工作流项目演练
Project 1 from JavaScript Algos and DS Certification.
JavaScript Algos和DS认证的项目1。
This is the blog version of my walkthrough. If you prefer video here is the YouTube video link.
这是我的演练的博客版本。 如果您喜欢视频,请访问YouTube视频链接 。
挑战 (The Challenge)
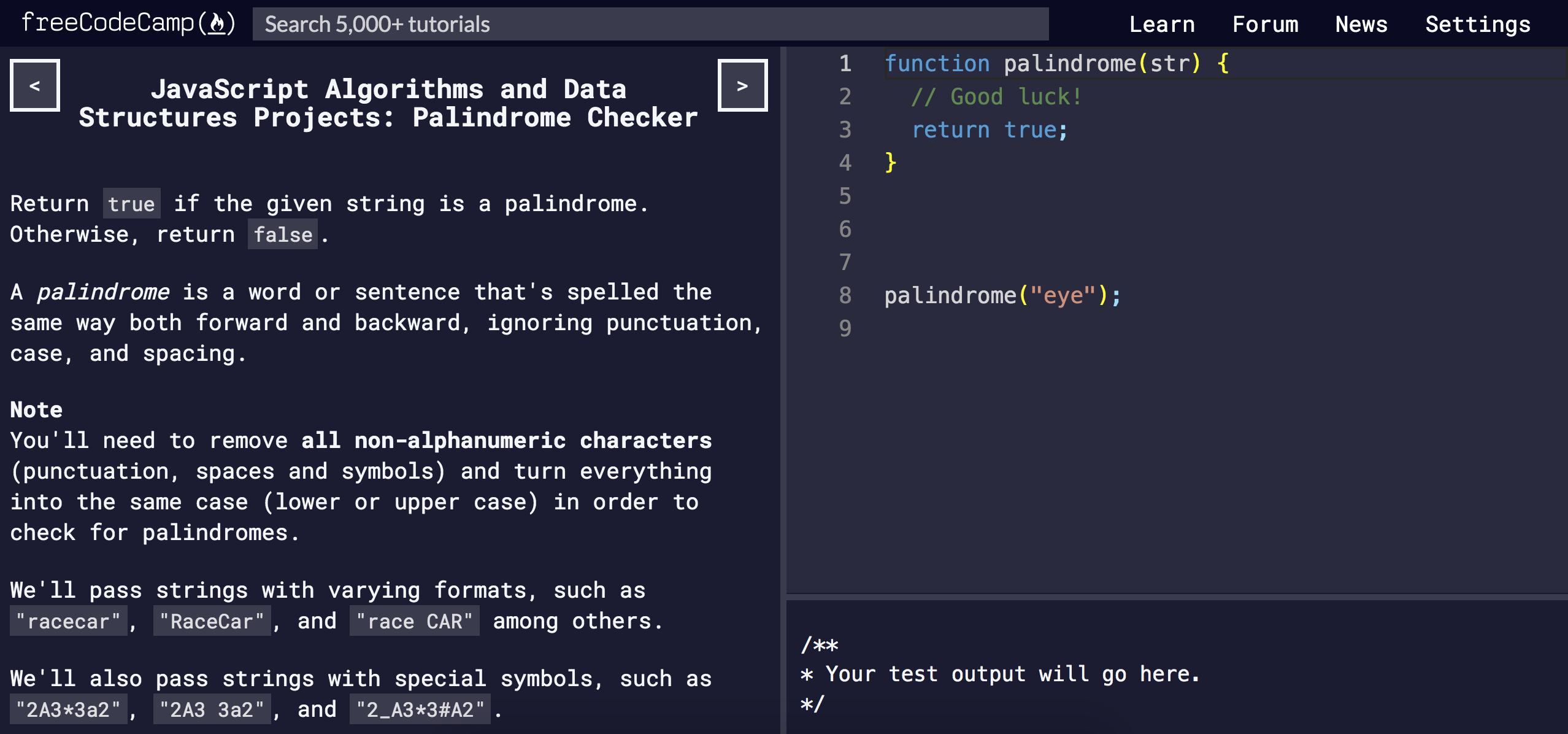
Write a function called palindrome
that takes a string, str
. If str
is a palindrome, return true
, otherwise return false
.
编写一个名为palindrome
的函数,该函数需要一个字符串str
。 如果str
是回文,则返回true
,否则返回false
。
什么是回文? (What Is a Palindrome?)
