Scala中的条件语句 (Conditional statements in Scala)
A conditional statement is the one which executes a block of code when a certain condition is fulfilled. Conditional statements help the program in decision making. Control statements or conditional statement decide which block of code need to be executed based on certain criteria.
条件语句是在满足特定条件时执行代码块的条件语句 。 条件语句有助于程序进行决策。 控制语句或条件语句根据某些条件决定需要执行哪个代码块。
In Scala, the following conditional statements are valid:
在Scala中,以下条件语句有效:
if condition: execute block if a condition is true.
if condition :如果条件为true,则执行块。
if - else condition: execute a block if a condition is true otherwise execute another block.
if-else condition :如果条件为true,则执行一个块,否则执行另一个块。
Nested if-else: It checks for another condition inside the conditional block. If condition inside if condition.
嵌套if-else :它检查条件块内的另一个条件。 如果条件在条件内。
if-else if Ladder: Used when multiple conditions need to be checked.
if-else if Ladder :在需要检查多个条件时使用。
1)Scala,如果有条件的话 (1) Scala if conditional statement)
If the condition is used when the user needs to choose between only one condition. This lets program execute a block if the condition is true otherwise continue with the flow of the program.
如果使用条件时,用户仅需在一个条件之间进行选择。 如果条件为真,这将使程序执行一个块,否则继续执行程序流程。
Block execute if condition in the statement is TRUE otherwise nothing is done.
如果语句中的条件为TRUE,则阻止执行,否则不执行任何操作。
Syntax:
句法:
if(condition){
//Statement...
}
Control flow diagram:
控制流程图:
Example code:
示例代码:
object MyClass {
def main(args: Array[String]) {
var myNumber = 21 ;
if(myNumber > 15){
print("Hey your number is bigger than fifteen")
}
print("\nYour number is "+ myNumber)
}
}
Output
输出量
Hey your number is bigger than fifteen
Your number is 21
Example explanation:
示例说明:
In the above example, the If condition check weather myNumber is greater than 15. If it's greater than fifteen the code prints: "Hey your number is bigger than fifteen" If myNumber is not greater than 15 the code continues it general flow.
在上面的示例中,如果条件检查天气myNumber大于15。如果大于15,则代码显示: “嘿,您的数字大于15”。如果myNumber不大于15,则代码继续执行常规流程。
2)if-else条件语句 (2) if-else conditional statement)
if-else condition is used when the user needs to choose between two conditions. This lets program execute a block if the condition is true otherwise execute another block of code and then continue with the flow of the program.
当用户需要在两个条件之间进行选择时,使用if-else条件。 如果条件为真,这将使程序执行一个块,否则执行另一个代码块,然后继续执行程序流程。
Block execute if condition in the statement is TRUE otherwise another block of code is executed.
如果语句中的条件为TRUE,则阻止执行,否则将执行另一个代码块。
Syntax:
句法:
if(condition){
//Statement...
}
else{
//Statement
}
Control flow diagram:
控制流程图:
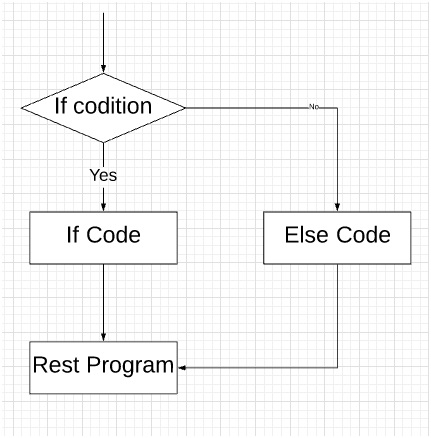
Example code:
示例代码:
object MyClass {
def main(args: Array[String]) {
var myNumber = 21 ;
if(myNumber > 15){
print("Hey your number is bigger than fifteen")
}
else{
print("Hey your number is smaller than fifteen")
}
print("\nYour number is "+ myNumber)
}
}
Output
输出量
Hey your number is bigger than fifteen
Your number is 21
Example explanation:
示例说明:
In the above example, the If condition check weather myNumber is greater than 15. If it's greater than fifteen the code prints: Hey your number is bigger than fifteen If myNumber is not greater than 15 the code prints: Hey your number is smaller than fifteen and then the code flow continues.
在上面的示例中,If条件检查天气myNumber大于15。如果大于15,则代码打印: 嘿,您的数字大于15如果myNumber不大于15,则代码打印: 嘿,您的数字小于15然后代码流继续。
3)嵌套的if-else条件语句 (3) Nested if-else conditional statement)
Nested if-else condition is used when the user needs to choose between a condition that is inside other condition. The flow goes into the first condition then inside the block of code anther condition which executes to execute another block of code.
