30 Seconds of Code is a brilliant collection of JavaScript snippets, digestible in ≤ 30 seconds. Anyone looking to master JavaScript should go through the entire thing.
30秒的代码是精妙JavaScript片段集合,可在≤30秒内消化。 任何想要精通JavaScript的人都应该仔细研究整个过程。
The list didn’t contain a function to rename multiple object keys, however, so I created a pull request that thankfully got merged!
该列表没有包含重命名多个对象键的功能,因此,我创建了一个合并请求的拉取请求 !
Here’s the official entry: https://30secondsofcode.org/object#renamekeys
这是官方条目: https : //30secondsofcode.org/object#renamekeys
I’ve previously written on renaming object keys, but we only changed one key at a time.
我以前写过重命名对象键的内容 ,但是我们一次只更改了一个键。
Then Adam Rowe kindly commented, asking how we might rename multiple object keys. I replied with this code sample after some thought and research.
然后Adam Rowe友善地评论,询问我们如何重命名多个对象键。 经过一番思考和研究,我回复了此代码示例。
renameKeys = (keysMap, obj) =>
Object.keys(obj).reduce(
(acc, key) => ({
...acc,
...{ [keysMap[key] || key]: obj[key] }
}),
{}
);
This was inspired by Ramda Adjunct’s renameKeys
function.
