mootools
CSS sprites are all the rage these days. And why shouldn't be? They're easy to implement, have great upside, and usually take little effort to create. Dave Shea wrote an epic CSS sprites navigation post titled CSS Sprites2 - It's JavaScript Time. In his post he outlined a method for enhancing the CSS sprite menu with jQuery. I loved his post so much that I converted his jQuery CSS sprites menu to MooTools.
如今,CSS精灵风行一时。 而为什么不应该呢? 它们易于实现,具有很大的优势,并且通常只需很少的努力即可创建。 Dave Shea撰写了史诗般CSS Sprites导航文章,标题为CSS Sprites2-It's JavaScript Time 。 在他的文章中,他概述了使用jQuery增强CSS Sprite菜单的方法。 我非常喜欢他的帖子,以至于将他的jQuery CSS sprites菜单转换为MooTools。
精灵图片 (The Sprite Image)
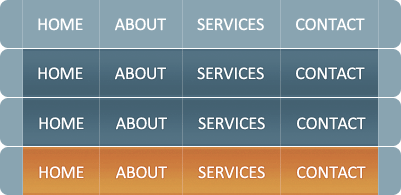
This is a great example of an efficient, useful sprite image.
这是高效,有用的Sprite图片的一个很好的例子。
HTML (The HTML)
<ul id="nav">
<li id="home" <?php echo $current == '' || $current == 'home' ? 'class="current"' : ''; ?>><a href="?home">Home</a></li>
<li id="about" <?php echo $current == 'about' ? 'class="current"' : ''; ?>><a href="?about">About</a></li>
<li id="services" <?php echo $current == 'services' ? 'class="current"' : ''; ?>><a href="?services">Services</a></li>
<li id="contact" <?php echo $current == 'contact' ? 'class="current"' : ''; ?>><a href="?contact">Contact</a></li>
</ul>
My code mostly mirrors the original ALA post's code but I've chose to use IDs instead of CSS classes.
我的代码主要是原始ALA帖子的代码,但我选择使用ID代替CSS类。
CSS (The CSS)
#nav { width: 401px; height: 48px; background: url(sprites-blue-nav.gif) no-repeat; position: absolute; top: 100px; left: 100px; padding:0; }
#nav li { display: inline; }
#nav li a:link, #nav li a:visited { position: absolute; top: 0; height: 48px; text-indent: -9000px; overflow: hidden; z-index: 10; }
#home a:link, #home a:visited { left: 23px; width: 76px; }
#home a:hover, #home a:focus { background: url(sprites-blue-nav.gif) no-repeat -23px -49px; }
#home a:active { background: url(sprites-blue-nav.gif) no-repeat -23px -98px; }
#home.current a:link, #home.current a:visited { background: url(sprites-blue-nav.gif) no-repeat -23px -147px; cursor: default; }
.nav-home, .nav-home-click { position: absolute; top: 0; left: 23px; width: 76px; height: 48px; background: url(sprites-blue-nav.gif) no-repeat -23px -49px; }
.nav-home-click { background: url(sprites-blue-nav.gif) no-repeat -23px -98px; }
#about a:link, #about a:visited { left: 100px; width: 82px; }
#about a:hover, #about a:focus { background: url(sprites-blue-nav.gif) no-repeat -100px -49px; }
#about a:active { background: url(sprites-blue-nav.gif) no-repeat -100px -98px; }
#about.current a:link, #about.current a:visited { background: url(sprites-blue-nav.gif) no-repeat -100px -147px; cursor: default; }
.nav-about, .nav-about-click { position: absolute; top: 0; left: 100px; width: 82px; height: 48px; background: url(sprites-blue-nav.gif) no-repeat -100px -49px; }
.nav-about-click { background: url(sprites-blue-nav.gif) no-repeat -100px -98px; }
#services a:link, #services a:visited { left: 183px; width: 97px; }
#services a:hover, #services a:focus { background: url(sprites-blue-nav.gif) no-repeat -183px -49px; }
#services a:active { background: url(sprites-blue-nav.gif) no-repeat -183px -98px; }
#services.current a:link, #services.current a:visited { background: url(sprites-blue-nav.gif) no-repeat -183px -147px; cursor: default; }
.nav-services, .nav-services-click { position: absolute; top: 0; left: 183px; width: 97px; height: 48px; background: url(sprites-blue-nav.gif) no-repeat -183px -49px; }
.nav-services-click { background: url(sprites-blue-nav.gif) no-repeat -183px -98px; }
#contact a:link, #contact a:visited { left: 281px; width: 97px; }
#contact a:hover, #contact a:focus { background: url(sprites-blue-nav.gif) no-repeat -281px -49px; }
#contact a:active { background: url(sprites-blue-nav.gif) no-repeat -281px -98px; }
#contact.current a:link, #contact.current a:visited { background: url(sprites-blue-nav.gif) no-repeat -281px -147px; cursor: default; }
.nav-contact, .nav-contact-click { position: absolute; top: 0; left: 281px; width: 97px; height: 48px; background: url(sprites-blue-nav.gif) no-repeat -281px -49px; }
.nav-contact-click { background: url(sprites-blue-nav.gif) no-repeat -281px -98px; }
Unfortunately there's plenty of CSS. That's generally the one downside of using sprites but the payoff comes with less requests to the server. My CSS differs from the original ALA post in that I accommodate for my IDs.
不幸的是,有很多CSS。 这通常是使用Sprite的缺点之一,但带来的好处是对服务器的请求减少。 我CSS与原始ALA帖子的不同之处在于,我可以容纳我的ID。
MooTools JavaScript (The MooTools JavaScript)
(function($) {
window.addEvent('domready',function() {
$('nav').getElements('li').each(function(li) {
//settings
var link = li.getFirst('a');
//fix background image
if(!li.hasClass('current')) {
link.setStyle('background-image','none');
}
//utility div
var div = new Element('div',{
'class': 'nav-' + li.get('id'),
opacity: 0
}).inject(li);
//background imagery
li.addEvents({
mouseenter: function() {
div.fade('in');
},
mouseleave: function() {
div.fade('out');
},
mousedown: function() {
div.addClass('nav-' + li.get('id') + '-click');
},
mouseup: function() {
div.removeClass('nav-' + li.get('id') + '-click');
}
});
});
});
})(document.id);
My MooTools version is less code and slightly more efficient as I'm not creating and disposing of the same DIVs over and over as the user hovers over each menu item. The menu has original, selected, hovered, and mousedown states. Awesome!
我的MooTools版本代码更少,效率更高一些,因为当用户将鼠标悬停在每个菜单项上时,我不会一遍又一遍地创建和处理相同的DIV。 菜单具有原始,选定,悬停和鼠标按下状态。 太棒了!
Do you use sprites for any of your menus? Share a link to a few -- I'd love to see what you've done!
您是否将精灵用于任何菜单? 分享一个链接-我很想看看你做了什么!
mootools