单链表遍历
单链表 (Single linked list)
Single linked list contains a number of nodes where each node has a data field and a pointer to next node. The link of the last node is to NULL, indicates end of list.
单个链表包含许多节点,其中每个节点都有一个数据字段和一个指向下一个节点的指针。 最后一个节点的链接为NULL,表示列表结尾。
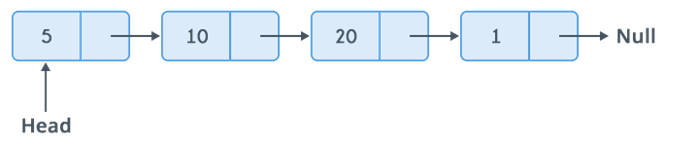
单链表结构 (Single linked list structure)
The node in the linked list can be created using struct.
可以使用struct创建链接列表中的节点。
struct node{
// data field, can be of various type, here integer
int data;
// pointer to next node
struct node* next;
};
链表上的基本操作 (Basic operations on linked list)
Traversing
遍历
Inserting node
插入节点
Deleting node
删除节点
单个链表的遍历技术 (Traversing technique for a single linked list)
Follow the pointers
跟随指针
Display content of current nodes
显示当前节点的内容
Stop when next pointer is NULL
当下一个指针为NULL时停止
C代码遍历链接列表并计算节点数 (C code to traverse a linked list and count number of nodes )
#include <stdio.h>
#include <stdlib.h>
struct node{
int data; // data field
struct node *next;
};
void traverse(struct node* head){
struct node* current=head; // current node set to head
int count=0; // to count total no of nodes
printf("\n traversing the list\n");
//traverse until current node isn't NULL
while(current!=NULL){
count++; //increase node count
printf("%d ",current->data);
current=current->next; // go to next node
}
printf("\ntotal no of nodes : %d\n",count);
}
struct node* creatnode(int d){
struct node* temp= (struct node*) malloc(sizeof(struct node));
temp->data=d;
temp->next=NULL;
return temp;
}
int main()
{
printf("creating & traversing linked list\n");
//same linked list is built according to image shown
struct node* head=creatnode(5);
head->next=creatnode(10);
head->next->next=creatnode(20);
head->next->next->next=creatnode(1);
traverse(head);
return 0;
}
Output
输出量
creating & traversing linked list
traversing the list
5 10 20 1
total no of nodes : 4
Time complexity:
时间复杂度:
O(n), for scanning the list of size n
O(n) ,用于扫描大小为n的列表
Space complexity:
空间复杂度:
O(1), no additional memory required
O(1) ,不需要额外的内存
单链表遍历