jquery选择器连续选择
It's time to write some JQuery now. Do check out the introductory article on JQuery first in case you haven't. Before we move to Selectors in JQuery, let's talk a bit about the general syntax first.
现在该写一些JQuery了。 如果没有,请先查看有关JQuery的介绍性文章 。 在转到JQuery中的Selectors之前,让我们先谈谈常规语法。
陈述 (Statements)
Almost everything in JQuery is a statement. This may not be the first time you're hearing this because most programming languages conceive every distinguishable line of code as a statement. In JQuery, all statements are preceded with a $ (dollar sign). This is also known as the JQuery keyword. For instance,
JQuery中的几乎所有内容都是一个语句 。 这可能不是您第一次听到此消息,因为大多数编程语言将每条可区分的代码行都视为一条语句。 在JQuery中,所有语句都以$(美元符号)开头。 这也称为JQuery关键字 。 例如,
document.getElementById("#sub-text");
$("#sub-text");
We have used a CSS selector above using JQuery. You can see how easy it is to select an element using a CSS selector in JQuery. You just write the JQuery keyword ($ sign) followed by a pair of parentheses ( () ) and put the CSS selector inside those parentheses.
我们在上面使用JQuery使用了CSS选择器 。 您可以看到在JQuery中使用CSS选择器选择元素有多么容易。 您只需编写JQuery关键字( $ sign ),然后加上一对括号(()) ,然后将CSS选择器放在这些括号内。
The above two statements, the former in Vanilla JS and the later in JQuery essentially do the same thing however there is a small, subtle yet important difference to note. Before we understand that and start coding some JQuery let's create a simple HTML page that we can play around with. I'm taking this boilerplate from the introductory article. All I have done till now is added materialize CDN for writing cool styles quickly and link to our local stylesheet,
上面的两个语句,在Vanilla JS中的前者和在JQuery中的后一个本质上是做相同的事情,但是要注意一个小的,微妙的但重要的区别。 在我们了解这一点并开始编写一些JQuery代码之前,让我们创建一个可以使用的简单HTML页面。 我将从介绍性文章中摘录该样板。 到目前为止,我所做的只是添加了实现CDN,以便快速编写酷炫的样式并链接到我们的本地样式表,
Index.html
Index.html
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<!-- Compiled and minified CSS -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/materialize/1.0.0/css/materialize.min.css">
<!-- Compiled and minified JavaScript -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/materialize/1.0.0/js/materialize.min.js"></script>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<script src="index.js"></script>
</body>
</html>
Let's add some content quickly so we can start using JQuery
<div class="container">
<h1 class="title">Explaining JQuery to Spongebob</h1>
<p id="intro-text">Can I say one word or two about Spongebob first?</p>
<div class="row">
</div>
<div class="card">
<div class="card-content">
<h5 class="sub-title">List of names of spongebob's buddies are</h5>
<ul class="center collection" id="list">
<li>Mr Krabs</li>
<li>Garry</li>
<li>Squidward Tentacles</li>
<li>Mrs Puff</li>
</ul>
</div>
</div>
</div>
Output
输出量
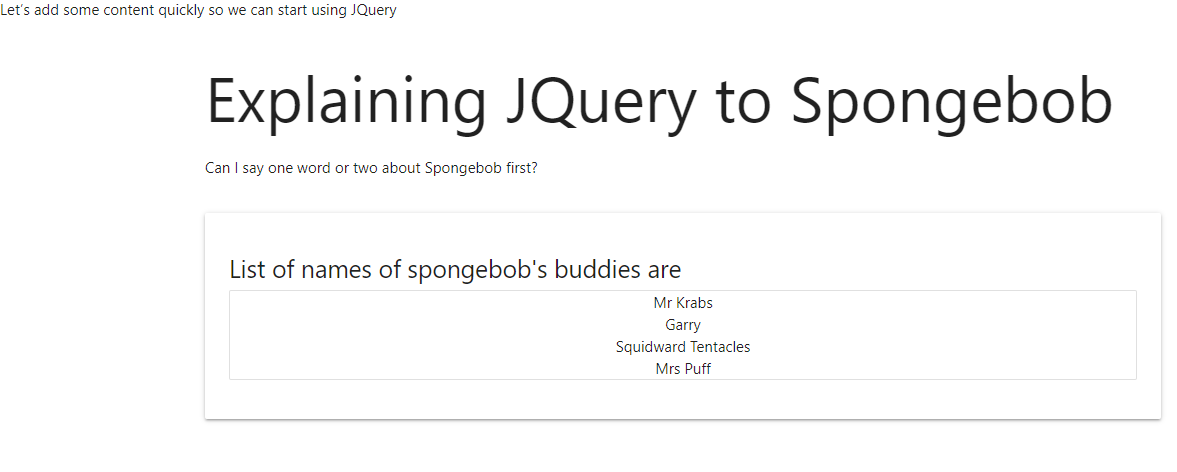
Now that we have the template setup, let's first see that subtle difference we spoke about,
现在我们有了模板设置,让我们首先看看我们谈到的细微差别,
console.log(document.querySelector('.title'));
console.log($('.title'));
