mootools
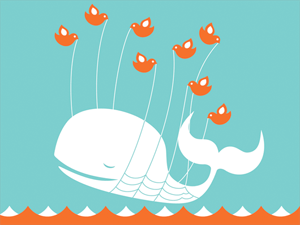
Twitter does some great stuff with JavaScript. What I really appreciate about what they do is that there aren't any epic JS functionalities -- they're all simple touches. One of those simple touches is the "Login" dropdown on their homepage. I've taken some time to duplicate that functionality with MooTools.
Twitter在JavaScript方面做得很棒。 我对它们的功能真正感到赞赏的是,它没有任何史诗般的JS功能-它们都是简单的操作。 这些简单的操作之一就是其主页上的“登录”下拉菜单。 我花了一些时间来复制MooTools的功能。
HTML (The HTML)
<div id="menu1"><div class="relative">
<a href="/demos" title="Popular MooTools Tutorials" id="dd1" class="dropdown" style="width:170px;text-decoration:none;"><span>Menu 1</span></a>
<div id="dropdown1" class="dropdown-menu">
<a href="/about-david-walsh" title="Learn a bit about me.">About Me</a>
<a href="/page/1" title="The David Walsh Blog">Blog</a>
<a href="/chat" title="#davidwalshblog IRC Chat">Chat</a>
<a href="/contact" title="Contact David Walsh">Contact Me</a>
<a href="/demos" title="CSS, PHP, jQuery, MooTools Demos">Demos & Downloads</a>
<a href="/js" title="ScrollSpy, Lazyload, Overlay, Context Menu">MooTools Plugins</a>
<a href="/network" title="David Walsh Blog, Script & Style, Band Website Template, Wynq">Network</a>
<a href="/web-development-tools" title="JS, CSS Compression">Web Dev Tools</a>
</div>
</div></div>
<div id="menu2"><div class="relative">
<a href="/demos" title="Popular MooTools Tutorials" id="dd2" class="dropdown" rel="dropdown2" style="width:170px;text-decoration:none;"><span>Menu 2</span></a>
<div id="dropdown2" class="dropdown-menu">
<p>Pellentesque habitant morbi tristique senectus et netus et malesuada fames ac turpis egestas. Vestibulum tortor quam, feugiat vitae, ultricies eget, tempor sit amet, ante. Donec eu libero sit amet quam egestas semper. Aenean ultricies mi vitae est. Mauris placerat eleifend leo.</p>
</div>
</div></div>
A series of DIVS wrapping a link (the dropdown "trigger") and a DIV containing the menu items.
一系列DIVS包装了一个链接(下拉“触发器”)和一个包含菜单项的DIV。
CSS (The CSS)
/* dropdowns: general */
a.dropdown { background: #88bbd4; padding: 4px 6px 6px; text-decoration: none; font-weight: bold; color: #fff; -webkit-border-radius: 4px; -moz-border-radius: 4px; border-radius: 4px; }
a.dropdown:hover { background: #59b; }
a.dropdown { position: relative; margin-left: 3px; }
a.dropdown span { background-image: url(toggle_down_light.png); background-repeat: no-repeat; background-position: 100% 50%; padding: 4px 16px 6px 0; }
a.dropdown.dropdown-active { color:#59b; background-color:#ddeef6; }
a.dropdown.dropdown-active span { background:url(toggle_up_dark.png) 100% 50% no-repeat; }
.dropdown-menu { background:#ddeef6; padding:7px 12px; position:absolute; top:16px; right:0; display:none; z-index:5000; -moz-border-radius-topleft: 5px; -moz-border-radius-bottomleft: 5px; -moz-border-radius-bottomright: 5px; -webkit-border-top-left-radius: 5px; -webkit-border-bottom-left-radius: 5px; -webkit-border-bottom-right-radius: 5px; }
.dropdown-menu p { font-size:11px; }
.dropdown-menu a:link, .dropdown-menu a:visited { font-weight:bold; color:#59b; text-decoration:none; line-height:1.7em; }
.dropdown-menu a:active, .dropdown-menu a:hover { color:#555; }
/* dropdowns: specific */
#menu1 { float:left; margin-right:20px; }
#dropdown1 { width:150px; }
#dropdown1 a { display:block; }
#menu2 { float:left; }
#dropdown2 { width:150px; font-size:11px; }
.relative { position:relative; }
There's a lot of CSS involved but most of it is simple visual styling as opposed to styling for JavaScript's sake. Do, however, note where relative and absolute positioning is used. The outermost DIV may be positioned absolutely if you'd like. Also note that I'm not doing anything to accommodate for rounded corners in IE -- I recommend DD_Roundies for that.
CSS涉及很多,但是大多数都是简单的视觉样式,而不是出于JavaScript的目的。 但是,请注意使用相对和绝对定位的位置。 如果您愿意,可以将最外面的DIV放在绝对位置。 另请注意,我没有做任何事情来容纳IE中的圆角-为此,我建议使用DD_Roundies 。
MooTools JavaScript (The MooTools JavaScript)
window.addEvent('domready',function() {
(function($) {
/* for keeping track of what's "open" */
var activeClass = 'dropdown-active', showingDropdown, showingMenu, showingParent;
/* hides the current menu */
var hideMenu = function() {
if(showingDropdown) {
showingDropdown.removeClass(activeClass);
showingMenu.setStyle('display','none');
}
};
/* recurse through dropdown menus */
$$('.dropdown').each(function(dropdown) {
/* track elements: menu, parent */
var menu = dropdown.getNext('div.dropdown-menu'), parent = dropdown.getParent('div');
/* function that shows THIS menu */
var showMenu = function() {
hideMenu();
showingDropdown = dropdown.addClass('dropdown-active');
showingMenu = menu.setStyle('display','block');
showingParent = parent;
};
/* function to show menu when clicked */
dropdown.addEvent('click',function(e) {
if(e) e.stop();
showMenu();
});
/* function to show menu when someone tabs to the box */
dropdown.addEvent('focus',function() {
showMenu();
});
});
/* hide when clicked outside */
$(document.body).addEvent('click',function(e) {
if(showingParent && !e.target || !$(e.target).getParents().contains(showingParent)) {
hideMenu();
}
});
})(document.id);
});
I've commented to the code to illustrate what each block does. In a nutshell:
我对代码进行了注释,以说明每个块的功能。 简而言之:
I create placeholder variables which will keep track of the current menu, dropdown, and parent for the opened menu. This functionality is only included because I don't want more than one menu to be open at a time.
我创建了占位符变量,这些变量将跟踪当前菜单,下拉菜单和打开菜单的父菜单。 仅包含此功能是因为我不想一次打开多个菜单。
- I create a function that hides the current menu -- this can be used from anywhere within the closure. 我创建了一个隐藏当前菜单的函数-可以在闭包内的任何位置使用它。
- I cycle through each dropdown and add events to relevant elements to show and hide menus. 我循环浏览每个下拉菜单,并将事件添加到相关元素以显示和隐藏菜单。
- I add an event to the body to close the current menu if the user clicks outside of the menu. 如果用户在菜单外单击,则会向主体添加一个事件以关闭当前菜单。
That's it!
而已!
Look forward to a MooTools Class version soon. Also look forward to jQuery and Dojo versions!
期待很快有MooTools类的版本。 也期待jQuery和Dojo版本!
mootools