这是受renameKeys
Adjunct的renameKeys
函数启发的。
keysMap
contains key/value pairs of your old/new object keys.keysMap
包含旧/新对象键的键/值对。obj
is the object to be changed.obj
是要更改的对象。
You might use it like this:
您可以这样使用它:
keysMap = {
name: 'firstName',
job: 'passion'
};
obj = {
name: 'Bobo',
job: 'Front-End Master'
};
renameKeys(keysMap, obj);
// { firstName: 'Bobo', passion: 'Front-End Master' }
Let’s step through it! We can write an expanded, debugger
-friendly version of this function:
让我们逐步完成它! 我们可以编写此函数的扩展的, debugger
友好的版本:
renameKeys = (keysMap, obj) => {
debugger;
return Object.keys(obj).reduce((acc, key) => {
debugger;
const renamedObject = {
[keysMap[key] || key]: obj[key]
};
debugger;
return {
...acc,
...renamedObject
};
}, {});
};
And we’ll use it like this:
我们将像这样使用它:
renameKeys(
{
name: 'firstName',
job: 'passion'
},
{
name: 'Bobo',
job: 'Front-End Master'
}
);
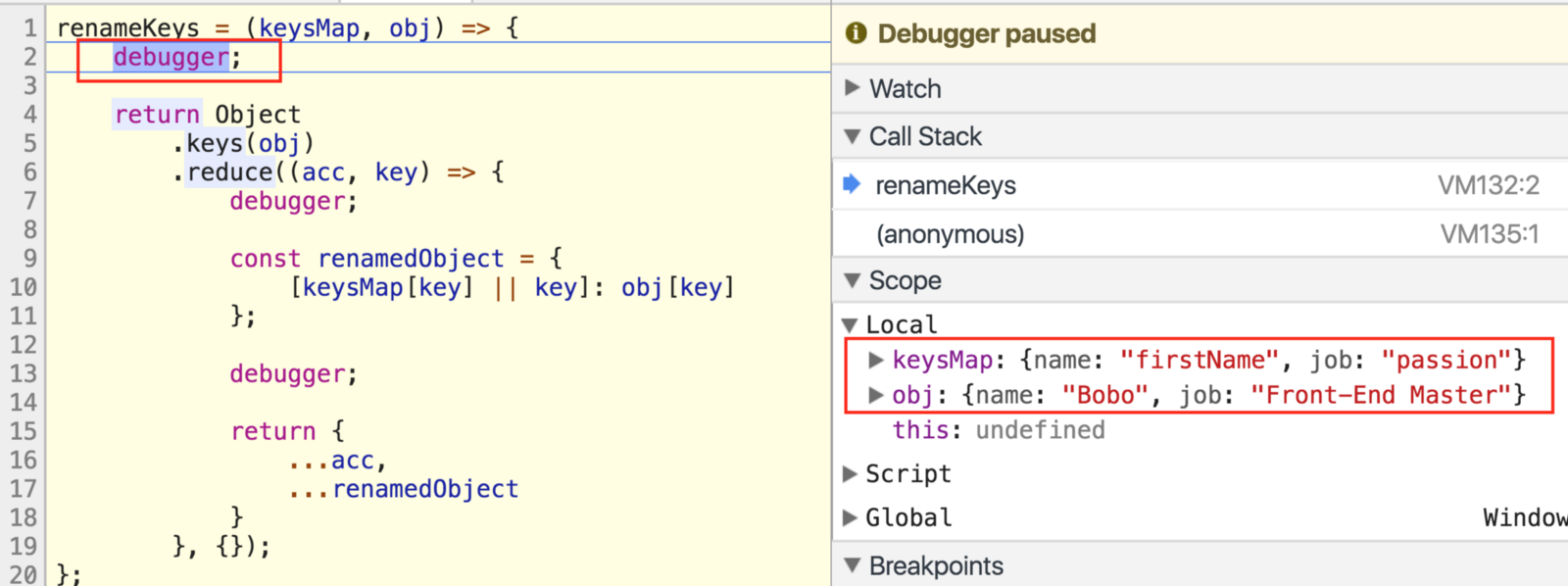
Pausing on line 2, we see that keysMap
and obj
have been properly assigned.
在第2行暂停,我们看到keysMap
和obj
已正确分配。
Here’s where the fun begins. Move to the next debugger
