红黑树节点插入和旋转
红黑树 (Red Black Tree )
A Red Black Tree is a type of self-balancing binary search tree, in which every node is colored with a red or black. The red black tree satisfies all the properties of the binary search tree but there are some additional properties which were added in a Red Black Tree. The height of a Red-Black tree is O(Logn) where (n is the number of nodes in the tree).
红黑树是一种自平衡二进制搜索树,其中的每个节点都用红色或黑色着色。 红黑树满足二进制搜索树的所有属性,但在红黑树中添加了一些其他属性。 一棵红黑树的高度是O(Logn),其中(n是树中的节点数)。
红黑树的性质 (Properties of Red Black Tree)
The root node should always be black in color.
根节点应始终为黑色。
Every null child of a node is black in red black tree.
节点的每个空子节点在红黑树中都是黑色的。
The children of a red node are black. It can be possible that parent of red node is black node.
红色节点的子节点为黑色。 红色节点的父节点可能是黑色节点。
All the leaves have the same black depth.
所有叶子都具有相同的黑色深度。
Every simple path from the root node to the (downward) leaf node contains the same number of black nodes.
从根节点到(向下)叶节点的每个简单路径都包含相同数量的黑色节点。
红黑树的表示形式 (Representation of Red Black Tree)
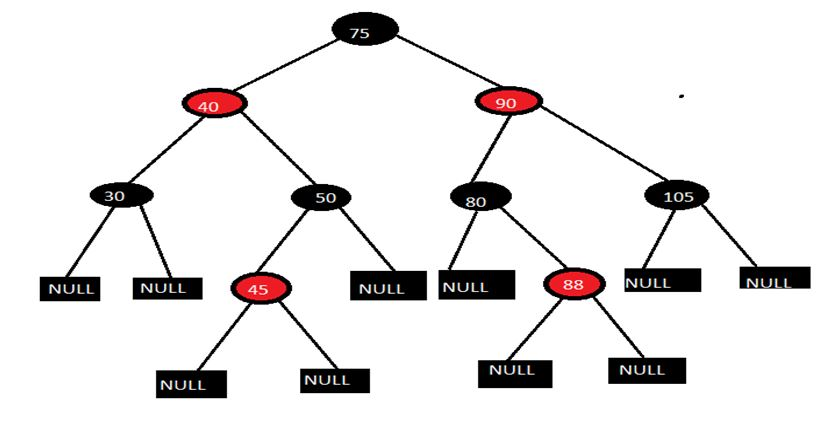
While representing the red black tree color of each node should be shown. In this tree leaf nodes are simply termed as null nodes which means they are not physical nodes. It can be checked easily in the above-given tree there are two types of node in which one of them is red and another one is black in color. The above-given tree follows all the properties of a red black tree that are
代表红黑树时,应显示每个节点的颜色。 在该树中,叶节点简称为空节点,这意味着它们不是物理节点。 在上面给出的树中可以很容易地检查它,其中有两种类型的节点,其中一种是红色,另一种是黑色。 上面给出的树遵循红黑树的所有属性,即
It is a binary search tree.
它是二叉搜索树。
The root node is black.
根节点是黑色的。
The children’s of red node are black.
红色结点的孩子是黑色的。
All the root to external node paths contain same number of black nodes.
所有根到外部节点的路径都包含相同数量的黑色节点。
Example: Consider path 75-90-80-88-null and 75-40-30-null in both these paths 3 black nodes are there.
示例:在这两个路径中都考虑路径75-90-80-88-null和75-40-30-null,那里有3个黑色节点。
红黑树的优点 (Advantages of Red Black Tree)
Red black tree are useful when we need insertion and deletion relatively frequent.
当我们需要相对频繁地插入和删除时,红黑树很有用。
Red-black trees are self-balancing so these operations are guaranteed to be O(logn).
红黑树是自平衡的,因此保证这些操作为O(logn)。
They have relatively low constants in a wide variety of scenarios.
在多种情况下,它们的常数相对较低。
插入红黑树 (Insertion in Red black Tree)
Every node which needs to be inserted should be marked as red.
每个需要插入的节点都应标记为红色。
Not every insertion causes imbalancing but if imbalancing occurs then it can be removed, depending upon the configuration of tree before the new insertion is made.
并非每个插入都会导致不平衡,但是如果发生不平衡,则可以将其删除,具体取决于在进行新插入之前树的配置。
In Red black tree if imbalancing occurs then for removing it two methods are used that are: 1) Recoloring and 2) Rotation
在红黑树中,如果发生不平衡,则使用两种方法将其消除: 1)重新着色和2)旋转
To understand insertion operation, let us understand the keys required to define the following nodes:
要了解插入操作 ,请让我们了解定义以下节点所需的键:
Let u is newly inserted node.
