jframe滑动窗口
A graphical user interface starts with a top-level container which provides a home for the other components of the interface, and dictates the overall feel of the application. In this tutorial, we introduce the JFrame class, which is used to create a simple top-level window for a Java application.
图形用户界面从顶级容器开始,该容器为该界面的其他组件提供了宿主,并决定了应用程序的整体感觉。 在本教程中,我们介绍JFrame类,该类用于为Java应用程序创建一个简单的顶层窗口。
导入图形组件 ( Import the Graphical Components )
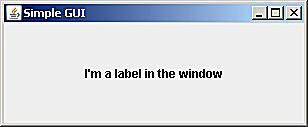
Open your text editor to start a new text file, and type in the following:
打开文本编辑器以启动新的文本文件,然后键入以下内容:
import java.awt.*;
import javax.swing.*;
Java comes with a set of code libraries designed to help programmers quickly create applications. They provide access to classes that perform specific functions, to save you the bother of having to write them yourself. The two import statements above let the compiler know that the application needs access to some of the pre-built functionality contained within the "AWT" and "Swing" code libraries.
Java带有一组旨在帮助程序员快速创建应用程序的代码库。 它们提供对执行特定功能的类的访问,以免您不得不自己编写它们的麻烦。 上面的两个import语句使编译器知道应用程序需要访问“ AWT”和“ Swing”代码库中包含的某些预构建功能。
AWT stands for “Abstract Window Toolkit.” It contains classes that programmers can use to make graphical components such as buttons, labels and frames. Swing is built on top of AWT, and provides an additional set of more sophisticated graphical interface components. With just two lines of code, we gain access to these graphical components, and can use them in our Java application.
AWT代表“抽象窗口工具包”。 它包含程序员可以用来制作图形组件(例如按钮,标签和框架)的类。 Swing建立在AWT之上,并提供了一组其他的更复杂的图形界面组件。 仅需两行代码,我们就可以访问这些图形组件,并可以在我们的Java应用程序中使用它们。
创建应用程序类 ( Create the Application Class )
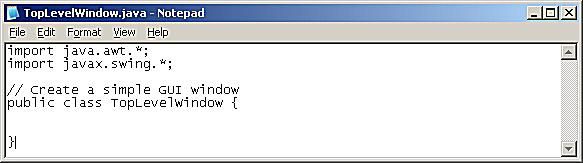
Below the import statements, enter the class definition that will contain our Java application code. Type in:
在import语句下面,输入将包含我们的Java应用程序代码的类定义。 输入:
//Create a simple GUI window
public class TopLevelWindow {
}
All the rest of the code from this tutorial goes between the two curly brackets. The TopLevelWindow class is like the covers of a book; it shows the compiler where to look for the main application code.
本教程中的所有其余代码都位于两个大括号之间。 TopLevelWindow类就像一本书的封面。 它显示了编译器在哪里查找主要应用程序代码。
创建构成JFrame的函数 ( Create the Function that Makes the JFrame )
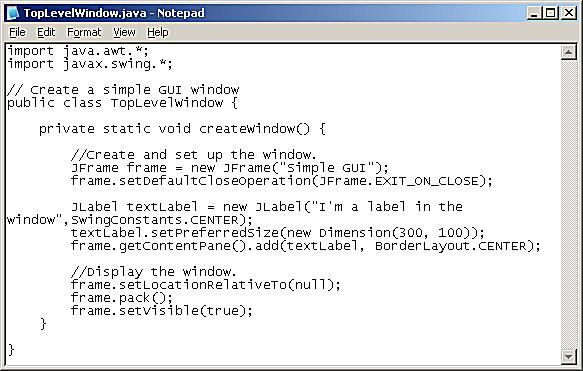
It's good programming style to group sets of similar commands into functions. This design makes the program more readable, and if you want to run the same set of instructions again, all you have to do is run the function. With this in mind, I'm grouping all the Java code that deals with creating the window into one function.
将相似命令集组合为函数是一种很好的编程风格。 这种设计使程序更具可读性,如果您想再次运行同一组指令,则只需运行该函数。 考虑到这一点,我将处理创建窗口的所有Java代码归为一个函数。
Enter the createWindow function definition:
输入createWindow函数定义:
private static void createWindow() {
}
All the code to create the window goes between the function’s curly brackets. Anytime the createWindow function is called, the Java application will create and display a window using this code.
创建窗口的所有代码都位于函数的大括号之间。 每当调用createWindow函数时,Java应用程序都将使用此代码创建并显示一个窗口。
Now, let's look at creating the window using a JFrame object. Type in the following code, remembering to place it between the curly brackets of the createWindow function:
现在,让我们看一下使用JFrame对象创建窗口。 输入以下代码,记住将其放在createWindow函数的大括号之间 :
//Create and set up the window.
JFrame frame = new JFrame("Simple GUI");
What this line does is create a new instance of a JFrame object called "frame". You can think of "frame" as the window for our Java application.
该行所做的是创建一个名为“ frame”的JFrame对象的新实例。 您可以将“框架”视为Java应用程序的窗口。
The JFrame class will do most of the work of creating the window for us. It handles the complex task of telling the computer how to draw the window to the screen, and leaves us the fun part of deciding how it's going to look. We can do this by setting its attributes, such as its general appearance, its size, what it contains, and more.
