高阶函数 (Higher-Order Functions)
A function that accepts and/or returns another function is called a higher-order function.
接受和/或返回另一个函数的函数称为高阶函数 。
It’s higher-order because instead of strings, numbers, or booleans, it goes higher to operate on functions. Pretty meta.
它是高阶的,因为它代替字符串,数字或布尔值,对函数进行运算会更高 。 相当元。
With functions in JavaScript, you can
使用JavaScript中的函数,您可以
- Store them as variables 将它们存储为变量
- Use them in arrays 在数组中使用它们
- Assign them as object properties (methods) 将它们分配为对象属性(方法)
- Pass them as arguments 将它们作为参数传递
- Return them from other functions 从其他函数返回它们
Like any other piece of data. That’s the key here.
像其他任何数据一样 。 这就是关键。
功能操作数据 (Functions Operate on Data)
字符串就是数据 (Strings Are Data)
sayHi = (name) => `Hi, ${name}!`;
result = sayHi('User');
console.log(result); // 'Hi, User!'
数字就是数据 (Numbers Are Data)
double = (x) => x * 2;
result = double(4);
console.log(result); // 8
布尔是数据 (Booleans Are Data)
getClearance = (allowed) => (allowed ? 'Access granted' : 'Access denied');
result1 = getClearance(true);
result2 = getClearance(false);
console.log(result1); // 'Access granted'
console.log(result2); // 'Access denied'
对象就是数据 (Objects Are Data)
getFirstName = (obj) => obj.firstName;
result = getFirstName({
firstName: 'Yazeed'
});
console.log(result); // 'Yazeed'
数组就是数据 (Arrays Are Data)
len = (array) => array.length;
result = len([1, 2, 3]);
console.log(result); // 3
These 5 types are first-class citizens in every mainstream language.
这五种语言是每种主流语言中的一等公民 。
What makes them first-class? You can pass them around, store them in variables and arrays, use them as inputs for calculations. You can use them like any piece of data.
是什么使他们成为一流? 您可以传递它们,将它们存储在变量和数组中,并将它们用作计算的输入。 您可以像使用任何数据一样使用它们。
函数可能也是数据 (Functions Can Be Data Too)
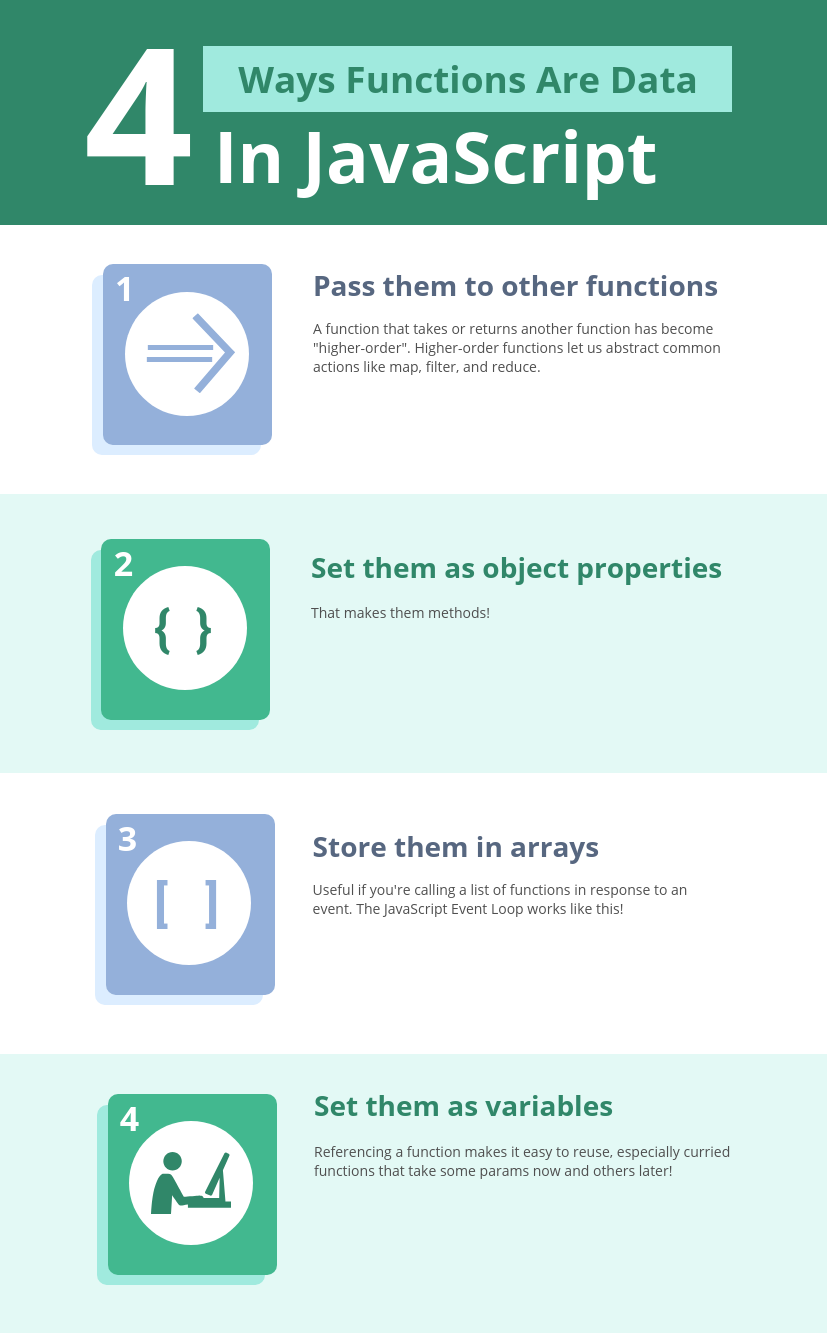
作为参数 (Functions as Arguments)
isEven = (num) => num % 2 === 0;
result = [1, 2, 3, 4].filter(isEven);
console.log(result); // [2, 4]
See how filter
uses isEven
to decide what numbers to keep? isEven
, a function, was a parameter to another function.
