home
视图进行一些更改。 使用以下命令更新home
视图:
@extends('layouts.app')
@section('title', 'Dashboard')
@section('content')
<div class="container">
<div class="row">
<div class="col-md-10 col-md-offset-1">
<div class="panel panel-default">
<div class="panel-heading">Dashboard</div>
<div class="panel-body">
<p>You are logged in!</p>
@if (Auth::user()->is_admin)
<p>
See all <a href="{{ url('admin/tickets') }}">tickets</a>
</p>
@else
<p>
See all your <a href="{{ url('my_tickets') }}">tickets</a> or <a href="{{ url('new_ticket') }}">open new ticket</a>
</p>
@endif
</div>
</div>
</div>
</div>
</div>
@endsection
结论 ( Conclusion )
That's it. Our Support Ticket Application is ready. You can check out the demo and also the project repository to view the complete source code. If you have any questions about the tutorial, let me know in the comments below
而已。 我们的支持票申请已准备就绪。 您可以签出演示以及项目存储库,以查看完整的源代码。 如果您对本教程有任何疑问,请在下面的评论中告诉我
翻译自: https://scotch.io/tutorials/build-a-support-ticket-application-with-laravel-part-2
票务小程序源码