vue 构建单页应用
Vue.js is simple. It is so simple that people often dismiss it as only suitable for small projects. While it is true the Vue.js core is just a view layer library, there are in fact a collection of tools that will enable you to build full-blown, large-scale SPAs (Single Page Applications) using Vue.js with a pleasant development experience.
Vue.js很简单。 它是如此简单,以至于人们经常认为它仅适用于小型项目。 虽然确实Vue.js核心只是一个视图层库,但实际上有一系列工具可让您使用Vue.js轻松构建功能完善的大型SPA(单页应用程序)开发经验。
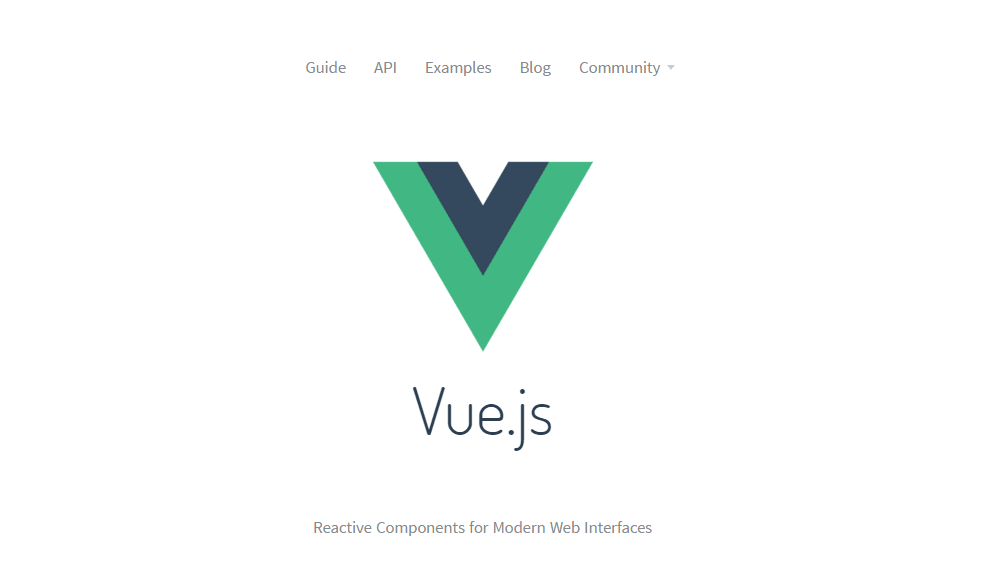
If you are already familiar with the basics of Vue.js but feel that the world of SPA is scary, this series is for you. We will first introduce the concepts, tools and libraries needed in this first article, and then will walk you through the full process of building an example app in the rest of the series.
如果您已经熟悉Vue.js的基础知识,但是觉得SPA的世界令人恐惧,那么本系列适合您。 我们将首先介绍第一篇文章中所需的概念,工具和库,然后将在本系列的其余部分中逐步引导您构建示例应用程序的全过程。
第一件事:什么是SPA? ( First Thing's First: What is a SPA? )
Single-Page Applications (SPAs) are web apps that load a single HTML page and dynamically update that page as the user interacts with the app. SPAs use AJAX and HTML5 to create fluid and responsive Web apps, without constant page reloads.
单页应用程序(SPA)是可加载单个HTML页面并在用户与应用程序交互时动态更新该页面的Web应用程序。 SPA使用AJAX和HTML5创建流畅且响应Swift的Web应用程序,而无需不断刷新页面。
As stated in the above description taken from Wikipedia, the main advantage of SPAs is that the app can respond to user interactions without fully reloading the page, resulting in a much more fluid user experience.
正如从Wikipedia摘录的上述说明所述,SPA的主要优势在于,该应用程序可以响应用户交互而无需完全重新加载页面,从而带来更加流畅的用户体验 。
As a nice side effect, a SPA also encourages the backend to focus on exposing data endpoints, which makes the overall architecture more decoupled and potentially reusable for other types of clients.
作为一个很好的副作用,SPA还鼓励后端将重点放在公开数据端点上,这使整个体系结构更加分离,并有可能被其他类型的客户端重用。
From the developer's perspective, the main difference between SPAs and a traditional backend-rendered app is that we have to treat the client side as an application with its own structure. Typically we will need to handle routing, data fetching and persistence, view rendering and the necessary build setup to facilitate a modularized codebase.
从开发人员的角度来看,SPA与传统的后端呈现的应用程序之间的主要区别在于,我们必须将客户端视为具有自己结构的应用程序。 通常,我们将需要处理路由,数据获取和持久性,视图渲染以及必要的构建设置,以促进模块化代码库的发展。
积木 ( The Building Blocks )
For a Vue.js-based SPA, here are the tools and libraries that we will use to fill in these gaps:
对于基于Vue.js的SPA,以下是我们将用来填补这些空白的工具和库:
View Layer: Vue.js, of course :)
视图层 :Vue.js,当然是:)
Routing: vue-router, the official router for Vue.js.
