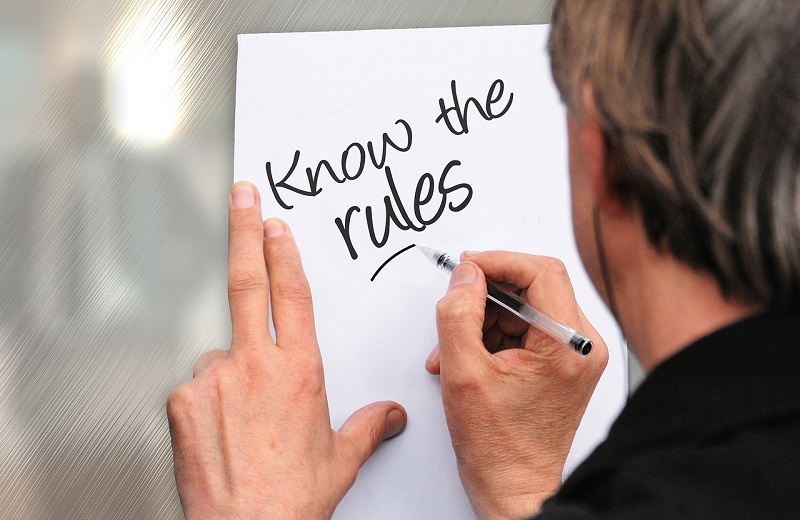
C program is the set of statement written in C programming language. Each program should follow the standards (rules) to make your code working everywhere.
C程序是用C编程语言编写的一组语句。 每个程序都应遵循标准(规则),以使您的代码在任何地方都可以工作。
1)评论/文档 (1) Comments/Documentation)
Comments are not required, but the comments are as important as code. So it is first rule that you should remember to comment/document the code properly. Read more: How to comment the code?
注释不是必需的,但是注释与代码一样重要。 因此,首要规则是您应该记住正确注释/记录代码。 阅读更多: 如何注释代码?
What you can document?
您可以记录什么?
Purpose of the code.
代码的目的。
Name of the programmer, reviewer, code writing, editing date etc.
程序员,审阅者,代码编写,编辑日期等名称。
Resources (inputs) that will be used to run the code.
将用于运行代码的资源(输入)。
Explain each logic, what is that for and why you used?
解释每种逻辑,这是做什么用的,为什么使用?
You can also write note for the developers, in which you can explain your logic/algorithm in details.
您还可以为开发人员写笔记,在其中可以详细说明您的逻辑/算法。
Each function declaration, definition should be documented.
每个函数的声明,定义都应记录在案。
Anything that you can explain, but there is no need to write unnecessary comments with the code that is already cleared. (Read more: Important rules to remember while writing C, C++ program).
您可以解释的所有内容,但无需使用已经清除的代码编写不必要的注释。 (阅读更多: 编写C,C ++程序时要记住的重要规则 )。
2)用分号(;)终止语句 (2) Statement termination by semicolon (;))
Each statement which does not has its body (please note that, statement without its body) must be terminated by the semicolon (;).
没有主体的每个语句(请注意,没有主体的语句)必须以分号(;)终止。
The statements which should be terminated
应当终止的陈述
All printf(), scanf() or any other function calls.
所有printf() , scanf()或任何其他函数调用。
All declarations like variables, constants, function, structures must be terminated by semicolon.
所有声明(例如变量,常量,函数,结构)都必须以分号终止。
All expressions must be terminated by the semicolon (;).
所有表达式必须以分号(;)终止。
Examples:
例子:
int a; //variable declarations
printf("Hello!"); // a print statement
sum = findSum (10,20,30); // a function calling statement
//etc
The statements which should not be terminated
不应终止的陈述
Header files include statements.
头文件包含语句。
Macro definition statements.
宏定义语句。
If statement, loop statements, function header with the function definitions.
if语句,循环语句,带有函数定义的函数头。
#include <stdio.h> //hesder file include section
#define MAX_LEN 1024 //Macro definition statement
int main() //main function defining section
{
//...
