mootools 元素追加
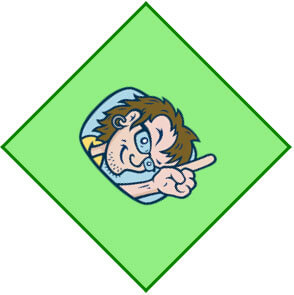
I was recently perusing the MooTools Forge and I saw a neat little plugin that allows for static element rotation: Fx.Rotate. Fx.Rotate is an extension of MooTools' native Fx class and rotates the element via CSS within each A-grade browser it supports. Let's examine how you can easily incorporate animated element rotation within your website.
最近,我正在仔细阅读MooTools Forge,我看到了一个简洁的小插件,允许静态元素旋转: Fx.Rotate 。 Fx.Rotate是MooTools原生Fx类的扩展,并通过CSS在支持的每个A级浏览器中旋转元素。 让我们研究一下如何轻松地将动画元素旋转合并到网站中。
MooTools JavaScript (The MooTools JavaScript)
How you use the plugin and when you instantiate the Fx.Rotate class is up to you. For the sake of this being an introduction to the class, we'll keep it simple and make the element rotate when a button is clicked:
如何使用插件以及何时实例化Fx.Rotate类由您决定。 为了使这成为该类的简介,我们将使其保持简单,并在单击按钮时使元素旋转:
window.addEvent('domready',function() {
var rotate = new Fx.Rotate('rotate-me',{duration:'long'});
document.id('do-rotate').addEvent('click',function() {
rotate.start(0,45); //showtime!
});
});
When the DOM is set, we assign a click listener to the button which rotates the element from 0 degrees (its original state) to 45 degrees. You can probably already tell that the start method is the animator. The class is super small because it extends Fx; Fx is where the animation logic is housed.
设置DOM后,我们为按钮分配一个点击侦听器,该按钮会将元素从0度(其原始状态)旋转到45度。 您可能已经知道start方法是动画师。 该类非常小,因为它扩展了Fx。 Fx是放置动画逻辑的位置。
MooTools类 (The MooTools Class)
I cannot say that the code, as it's presently available, is up to MooTools' standards:
我不能说该代码(目前可用)符合MooTools的标准:
(function (window,undef){
var deg2radians = Math.PI * 2 / 360
, prefix = "";
function getMatrix(deg){
rad = deg * deg2radians ;
costheta = Math.cos(rad);
sintheta = Math.sin(rad);
a = parseFloat(costheta).toFixed(8);
c = parseFloat(-sintheta).toFixed(8);
b = parseFloat(sintheta).toFixed(8);
d = parseFloat(costheta).toFixed(8);
return [a,b,c,d];
}
if (Browser.Engine.gecko) prefix = 'moz';
if (Browser.Engine.webkit) prefix = 'webkit';
if (Browser.Engine.presto) prefix = 'o';
Fx.Rotate = new Class({
Extends : Fx
, element : null
, initialize : function(el,options){
this.element = $(el);
this.element.setStyle("-"+prefix+"-transform-origin","center center");
if (Browser.Engine.trident){
this.element.setStyle("filter","progid:DXImageTransform.Microsoft.Matrix(sizingMethod='auto expand')");
}
this.parent(options);
}
, start : function(from,to){
this.parent(from,to);
}
, set : function (current){
if (Browser.Engine.trident) current *=-1;
var matrix = getMatrix(current);
if (Browser.Engine.trident){
this.element.filters.item(0).M11 = matrix[0];
this.element.filters.item(0).M12 = matrix[1];
this.element.filters.item(0).M21 = matrix[2];
this.element.filters.item(0).M22 = matrix[3];
}else{
this.element.setStyle("-"+prefix+"-transform","matrix("+matrix[0]+","+matrix[1]+","+matrix[2]+", "+matrix[3]+", 0, 0)");
}
}
});
})(this);
While this code works (and that's what's most important), I'd prefer to see the class look like this:
尽管此代码有效(这是最重要的),但我更希望看到该类如下所示:
Fx.Rotate = new Class({
Extends: Fx,
initialize: function(element,options) {
this.element = document.id(element);
this.prefix = (Browser.Engine.gecko ? 'moz' : (Browser.Engine.webkit ? 'webkit' : 'o'));
this.radions = Math.PI * 2 / 360;
if(Browser.Engine.trident) { // IE
this.element.setStyle('filter','progid:DXImageTransform.Microsoft.Matrix(sizingMethod="auto expand")');
}
else {
this.element.setStyle('-' + this.prefix + '-transform-origin','center center');
}
this.parent(options);
},
start: function(from,to) {
this.parent(from,to);
},
set: function(current) {
if (Browser.Engine.trident) current *=-1;
var matrix = getMatrix(current);
if (Browser.Engine.trident){
this.element.filters.item(0).M11 = matrix[0];
this.element.filters.item(0).M12 = matrix[1];
this.element.filters.item(0).M21 = matrix[2];
this.element.filters.item(0).M22 = matrix[3];
}else{
this.element.setStyle('-' + this.prefix + '-transform','matrix(' + matrix[0] + ',' + matrix[1] + ',' + matrix[2] + ', ' + matrix[3] + ', 0, 0)');
}
},
getMatrix: function(deg) {
var rad = deg * (this.radions), costheta = Math.cos(rad), sintheta = Math.sin(rad);
var a = parseFloat(costheta).toFixed(8),
c = parseFloat(-sintheta).toFixed(8),
b = parseFloat(sintheta).toFixed(8),
d = parseFloat(costheta).toFixed(8);
return [a,b,c,d];
}
});
Just a bit of reorganization; no real change of logic. Mine is a bit prettier -- at that cost is the re-check of logic for each instance but the processing to do that is so negligible that I'm not concerned about it.
只是一些重组; 逻辑没有真正的改变。 我的有点漂亮-这样做的代价是每个实例的逻辑重新检查,但是这样做的过程可忽略不计,以至于我不关心它。
While it's a tad hard to think of an "every day, practical" use of this class, I think that this snippet has its merits and would probably work out great for some purposes of someone's website! Also, take a trip down memory lane and check out Fx.Explode!
虽然很难想到该类的“日常实用”用法,但我认为此片段有其优点,并且对于某人的网站的某些目的而言可能会非常有用! 另外,沿着内存通道旅行并检查Fx.Explode!
mootools 元素追加