Beacons are small requests that our apps make to report some information "home", to the server. Beacons are often used to report visitor stats, JS errors, performance metrics.
信标是我们的应用向服务器报告一些“家”信息的小要求。 信标通常用于报告访客统计信息,JS错误,性能指标。
Beacons often don't return any data back to the client, but some do.
信标通常不将任何数据返回给客户端,但是有些却可以。
使用范例 (Example use)
<div id="app">Awesome app is awesome</div>
<script>
// gather data somehow
var data = {
time: Date.now(),
pixelRatio: window.devicePixelRatio
};
// construct query string
var qs = Object.keys(data).map(function(key){
return encodeURIComponent(key) + '=' + encodeURIComponent(data[key]);
}).join('&');
// send beacon
new Image().src = '/stats.png?' + qs; // "/stats.png?time=1391976334544&pixelRatio=1"
</script>
衡量信标的性能 (Measuring the beacon's performance)
So now you want to know what's the preformance of the beacon - how long did it take to send the beacon out and get a response back.
因此,现在您想知道该信标的性能如何-将信标发送出去并获得回复需要花费多长时间。
Simplest thing - add onload
and onerror
(you'll see why) handlers:
最简单的事情-添加onload
和onerror
(您将看到原因)处理程序:
// reporting beacon perf
var reported = false;
var start = Date.now();
function reportBeaconPerf() {
if (reported) {
return; // bye
}
reported = true;
new Image().src = '/perf.png?beacon_took=' + (Date.now() - start);
// "/perf.png?beacon_took=34802"
}
// send beacon
var i = new Image;
i.onerror = i.onload = reportBeaconPerf;
i.src = '/stats.png?' + qs;
You use onload
and onerror
just in case you decide to return an empty "204 No Content" response from the stats.png
beacon. In this case the response is not a valid image, so some browsers may decide, understandably, to fire an error event.
如果决定从stats.png
信标返回空的“ 204 No Content”响应,则可以使用onload
和onerror
。 在这种情况下,响应不是有效的图片,因此可以理解,某些浏览器可能会决定触发错误事件。
需要更多性能数据! (Need more perf data!)
Time from declaring you want to make a beacon request (which may be different from the time the browser actually makes it) to the time you get an event back is cool. But you know what's cooler - getting more (and more accu-rat) data. E.g. DNS resolution, connect time, etc. One lump number doesn't tell you where you should focus to improve performance. You need details.
从宣告您要发出信标请求(可能与浏览器实际发出请求的时间不同)到您重新获得事件的时间都是很酷的事情。 但是您知道什么更酷-获取更多(和更多准确率)数据。 例如DNS解析度,连接时间等。一个总号并不能告诉您应该关注哪些方面来提高性能。 您需要详细信息。
And details you get, thanks to the resource timing API now present in many-a-user-agent. Resource timing is not the same as navigation timing, but the adoption looks promising (http://caniuse.com/#feat=nav-timing). Plus, you're not going to beacon every single time. And probably perf improvements targeted at one browser will help the others (that haven't heard of resource timing yet) too.
借助许多用户代理中现在提供的资源计时API,您可以获得详细信息。 资源计时与导航计时不同,但采用的前景看好( http://caniuse.com/#feat=nav-timing )。 另外,您不会每次都信标。 而且针对一种浏览器的性能改进可能也会对其他浏览器(尚未听说过资源计时)有所帮助。
Here's a nice intro to resource timing.
To see the magic in action:
要查看魔术效果:
In Chrome, go to http://phpied.com
在Chrome浏览器中,转到http://phpied.com
- open the console 打开控制台
type
类型
> var src = "http://phpied.com/favicon.ico?blah=blargh!"; > new Image().src = src;
type
performance.getEntries()
键入
performance.getEntries()
- Inspect the last entry in the array 检查数组中的最后一个条目
Alternatively, you can also use getEntriesByName()
, since you have the URL, e.g.