.
这就是乐趣的开始。 移至下一个debugger
。
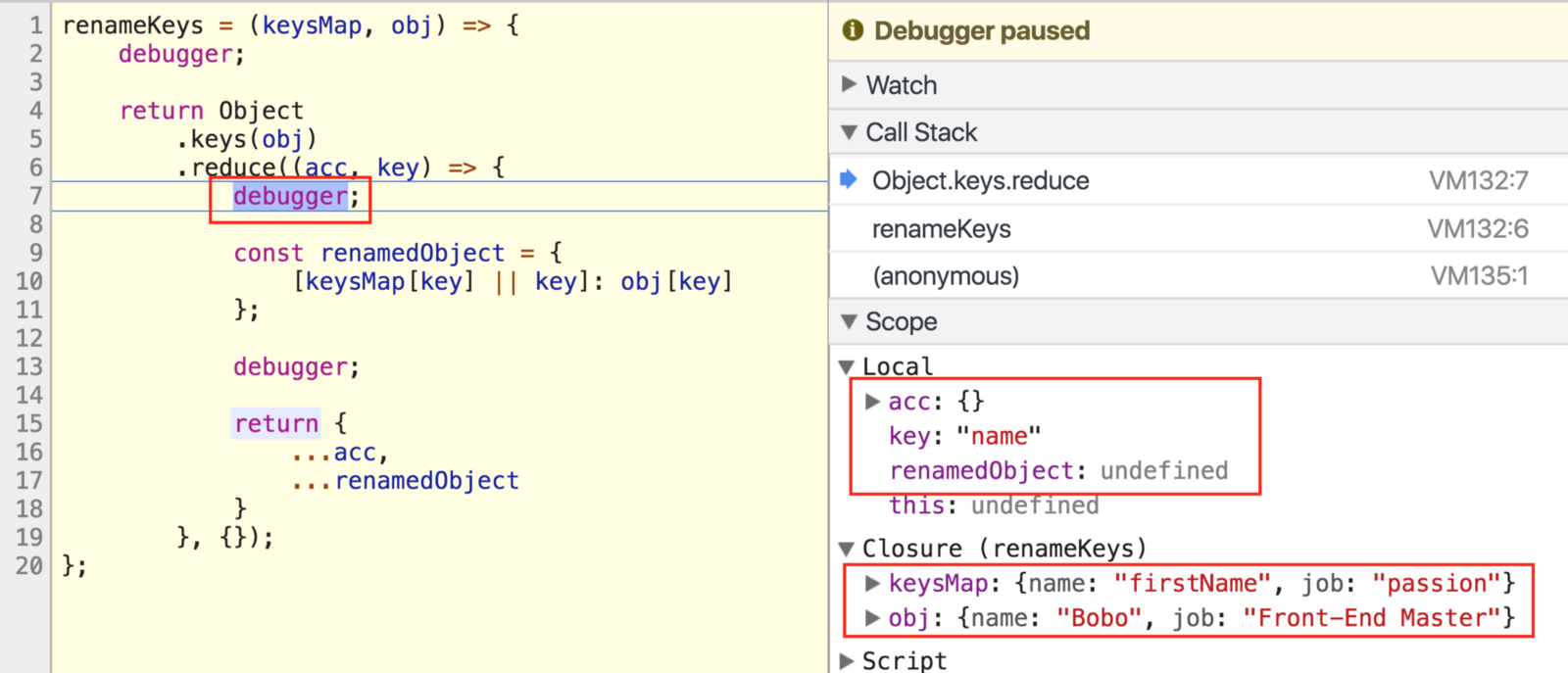
Inspect our local variables on line 7:
检查第7行的局部变量:
acc: {}
because that’sArray.reduce()
’s initial value (line 19).acc: {}
因为那是Array.reduce()
的初始值(第19行)。key: “name”
because it’s the first key fromObject.keys(obj)
.key: “name”
因为它是Object.keys(obj)
的第一个键。renamedObject: undefined
renamedObject: undefined
Also notice that we can access keysMap
and obj
from the parent function’s scope.
还要注意,我们可以从父函数的作用域访问keysMap
和obj
。
Guess what renamedObject
will be. Like in my aforementioned post, we’re using computed property names to dynamically assign renamedObject
's key.
猜想renamedObject
将是什么。 就像在我前面提到的文章中一样 ,我们使用计算出的属性名称来动态分配renamedObject
的键。
If keysMap[key]
exists, use it. Otherwise, use the original object key. In this case, keysMap[key]
is “firstName”
.
如果keysMap[key]
存在,请使用它。 否则,请使用原始对象密钥。 在这种情况下, keysMap[key]
是“firstName”
。
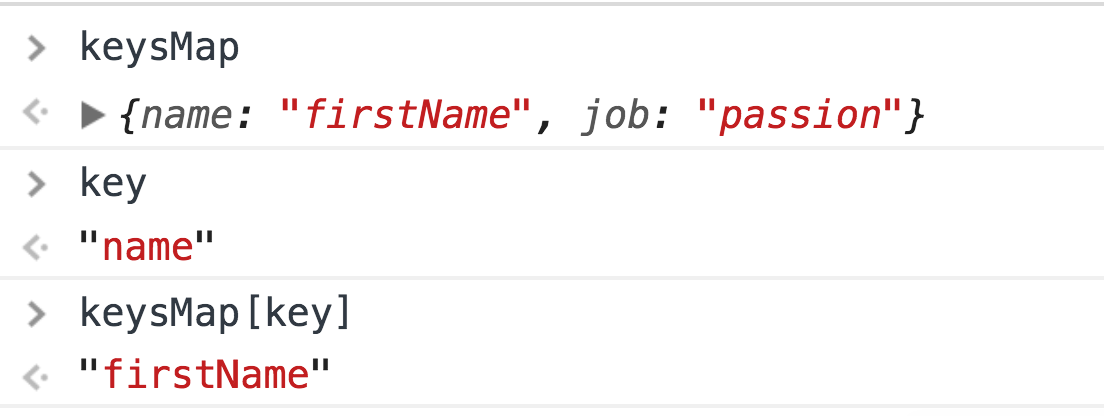
That’s renamedObject
's key, what about its corresponding value?
那就是renamedObject
的键,它的对应值如何?
