JavaScript表格 (JavaScript Forms)
One of the key tasks of a frontend developer is to carry out form validations for certain types of fields in a form. You must have noticed when you're setting up a password for your account on a website, you're allowed to enter only certain characters. That password field is an input field that is a part of the form that comprises other fields such as username, email, etc. In general, almost every website needs a form since users sign up and login is nothing but an HTML form that takes values from the user.
前端开发人员的关键任务之一是对表单中某些类型的字段执行表单验证 。 在网站上为帐户设置密码时,您一定已经注意到,只允许输入某些字符。 该密码字段是输入字段,它是包含其他字段(例如用户名,电子邮件等)的表单的一部分。通常,几乎每个网站都需要一个表单,因为用户注册和登录只不过是带有值HTML表单来自用户。
On filling the form, you press sign up or log in which performs certain other operations like taking you to your account or prompting an error if there was a login error. Your form on the web reacts to events that are triggered on clicking the submit button or pressing enter if the field is in focus. Hence forms are quite important to understand. Since they're never static, we dynamically interact with the user using the form field to carry out the next sequence of tasks. In this article, we'll look at how to build a basic form and how to react to events of the form?
在填写表格时,您可以按注册或登录来执行某些其他操作,例如将您带到您的帐户或在出现登录错误时提示错误。 如果您关注该字段,则您在Web上的表单会对单击提交按钮或按Enter触发的事件做出React。 因此,表格非常重要。 由于它们永远不是静态的,因此我们使用form字段与用户进行动态交互以执行下一个任务序列。 在本文中,我们将研究如何构建基本表单以及如何对表单事件做出React?
Let's get started by creating a simple HTML form with some basic styles...
让我们开始创建具有一些基本样式的简单HTML表单 ...
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Forms</title>
</head>
<style>
form {
margin: 20px auto;
padding: 20px 20px;
text-align: center;
width: 400px;
height: 400px;
background: rgba(85, 84, 165, 0.356);
}
h2 {
font-family: 'Gill Sans', 'Gill Sans MT', Calibri, 'Trebuchet MS', sans-serif;
font-size: 40px;
color: white;
}
input {
background: white;
border: 2px solid gainsboro;
height: 30px;
padding: 10px auto;
margin: 10px auto;
}
input::placeholder {
color: rgba(86, 90, 141, 0.61);
text-transform: uppercase;
letter-spacing: 3px;
text-align: center;
}
input[type=submit] {
border: 2px solid gainsboro;
border-radius: 8px;
cursor: pointer;
background-color: rgb(97, 176, 179);
color: whitesmoke;
padding: 5px 5px;
margin: 10px;
text-transform: uppercase;
box-shadow: 2px 2px 2px rgb(15, 52, 83);
}
</style>
<body>
<form action="">
<h2>Form</h2>
<input type="text" placeholder="name" id="name">
<input type="submit" value="submit">
</form>
</body>
Okay so now we have a very basic form ready which looks something like this.
好的,现在我们已经准备好一个非常基本的表单,看起来像这样。
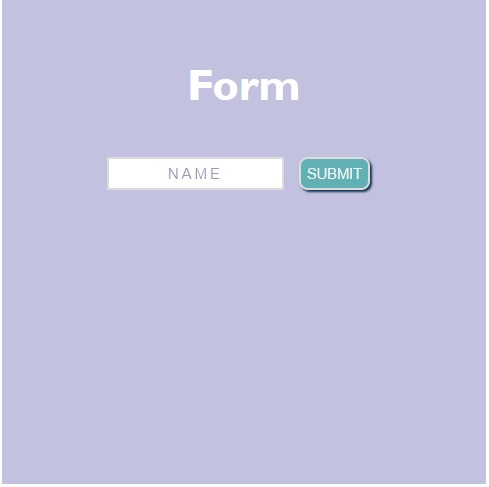
Okay so let's imagine you have this form on your website and you need to send the information about the name user enters your backend which validates this name in their database and sends a response. Imagine this like a very basic version of the login form. We have a simple name field and every user in our database has a unique name and on clicking submit we need to check if the name user-entered exists in our database or not for login. Thus we need to capture the value entered in the input field and bind that to an event listener to our submit button.
好的,让我们假设您的网站上有此表单,并且需要发送有关用户输入您的后端的名称的信息,该后端将在他们的数据库中验证该名称并发送响应。 可以将其想象成一个非常基本的登录表单版本。 我们有一个简单的名称字段,数据库中的每个用户都有一个唯一的名称,单击“提交”后,我们需要检查用户输入的名称是否存在于我们的数据库中或不用于登录。 因此,我们需要捕获在输入字段中输入的值,并将其绑定到事件侦听器的提交按钮。
An easy way to do this is to use the click event on the submit button. However, click events only react to clicks and a versatile form reacts to pressing enter when the field is in focus. Hence we will use a submit event for this purpose.
一种简单的方法是在提交按钮上使用click事件。 但是,单击事件仅对单击做出React,而通用字段则在焦点突出时对按Enter做出React。 因此,我们将为此目的使用Submit事件。
So first let's capture the submit button and add a submit event to it...
因此,首先让我们捕获提交按钮并向其添加一个提交事件 ...