另外,您也可以使用getEntriesByName()
,因为您拥有URL,例如
performance.getEntriesByName(src)[0];
performance.getEntriesByName(src)[0];
You'll see something like:
您会看到类似以下内容:
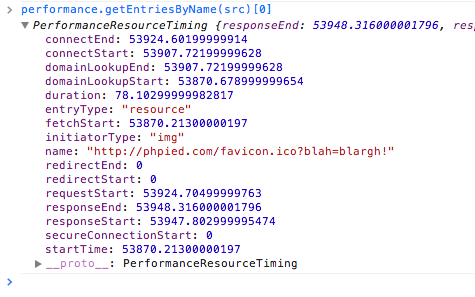
Pretty cool, huh? DNS resolution and all.
太酷了吧? DNS解析和所有。
All the values are relative to performance.timing.navigationStart
, in case you need absolute values.
如果需要绝对值,则所有值都与performance.timing.navigationStart
。
Same-origin restrictions apply, so if you're beaconning to a different domain, make sure the response adds the Timing-Allow-Origin: *
HTTP header, like so:
应用同源限制,因此,如果您要连接到其他域,请确保响应添加了Timing-Allow-Origin: *
HTTP标头,如下所示:
$ curl -I http://connect.facebook.net/en_US/all.js
HTTP/1.1 200
Cache-Control: public, max-age=1200
Content-Type: application/x-javascript; charset=utf-8
...
Timing-Allow-Origin: *
改善信标性能 (Improving beacon perf)
Now that you know how to measure the beacon perf, you can look at the data and see where you have room for improvements. Here are some pointers to get you started.
既然您知道了如何测量信标性能,就可以查看数据并查看有待改进的地方。 这里有一些提示可以帮助您入门。
Async.
异步。
new Image().src
is already async, but whatever the file where this JS code lives should be loaded asynchronously as well.
new Image().src
已经是异步的,但是无论此JS代码所在的文件是什么,也都应该异步加载。
Tiny response.
微小的React。
The smallest response body you can have is 1x1 GIF. PNG is superior format that yields smaller file sizes, but not when it comes to tiny images. The 1x1 image should be 42 bytes. Anything above this is a waste.
您可以拥有的最小响应主体是1x1 GIF。 PNG是一种高级格式,可产生较小的文件大小,但对于小图像则不会。 1x1图片应为42个字节。 高于此的任何都是浪费。
No response.
没有React。
Switch to a 204 response and make the body 0 bytes.
切换到204响应并使主体为0字节。
Respond, then log.
响应,然后登录。
It's better to send the header, and flush the output first, and then do your logging. That way the client isn't waiting for you to do your back end work.
最好发送标头,然后先刷新输出,然后再进行日志记录。 这样,客户就不再等待您完成后端工作。
Unless, of course, the response is dependent on back end work. In which case, back to the good old profiling of the craziness you're doing server-side. No network involved here.
当然,除非响应取决于后端工作。 在这种情况下,请回到您在服务器端进行的疯狂行为的良好分析。 这里没有网络。
Remove HTTP headers.
删除HTTP标头。
With the big axe. You may send tiny or 0-size response bodies, but what's the benefit if you send tons of headers? Cookie
header being the worst.
用大斧头。 您可以发送很小的或0尺寸的响应正文,但是如果发送大量的标头,有什么好处呢? Cookie
标头是最差的。
谢谢! (Thanks!)
Thanks for reading! Now go make your beacons faster!
谢谢阅读! 现在,使您的信标速度更快!
Also keep an eye on Philip's articles here - http://www.lognormal.com/blog/
也请在此处关注Philip的文章-http: //www.lognormal.com/blog/
And please come back and add to that list above with your discoveries.
然后,请返回并添加到上面的列表中,并添加您的发现。
Tell your friends about this post on Facebook and Twitter
在Facebook和Twitter上告诉您的朋友有关此帖子的信息