JFrame类将为我们完成创建窗口的大部分工作。 它处理了告诉计算机如何将窗口绘制到屏幕上的复杂任务,而使我们感到无聊的是决定它的外观。 我们可以通过设置其属性来实现此目的,例如其外观,大小,包含的内容等等。
For starters, let's make sure that when the window is closed, the application also stops. Type in:
首先,请确保关闭窗口后,应用程序也将停止。 输入:
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
The JFrame.EXIT_ON_CLOSE constant sets our Java application to terminate when the window is closed.
JFrame.EXIT_ON_CLOSE 常量将我们的Java应用程序设置为在关闭窗口时终止。
将JLabel添加到JFrame ( Add a JLabel to the JFrame )
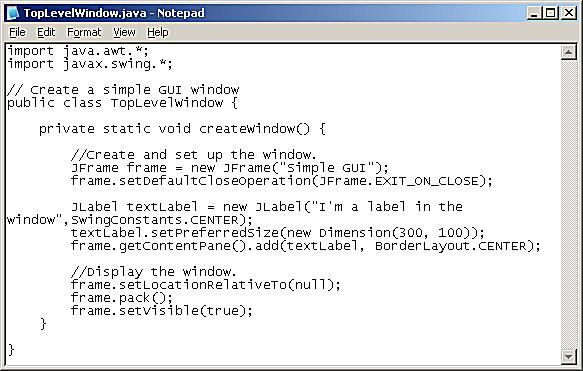
Since an empty window has little use, let's now put a graphical component inside it. Add the following lines of code to the createWindow function to create a new JLabel object
由于空窗口几乎没有用,现在让我们在其中放置一个图形组件。 将以下代码行添加到createWindow函数中,以创建一个新的JLabel对象
JLabel textLabel = new JLabel("I'm a label in the window",SwingConstants.CENTER); textLabel.setPreferredSize(new Dimension(300, 100));
A JLabel is a graphical component that can contain an image or text. To keep it simple, it’s filled with the text “I’m a label in the window.” and its size has been set to a width of 300 pixels and height of 100 pixels.
JLabel是可以包含图像或文本的图形组件。 为简单起见,其中填充了文本“我是窗口中的标签”。 并且其大小已设置为300像素的宽度和100像素的高度。
Now that we have created the JLabel, add it to the JFrame:
现在我们已经创建了JLabel,将其添加到JFrame中:
frame.getContentPane().add(textLabel, BorderLayout.CENTER);
The last lines of code for this function are concerned with how the window is displayed. Add the following to ensure that the window appears in the center of the screen:
此功能的最后代码行与窗口的显示方式有关。 添加以下内容以确保该窗口出现在屏幕中央:
//Display the window
frame.setLocationRelativeTo(null);
Next, set the window's size:
接下来,设置窗口的大小:
frame.pack();
The pack() method looks at what the JFrame contains, and automatically sets the size of the window. In this case, it ensures the window is big enough to show the JLabel.
pack()方法查看JFrame包含的内容,并自动设置窗口的大小。 在这种情况下,它确保窗口足够大以显示JLabel。
Finally, we need show the window:
最后,我们需要显示窗口:
frame.setVisible(true);
创建应用程序入口点 ( Create the Application Entry Point )
All that's left to do is add the Java application entry point. This calls the createWindow() function as soon as the application is run. Type in this function below the final curly bracket of the createWindow() function:
剩下要做的就是添加Java应用程序入口点。 一旦应用程序运行,这将立即调用createWindow()函数。 在createWindow()函数的最后花括号下方输入此函数:
public static void main(String[] args) {
createWindow();
}
到目前为止检查代码 ( Check the Code So Far )

This is a good point to make sure your code matches the example. Here is how your code should look:
这是确保您的代码与示例匹配的好方法。 这是代码的外观:
import java.awt.*;
import javax.swing.*;
// Create a simple GUI window
public class TopLevelWindow {
private static void createWindow() {
//Create and set up the window.
JFrame frame = new JFrame("Simple GUI");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JLabel textLabel = new JLabel("I'm a label in the window",SwingConstants.CENTER);
textLabel.setPreferredSize(new Dimension(300, 100));
frame.getContentPane().add(textLabel, BorderLayout.CENTER);
//Display the window.
frame.setLocationRelativeTo(null);
frame.pack();
frame.setVisible(true);
}
public static void main(String[] args) {
createWindow();
}
}
保存,编译并运行 ( Save, Compile and Run )
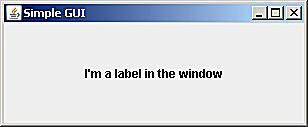
Save the file as "TopLevelWindow.java".
将文件另存为“ TopLevelWindow.java”。
Compile the application in a terminal window using the Javac compiler. If you’re unsure how to do so, look at the compilation steps from the first Java application tutorial.
使用Javac编译器在终端窗口中编译应用程序。 如果不确定如何执行此操作,请查看第一个Java应用程序教程中的编译步骤 。
javac TopLevelWindow.java
Once the application compiles successfully, run the program:
应用程序成功编译后,运行程序:
java TopLevelWindow
After pressing Enter, the window will appear, and you will see your first windowed application.
按Enter键后,将出现一个窗口,您将看到第一个窗口化的应用程序。
Well done! this tutorial is the first building block to making powerful user interfaces. Now that you know how to make the container, you can play with adding other graphical components.
做得好! 本教程是构建强大的用户界面的第一个构建块。 既然您知道如何制作容器,就可以添加其他图形组件。
翻译自: https://www.thoughtco.com/create-a-simple-window-using-jframe-2034069
jframe滑动窗口