令u为新插入的节点。
p is the parent node of u.
p是u的父节点。
g is the grandparent node of u.
g是u的祖父母节点。
Un is the uncle node of u.
Un是u的叔节点。
When insertion occurs the new node is inserted which is already a balanced tree. But after inserting many nodes there can be an imbalancing condition occurs which violates the property of the red black tree. So in this case first we try to recolor first then we go for a rotation.
发生插入时,将插入已经是平衡树的新节点 。 但是在插入许多节点之后,可能会出现不平衡状况,这会违反红黑树的属性。 因此,在这种情况下,我们首先尝试重新着色,然后进行旋转。
Case 1)
情况1)
The imbalancing is concerned with the color of grandparent child i.e. Uncle Node. If uncle node is red then there are four cases then in this, by doing recoloring imbalancing can be removed.
这种失衡与祖父母的孩子,即Node叔叔的肤色有关。 如果叔叔节点是红色的,那么有四种情况,通过重新着色可以消除不平衡。
1) LRr imbalance
1)LRr不平衡
In this Red Black Tree violates its property in such a manner that parent and inserted child will be in a red color at a position of left and right with respect to grandparent. Therefore it is termed as Left Right imbalance.
在这棵红黑树中,其侵犯其财产的方式是,父级和插入的子级在祖父母的左右位置将呈红色。 因此,它被称为左右不平衡。
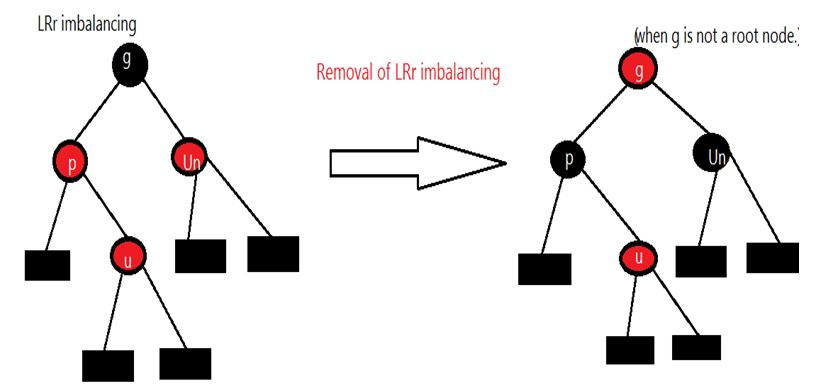
Removal of LRr imbalance can be done by:
可以通过以下方法消除LRr不平衡:
Change color of p from red to black.
将p的颜色从红色更改为黑色。
Change color of Un from red to black.
将Un的颜色从红色更改为黑色。
Change color of g from black to red, provided g is not a root node.
如果g不是根节点,则将g的颜色从黑色更改为红色。
Note: If given g is root node then there will be no changes in color of g.
注意:如果给定的g是根节点,则g的颜色不会发生变化。
2) LLr imbalance
2)LLr不平衡
In this red black tree violates its property in such a manner that parent and inserted child will be in a red color at a position of left and left with respect to grandparent. Therefore it is termed as LEFT LEFT imbalance.
在这棵红黑树中,其侵犯其性质的方式是,父级和所插入的子级在相对于祖父母的左右位置处将呈红色。 因此,它被称为LEFT LEFT不平衡。
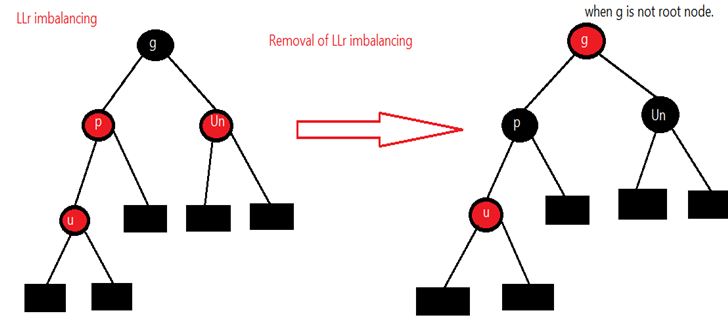
Removal of LLr imbalance can be done by:
可以通过以下方法消除LLr不平衡:
Change color of p from red to black.
将p的颜色从红色更改为黑色。
Change color of Un from red to black.
将Un的颜色从红色更改为黑色。
Change color of g from black to red, provided g is not a root node.
如果g不是根节点,则将g的颜色从黑色更改为红色。
Note: If given g is root node then there will be no changes in color of g.