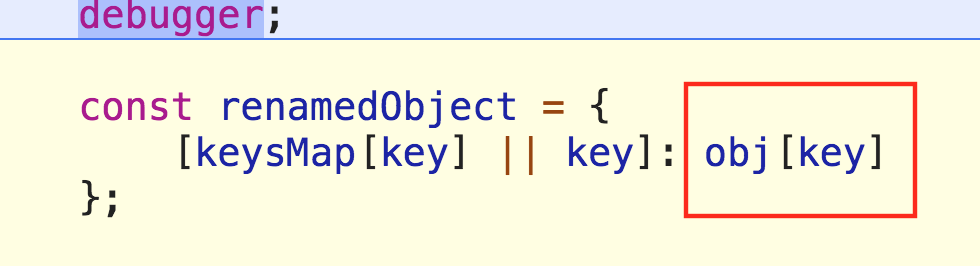
It’s obj[key]
: "Bobo"
. Hit the next debugger
and check it out.
它是obj[key]
: "Bobo"
。 点击下一个debugger
并签出。
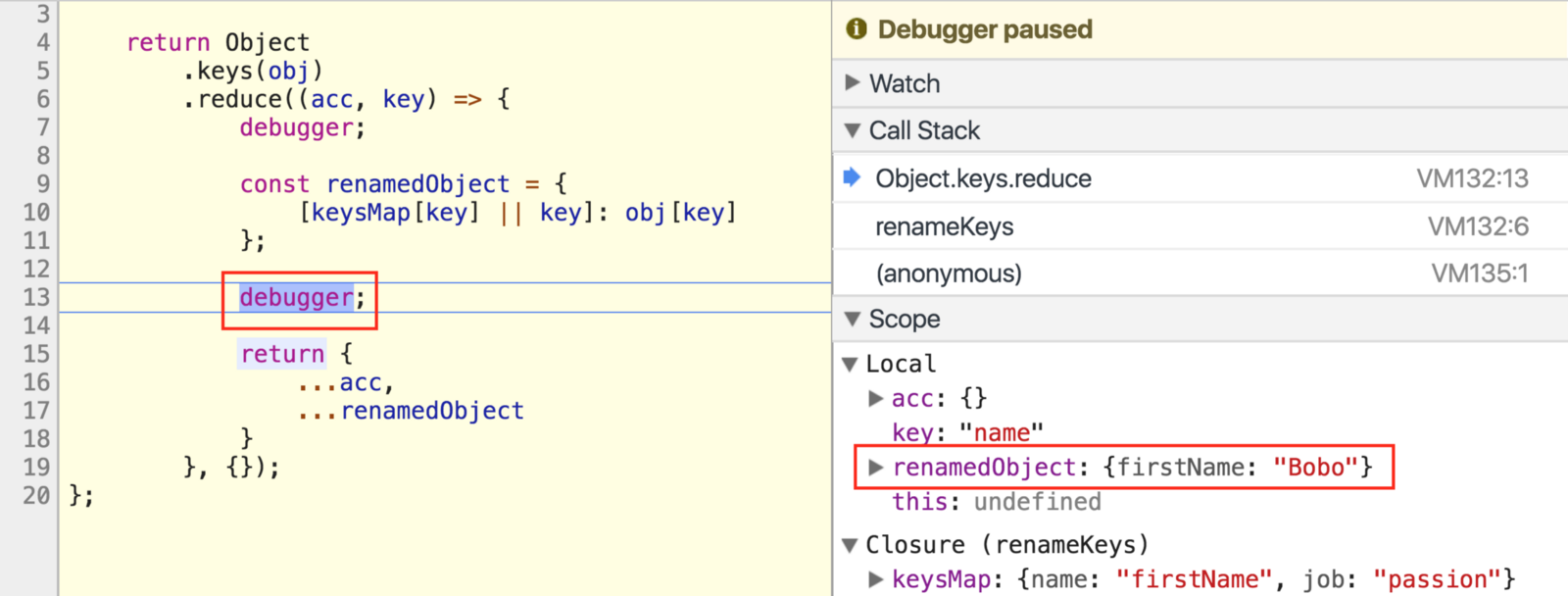
renamedObject
is now { firstName: “Bobo” }
.