当用户需要在其他条件内的条件之间进行选择时,使用嵌套的if-else条件。 流程进入第一个条件,然后进入代码块条件内,并执行另一个代码块。
Block execute if condition in the statement is TRUE otherwise another block of code is executed. If the first block of code is executed then inside this block there is another conditional statement that leads to another block of code.
如果语句中的条件为TRUE,则阻止执行,否则将执行另一个代码块。 如果执行了第一个代码块,则在该块内部有另一个条件语句导致另一个代码块。
Syntax:
句法:
if(condition 1){
if(condition 2){
//Statement
}
}
else{
//Statement
}
Control flow diagram:
控制流程图:
Example code:
示例代码:
object MyClass {
def main(args: Array[String]) {
var myNumber = 21 ;
var grade = "a"
if(myNumber > 15){
print("Hey your number is bigger than fifteen")
if( grade == "a"){
print("Your grade is a")
}
}
else{
print("Hey your number is smaller than fifteen")
}
print("\nYour number is "+ myNumber)
}
}
Output
输出量
Hey your number is bigger than fifteen
Your grade is a
Your number is 21
Example explanation:
示例说明:
In this code, two variables are defined as myNumber and grade. First condition checks for myNumber, if it is greater than 15 than the flow gets into the block and prints "Hey your number is bigger than fifteen". then it checks the condition for the grade, if the grade is equal to "a" then it prints "Your grade is a". If myNumber is not greater than 15 then code execute the else statement that is Hey your number is smaller than fifteen. And finally goes through the rest code of the program.
在此代码中,两个变量定义为myNumber和grade 。 第一个条件检查myNumber ,如果它大于15则流进入块并打印“嘿,您的数字大于十五” 。 然后检查等级的条件,如果等级等于“ a”,则显示“您的等级是a” 。 如果myNumber不大于15,则代码执行else语句,嘿,您的数字小于15。 最后遍历程序的其余代码。
4)If-else if Ladder (4) If-else if Ladder)
In the if-else-if loop, the code flow into multiple conditions and if one goes FALSE, then another condition is executed and the condition checks go on. If all the conditions are FALSE, then finally, the else block is executed.
在if-else-if循环中,代码进入多个条件,如果一个条件为FALSE,则执行另一条件,条件检查继续进行。 如果所有条件都为FALSE,则最后执行else块。
The flow goes on with checking conditions one by one. Until any one condition gets TRUE or the final else condition comes.
流程继续逐一检查条件。 直到任何一个条件为TRUE或最终其他条件到来。
Syntax:
句法:
if(condition 1){
//Statement
}
else if(condition 2){
//Statement
}
else if(condition 3){
//Statement
}
.
.
.
else{
//Statement
}
Control flow diagram:
控制流程图:
Example code:
示例代码:
object MyClass {
def main(args: Array[String]) {
var myNumber = 5 ;
if(myNumber > 20){
print("Hey your number is bigger than twenty")
}
else if(myNumber > 15){
print("Hey your number is bigger than fifteen")
}
else{
print("Hey your number is smaller than fifteen")
}
print("\nYour number is "+ myNumber)
}
}
Output
输出量
Hey your number is smaller than fifteen
Your number is 5
Example explanation:
示例说明:
In this code, the program initializes one variable muNumber. Then it checks the value of myNumber to be greater than 20 in the first condition if the condition is true the code prints "Hey your number is bigger than Twenty". If this gets FALSE than it is checked for value to be greater than 15 in the second condition if this gets TRUE than the code prints "Hey your number is bigger than fifteen". At last, if all conditions are FALSE, it goes to the else condition that will execute when all are FALSE and the code prints "Hey your number is smaller than fifteen". After the execution of the else loop, the remaining code will get executed.
在此代码中,程序将初始化一个变量muNumber。 然后,如果条件为true,则在第一个条件中检查myNumber的值是否大于20,代码将输出“嘿,您的数字大于20” 。 如果结果为FALSE,则在第二个条件下检查其值是否大于15(如果结果为TRUE),则代码将显示“嘿,您的数字大于15” 。 最后,如果所有条件都为FALSE,则转到在所有条件都为FALSE时执行的else条件,并且代码显示“嘿,您的数字小于十五” 。 执行else循环后,其余代码将被执行。
翻译自: https://www.includehelp.com/scala/conditional-statements-in-scala.aspx