注意:如果给定的g是根节点,则g的颜色不会发生变化。
3) RRr imbalance
3)RRr不平衡
In this red black tree violates its property in such a manner that parent and inserted child will be in a red color at a position of right and right with respect to grandparent. Therefore it is termed as RIGHT RIGHT imbalance.
在这棵红黑树中,其侵犯其财产的方式是,父母和所插入的孩子相对于祖父母在正确的位置和正确的位置将变成红色。 因此,它被称为RIGHT RIGHT不平衡。
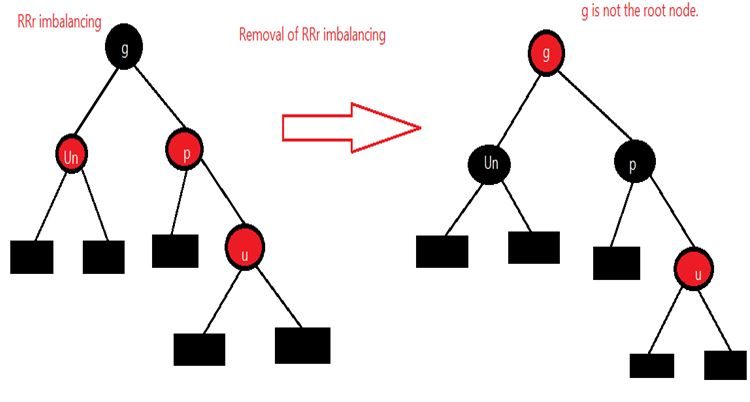
Removal of RRr imbalance can be done by:
可以通过以下方法消除RRr不平衡:
Change color of p from red to black.
将p的颜色从红色更改为黑色。
Change color of Un from red to black.
将Un的颜色从红色更改为黑色。
Change color of g from black to red, provided g is not a root node.
如果g不是根节点,则将g的颜色从黑色更改为红色。
Note: If given g is root node then there will be no changes in color of g.
注意:如果给定的g是根节点,则g的颜色不会发生变化。
4) RLr imbalance
4)RLr不平衡
In this red black tree violates its property in such a manner that parent and inserted child will be in a red color at a position of right and left with respect to grandparent. Therefore it is termed as RIGHT LEFT imbalance.
在这棵红黑树中,其侵犯其性质的方式是,父代和插入的子代在祖父母的左右位置将呈红色。 因此,它被称为右向不平衡。
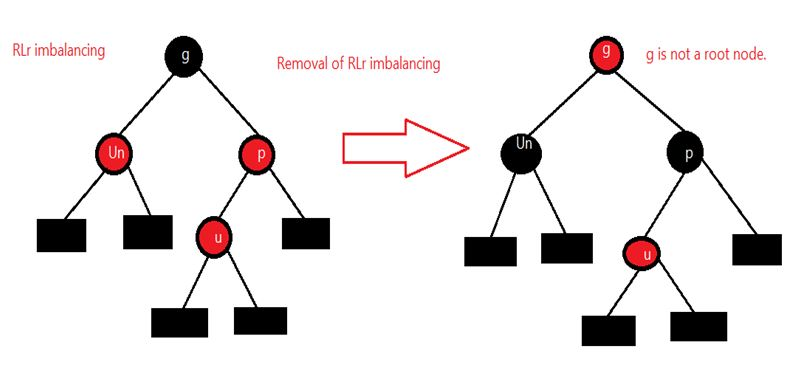
Removal of RLr imbalance can be done by:
可以通过以下方法消除RLr不平衡:
Change color of p from red to black.
将p的颜色从红色更改为黑色。
Change color of Un from red to black.
将Un的颜色从红色更改为黑色。
Change color of g from black to red, provided g is not a root node.
如果g不是根节点,则将g的颜色从黑色更改为红色。
Note: If given g is root node then there will be no changes in color of g.
注意:如果给定的g是根节点,则g的颜色不会发生变化。
Case 2)
情况2)
The imbalancing can also be occurred when the child of grandparent i.e. uncle node is black. Then know also four cases will be arises and in this case imbalancing can be removed by using rotation technique.
当祖父母的孩子(即叔叔节点)是黑人时,也可能发生不平衡。 然后知道还会出现四种情况,在这种情况下,可以使用旋转技术消除不平衡。
LR imbalancing
LR失衡
LL imbalancing
LL失衡
RR imbalancing
RR失衡
RL imbalancing
RL失衡
LL and RR imbalancing can be removed by following two steps i.e. are:
可以通过以下两个步骤来消除LL和RR不平衡 :
Apply single rotation of p about g.
绕g旋转p一次。
Recolor p to black and g to red.