现在renamedObject
是{ firstName: “Bobo” }
。
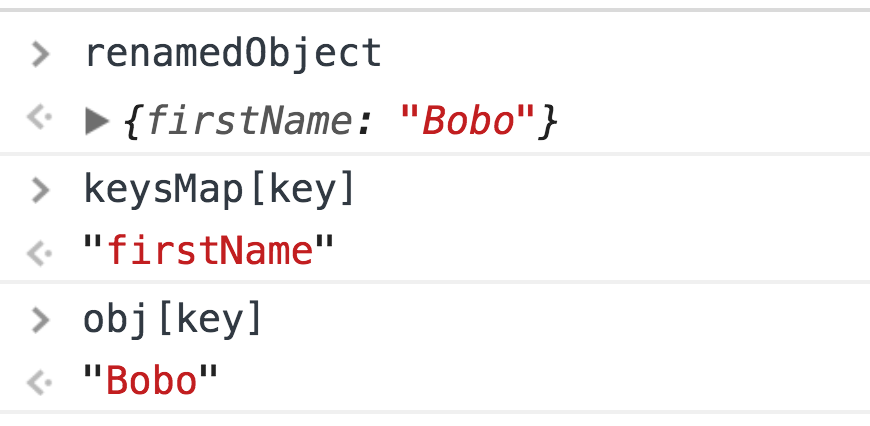
Now using the spread operator, we’ll merge acc
and renamedObject
. Remember that acc
is currently .reduce
's initial value: an empty object.
现在使用散布运算符,我们将合并acc
和renamedObject
。 请记住, acc
当前是.reduce
的初始值:一个空对象。
So merging acc
and renamedObject
just results in a clone of renamedObject
.
因此,将acc
和renamedObject
合并只会导致得到一个renamedObject
的克隆。
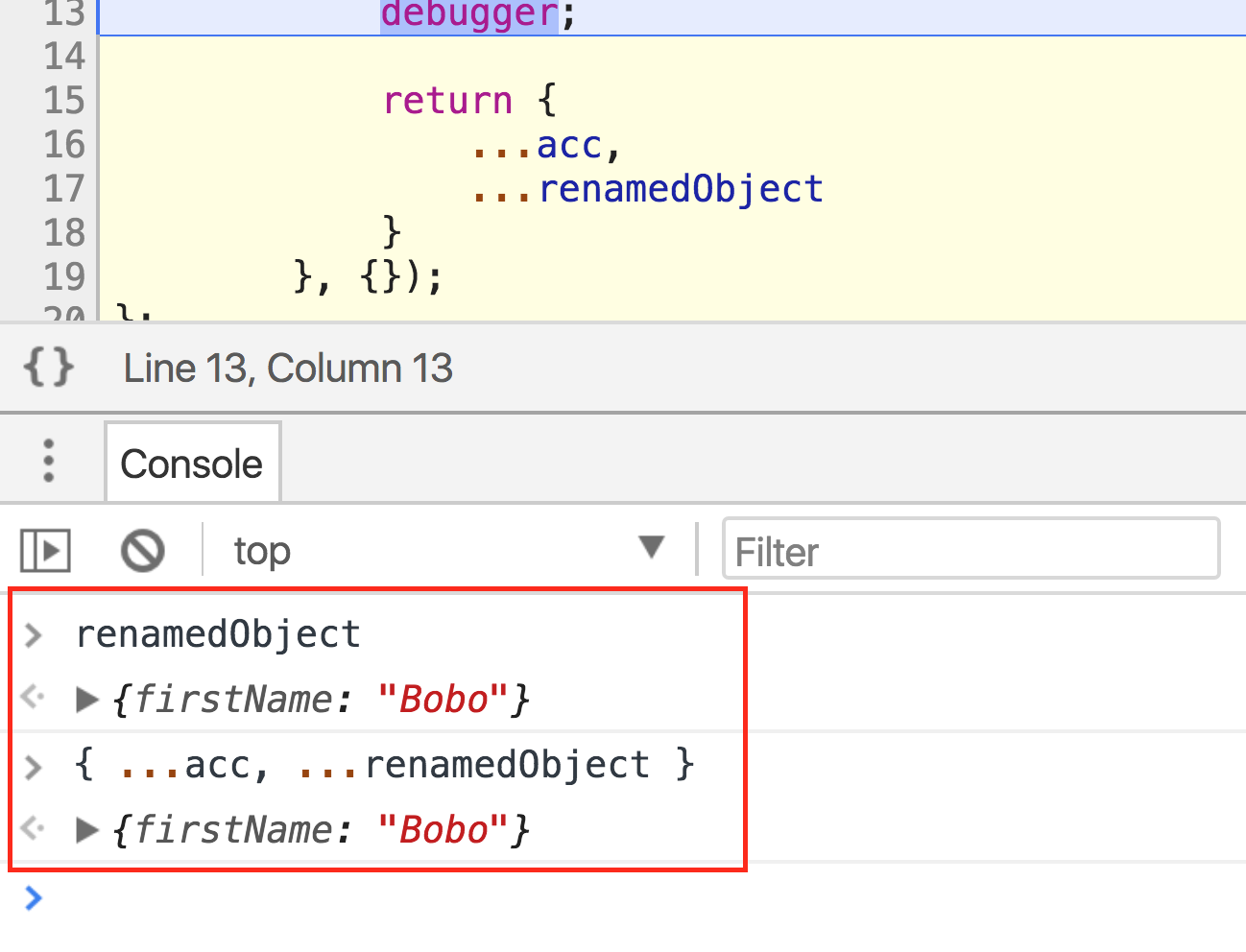
Since we’re returning this object, however, it becomes acc
in .reduce
’s next iteration. Move to the next debugger
to see this.