}
//function header with its definition
int findSum(int a, int b, int c)
{
return (a+b+c);
}
if(a > b) //if statement
large =a;
else
large =b;
//etc
3)令牌,标识符相关规则 (3) Tokens, identifiers related rules)
You must be aware about the C language tokens to differentiate what is keyword, what is identifier etc.
您必须了解C语言令牌,才能区分什么是关键字,什么是标识符等。
Some of the points that you must know:
您必须知道的一些要点:
Keywords are the reserved words in the compilers and you cannot use them for other purposes.
关键字是编译器中的保留字,您不能将其用于其他目的。
C language is cases sensitive programming language. Here, small case identifiers and uppercase identifiers are different. So, if you have declared a variable with name Num then you must have to use Num everywhere. You cannot use num, NUM etc instead of Num.
C语言是区分大小写的编程语言。 在此,小写字母标识符和大写字母标识符不同。 因此,如果您声明了一个名称为Num的变量,那么您必须在所有地方都使用Num 。 您不能使用num , NUM等代替Num 。
Same as keyword, library functions are also declared in the header files and you must have to include header file in the program. For example, if you are using sqrt() function to find the square root of any number, you will have to include math.h header file.
与关键字相同,库函数也在头文件中声明,并且您必须在程序中包括头文件。 例如,如果使用sqrt()函数查找任何数字的平方根,则必须包括math.h头文件。
4)关于标识符/变量声明的规则 (4) Rules about identifier/variable declarations)
Lowercase, uppercase alphabets, digits and underscore (_) are allowed to define an identifier (i.e. variable name, constant name, function name etc.).
允许使用小写,大写字母,数字和下划线(_)定义标识符(即变量名,常量名,函数名等)。
Only an alphabet or underscore (_) can be used as first letter of the identifier.
只能将字母或下划线(_)用作标识符的首字母。
White spaces and other special characters are not allowed in an identifier name, if you have two words in a variables/identifier name, you can use either underscore (_) to separate them or you can also write identifier name in camel case style.
标识符名称中不允许使用空格和其他特殊字符,如果变量/标识符名称中包含两个单词,则可以使用下划线(_)分隔它们,也可以使用驼峰式的样式写标识符名称。
For example: if you want to declare a variable to store roll number, the variable name should be
例如:如果要声明一个变量来存储卷号,则变量名应为
roll_number or rollNumber or anything like that but you cannot use white spaces.
roll_number或rollNumber或类似的名称,但不能使用空格。
Identifier must be meaningful and descriptive.
标识符必须有意义且具有描述性。
Read more: Identifier/variable name conventions in C language
阅读更多: C语言中的标识符/变量名称约定
5)代码缩进 (5) Code indentation )
I have deal with many programmers who just write the code and they don’t care about the indentation, since indentation is not compulsory for the compiler. But it’s most important while writing the program. An indented code can read, edit easily.
我与许多只写代码的程序员打交道,他们并不关心缩进,因为缩进对于编译器不是强制性的。 但这在编写程序时最重要。 缩进的代码可以轻松读取,编辑。
Example of indented code:
缩进代码示例:
#include <stdio.h>
int main()
{
int a;
int b;
int large;
a = 10;
b = 20;
if(a>b)
{
large = a;
}
else
{
large = b;
}
printf("Largest number is: %d\n",large);
return 0;
}
Example of un-indented code:
缩进代码示例:
#include <stdio.h>
int main()
{
int a;
int b;
int large;
a = 10;
b = 20;
if(a>b)
{
large = a;
}
else
{
large = b;
}
printf("Largest number is: %d\n",large);
return 0;
}
Search for C programming courses for beginners on classpert.com
在classpert.com上为初学者搜索C编程课程
翻译自: https://www.includehelp.com/c/some-basic-rules-of-writing-a-c-program.aspx