看看filter
如何使用isEven
决定要保留哪些数字? isEven
是一个函数 ,是另一个函数的参数。
It’s called by filter
for each number, and uses the returned value true
or false
to determine if a number should be kept or discarded.
每个数字都会被filter
调用,并使用返回值true
或false
来确定是否应保留或丢弃某个数字。
返回函数 (Returning Functions)
add = (x) => (y) => x + y;
add
requires two parameters, but not all at once. It’s a function asking for just x
, that returns a function asking for just y
.
add
需要两个参数,但不是一次全部。 这是一个仅要求x
的函数,它返回仅要求y
的函数。
Again, this is only possible because JavaScript allows functions to be a return value — just like strings, numbers, booleans, etc.
同样,这仅是可行的,因为JavaScript允许函数作为返回值-就像字符串,数字,布尔值等。
You can still supply x
and y
immediately, if you wish, with a double invocation
如果愿意,您仍然可以立即提供x
和y
两次调用
result = add(10)(20);
console.log(result); // 30
Or x
now and y
later:
或x
现在和y
之后:
add10 = add(10);
result = add10(20);
console.log(result); // 30
Let’s rewind that last example. add10
is the result of calling add
with one parameter. Try logging it in the console.
让我们倒回最后一个例子。 add10
是使用一个参数调用add
的结果。 尝试将其记录在控制台中。

add10
is a function that takes a y
and returns x + y
. After you supply y
, it hurries to calculate and return your end result.
add10
是一个接受y
并返回x + y
的函数。 提供y
,它会急于计算并返回最终结果。

更高的可重用性 (Greater Reusability)
Probably the greatest benefit of HOFs is greater reusability. Without them, JavaScript’s premiere Array methods — map
, filter
, and reduce
— wouldn’t exist!
HOF的最大好处可能是更高的可重用性。 没有它们,JavaScript的首要Array方法( map
, filter
和reduce
)将不复存在!
Here’s a list of users. We’re going to do some calculations with their information.
这是用户列表。 我们将对它们的信息进行一些计算。
users = [
{
name: 'Yazeed',
age: 25
},
{
name: 'Sam',
age: 30
},
{
name: 'Bill',
age: 20
}
];
地图 (Map)
Without higher-order functions, we’d always need loops to mimic map
's functionality.
没有高阶函数,我们总是需要循环来模仿map
的功能。
getName = (user) => user.name;
usernames = [];
for (let i = 0; i < users.length; i++) {
const name = getName(users[i]);
usernames.push(name);
}
console.log(usernames);
// ["Yazeed", "Sam", "Bill"]
Or we could do this!
或者我们可以这样做!
usernames = users.map(getName);
console.log(usernames);
// ["Yazeed", "Sam", "Bill"]
过滤 (Filter)
In a HOF-less world, we’d still need loops to recreate filter
's functionality too.
在没有HOF的世界中,我们仍然仍然需要循环来重新创建filter
的功能。
startsWithB = (string) => string.toLowerCase().startsWith('b');
namesStartingWithB = [];
for (let i = 0; i < users.length; i++) {
if (startsWithB(users[i].name)) {
namesStartingWithB.push(users[i]);
}
}
console.log(namesStartingWithB);
// [{ "name": "Bill", "age": 20 }]
Or we could do this!
或者我们可以这样做!
namesStartingWithB = users.filter((user) => startsWithB(user.name));
console.log(namesStartingWithB);
// [{ "name": "Bill", "age": 20 }]
减少 (Reduce)
Yup, reduce too… Can’t do much cool stuff without higher-order functions!! ?
是的,也要减少……没有高阶函数就不能做很多很棒的事情! ?
total = 0;
for (let i = 0; i < users.length; i++) {
total += users[i].age;
}
console.log(total);
// 75
How’s this?
这个怎么样?
totalAge = users.reduce((total, user) => user.age + total, 0);
console.log(totalAge);
// 75
摘要 (Summary)
- Strings, numbers, bools, arrays, and objects can be stored as variables, arrays, and properties or methods. 字符串,数字,布尔值,数组和对象可以存储为变量,数组以及属性或方法。
- JavaScript treats functions the same way. JavaScript以相同的方式对待函数。
This allows for functions that operate on other functions: higher-order functions.
这允许在其他功能上运行的功能: 高阶功能 。
- Map, filter, and reduce are prime examples — and make common patterns like transforming, searching, and summing lists much easier! 映射,过滤和归约是主要示例-使转换,搜索和汇总列表之类的通用模式变得更加容易!
I’m on Twitter if you’d like to talk. Until next time!
Take care, Yazeed Bzadough yazeedb.com
保重,Yazeed Bzadough yazeedb.com
翻译自: https://www.freecodecamp.org/news/a-quick-intro-to-higher-order-functions-in-javascript-1a014f89c6b/