The above two statements should essentially do the same thing, and they actually do. They both get us a reference to the HTML element with a class name of the title. However,
上面的两个语句在本质上应该做同样的事情,并且实际上是在做。 它们都为我们提供了带有标题类名HTML元素的引用。 然而,
Output
输出量
<h1 class="title">Explaining JQuery to Spongebob >/h1>
k.fn.init [h1.title, prevObject: k.fn.init(1)]
The second statement returns us somewhat an array wrapped around the element. It looks a lot like an array however, to be accurate it's a JQuery Object. No matter what you reference to, a JQuery statement always returns a JQuery Object. You can also verify this,
第二条语句返回一些包裹在元素周围的数组。 它看起来很像一个数组,但准确地说,它是一个JQuery对象。 无论引用什么,JQuery语句始终返回JQuery对象。 您也可以验证
typeof(title);
Output
输出量
"Object"
Why JQuery wraps elements in a JQuery object wrapper is simply because we have loads of different properties and methods available to us then? This becomes very useful when we're animating elements using JQuery.
为什么JQuery在JQuery对象包装器中包装元素仅仅是因为我们拥有大量可用的不同属性和方法呢? 当我们使用JQuery为元素设置动画时,这将非常有用。
title.animate;
Output
输出量
ƒ (t,e,n,r){var i=k.isEmptyObject(t),o=k.speed(e,n,r),a=function(){var e=dt(this,k.extend({},t),o);(i||Q.get(this,"finish"))&&e.stop(!0)};return a.finish=a,i||!1===o.queue?this.each(a):this.queue(o.que...
However, we also have the freedom to unwrap our element and return a regular Vanilla JS object and use the common Vanilla JS methods available on it.
但是,我们也可以自由地展开元素并返回常规的Vanilla JS对象,并使用其上可用的常见Vanilla JS方法。
title[0];
Output
输出量
<h1 class="title">Explaining JQuery to Spongebob</h1>
Notice how the 0th element inside the JQuery object is actually that HTML element so we can simply obtain it using the indexing method. However, now we don't have access to the animation methods,
注意,JQuery对象中的第0个元素实际上是那个HTML元素,因此我们可以简单地使用索引方法来获取它。 但是,现在我们无法访问动画方法,
title[0].animate;
Output
输出量
ƒ animate() { [native code] }
Selectors are used to grab content from the web page. We can use simple CSS selectors to grab elements from the DOM using JQuery.
选择器用于从网页中获取内容。 我们可以使用简单CSS选择器通过JQuery从DOM中获取元素。
const title=$('.container h1');
console.log(title);
Output
输出量
k.fn.init [h1.title, prevObject: k.fn.init(1)]
We can also target ids.
我们还可以定位ID。
const list=$('#list');
console.log(list);
Output
输出量
k.fn.init [ul#list.center.collection]
0: ul#list.center.collection
length: 1
__proto__: Object(0)
If you know CSS, using JQuery selectors will come super easy to you.
如果您知道CSS,那么使用JQuery选择器对您来说将非常容易。
list[0];
Output
输出量
<ul class="center collection" id="list">...</ul>
We can also get the first <li> using,
我们还可以使用第一个<li>
const firstFriend=$('ul li:first')[0];
console.log(firstFriend);
Output
输出量
<li>Mr. Krabs</li>
The *is the universal selectors and grabs the whole HTML of the page.
*是通用选择器,可获取页面的整个HTML。
const everything=$('*')[0];
console.log(everything);
Output
输出量
<html lang="en"><head>…</head><body cz-shortcut-listen="true">...</body></html>
翻译自: https://www.includehelp.com/code-snippets/selectors-in-jquery.aspx
jquery选择器连续选择