路由 : vue-router ,Vue.js的官方路由器。
State Management: vuex, a state-management solution inspired by Flux/Redux, but designed specifically for Vue.
状态管理 : vuex ,一种受Flux / Redux启发的状态管理解决方案,但专门为Vue设计。
Server Communication: vue-resource, plugin for interfacing with a RESTful backend.
服务器通信 : vue-resource ,用于与RESTful后端接口的插件。
Build Tool: Webpack + vue-loader for modules, hot-reloading, ES2015, pre-processors and most importantly, single-file Vue components.
构建工具 : Webpack + vue-loader,用于模块,热重装,ES2015,预处理器,最重要的是单文件Vue组件。
Let's take a closer look at each part.
让我们仔细看看每个部分。
视图层 (The View Layer)
This series assumes you are already familiar with the basics of Vue.js. If you are not, you should be able to quickly pick it up by going through official guide and other tutorials available.
本系列假定您已经熟悉Vue.js的基础知识。 如果不是这样,您应该可以通过阅读官方指南和其他可用教程来快速进行选择。
The core concept when using Vue.js for large SPAs is dividing your application into many nested, self-contained components. We also want to carefully design how these components interact with one another by leveraging component props for the data flow and custom events for communication. By doing so, we dissect the complexity into small, decoupled units that are tremendously easier to maintain.
将Vue.js用于大型SPA时的核心概念是将您的应用程序划分为许多嵌套的独立组件。 我们还想通过利用组件道具的数据流和自定义事件进行通信来仔细设计这些组件之间如何交互。 通过这样做,我们将复杂性分解为小型,解耦的单元,这些单元非常容易维护。
路由 (Routing)
The official vue-router library handles client side routing, and supports both hash mode and HTML5 history mode. It is a bit different from standalone routing libraries in that it deeply integrates with Vue.js and makes the assumption that we are mapping nested routes to nested Vue components.
官方的vue-router库处理客户端路由,并支持哈希模式和HTML5历史记录模式。 它与独立路由库有点不同,因为它与Vue.js进行了深度集成,并假设我们将嵌套路由映射到嵌套Vue组件。
When using vue-router, we implement components that serve as "pages", and within these components we can implement hook functions that are called when the route changes.
使用vue-router时,我们实现了充当“ 页面 ”的组件,并且在这些组件中,我们可以实现在路由更改时调用的挂钩函数。
国家管理 (State Management)
State management is a topic that only arises when your application's complexity grows beyond a certain level. When you have multiple components that need to share and mutate application state, it can get very hard to reason about and maintain if you don't have a layer in your application that is dedicated to managing such shared state.
状态管理是仅在您的应用程序的复杂性超过一定水平时出现的主题。 当您有多个需要共享和更改应用程序状态的组件时,如果您的应用程序中没有专门用于管理此类共享状态的层,则很难进行推理和维护。
This is where Vuex comes in. You don't necessarily need Vuex if you application is relatively simple - but if you are interested, here's an excellent intro on what problem it solves by Anirudh Sanjeev.
这是Vuex出现的地方。如果您的应用程序相对简单,则不一定需要Vuex-但是,如果您有兴趣,这里有Anirudh Sanjeev解决了什么问题的精彩介绍 。
服务器通讯 (Server Communication)
We will be working with a RESTful backend in the example, so we are using the vue-resource plugin which is maintained by the PageKit team. Do note that Vue.js SPAs are backend-agnostic and can basically work with any data fetching solution you prefer, for example fetch, restful.js, Firebase or even Falcor.
在示例中,我们将使用RESTful后端,因此我们将使用由PageKit团队维护的vue-resource插件。 请注意,Vue.js SPA与后端无关,并且基本上可以与您喜欢的任何数据获取解决方案一起使用,例如fetch , restful.js , Firebase甚至Falcor 。
制作工具 (Build Tool)
This is probably the biggest hurdle that you'll have to jump through if you are not familiar with the frontend build tool scene, and we will try to explain it here. Feel free to skip this section if you are already experienced with Webpack.
如果您不熟悉前端构建工具场景,这可能是您必须克服的最大障碍,我们将在这里尝试进行解释。 如果您已经对Webpack有所了解,请随时跳过本节。
First, the entire build tool chain relies on Node.js, and we will be managing all our library and tool dependencies using NPM. Although NPM started out as the package manager for Node.js backend modules, it is now widely used for frontend package management too. Because all NPM packages are authored using the CommonJS module format, we need special tooling to "bundle" these modules into files that are suitable for final deployment. Webpack is exactly such a tool, and you may have also heard of a similar tool called Browserify.