A palindrome is a word that reads the same forwards and backwards. Some examples are
回文是一个单词,前后读相同。 一些例子是
- Eye 眼
- Racecar 赛车
- A Man, A Plan, A Canal – Panama! 一个人,一个计划,一条运河–巴拿马!
Whether you read these left-to-right or right-to-left, you get the same sequence of characters. We’ll be ignoring punctuation like commas, periods, question marks, exclamation points, and casing.
无论您从左到右还是从右到左阅读,都将获得相同的字符序列。 我们将忽略逗号,句点,问号,感叹号和大小写等标点符号。
步骤0-远离代码 (Step 0 - Step Away from the Code)
I like keeping this mind during any interview or problem I have to solve at work. Rushing into the code first is usually a losing strategy, because now you have to consider syntax while trying to solve the problem in your head.
我喜欢在工作中必须解决的任何面试或问题时保持头脑清醒。 首先进入代码通常是一个失败的策略,因为现在您必须在尝试解决语法问题时考虑语法。
Code should come last
代码应排在最后
Don't let your nerves get the better of you. Instead of frantically hacking at a solution and elevating your blood pressure, take a deep breath and try to write it out on a whiteboard or in a notebook.
不要让您的神经变得更好。 与其疯狂地寻找解决方案并提高血压,不如深呼吸,尝试将其写在白板或笔记本上。
Once you've thought out a solution, the code comes easy. All the hard work happens in your mind and notes, not on the keyboard.
一旦您想到了解决方案,代码就变得容易了。 所有的辛苦工作都发生在您的脑海和笔记上,而不是键盘上。
步骤1-平衡所有套管 (Step 1 - Equalize All Casing)
A palindrome is valid whether or not its casing reads the same forwards or backwards. So "Racecar" is valid even though it's technically spelt "racecaR" backwards.
回文无论其外壳向前还是向后读取相同,都是有效的。 因此,“ Racecar”即使在技术上拼写为“ racecaR”,也仍然有效。
To safeguard us against any casing issues, I'll add a comment saying we'll lowercase everything.
为了保护我们免受任何套管问题的影响,我将添加一条评论,说我们将小写所有内容。
Here's my code so far (notice I wrote no real code yet).
到目前为止,这是我的代码(注意,我尚未编写任何实际代码)。
function palindrome(str) {
// 1) Lowercase the input
}
palindrome("eye");
第2步-去除非字母数字字符 (Step 2 - Strip Non-Alphanumeric Characters)
Just like the casing scenario, a palindrome is valid even if the punctuation and spaces aren't consistent back and forth.
就像套管情况一样,即使标点符号和空格来回前后不一致,回文图也是有效的。
For example "A Man, A Plan, A Canal – Panama!" is valid because we examine it without any marks or spaces. If you do that and lowercase everything, it becomes this.
例如“一个人,一个计划,一条运河-巴拿马!” 之所以有效,是因为我们检查时没有任何标记或空格。 如果您这样做并且将所有内容都小写,它就会变成这样。
"A Man, A Plan, A Canal – Panama!"
// lowercase everything
// strip out non-alphanumeric characters
"amanaplanacanalpanama"
Which reads the same forwards and backwards.
向前和向后读取相同的内容。
字母数字是什么意思? (What does alphanumeric mean?)
It means "letters and numbers", so anything from a-z and 0-9 is an alphanumeric character. In order to properly examine our input non-alphanumeric characters (spaces, punctuation, etc) must go.
它的意思是“字母和数字”,因此az和0-9之间的任何字符都是字母数字字符。 为了正确检查我们输入的非字母数字字符(空格,标点符号等)必须使用。
Here's our updated pseudocode.
这是我们更新的伪代码。
function palindrome(str) {
// 1) Lowercase the input
// 2) Strip out non-alphanumeric characters
}
palindrome("eye");
步骤3-比较字符串的反向 (Step 3 - Compare String to Its Reverse)
Once our string's properly cleaned up, we can flip it around and see if it reads the same.
正确清理字符串后,我们可以将其翻转,看是否读取相同的字符串。
I'm thinking a comparison along these lines
我正在考虑按照这些思路进行比较
return string === reversedString
I'm using triple equals (===
) for comparison in JavaScript. If the two strings are identical, it's a palindrome and we return true
! If not we return false
.
我在JavaScript中使用三重等于( ===
)进行比较。 如果两个字符串相同,则为回文,我们返回true
! 如果不是,则返回false
。
Here's our updated pseudocode.
这是我们更新的伪代码。
function palindrome(str) {
// 1) Lowercase the input
// 2) Strip out non-alphanumeric characters
// 3) return string === reversedString
}
palindrome("eye");
执行步骤1-小写 (Executing Step 1 - Lowercase)
This is the easiest step. If you are unsure how to lowercase something in JavaScript, a quick Google search should lead to the toLowerCase
method.
