路径总和
Problem statement
问题陈述
Given a Binary Tree T and a sum S, write a program to check whether there is a root to leaf path in that tree with the input sum S.
给定二叉树T和总和S ,编写一个程序来检查输入树的总和S是否在该树中存在根到叶的路径 。
Example:
例:
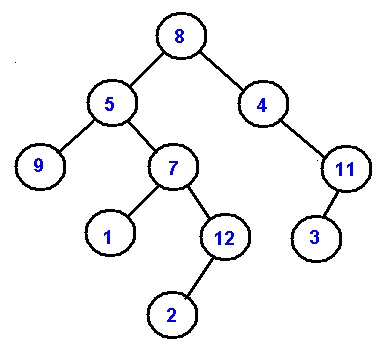
Fig: Binary Tree
无花果:二叉树
In the above example, the root is 8 & the leaf nodes are 9,1,2,3. There are many root to leaf paths like:
8->5->9
8->5->7->1
8->5->7->12->2
And so on.
在上面的示例中,根为8,叶节点为9,1,2,3 。 叶子到根的路径很多,例如:
8-> 5-> 9
8-> 5-> 7-> 1
8-> 5-> 7-> 12-> 2
等等。
The sum for a root to leaf path is the sum of all intermediate nodes, the root & leaf node, i.e., sum of all the nodes on the path. Like for the first path in the above example the root to leaf path sum is 22 (8+5+9)
根到叶路径的总和是所有中间节点(根与叶节点)的总和,即路径上所有节点的总和。 就像上面示例中的第一个路径一样, 根到叶的路径总和为22(8 + 5 + 9)
Our program must be to determine whether the given sum is same as anythe root to leaf path sum. There may be case that more than one root to leaf path has exactly same sum of S, but that’s not of any concern, Our function is a Boolean function to return only yes or not on basis of input.
我们的程序必须是确定给定的总和是否与根到叶的路径总和相同。 可能存在多个根到叶路径具有完全相同的S之和的情况,但这无关紧要。我们的函数是一个布尔函数,根据输入仅返回yes或不返回。
Let for an example input:
让我们输入一个示例:
S= 26 & T be the above binary tree in example
S = 26 & T是上述示例中的二叉树
Our program should return "YES" as there is a root to leaf path exists which is 8->4->11->3
我们的程序应返回“ YES”,因为存在到叶路径的根,即8-> 4-> 11-> 3
S=8 & T be the above binary tree in example
S = 8 & T是上面的二叉树示例
Our program should return "NO" as there is no root to leaf path exists which has sum 8
我们的程序应返回“ NO”,因为不存在存在到总和为8的叶路径的根
Algorithm
算法
We need to construct a Boolean function hasRootToLeafSum () which will accept a sum value S & a binary tree T
我们需要构造一个布尔函数hasRootToLeafSum() ,该函数将接受和值S和二叉树T
We can implement the above function using recursion. The idea is to subtract current node value & continue to check for both subtrees recursively until we reach the base case. The base case can be of two type:
我们可以使用递归来实现上述功能。 想法是减去当前节点值并继续递归检查两个子树,直到我们达到基本情况为止。 基本案例可以有两种类型:
Root node for the subtrees are NULL & sum is 0 too. This is a valid terminating step. We return TRUE here.
子树的根节点为NULL,总和也为0。 这是一个有效的终止步骤。 我们在这里返回TRUE 。
The sum is 0 & we have reached a leaf node. This is of course the valid terminating step. We return TRUE here.
总和为0,我们已经到达叶节点。 当然,这是有效的终止步骤。 我们在这里返回TRUE 。
FunctionhasRootToLeafSum (sum value S, root of Binary tree T)
函数hasRootToLeafSum(总和S,二叉树的根T)
1. Set path to FALSE. Path represent the Boolean
variable which determines whether there is a root to leaf pathor not.
2. Consider the base cases.
• If (root==NULL&&S==0) //case a
return true;
• Subtract the root node value & check whether we have reached
the leaf node with remaining S, 0.
If (S==0 && root->left==NULL &&root->right==NULL)
return true;
3. Recursively check for both right & left subtrees.
If(root->left) //for left subtree
path=path||hasRootToLeafSum (root->left, S);
If (root->right)//for right subtree
path=path||hasRootToLeafSum (root->right, S);
4. Return path ( FALSE or TRUE)
If the function return FALSE then no path is found else there is a path.
根到叶路径总和的C ++实现 (C++ implementation of Root to leaf Path Sum)
#include<bits/stdc++.h>
using namespace std;
class tree{ //tree node
public:
int data;
tree *left;
tree *right;
};
bool hasRootToLeafSum(tree *root, int s){
bool path=false; //declare boolean variable path
//base condition checking
if(root==NULL && s==0)
return true;
s-=root->data; //subtract current root value
//checking whether leaf node reached and remaining sum =0
if(s==0 && root->left==NULL && root->right==NULL)
return true;
//recursively done for both subtrees
if(root->left){//for left subtree
path=path||hasRootToLeafSum(root->left, s);
}
if(root->right){//for right subtree
path=path||hasRootToLeafSum(root->right, s);
}
return path;
}
tree* newnode(int data){ //creating new nodes
tree* node = (tree*)malloc(sizeof(tree));
node->data = data;
node->left = NULL;
node->right = NULL;
return(node);
}
int main() {
//**same tree is builted as shown in example**
cout<<"tree in the example is build here"<<endl;
//building the tree like as in the example
tree *root=newnode(8);
root->left= newnode(5);
root->right= newnode(4);
root->right->right=newnode(11);
root->right->right->left=newnode(3);
root->left->left=newnode(9);
root->left->right=newnode(7);
root->left->right->left=newnode(1);
root->left->right->right=newnode(12);
root->left->right->right->left=newnode(2);
int s;
cout<<"enter input sum S......"<<endl;
cin>>s;
if(hasRootToLeafSum(root,s))//if there exists such a path
cout<<"A root to leaf path with this sum exists"<<endl;
else
cout<<"No such a path exists"<<endl;
return 0;
}
Output
输出量
First run:
tree in the example is build here
enter input sum S......
26
A root to leaf path with this sum exists
Second run:
tree in the example is build here
enter input sum S......
8
No such a path exists
翻译自: https://www.includehelp.com/icp/root-to-leaf-path-sum.aspx
路径总和