但是,由于我们返回此对象,因此它在.reduce
的下一次迭代中变为acc
。 移至下一个debugger
以查看此内容。
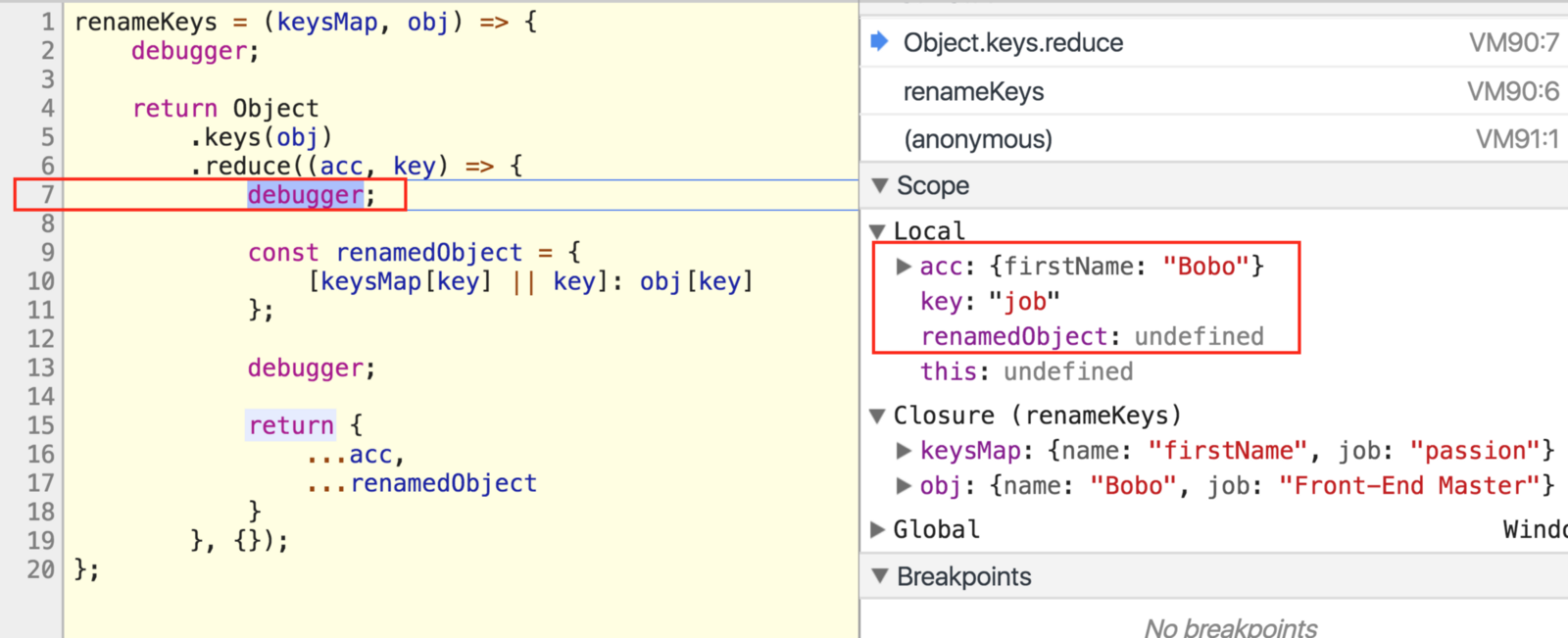
We’re inside .reduce
's again, because there’s one more key
to process. We see that acc
is now { firstName: "Bobo" }
.