这是最简单的步骤。 如果不确定如何在JavaScript中将小写形式转换为小写,则应通过Google的快速搜索找到toLowerCase
方法。
This is a method available on all string, so we can use it to lowercase our input before doing anything else.
这是所有字符串上可用的方法,因此我们可以在执行其他任何操作之前使用它来减小输入的大小写。
I'll store the lowercase version in a variable called alphanumericOnly
because we're eventually going to remove alphanumeric characters too.
我将小写版本存储在名为alphanumericOnly
的变量中,因为我们最终还将删除字母数字字符。
function palindrome(str) {
// 1) Lowercase the input
const alphanumericOnly = str.toLowerCase();
// 2) Strip out non-alphanumeric characters
// 3) return string === reversedString
}
palindrome("eye");
执行步骤2-仅字母数字 (Executing Step 2 - Alphanumeric Only)
We'll have to dive a bit deeper here, as this is the toughest step. How exactly are we going to purify a string of its non-alphanumeric characters?
我们将不得不在这里进行更深入的研究,因为这是最艰难的一步。 我们将如何精确地纯化其非字母数字字符的字符串?
.match方法 (The .match method)
Just like toLowerCase
all strings support a method called match
. It takes a parameter indicating what character(s) you'd like to look for in a given string.
就像toLowerCase
一样,所有字符串都支持一种称为match
的方法。 它带有一个参数,该参数指示您要在给定字符串中查找哪些字符。
Let's use my name as an example.
让我们以我的名字为例。
myName = 'yazeed';
myName.match('e');
// ["e", index: 3, input: "yazeed", groups: undefined]
As you can see .match
returns an array with some information. The part we care about is the first element, 'e'
. That's the match we found in the string 'yazeed'
.
如您所见, .match
返回一个包含一些信息的数组。 我们关心的部分是第一个元素'e'
。 这就是我们在字符串'yazeed'
找到的匹配'yazeed'
。
But my name has two e's! How do we match the other one?
但是我的名字有两个e! 我们如何匹配另一个?
正则表达式(Regex) (Regular Expressions (Regex))
The .match
method's first parameter can instead be a regular expression.
.match
方法的第一个参数可以是正则表达式 。
Regular Expression - A sequence of characters that define a search pattern. Also known as "Regex".
正则表达式-定义搜索模式的字符序列。 也称为“正则表达式”。
Instead of quotation marks for a string, put your parameter between forward slashes.
您可以将参数放在正斜杠之间,而不是用引号引起来。
myName = 'yazeed';
myName.match(/e/);
// ["e", index: 3, input: "yazeed", groups: undefined]
We get the same result so who cares? Well check this out, regex allows us to add flags.
我们得到相同的结果,所以谁在乎? 好了,检查一下,正则表达式允许我们添加标志 。
Regex Flag - An indicator that tells Regex to do something special.
正则表达式标志-指示正则表达式执行特殊操作的指示器。
myName = 'yazeed';
myName.match(/e/g);
// ^^ Notice the little g now ^^
// ["e", "e"]
We got back all the e's! If you try an a or z, you get an array of just one match. Makes sense.
我们找回了所有的e! 如果您尝试使用a或z,则会得到一个只有一个匹配项的数组。 说得通。
myName.match(/a/g);
// ["a"]
myName.match(/z/g);
// ["z"]
查找所有字母数字字符 (Finding all alphanumeric characters)
So regex not only matches patterns, but it can match many of the same kind of pattern! This sounds perfect for our algorithm's next step.
因此,正则表达式不仅可以匹配模式,而且可以匹配许多相同类型的模式! 这听起来很适合我们算法的下一步。
If you Google a bit, this may be the regex you find for matching all alphanumeric characters.
如果您对Google感兴趣,则可能是找到的用于匹配所有字母数字字符的正则表达式。
/[a-z0-9]/g
You're looking at the definition of alphanumeric. This regex can be broken into 3 parts.
您正在查看字母数字的定义。 此正则表达式可分为3部分。
A character set
[]
- match any character between these brackets.字符集
[]
-匹配这些括号之间的任何字符。a-z
- match all lowercase lettersaz
匹配所有小写字母0-9
- match all numbers0-9
匹配所有数字
Running it on myName
returns an array of every letter.