<script>
const form = document.querySelect('form');
form.addEventListener('submit', e => {})
</script>
The default behavior of the submit event is to reload the page. Try clicking the submit button you'll see your page reloading. We don't need that, so let's remove it. We have the event object available to us and we can tell our form using that event object to prevent the default behavior of our submit event.
Submit事件的默认行为是重新加载页面。 尝试单击“提交”按钮,您将看到页面正在重新加载。 我们不需要它,因此让我们将其删除。 我们有可用的事件对象,我们可以使用该事件对象告诉表单,以防止提交事件的默认行为。
e.preventDefault();
Alright, now let's capture the value of our input field. We have a traditional way of doing this in which we first add a query selector and then use the value property.
好了,现在让我们捕获输入字段的值。 我们有一种传统的实现方法,首先添加查询选择器,然后使用value属性。
const name=document.querySelector('#name')
console.log(name.value);
We can also directly use the form variable to get the value of the input field by referencing the id assigned to that field.
我们还可以通过引用分配给该字段的ID,直接使用form变量获取输入字段的值。
console.log(form.name.value);
In the case of multiple input fields in a form, this method comes in very handy as it saves time and code where we attach every input field to a variable to reference it.
对于表单中的多个输入字段,此方法非常方便,因为它节省了时间和代码,我们将每个输入字段附加到变量以引用它。
< script >
const form = document.querySelector('form');
//const name=document.querySelector('#name');
form.addEventListener('submit', e => {
e.preventDefault();
//console.log(name.value);
console.log(form.name.value);
})
< /script>
That's how you listen to form events and capture the input field values.
这就是您侦听表单事件并捕获输入字段值的方式。
We can do all sorts of things by triggering the submit event.
我们可以通过触发Submit事件来做各种各样的事情。
HTML provides us with some basic validations that we can add in our form. Let's try to implement these. First, let's restructure our form and add some more input fields.
HTML为我们提供了一些基本的验证,可以将其添加到表单中。 让我们尝试实现这些。 首先,让我们重组表单并添加更多输入字段。
<form action="">
<h2>Form</h2>
<input type="text" placeholder="name" id="name">
<br>
<input type="text" placeholder="email" id="email">
<br>
<input type="text" placeholder="password" id="password">
<br>
<input type="text" placeholder="userid" id="name">
<br>
<input type="text" placeholder="date of joining" id="dateofjoining">
<br>
<input type="submit" value="submit">
</form>
We have an email, password, and date of joining fields as well. The type property of the input field is an in build validator for the outfield. Currently, all fields have a type of text which states that these fields can be populated by any text which could basically include any and every character. Logically, we must not let the user enter any letters in the userid field, the date of joining should be made a date picker for proper formatting and data uniformity. Similarly, we should prevent showing the password to the user as she types for security reasons. We can do these by simply setting the corresponding types to the fields depending on the values we want the user to enter.
我们也有电子邮件 , 密码和加入字段的日期 。 输入字段的type属性是外场的内置验证器。 当前,所有字段都有一种文本类型,指出这些字段可以由基本上可以包含任何字符的任何文本填充。 从逻辑上讲,我们绝不能让用户在userid字段中输入任何字母,加入日期应设为日期选择器,以实现正确的格式设置和数据统一性。 同样,出于安全原因,我们应避免在用户输入密码时向其显示密码。 我们可以通过简单地将相应类型设置为字段来完成这些操作,具体取决于我们希望用户输入的值。
<form action="">
<h2>Form</h2>
<input type="text" placeholder="name" id="name">
<br>
<input type="text" placeholder="email" id="email">
<br>
<input type="password" placeholder="password" id="password">
<br>
<input type="number" placeholder="userid" id="name">
<br>
<input type="date" placeholder="date of joining" id="dateofjoining">
<br>
<input type="submit" value="submit">
</form>
We have used the type number for userID, added a date picker to the date of joining and the type password hides the text that the user enters. Thus we have added some checks to our fields which makes our form a little better than before.
我们已将类型编号用于userID ,在加入日期中添加了一个日期选择器,并且类型密码隐藏了用户输入的文本。 因此,我们在字段中添加了一些检查,这使我们的表单比以前更好了。
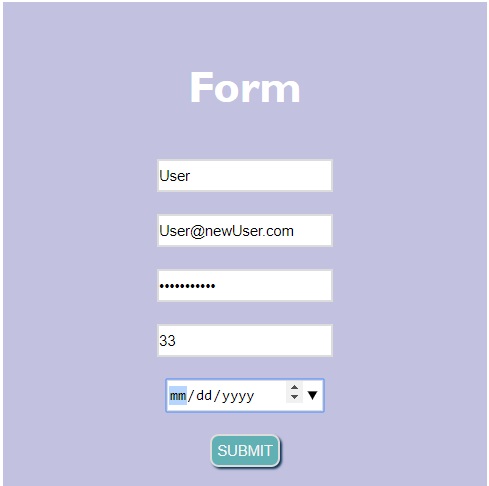
If you want to learn how to validate forms, read the article on Regular Expressions in JavaScript where we build a more intensive form and validate their fields using regular expressions.
如果您想学习如何验证表单,请阅读有关JavaScript中正则表达式的文章,我们在其中构建了更深入的表单,并使用正则表达式验证其字段。
翻译自: https://www.includehelp.com/code-snippets/forms-in-javascript.aspx