将p重新着色为黑色,将g重新着色为红色。
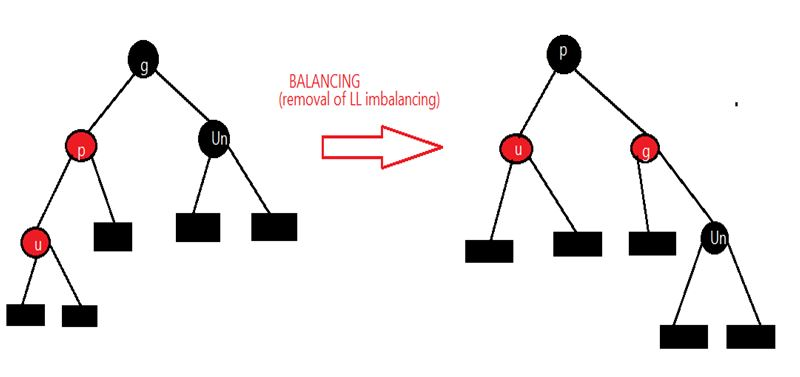
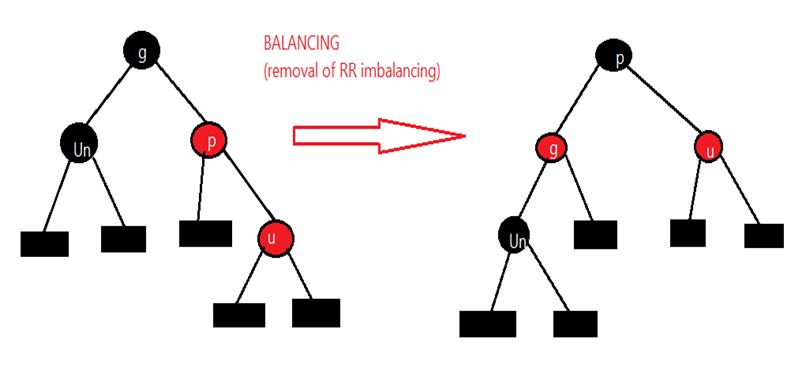
LR and RL imbalancing can be removed by following steps:
可以通过以下步骤消除LR和RL不平衡:
Apply double rotation of u about p followed by u about g.
将u旋转约p,然后再旋转约g。
For LR imbalancing recolor u to black and recolor p and g to red.
对于LR失衡,将u重新着色为黑色,将p和g重新着色为红色。
For RL imbalancing recolor u to black and recolor g and p to red.
对于RL失衡,将u重新着色为黑色,将g和p重新着色为红色。
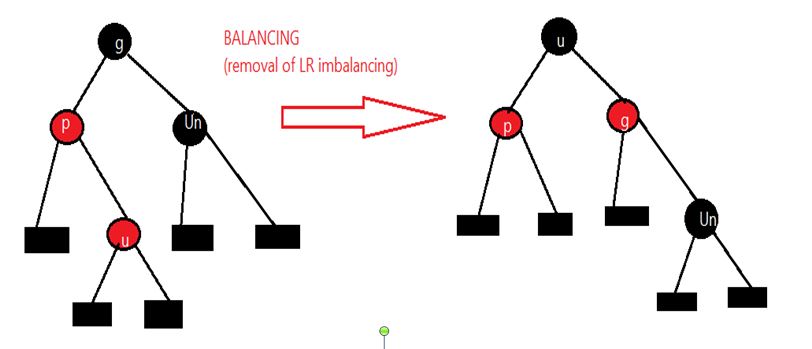
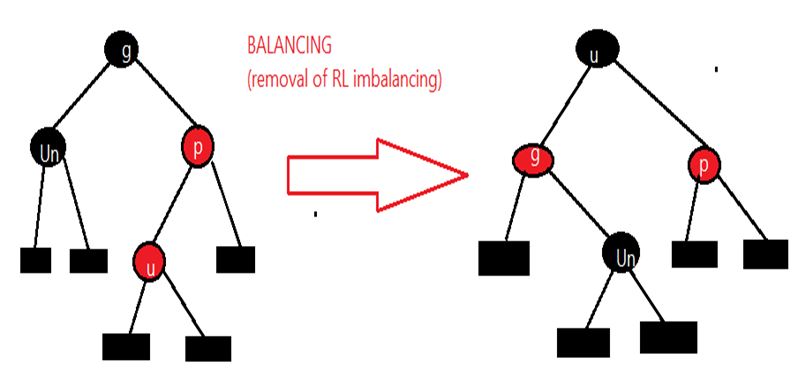
Note:
注意:
While inserting any node its color is by default red.
插入任何节点时,其颜色默认为红色。
If uncle node is NULL then it will be consider as black.
如果叔节点为NULL,则将其视为黑色。
翻译自: https://www.includehelp.com/data-structure-tutorial/red-black-tree.aspx
红黑树节点插入和旋转