在myName
上运行它会返回每个字母的数组。
myName = 'yazeed';
myName.match(/[a-z0-9]/g);
// ["y", "a", "z", "e", "e", "d"]
Let's try it with one of the project's test cases. How about this crazy one they expect to be a palindrome?
让我们用项目的一个测试用例来尝试一下。 他们期望这是一个回文症,这又如何呢?
crazyInput = '0_0 (: /-\ :) 0-0';
crazyInput.match(/[a-z0-9]/g);
// ["0", "0", "0", "0"]
Wow without the crazy characters it's just four zeroes. Yep that's a palindrome! I'll update our code.
哇,没有疯狂的角色,它只是四个零。 是的,这是回文! 我将更新代码。
function palindrome(str) {
const alphanumericOnly = str
// 1) Lowercase the input
.toLowerCase()
// 2) Strip out non-alphanumeric characters
.match(/[a-z0-9]/g);
// 3) return string === reversedString
}
palindrome("eye");
执行步骤3-将字符串与其相反的字符串进行比较 (Executing Step 3 - Compare String to Its Reverse)
Remember that .match
returns an array of matches. How can we use that array to compare our cleaned up string to its reversed self?
请记住, .match
返回一个匹配数组 。 我们如何使用该数组将清理后的字符串与其反向自身进行比较?
Array.reverse (Array.reverse)
The reverse
method, true to its name, reverses an array's elements.
顾名思义, reverse
方法可反转数组的元素。
[1, 2, 3].reverse();
// [3, 2, 1]
This looks pretty useful! After matching all alphanumeric characters, we can flip that array and see if everything still lines up.
这看起来很有用! 匹配所有字母数字字符后,我们可以翻转该数组,看看是否仍然对齐。
But comparing arrays isn't as straightforward as comparing strings, so how can we turn that array of matches back into a string?
但是比较数组并不像比较字符串那样简单,那么我们如何才能将匹配项数组转换回字符串呢?
Array.join (Array.join)
The join
method stitches your array's elements together into a string, optionally taking a separator.
join
方法将数组的元素缝在一起成为一个字符串,可以选择使用一个分隔符 。
The separator is the first parameter, you don't need to supply it. It'll basically "stringify" your array.
分隔符是第一个参数,您不需要提供它。 基本上,它将“字符串化”您的数组。
[1, 2, 3].join();
// "1,2,3"
If you do supply it, the separator goes in between each element.
如果确实提供它,则分隔符会插入每个元素之间。
[1, 2, 3].join('my separator');
// "1my separator2my separator3"
[1, 2, 3].join(',');
// "1,2,3"
[1, 2, 3].join(', ');
// "1, 2, 3"
[1, 2, 3].join('sandwich');
// "1sandwich2sandwich3"
Let's see how this would fit into our algorithm.
让我们看看这将如何适合我们的算法。
'Ra_Ce_Ca_r -_-'
.toLowerCase()
.match(/[a-z0-9]/g)
.join('');
// "racecar"
See how doing all that simply recreates the original string without punctuation or mixed casing?
看看如何简单地重新创建原始字符串而不使用标点符号或混合大小写?
What if we reverse it?
如果我们撤消该怎么办?
'Ra_Ce_Ca_r -_-'
.toLowerCase()
.match(/[a-z0-9]/g)
// flip it around
.reverse()
.join('');
// "racecar"
That's a palindrome! My name would not be a palindrome.
那是回文! 我的名字不会是回文。
'yazeed'
.toLowerCase()
.match(/[a-z0-9]/g)
// flip it around
.reverse()
.join('');
// "deezay"
Seems we have our solution. Let's see the final code.
似乎我们有解决方案。 让我们看看最终的代码。
最终密码 (The Final Code)
function palindrome(str) {
const alphanumericOnly = str
// 1) Lowercase the input
.toLowerCase()
// 2) Strip out non-alphanumeric characters
.match(/[a-z0-9]/g);
// 3) return string === reversedString
return alphanumericOnly.join('') ===
alphanumericOnly.reverse().join('');
}
palindrome("eye");
Input this and run the tests, and we're good!
输入并运行测试,我们很好!