我们再次进入.reduce
内部,因为还有另一个要处理的key
。 我们看到acc
现在是{ firstName: "Bobo" }
。
The same process runs again, and renamedObject
is properly created.
再次运行相同的过程,并正确创建了renamedObject
。
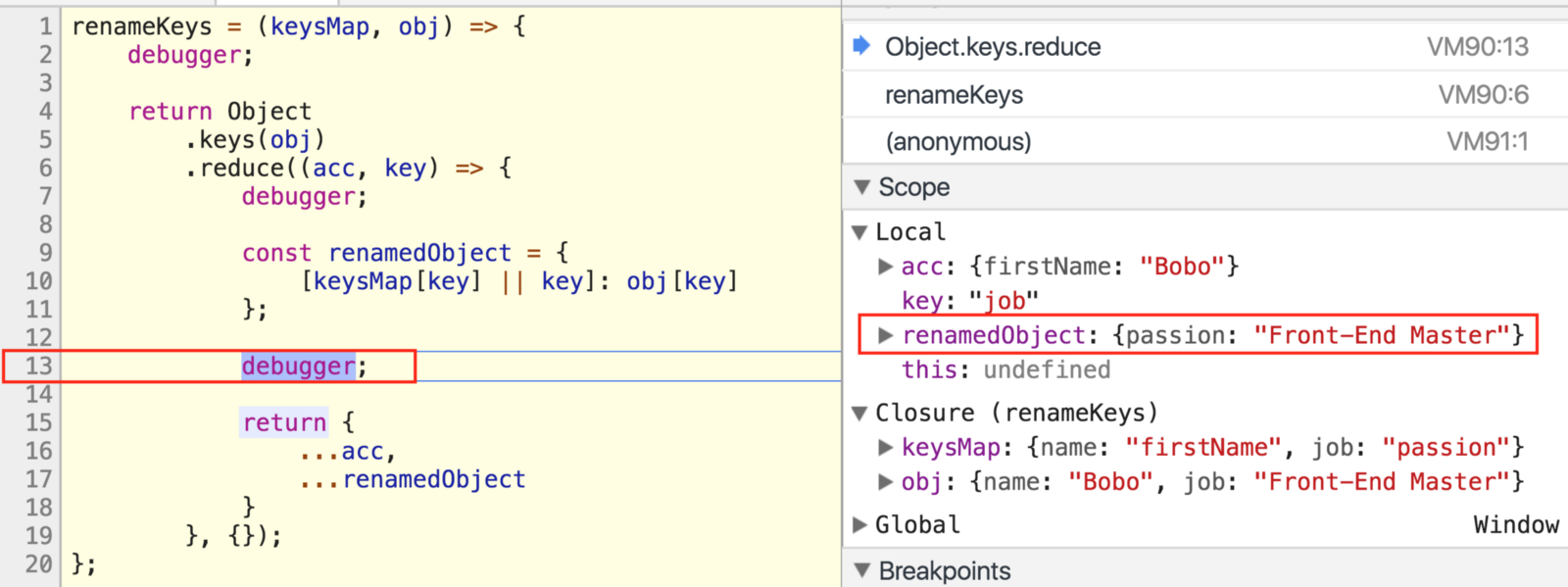
This time, merging acc
and renamedObject
actually makes a difference.