首先,整个构建工具链依赖于Node.js ,我们将使用NPM管理所有库和工具依赖项。 尽管NPM最初是作为Node.js后端模块的软件包管理器,但现在它也广泛用于前端软件包管理。 因为所有NPM软件包都是使用CommonJS模块格式编写的,所以我们需要特殊的工具将这些模块“捆绑”到适合最终部署的文件中。 Webpack正是这样的工具,您可能还听说过类似的工具Browserify。
We will be using Webpack for the series because it provides more advanced functionalities out of the box, such as hot-reloading, bundle-splitting and static asset handling.
我们将在该系列中使用Webpack,因为它提供了开箱即用的更多高级功能,例如热重装,捆绑拆分和静态资产处理。
Both Webpack and Browserify exposes APIs that allows us to load more than just CommonJS modules: for example, we can directly require()
an HTML file by transforming it into a JavaScript string.
Webpack和Browserify都公开了API,它们使我们不仅可以加载CommonJS模块:例如,我们可以通过将HTML文件转换为JavaScript字符串来直接require()
HTML文件。
By treating everything for your frontend including HTML, CSS and even image files as module dependencies that can be arbitrarily transformed during the bundling process, Webpack actually covers most of the build tasks that you will encounter when building a SPA. We are primarily going to build the example using Webpack and plain NPM scripts, without the need for a task runner like Gulp or Grunt.
通过将前端的所有内容(包括HTML,CSS甚至图像文件)视为模块依赖关系,可以在捆绑过程中任意转换这些内容,Webpack实际上涵盖了构建SPA时将遇到的大多数构建任务。 我们主要将使用Webpack和普通的NPM脚本来构建示例,而不需要像Gulp或Grunt这样的任务运行器。
We will also be using vue-loader which enables us to author Vue components in a single file:
我们还将使用vue-loader ,它使我们能够在单个文件中创作Vue组件:
// app.vue<template>
<h1 class="red">{{msg}}</h1>
</template>
<script>
export default {
data () {
return {
msg: 'Hello world!'
}
}
}
</script>
<style>
.red {
color: #f00;
}
</style>
In addition, the combination of Webpack and vue-loader
gives us:
另外,Webpack和vue-loader
为我们提供了:
ES2015 by default. This allows us to use future JavaScript syntax today, which results in more expressive and concise code.
默认为ES2015 。 这使我们能够在今天使用将来JavaScript语法,从而使代码更具表达力和简洁性。
Embedded pre-processors. You can use your pre-processors of choice inside single-file Vue components, for example using Jade for the template and SASS for the styles.
嵌入式预处理器 。 您可以在单文件Vue组件中使用选择的预处理器,例如,使用Jade作为模板,使用SASS作为样式。
CSS output inside Vue components are autoprefixed. You can also use any PostCSS plugins you like.
Vue组件内部CSS输出是自动前缀的 。 您也可以使用任何喜欢的PostCSS插件。
Scoped CSS. By adding a
scoped
attribute to the<style>
, vue-loader will simulate scoped CSS by rewriting the template and style output so that the CSS for a specific component will not affect other parts of your app.范围CSS 。 通过向
<style>
添加scoped
属性,vue-loader将通过重写模板和样式输出来模拟范围CSS,以使特定组件CSS不会影响应用程序的其他部分。Hot Reload. When editing a Vue component during development, the component will be "hot swapped" into the running app, maintaining the app state without having the reload the page. This greatly improves the development experience.
热装 。 在开发过程中编辑Vue组件时,该组件将被“热交换”到正在运行的应用程序中,从而保持应用程序状态而无需重新加载页面。 这大大改善了开发经验。
设置 ( Setting it Up )
Now with all these fancy features, it could be a really daunting task to assemble the build stack yourself! Luckily, Vue provides vue-cli
, a command-line interface that makes it trivially easy to get started:
现在有了所有这些精美的功能,亲自组装构建堆栈可能是一项艰巨的任务! 幸运的是,Vue提供了vue-cli
,这是一个命令行界面,使入门变得非常容易:
npm install -g vue-cli
vue init webpack my-project
Answer the prompts, and the CLI will scaffold a project with all the aforementioned features working out of the box. All you need to do next is:
回答提示,CLI将为一个具有上述所有功能的项目提供方便。 接下来您需要做的是:
cd my-project
npm install # install dependencies
npm run dev # start dev server at http://localhost:8080
For full details on what is included in the generated project, check out the project template documentation.
有关所生成项目中包含的内容的完整详细信息,请查看项目模板文档 。
下一个... ( Next... )
We haven't really written any real app code so far, but I hope we have got you excited about learning more. In the next article, Ryan Chenkie will start taking us through a series of building a full-fledged SPA using this stack. Stay tuned!
到目前为止,我们还没有真正编写任何真正的应用程序代码,但我希望我们对您有更多的了解感到兴奋。 在下一篇文章中, Ryan Chenkie将开始带领我们完成一系列使用此堆栈构建完整的SPA的过程。 敬请关注!
翻译自: https://scotch.io/tutorials/build-a-single-page-time-tracking-app-with-vue-js-introduction
vue 构建单页应用