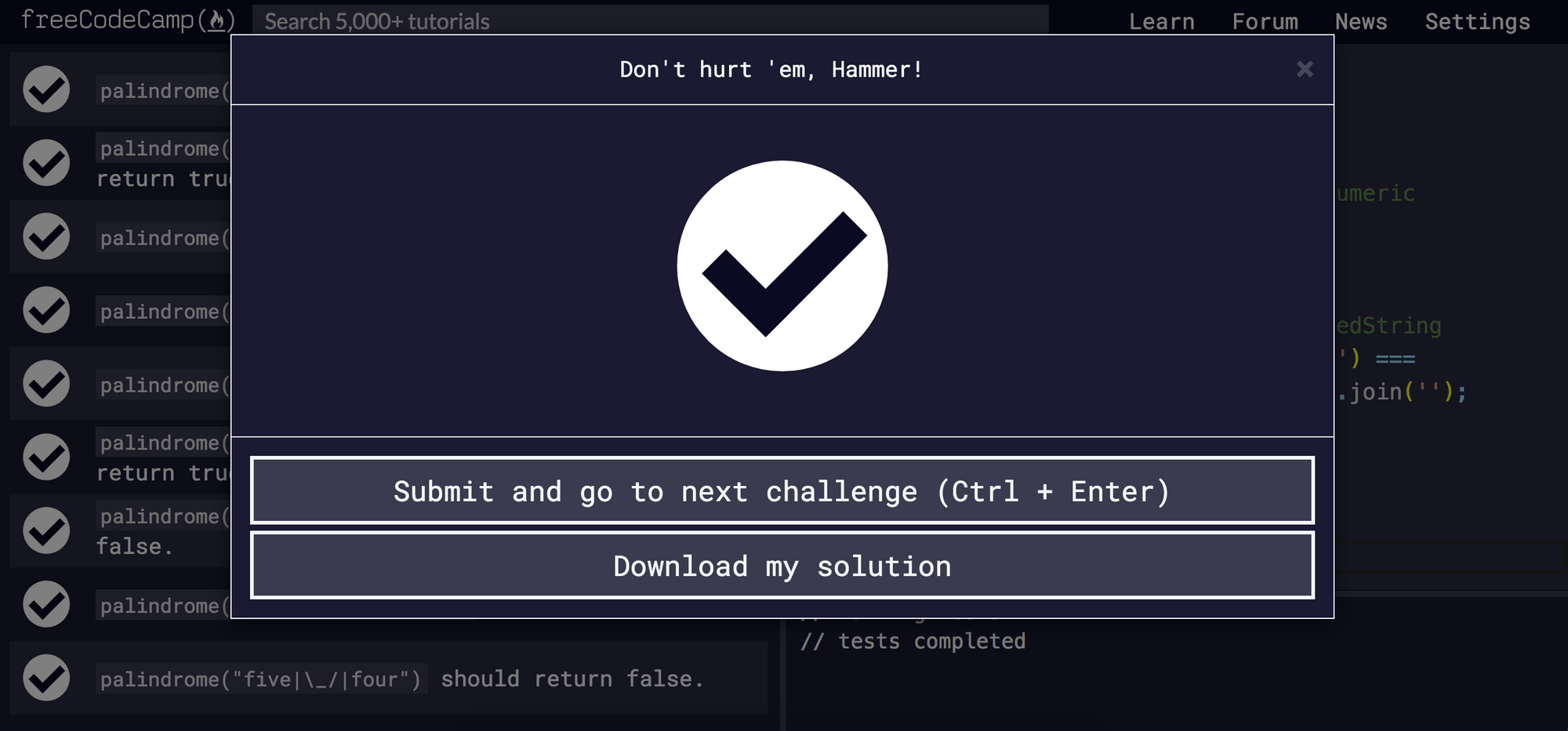
摘要 (Summary)
Lowercase input via
str.toLowerCase()
;通过
str.toLowerCase()
输入小写str.toLowerCase()
;Match all alphanumeric characters using a regular expression via
str.match(/[a-z0-9]/g)
.使用正则表达式通过
str.match(/[a-z0-9]/g)
匹配所有字母数字字符。Use
Array.reverse
andArray.join
on the alphanumeric matches to compare the original against its reversed self. If they're identical we get backtrue
, otherwise we get backfalse
!在字母数字匹配
Array.join
上使用Array.reverse
和Array.join
将原始值与其反转后的自身进行比较。 如果它们相同,则返回true
,否则返回false
!
谢谢阅读 (Thanks for reading)
If you'd like a video with even more detail, here's the YouTube version again!
如果您想获得更详细的视频,请再次使用YouTube版本 !
For more content like this, check out https://yazeedb.com. And please let me know what else you'd like to see! My DMs are open on Twitter.
有关此类的更多内容,请访问https://yazeedb.com 。 并且,请让我知道您还想看到什么! 我的DM在Twitter上打开。
Until next time!
直到下一次!
翻译自: https://www.freecodecamp.org/news/freecodecamp-palindrome-checker-walkthrough/
工作流项目演练