这次,将acc
和renamedObject
合并实际上有所不同。
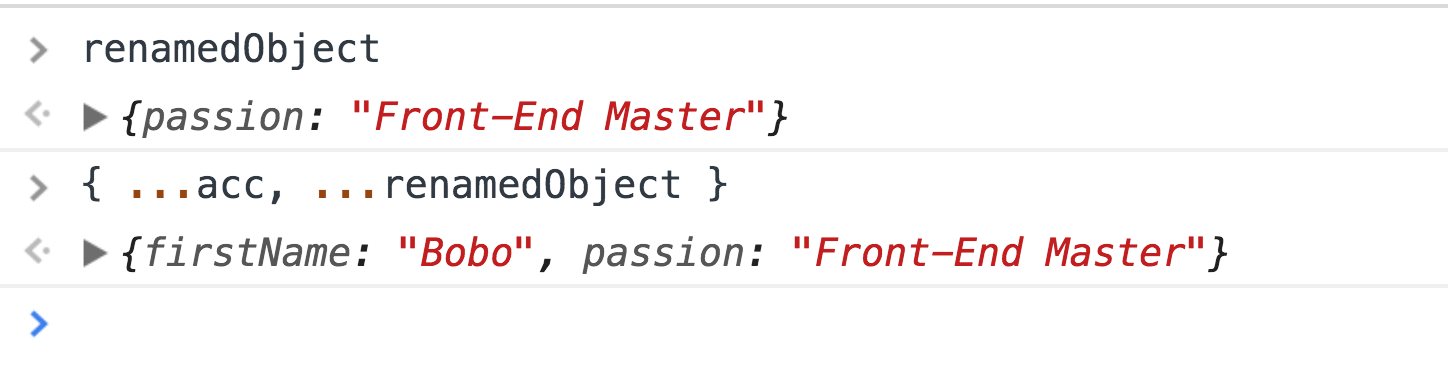
Run past this debugger
to return that object, and you’re done!
经过debugger
以返回该对象,您就完成了!
Here’s the final output:
这是最终的输出:
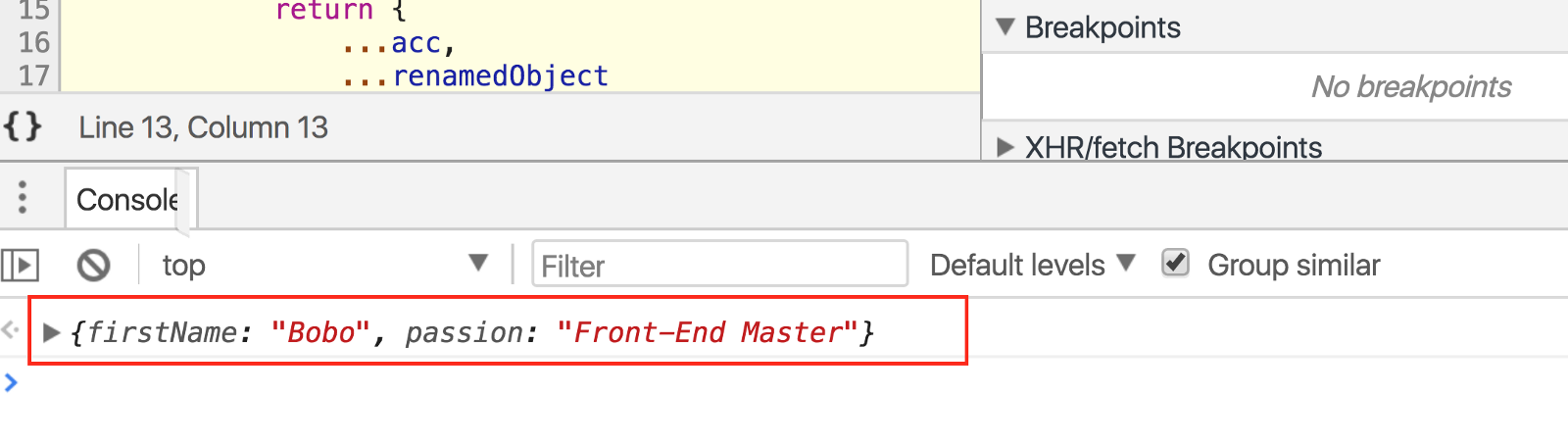
Have fun renaming all the keys, until next time!
重命名所有按键 ,直到下一次,玩得开心!